java.io.FileNotFoundException – How to solve File Not Found Exception (with video)
In this tutorial, we will discuss how to solve the java.io.FileNotFoundException – FileNotFoundException
in Java. This exception is thrown during a failed attempt to open the file denoted by a specified pathname.
Also, this exception can be thrown when an application tries to open a file for writing, but the file is read-only, or the permissions of the file do not allow the file to be read by any application.
You can also check this tutorial in the following video:
This exception extends the IOException
class, which is the general class of exceptions produced by failed or interrupted I/O operations. Also, it implements the Serializable
interface and finally, the FileNotFoundException
exists since the first version of Java (1.0).
1. The FileNotFoundException in Java
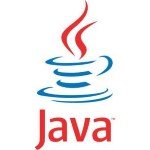
The following constructors throw a FileNotFoundException
when the specified filename does not exist: FileInputStream
, FileOutputStream
, and RandomAccessFile
. These classes aim to obtain input bytes from a file in a file system, while the former class supports both reading and writing to a random access file.
The following snippet reads all the lines of a file, but if the file does not exist, a java.io.FileNotFoundException is thrown.
FileNotFoundExceptionExample.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | import java.io.BufferedReader; import java.io.File; import java.io.FileReader; import java.io.IOException; public class FileNotFoundExceptionExample { private static final String filename = "input.txt" ; public static void main(String[] args) { BufferedReader rd = null ; try { // Open the file for reading. rd = new BufferedReader( new FileReader( new File(filename))); // Read all contents of the file. String inputLine = null ; while ((inputLine = rd.readLine()) != null ) System.out.println(inputLine); } catch (IOException ex) { System.err.println( "An IOException was caught!" ); ex.printStackTrace(); } finally { // Close the file. try { rd.close(); } catch (IOException ex) { System.err.println( "An IOException was caught!" ); ex.printStackTrace(); } } } } |
In case the file is missing, the following output is produced:
An IOException was caught! java.io.FileNotFoundException: input.txt (No such file or directory) at java.io.FileInputStream.open(Native Method) at java.io.FileInputStream.(FileInputStream.java:146) at java.io.FileReader.(FileReader.java:72) at main.java.FileNotFoundExceptionExample.main(FileNotFoundExceptionExample.java:16) Exception in thread "main" java.lang.NullPointerException at main.java.FileNotFoundExceptionExample.main(FileNotFoundExceptionExample.java:30)
The following snippet tries to append a string at the end of a file. If the file does not exist, the application creates it. However, if the file cannot be created, is a directory, or the file already exists but its permissions are sufficient for changing its content, a FileNotFoundException
is thrown.
FileNotFoundExceptionExample_v2.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | import java.io.BufferedWriter; import java.io.File; import java.io.FileWriter; import java.io.IOException; public class FileNotFoundExceptionExample_v2 { private static final String filename = "input.txt" ; public static void main(String[] args) { BufferedWriter wr = null ; try { // Open the file for writing, without removing its current content. wr = new BufferedWriter( new FileWriter( new File(filename), true )); // Write a sample string to the end of the file. wr.write( "A sample string to be written at the end of the file!\n" ); } catch (IOException ex) { System.err.println( "An IOException was caught!" ); ex.printStackTrace(); } finally { // Close the file. try { wr.close(); } catch (IOException ex) { System.err.println( "An IOException was caught!" ); ex.printStackTrace(); } } } } |
If the file exists and is a directory, the following exception is thrown:
An IOException was caught! java.io.FileNotFoundException: input.txt (Is a directory) at java.io.FileOutputStream.open(Native Method) at java.io.FileOutputStream.(FileOutputStream.java:221) at java.io.FileWriter.(FileWriter.java:107) at main.java.FileNotFoundExceptionExample_v2.main(FileNotFoundExceptionExample_v2.java:16) Exception in thread "main" java.lang.NullPointerException at main.java.FileNotFoundExceptionExample_v2.main(FileNotFoundExceptionExample_v2.java:28)
If the file exists, but it doesn’t have the appropriate permissions for writing, the following exception is thrown:
An IOException was caught! java.io.FileNotFoundException: input.txt (Permission denied) at java.io.FileOutputStream.open(Native Method) at java.io.FileOutputStream.(FileOutputStream.java:221) at java.io.FileWriter.(FileWriter.java:107) at main.java.FileNotFoundExceptionExample_v2.main(FileNotFoundExceptionExample_v2.java:16) Exception in thread "main" java.lang.NullPointerException at main.java.FileNotFoundExceptionExample_v2.main(FileNotFoundExceptionExample_v2.java:28)
Finally, the aforementioned exception can occur when the requested file exists, but it is already opened by another application.
2. How to deal with the java.io.filenotfoundexception
- If the message of the exception claims that there is no such file or directory, then you must verify that the specified is correct and actually points to a file or directory that exists in your system.
- If the message of the exception claims that permission is denied then, you must first check if the permissions of the file are correct and second, if the file is currently being used by another application.
- If the message of the exception claims that the specified file is a directory, then you must either alter the name of the file or delete the existing directory (if the directory is not being used by an application).
Important: For those developers that use an IDE to implement their Java applications, the relative path for every file must be specified starting from the level where the src
directory of the project resides.
3. Download the Eclipse Project
This was a tutorial about the FileNotFoundException
in Java.
The Eclipse project of this example: java.io.FileNotFoundException – How to solve File Not Found Exception
Last updated on Oct. 12th, 2021
have weird character in my directory/path as String”E: estilename.png”during runtime and get a ioexception file not found error.
How do I set the relative path of the file