Java Tutorial for Beginners (with video)
Welcome to this Java Core introductory tutorial for beginners! We will show you a java programming tutorial programming languages like Java are composed of syntax
and instructions
written by humans and performed by computers.
1. Java Tutorial – Introduction
In this core java tutorial for beginners, we’ll introduce the Java Programming Language, we’ll see where we can use it and what are the different types of Java applications. Finally, we’ll explain the simplest java starter program
: The famous Hello World Java example, in addition to other very basic programs
.
You can also check our video about java tutorial for beginners:
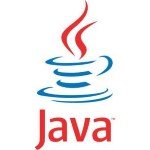
2. What is Java?
Java is one of the most popular programming languages
and platforms
. Released by Sun Microsystems in 1995 by James Gosling known as “The father of Java” with other team members. It was later acquired by the Oracle Corporation.
Java is a high level, simple, object-oriented, portable, robust, and very secure language. Java is loved by people because it’s guaranteed to be “write once, run everywhere” meaning that the written code runs in any platform (Windows, macOS, Mobile…etc.)
2.1 What makes Java particularity?
Java particularity is most importantly shown by these points:
- Object Oriented − In Java, everything is an
Object
. See OOPS-Concepts. - Portable – Java is compiled into
bytecode
which is interpreted by the Virtual Machine (JVM) on whichever platform it is being run on. - Simple − Java is easy to learn.
- Secure – Java compiles all the code into bytecode which is not readable by humans. In addition, Java enables to development of virus-free and tamper-free systems and uses public-key encryption for Authentication techniques.
- Robust − Java has a strong memory management system that helps eliminate errors during
compile
time error checking and runtime checking.
2.2 What is Java Platform?
Java Platform is a collection of hardware or software that helps programmers to efficiently develop and run Java applications. It includes a runtime environment (JRE) and a set of libraries (Java API).
2.3 What is JVM?
Java Virtual Machine (JVM) is a part of the Java Run Environment (JRE). Java compiler produces code for the Java Virtual Machine and the JVM runs it.
3. Where can we use Java Applications?
Java is used everywhere. Since it’s secured and reliable, it’s widely used for developing Java desktop applications (Ninety-seven percent of the world’s enterprise desktops), web apps, banking applications, embedded systems, data centers, games (such as Minecraft), Robotics, billions of cell phones apps, IDE (such as Eclipse, Netbeans and Intellij), etc.
4. What are the different types of Java Applications?
You can find mainly 6 types of Java applications :
- Standalone Application: This is the traditional desktop application that we need to install on every machine. Examples: Acrobat reader, Media player, ThinkFree, antiviruses, etc. The modern way to develop a desktop application in Java is using JavaFX.
- Web Application: This is a collection of web components (such as Java Servlets, JSP pages) and frameworks (such as Spring, JSF, GWT, Struts, Hibernate…etc.) used to create dynamic websites. These java web technologies are used to develop E-commerce platforms, government web apps, healthcare web apps, banking web apps…etc.
- Enterprise Applications: These are Java applications written for enterprises; the leading technology here is Java EE which is composed of a set of APIs (EJB, JMS, JPA, JTA, etc.) It has advantages of high-level security, load balancing, and clustering.
- Web services: The java web services let your Java application interact with other applications developed in another language (PHP, .NET, Etc.). The RESTfull services are created using Spring MVC. You can find other types of web services (SOAP for example).
- Big Data technologies: Java is used in Hadoop, ElasticSearch, and other big data technologies. There are other dominating Big data technologies like MongoDB, which is written in C++.
- Android Application: Java is an official language of Android development. Almost any app on your Android mobile is actually written in Java programming language: Messaging, phone call, Agenda, Calculator…etc.
5. Java basic examples
Our examples in this core Java tutorial are easy to learn. So, happy learning!
5.1 How to create your first Java Program?
Certainly our first java program will be the traditionnel and the simplest program called “HelloWorld” that outputs on the screen: “Hello, World!”.
In our examples, we’ll use Intellij IDEA IDE. You can use instead Eclipse or NetBeans.
Before we start, it’s important to understand that Java programs are composed of pieces called Classes
which include other smaller pieces called methods
(or functions
). These methods
perform tasks and return
information.
Firstly, open your prefered IDE. After that, create a new Java project called HelloWorld
. Add a new Java class
named: HelloWorld.java
and write inside the code
below:
class HelloWorld { }
Well done! you’ve just created your first Java class
! As you can see, we have named the file
the same as the class
name. This should be always respected in Java Programs.
Let’s explore what we’ve written:
The word class
is a Java keyword
(also called reserved words
) used to say that what is coming next is a Java class
. It should always be written with lowercase letters and is immediately followed by the class
name
. The name
can be anything but should respect the Java naming conventions.
Every class
and every method in Java begins with opening braces {
and ends with matching closing braces}
.
So this is your first Java program
, but in order to run it, we need to add a method
.
Let’s write this method
called main
inside our HelloWorld
Java Class
:
public class HelloWorld { public static void main(String[]args){ System.out.println("Hello, World!"); } }
Prints:
Hello, World!
We know that class is the keyword that declares a class in Java. Let’s understand what the other keywords consist of:
Public: this keyword is used to say that our “main” method is visible to all the other classes in the program. It’s an access specifier.
Static
: This keyword is used to make our main
method
static
meaning that our method
is called by the JVM without creating an object
for our HelloWorld class
. We do not need to create an object
for static
methods
to run. They can run themselves.
Void
: This keyword
represents the return type
. Void
means that our main
method
will not return
anything.
The main()
method
is the most important method
in a Java Program
. This is the entry point
method
from which the JVM can run your program. Therefore all your logic must be inside the main()
method
. A Java Program without a main()
method
causes a compilation
error
.
String [] args
: This consists of an array
. Its type
is: String
and its name
is: args
. It’s used for command-line arguments
that are passed as strings
.
System.out.println
: This is a function
used to print something on the console.
Now, the program is ready to be run. If you want to know How to Compile and Run this simple Java HelloWorld Program, please refer to our tutorial.
5.2 Other core Java basic examples:
First thing first we gonna talk about variables
.
After that, we gonna use our first project Hello World to add some new lines of code
.
So, what is a variable
? A variable
is a place in your computer memory like a box that you can store something in. You can have hundreds and thousands of boxes (variables
) in Java where you will store information about your program
. Variables
make the program very useful. But in Java, we need to define the data type
of the variable
in order to use it. The very basic data type
is int
short for Integer.
Integer in math numbers is a whole number and it’s the same thing in Java. So, in Java, we have to define first the data type
of the variable
followed by the name
of the variable
. Finally, we should assign a value
to that variable by using the equal =
sign. Let’s see that in action, go to your HelloWorld.java class
and add this line of code to your program:
int firstNumber=2;
Now, your program looks like below:
public class HelloWorld { public static void main(String[]args){ System.out.println("Hello, World!"); int firstNumber=2; } }
In order to print that variable
to the Console, remember which java function
we should use? Well done! It’s the System.out.println()
function
. But, instead of printing the phrase in double-quotes “Hello, world!” that represents a String
(another Java basic data type), we will print the content of the variable
firstNumer
. Can you figure out what we’ll do? Very good, we’ll just put the name
of our variable
between the two brackets of the function
without adding the double-quotes. Remember that using the double- quotes indicates to Java that the data type
used is a String
:
System.out.println(firstNumber);
Now, your program looks like this:
public class HelloWorld { public static void main(String[]args){ System.out.println("Hello, World!"); int firstNumber=2; System.out.println(firstNumber); } }
Run your program, you can see printed in your console:
Hello, World! 2
The good thing about variables
is that we can change their value
, just like boxes, we can replace their content. In other words, we can assign a number
to our variable
firstNumber
then change it and assign another number
to it. Let’s try it out:
public class HelloWorld { public static void main(String[]args){ System.out.println("Hello, World!"); int firstNumber=2; System.out.println(firstNumber); //We replace 2 by 7 in our variable firstNumber firstNumber=7; System.out.println(firstNumber); } }
Run again your program, you can see printed in your console:
Hello, World! 2 7
We can also put in our variable
a mathematical expression, just replace the value 7 by 7+4, run your program again then take a look at your console.
public class HelloWorld { public static void main(String[]args){ System.out.println("Hello, World!"); int firstNumber=7+4; System.out.println(firstNumber); } }
You should see printed the result of the addition 7+4:
Hello, World! 11
That is because Java calculates the mathematical expression
7+4 then replace it with its result into the memory location of the variable
containing that expression
.
You can write a longer mathematical expression
, use brackets
and do more complex operations
: (7+4)+(5*9);
public class HelloWorld { public static void main(String[]args){ System.out.println("Hello, World!"); int firstNumber=(7+4)+(5*9); System.out.println(firstNumber); } }
This program will print:
Hello, World! 56
Now, add a second variable
of type int
. Name it: secondNumber
and assign to it the value: 9. Finally, print it out!
int secondNumber=9; System.out.println(secondNumber);
Okay, you can say you’ve started programming in Java. The complete program looks like below:
public class HelloWorld { public static void main(String[]args){ System.out.println("Hello, World!"); int firstNumber=7; System.out.println(firstNumber); int secondNumber=9; System.out.println(secondNumber); } }
Add a third variable
of type int
named result
. Assign to it the addition of the two other variables
:
int result= firstNumber+secondNumber;
Now, if we want to print each variable
name
with its value, what do you think we should do? Try this line of code:
System.out.println("firstNumber");
Prints:
firstNumber
As you can see in this core java programming tutorial, now that we have put our variable
firstNumber
between double-quotes, Java will interpret it as a String
and no more as a variable
. Therefore, if we want to print the name
of our variable
followed by its value, we should print: “firstName”+firstName
Here the +
sign is used to concatenate
the String
“firstName”
and the int
value
of the variable
firstName
.
Focus on these lines below and try to guess what will they print:
public class HelloWorld { public static void main(String[]args){ System.out.println("Hello, World!"); int firstNumber=7; int secondNumber=9; int result= firstNumber+secondNumber; System.out.println("My firstNumber is:"+firstNumber); System.out.println("My secondNumber is:"+secondNumber); System.out.println("My result= firstNumber+secondNumber="+result); System.out.println("The double of my result="+result*2); }
These lines will print:
Hello, World! My firstNumber is:7 My secondNumber is:9 My result= firstNumber+secondNumber=16 The double of my result=32
6. Summary
In this article, we covered a core java programming tutorial, where we can use it, its applications then concluded our article by the famous Hello World program and some other Java basic examples.
Just remember that:
• JVM is the Java Virtual Machine. It’s the engine that runs the Java Code. It converts Java bytecode into native machine language. If you don’t have a JVM installed on your machine, how to install JVM.
• “Java is a programming language as well as a Platform”.
• Every Java Program needs a main() method to be executed: It’s the Entry Point.
• Every java application(program) must have at least one class.
7. More articles
- Best Way to Learn Java Programming Online
- Java Hello World Example
- 150 Java Interview Questions and Answers
- What is Java used for
- Java Constructor Example
- For Each Loop Java 8 Example
- Simple while loop Java Example
- Printf Java Example
- Java Set Example
- Java Collections Tutorial
- Polymorphism Java Example
- Try Catch Java Example
- Java Stack Example
- Java Queue Example
- Java Switch Case Example
- Java Map Example
- Java API Tutorial
- java.util.Scanner – Scanner Java Example
- Java List Example
- Java Array – java.util.Arrays Example
- ArrayList Java Example – How to use arraylist
8. Download the Source Code
That was a core java programming tutorial for beginners.
You can download the full source code of this example here: Java Tutorial for Beginners
Last updated on Apr. 26th, 2021