Spring Boot Interview Questions and Answers
In this article, we are going to discuss some of the Spring Boot interview questions and answers. This is a summary of some of the most important questions concerning the Spring Boot Framework, that you may be asked to answer in an interview! There is no need to worry about your next interview test because Java Code Geeks are here for you!
Spring Boot is currently the framework of choice for Java Enterprise application developers. As a Java web developer, it has become a mandatory requirement to know Spring Boot.
Table Of Contents
- 1. Basic Concepts
-
- 1.1. What is the difference between the Spring Framework and Spring Boot?
- 1.2. What is the difference between the Spring Framework and Spring Boot?
- 1.3. What are the underlying design patterns in Spring?
- 1.4. Explain the different bean scopes
- 1.5. How does Spring (Spring MVC) work? Explain the request processing flow.
- 1.6. Explain the different strategies used to handle the exception
- 1.7. What are the different modules in Spring Framework or explain Spring’s architecture
- 1.8. What is Aspect-Oriented Programming (AOP)?
- 2. Spring Boot Basics
- 3. Spring Boot Annotations
-
- 3.1. Mention some of the Spring Boot’s important annotations
- 3.2. What do you understand by @Qualifier and @Primary?
- 3.3. What does annotation @SpringBootApplication do?
- 3.4. Mention some of the Spring Boot’s test features
- 3.5. Explain Spring boot conditional annotations and give use cases to use them
- 4. Spring Boot Advanced Topics
-
- 4.1. What are Spring Boot starters?
- 4.2. What is actuator and how do you configure?
- 4.3. What is Spring Boot CLI and how do you use it?
- 4.4. What do you understand by auto-configuration? How can you write a custom auto-configuration?
- 4.5. What is Spring Data REST?
- 4.6. Difference between conditional annotation and profiles
- 4.7. How do you achieve transaction management?
- 4.8. Mention some of the configuration parameters available in Spring Boot
- 5. Conclusion
1. Basic Concepts
In this section, we are going to see some of the interview questions related to basic knowledge on Spring/Spring Boot that may be asked in the interview.
1.1 What is the difference between the Spring Framework and Spring Boot?
Spring Boot is built on top of Spring Framework. Below are the differences between Spring and Spring Boot,
Spring | Spring Boot |
---|---|
Spring is built keeping flexibility as the focus. However, it needs a lot of configuration to be done around to integrate with other frameworks. | Spring Boot is built to keep the configuration hassle less. Spring boot starters provide basic weaving and developers can focus more on solving business problems. |
Spring lacks auto-configuration support | Spring Boot comes with auto-configuration, it tries to automatically configure the application based on the jars added |
Developers have to configure we server themselves | Spring Boot comes with an embedded web server and developers don’t have to worry about configuring their local development environment |
1.2 What do you understand by Inversion of Control?
Inversion of Control or Dependency Injection is a technique in software engineering where an object or container can provide the dependencies of another object. In traditional programming, we use the new
operator to create an instance. If the classes’ construct (Additional constructor parameters), all the classes making use of it have to be changed. If we pass on the control of creating an object to a central container then only that part is affected all the changes are at one single place. This way Inversion of Control allows us to have loosely coupled classes.
1.3 What are the underlying design patterns in Spring?
Spring is a very mature framework and below are the different design patterns used in Spring,
- Dependency Injection or Inversion of Control – we have discussed it in the previous question
- Factory Pattern – Spring container uses the factory pattern to construct the different Spring beans
- Proxy Pattern – In proxy pattern a class is used to represent the functionality of another class. This pattern is widely used in AOP
- Singleton Pattern – This pattern ensures that only one instance of the object exists in the application. By default beans in Spring Container is a singleton.
- Front Controller Pattern – This pattern centralizes request handling.
- Template Method Pattern – Spring Framework provides templates for many commonly used integrations like JDBC, JMS, JPA and so on, lets programmers complete the actual implementation.
I suggest you read up more on the design patterns to be able to answer any follow-up question.
1.4 Explain the different bean scopes
In the Spring bean scope can be defined using @Scope
annotation. Below are the different bean scopes supported,
Scope | What it means |
---|---|
Singleton | Single bean instance per Spring IoC container. This is the default scope |
Prototype | A new instance of the bean is created each time a bean is requested |
Request | A single instance of the bean will be created and available throughout the HTTP request lifecycle. This scope is applicable only in Spring Web Application. |
Session | A single instance of the bean will be created and available throughout the HTTP session lifecycle. This scope is applicable only in Spring Web Application. |
Application | A single instance of the bean will be created and available throughout the lifecycle of a ServletContext . This scope is applicable only in Spring Web Application. |
Websocket | A single instance of the bean will be created and available throughout the lifecycle of a WebScocket . This scope is applicable only in Spring Web Application. |
1.5 How does Spring (Spring MVC) work? Explain the request processing flow.
Dispatcher Servlet is the heart of Spring Application. It abstracts away mapping of HTTP requests to processing classes, parsing of requests and generation of responses. Dispatcher servlet looks up to context configuration for the bean declarations. It sets up all supporting beans like Handler Mapping, View Resolvers, Local Context and so on. Based on the configuration it dispatches the request to different controllers. It is responsible for writing the response back by making use of view templates.
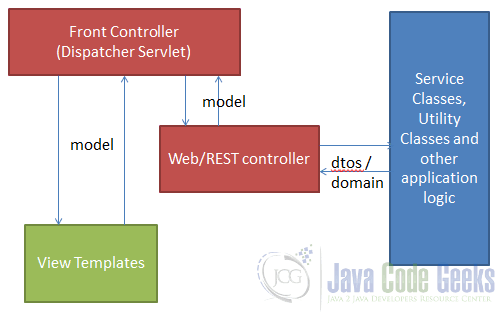
1.6 Explain the different strategies used to handle the exception
To manage Exceptions Spring offers below classes,
ExceptionHandlerExceptionResolver
– generic exception handlerDefaultHandlerExceptionResolver
– handler to handle set of predefined exceptionsSimpleMappingExceptionResolver
– exception handler to map custom exceptions to error pages. Existing as part of the framework since 2003.
Below are the different strategies to handle exceptions in Spring
Return an error message to be displayed – This is the simple way of handling exception. The exception handle method is responsible for returning the appropriate error message. Below is the code snippet
@RequestMapping(value = "/doSomething") public String doSomething() { throw new NullPointerException("some message"); } @ExceptionHandler(NullPointerException.class) @ResponseBody public String handleException(NullPointerException.class) { return ex.getMessage(); }
Return a dedicated error page – This strategy lets us return a generic error page and hides all the exceptions from the end-user. Use the same annotation @ExceptionHandler
, from the method return the error page.
Return custom error message and code – A custom error code and message can be return from the exception handler method. This can be done by adding response status and reason to the annotation @ResponseStatus
.
Redirect to custom error page with a custom message – This uses real HTTP redirect to navigate to error page.
Exception handling with @ControllerAdvice
– This allows you to centralize the exception handling across the application not just at the controller level. You can read up Spring documentation to understand more about Controller advice.
1.7 What are the different modules in Spring Framework or explain Spring’s architecture
Below is the Spring architecture in simple form,
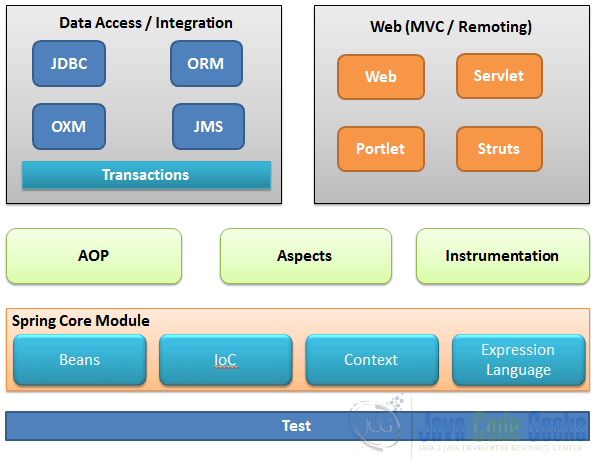
- Spring Core – this makes the fundamental parts of the framework and supports core components like container, expression language, beans
- Spring web – enables web applications and provides support for Struts, Portlets, Servlets
- Data Access – provides the basic infrastructure to perform data access. It supports JDBC, JPA and popular ORMs
- AOP – provides infrastructure support for Aspect Oriented Programming and integration with AspectJ
You can read up more in the article.
1.8 What is Aspect-Oriented Programming (AOP)?
AOP provides a paradigm to handle cross-cutting concerns. It increases the modularity of the application. AOP involve below terminologies,
- Business Object – This is a normal application class containing the business logic
- Aspect – an aspect is a cross-cutting concern. For example, logging across the application.
- Joinpoint – In spring it represents the method execution
- Advice – advice is the action taken by the aspect at a joinpoint
- Pointcut – predicate to match advice to be applied by an aspect at a particular joinpoint
This is at a very high level. Go through the Spring’s documentation to learn more about Aspect-Oriented Programming.
2. Spring Boot basics
2.1 Why Spring Boot is so popular or what are the features offered by Spring Boot?
Spring Boot is an opinionated framework built on top of Spring. Its main aim is to reduce the boilerplate configuration in Spring. By doing so you can get started quickly and focus more on writing the business logic. Some of the other features which make it popular are as below,
- We can now build a standalone application with Spring Boot
- Embedded webserver (Tomcat, Jetty). Makes development environment setup easy
- Starter poms simplify the maven configuration and dependencies are handled in the parent pom
- Production-ready health and metrics services through the actuator
2.2 What does parent pom mean?
Parent pom helps to remove duplicates or the redundancies in the configuration using inheritance of pom files. Parent POM file constitutes,
- Common Data (Developer name, SCM address, Distribution ..etc)
- Version rand any other constants
- Common resources and configurations
- Common dependencies
3. Spring Boot annotations
3.1 Mention some of the Spring Boot’s important annotations
Spring Boot virtually has removed XML based configuration. It encourages us to use more and more annotations. Some of the important annotations are as follows,
@SpringBootApplication
@EnableAutoConfiguration
@Conditional
@Qualifier
@ComponentScan
@Controller
and@RestController
- Regular Spring Framework and JPA annotations
You can read more on Spring/ Spring Boot annotations in this article.
3.2 What do you understand by @Qualifier
and @Primary
?
Spring Framework throws NoUniqueBeanDefinitionException
when there is more than one bean of the same type is available in the container. When we have multiple implementations of an interface and we try to use it by using interface name, we encounter this problem. It can be resolved using @Qualifier
annotation.
By including @Qualifier
annotation together with the name of the implementation (@Qualifier("someBean")
) we want to use we can avoid ambiguity.
@Primary
annotation can be used to specify which bean implementation to use by default whenever there is any ambiguity.
3.3 What does annotation @SpringBootApplication
do?
This annotation is used on the main class to enable quite a lot of other annotations like,
@Configuration
– enables java-based Spring configuration@ComponentScan
– enable component scanning@EnableAutoConfiguration
– enables Spring Boot’s autoconfiguration.
@SpringBootApplication
combines the above annotations and readies up the Spring Boot application.
3.4 Mention some of the Spring Boot’s test features
Spring Boot has improved testing with number of annotations and utilities. Spring boot test support is provided by two modules spring-boot-test
(contains core items) and spring-boot-test-autoconfiguration
. The dependency spring-boot-starter-test
imports both of these modules.
All JUnit annotations are valid to write unit tests. @SpringBootTest(webEnvironment=WebEnvironment.RANDOM_PORT)
test runs the controllers on a random port in a fully qualified spring container and lets us write integration tests. To inject specific port use @LocalServerPort
annotation.
Use @WebMvcTest
to auto-configure the Spring MVC infrastructure and lets us test the Spring MVC controllers.
@JpaDataTest
can be used to test the JPA applications or repository classes.
3.5 Explain Spring boot conditional annotations and give use cases to use them
Spring 4.0 introduced @Conditional
annotation and it enables conditional checking for bean registration. Spring Boot has simplified the @Conditional annotations by providing several predefined implementations. For example,
@ConditionalOnBean
and@ConditionalOnMissingBean
– allows configuration to be skipped based on the presence or absence of a specific bean-
@ConditionalOnClass
and@ConditionalOnMissingClass
– allows configuration to be skipped based on the presence or absence of a specific class @ConditionalOnProperty
– allows configuration to be included based on a Spring environment property@ConditionalOnResource
– allows configuration to be included only if a specific resource is present@ConditionalOnWebApplication
and@ConditionalOnNotWebApplication
– allow configuration to be included based on whether it is a web application or not a web application@ConditionalOnExpression
– allows configuration to be skipped based on an expression
These annotations are useful when some beans have to be included for testing or some beans should not be included in the production environment.
4. Spring Boot advanced topics
4.1 What are Spring Boot starters?
In a complex Spring application, dependency management is quite critical and doing it manually is error-prone. Spring Boot starters are built to address this issue. Starter POMs come as a savior and they manage all the dependencies, library versions are automatically taken care of.
Web starter redies up the all the required dependencies for Spring Web application.
Test starter used to provide the Spring test dependencies. It enables us to use JUnit, Hamcrest, and Mockito.
Data JPA Starter lets us test the JPA classes and repositories.
Apart from these there are several other starters that really reduce the boilerplate configuration required around the library integrations.
4.2 What is actuator and how do you configure?
This module of Spring boot provides production-ready monitoring features. The simple way to enable spring-boot-actuator
is to include spring-boot-actuator-stater
.
Actuator endpoints let you monitor and interact with your application. Who can access the actuator endpoints can be controlled by application security configurations. Spring Boot Actuator endpoints can be exposed either on JMX or HTTP.
Some examples to actuator endpoints are,
Endpoint | Description |
---|---|
auditevents |
Provides audit information of the application and it requires an AuditEventsRepository bean |
beans |
Displays a complete list of available beans in the application |
conditions |
Lists conditions executed on Configuration and AutoConfiguration |
health |
Shows application health information |
flyway |
Shows flyway database migrations that have been applied |
sessions |
Allows retrieval and deletion of sessions |
shutdown |
Allows to gracefully shutdown the application |
There are many other actuator endpoints. Read through Spring documentation to gain more understanding.
4.3 What is Spring Boot CLI and how do you use it?
The Spring Boot CLI is a command-line interface for the Spring Boot application. Spring Boot CLI is the fastest way to create Spring application. Some of the features offered by Spring Boot CLI are as below,
- Provides an interface to run and test the Spring Boot application from the command line
- It internally uses Spring Boot Starter and Spring Boot AutoConfiguration
- It resolves all the dependencies automatically
- It has support for Groovy
4.4 What do you understand by auto-configuration? How can you write a custom auto-configuration?
Auto-configuration is one of the important features offered by Spring Boot as compared to Spring. It tries to automatically configure the application based on the jar dependencies added in the application. If HSQL DB is in the classpath and it isn’t configured manually by you, then Spring Boot tries to configure an in-memory database for you.
To create custom auto-configuration,
- Create custom class annotated with
@Configuration
- Register class as an auto-configuration candidate by adding the class under key
org.springframework.boot.autoconfigure.EnableAutoConfiguration
in the fileresources/META-INF/spring.factories
. You can specify conditions using annotations to indicate include auto-configuration only when the bean definition is missing. - If we want to disable auto-configuration then add the annotation
@EnableAutoConfiguration
with excludes attribute containing auto-configuration to exclude.
4.5 What is Spring Data REST?
Spring Data REST makes it easy to build REST services on top of Spring Data Repositories. It exposes hypermedia driven HTTP resources on top of the application’s entity model classes. The dependency spring-boot-starter-data-rest
enables Spring Data REST. Spring Data REST itself is a Spring MVC application and it can be integrated with an existing application with a little effort.
4.6 Difference between conditional annotation and profiles
Both conditional annotations and profiles can be used to conditionally configure spring beans.
@Profile
annotation can be used to check the conditions based on the environment only. Different application configurations can be loaded based on the environment.
@Conditional
is more generalized and gives more fine-grained control over the bean configuration.
4.7 How do you achieve transaction management?
Most of the enterprise applications are backed by a database and store one or the other sorts of data. A database transaction is the single unit of logical work that access or modify the data.
Transaction management can be achieved by either using annotation @Transactional
or by configuring AOP.
To achieve transaction management using annotation,
- Add
@EnableTransactionManagement
annotation to the configuration class (If we have spring-data or spring-tx dependency in our CLASSPATH spring boot does it for us) - Now it lets us use
@Transactional
annotation either at the class level or at the method level - Further, we can customize timeout, rollback strategies, read-only flag ..etc for a single transaction
The @Transactional
works by creating proxy of your class and intercepting the annotated method. When you call the annotated method within the same class in another method, the transaction doesn’t work as it bypasses the proxies. This can be eliminated by setting up transaction management using AOP.
4.8 Mention some of the configuration parameters available in Spring Boot
Spring boot lets us specify various configuration properties either in the application.properties
file or in application.yml
file or through the command line.
We can configure log levels, server ports, actuator endpoints, cache, database parameters, JMX and so on. It offers a long list of configurations and I recommend you to go through the Spring documentation to have a good understanding.
5. Conclusion
In this article, we have seen some of the Spring and Spring Boot interview questions. You can gather more information by going through the Spring Documentation.
Ok, so now you are ready for your interview! Don’t forget to check our Examples dedicated subsection!
If you enjoyed this, then subscribe to our newsletter to enjoy weekly updates and complimentary whitepapers! Also, check out our courses for more advanced training!
You are welcome to contribute with your comments and we will include them in the article!