Java List Example
1. List Interface
In this post, we feature a comprehensive article on Java List. We are going to check in-depth the java.lang.List
in Java. We are also going to see some methods that are declared in the Collection interface which allow you to add an element, sort it, find the size, and remove an element (or elements) from a list collection.
2. List in Collection Hierarchy
There are four concrete implementation classes in for List
interface in java. List
interface extends the Collection
interface for some common operation to be supported by all implementation classes with iterator capability. Here is how all List
implemented classes fit into the collection class hierarchy.
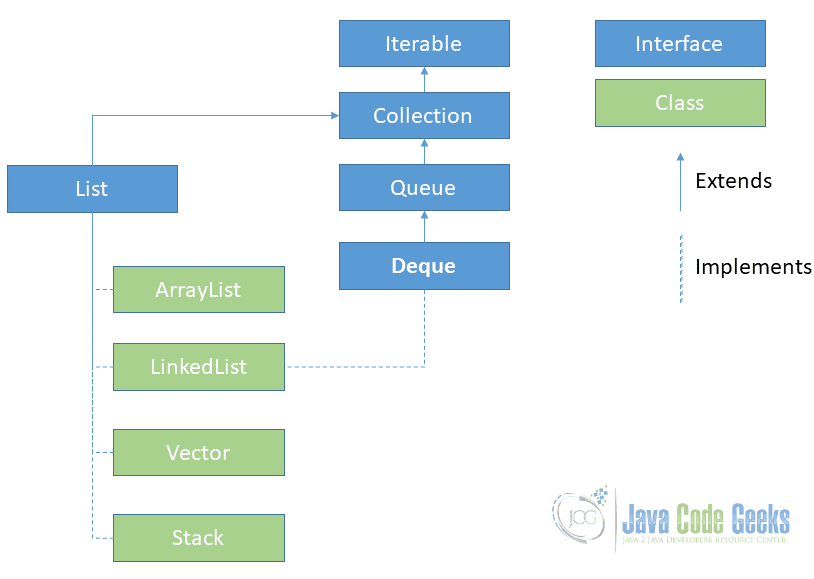
You can also check the ArrayList Java Example – How to use arraylist in the following video:
2.1 Iterable Interface
This is the top-most interface in the collection hierarchy. Implementing this interface allows the object to be the target of the for-each loop statement.
Until java8
, Iterable Interface
was having only one method iterator()
which returns an iterator over the element of type on which it is getting called.
In java8
two more default method got added.
-
splitIterator()
– Creates aSpliterator
over the elements described by thisIterable
. foreach()
– Performs a given action for each element ofIterable
until all elements have been processed or the action throws an exception.
2.2 Collection Interface
There are many methods declared in the Collection interface deciding very basic functionally for each type of classes in Collection Framework. E.g addition, removal, find, sort, size and implementation of equals() and hashCode() method. Below is the list of collection interface methods:
public boolean add(E e)
: to insert an element in this collection.public boolean addAll(Collection<? extends E> c)
: to insert the specified collection elements in the invoking collection.-
public void clear()
: This method removes elements from the list. It removes the total number of elements from the collection. boolean contains(Object o)
: Returns true if this collection contains the specified element.public boolean isEmpty()
: checks if this collection is empty.public Iterator iterator()
: returns an iterator over the elements in this collection.default Stream<E> parallelStream()
: Returns a possibly parallelStream
. It is allowable for this method to return a sequential stream.-
public boolean remove(Object element)
: to (delete) remove an element from the list collection. public boolean removeAll(Collection<?> c)
: to delete all the elements of the specified collection from the invoking collection.default boolean removeIf(Predicate<? super E> filter)
: Added injava8
to delete all the elements of the collection that satisfy the specified condition in the predicate.-
public int size()
: returns the total number of elements in the collection. -
public boolean retainAll(Collection<?> c)
: deletes all the elements of invoking collection except the specified collection. public Object[] toArray()
: converts the collection into an array.public <T> T[] toArray(T[] a)
: Returns an array containing all of the elements in this collection; the runtime type of the returned array is that of the specified array.
2.3 List Interface
Below are some important properties of List in java-
- It is a child interface of
Collection interface
in Collection Framework - We use
List
implemented classes, if we want to store a group of elements in a single entity by allowing duplicate and preserving insertion order. List
allownull
values and since it doesn’t restrict duplicates so allows multiple duplicate elements also.- It allows zero-based index access to its elements.
- It also provides
ListIterator
which allows insertion, replacement and bidirectional access of its element. We can also pass an optional index to get aListIterator
starting from a particular index in theList
There are four implementation classes of List
interface; namely ArrayList
, LinkedList
, Stack
, Vector
.
While instantiating List interface we should provide one of the implementation class objects as below:
List arrayList= new ArrayList(); List linkedList= new LinkedList(); List vector= new Vector(); List stack= new Stack();
Lets take each implementation class in detail.
2.3.1 ArrayList
ArrayList is a non-synchronized re-sizable implementation of List
interface. ArrayList
can be created in the following ways –
creating ArrayList
// ArrayList with default constructor List<Integer> integerList = new ArrayList<Integer>(); // ArrayList with default capicity List<Integer> integerListWithInnitialCapacity = new ArrayList<Integer>(10); // ArrayList with other collection class List<Integer> integerListWithOtherCollection = new ArrayList<Integer>(Arrays.asList(1, 2, 3, 4, 5));
We will use the first and sometimes third way of instantiating ArrayList
here onwards.
Adding Elements in ArrayList
Adding elements in ArrayList
//Adding element at the end of the list
integerList.add(1);
System.out.println(integerList);
//Adding collection of elements at the end of the list
integerList.addAll(integerListWithOtherCollection);
System.out.println(integerList);
//Adding element on perticular index of the list
integerList.add(3, 100);
System.out.println(integerList);
//Adding collection of elements on perticular index of the list
integerList.addAll(0, integerListWithOtherCollection);
System.out.println(integerList);
Result
[1] [1, 1, 2, 3] [1, 1, 2, 100, 3] [1, 2, 3, 1, 1, 2, 100, 3]
Clearing All List elements
List<Integer> integerListWithOtherCollection = new ArrayList<Integer>(Arrays.asList(1, 2, 3)); System.out.println(integerListWithOtherCollection); integerListWithOtherCollection.clear(); System.out.println(integerListWithOtherCollection);
Result
[1, 2, 3] []
Checking Existance of List element
List<Integer> integerListWithOtherCollection = new ArrayList<Integer>(Arrays.asList(1, 2, 3)); System.out.println(integerListWithOtherCollection.contains(3)); System.out.println(integerListWithOtherCollection.contains(7));
Result
true false
Iterating List elements
// Iterate using iterator Iterator<Integer> integerListWithOtherCollectionItr = integerListWithOtherCollection.iterator(); System.out.println("Using Iterator"); while (integerListWithOtherCollectionItr.hasNext()) System.out.println(integerListWithOtherCollectionItr.next()); System.out.println("Using forEach()"); // Iterate using forEach() integerListWithOtherCollection.forEach(System.out::println);
Result
Using Iterator 1 2 3 Using forEach() 1 2 3
Getting Element By index
List<Integer> integerListWithOtherCollection = new ArrayList<Integer>(Arrays.asList(1, 2, 3)); System.out.println(integerListWithOtherCollection); // Getting Element By index System.out.println("Element at index 1 is: " + integerListWithOtherCollection.get(1)); System.out.println("Element at index 2 is: " + integerListWithOtherCollection.get(2));
Result
[1, 2, 3] Element at index 1 is: 2 Element at index 2 is: 3
Getting index of element
System.out.println("Index of 3 is: " + integerListWithOtherCollection.indexOf(3));
System.out.println("Index of 4 is: " + integerListWithOtherCollection.indexOf(4));
Result
Index of 3 is: 2 Index of 4 is: -1
Removing element from list
List<String> integerListWithOtherCollection = new ArrayList<String>(Arrays.asList("Jack", "is", "a", "good", "kid", "but", "he", "is", "very", "noughty")); System.out.println(integerListWithOtherCollection); //Removing Element from 2nd index integerListWithOtherCollection.remove(2); System.out.println(integerListWithOtherCollection); //Removing object from List integerListWithOtherCollection.remove("but"); System.out.println(integerListWithOtherCollection); //Removing collection of element from list integerListWithOtherCollection.removeAll(Arrays.asList("very")); System.out.println(integerListWithOtherCollection); //Removing collection based in some condition integerListWithOtherCollection.removeIf(x -> x.contains("good")); System.out.println(integerListWithOtherCollection);
Result
[Jack, is, a, good, kid, but, he, is, very, noughty] [Jack, is, good, kid, but, he, is, very, noughty] [Jack, is, good, kid, he, is, very, noughty] [Jack, is, good, kid, he, is, noughty] [Jack, is, kid, he, is, noughty]
Setting element value in list
List<Integer> integerList = new ArrayList<Integer>(Arrays.asList(1, 2, 3)); System.out.println("Initial List" + integerList); integerList.set(2, 5); System.out.println("List after replacing 2nd index value to 5" + integerList);
Result
[1, 2, 3] [1, 2, 5]
Getting sublist from list
List<String> integerListWithOtherCollection = new ArrayList<String>( Arrays.asList("Jack", "is", "a", "good", "kid", "but", "he", "is", "very", "noughty")); System.out.println(integerListWithOtherCollection.subList(0, 5));
Result
jack, is, a, good, kid]
2.3.2 LinkedList
LinkedList
is a non-synchronized implementation of Doubly-linked list
by using List
and Deque
interfaces and can be created in the following ways –
creating LinkedList
// LinkedList with default constructor LinkedList<String> linkedList = new LinkedList<String>(); // LinkedList with other collection class LinkedList<String> linkedListFromCollection = new LinkedList<String>(Arrays.asList("Jack", "John"));
We will use the first and sometimes third way of instantiating LinkedList
here onwards.
Adding elements in LinkedList
// Creating empty LinkedList LinkedList<String> linkedList = new LinkedList<String>(); System.out.println(linkedList); // adding an element at end of LinkedList using add() linkedList.add("Jack"); linkedList.add("John"); System.out.println(linkedList); // adding an element at 0th index LinkedList linkedList.add(0, "Hill"); System.out.println(linkedList); // adding an collection at end of LinkedList linkedList.addAll(Arrays.asList("Andrews", "Elizabeth")); System.out.println(linkedList); // adding an collection at 1st index of LinkedList linkedList.addAll(1, Arrays.asList("Cedric", "Aaron")); System.out.println(linkedList); // adding an element at start of LinkedList linkedList.addFirst("Roger"); System.out.println(linkedList); // adding an element at end of LinkedList linkedList.addLast("Jeanette"); System.out.println(linkedList);
Result
[] [Jack, John] [Hill, Jack, John] [Hill, Jack, John, Andrews, Elizabeth] [Hill, Cedric, Aaron, Jack, John, Andrews, Elizabeth] [Roger, Hill, Cedric, Aaron, Jack, John, Andrews, Elizabeth] [Roger, Hill, Cedric, Aaron, Jack, John, Andrews, Elizabeth, Jeanette]
Clearing All LinkedList elements
// Creating linkedList with collection LinkedList<String> linkedList = new LinkedList<String>(Arrays.asList("Jack", "John")); System.out.println(linkedList); // Clearing LinkedList linkedList.clear(); System.out.println(linkedList);
Result
[Jack, John] []
Checking Existance of LinkedList element
// Creating linkedList with collection LinkedList<String> linkedList = new LinkedList<String>(Arrays.asList("Jack", "John")); System.out.println("initial linkedList: " + linkedList); // checking Jack is there in LinkedList System.out.println("Is Jack there in List: " + linkedList.contains("Jack")); // checking Hill is there in LinkedList System.out.println("Is Hill there in List: " + linkedList.contains("Hill"));
Result
initial linkedList: [Jack, John] Is Jack there in List: true Is Hill there in List: false
Iterating List elements
LinkedList<String> linkedList = new LinkedList<String>(); linkedList.addAll(Arrays.asList("Andrews", "Elizabeth", "Bob")); System.out.println("Using default Iterator"); Iterator<String> linkedListIterator = linkedList.iterator(); while (linkedListIterator.hasNext()) System.out.println(linkedListIterator.next()); System.out.println("Using default ListIterator"); Iterator<String> listIterator = linkedList.listIterator(); while (listIterator.hasNext()) System.out.println(listIterator.next()); System.out.println("Using default ListIterator from specified index"); Iterator<String> listIteratorFrom1stIndex = linkedList.listIterator(1); while (listIteratorFrom1stIndex.hasNext()) System.out.println(listIteratorFrom1stIndex.next()); System.out.println("Using default DecendingIterator"); Iterator<String> decendingListIterator = linkedList.descendingIterator(); while (decendingListIterator.hasNext()) System.out.println(decendingListIterator.next()); System.out.println("Using for each loop"); linkedList.forEach(System.out::println);
Result
Using default Iterator Andrews Elizabeth Bob Using default ListIterator Andrews Elizabeth Bob Using default ListIterator from specified index Elizabeth Bob Using default DecendingIterator Bob Elizabeth Andrews Using for each loop Andrews Elizabeth Bob
Getting LinkedList elements
LinkedList<String> linkedList = new LinkedList<String>(); linkedList.addAll(Arrays.asList("Andrews", "Elizabeth", "Bob")); // First Element using element() method System.out.println("First Element using element() method: " + linkedList.element()); // First Element using getFirst() method System.out.println("First Element using getFirst() method: " + linkedList.getFirst()); // First Element using peek() method System.out.println("First Element using peek() method: " + linkedList.peek()); // First Element using peekFirst() method System.out.println("First Element using peekFirst() method: " + linkedList.peekFirst()); // Last Element using getLast() method System.out.println("Last Element using getLast() method: " + linkedList.getLast()); // Last Element using peekLast() method System.out.println("Last Element using peekLast() method: " + linkedList.peekLast()); // 1st index Element using get() method System.out.println("1st index Element using get() method: " + linkedList.get(1));
Result
First Element using element() method: Andrews First Element using getFirst() method: Andrews First Element using peek() method: Andrews First Element using peekFirst() method: Andrews Last Element using getLast() method: Bob Last Element using peekLast() method: Bob 1st index Element using get() method: Elizabeth
Getting Index of element from LinkedList
LinkedList<String> linkedList = new LinkedList<String>(Arrays.asList("Andrews", "Elizabeth", "Andrews")); System.out.println("First Index of Andrews is: " + linkedList.indexOf("Andrews")); System.out.println("Last Index of Andrews is: " + linkedList.lastIndexOf("Andrews")); System.out.println("First Index of Bob is: " + linkedList.lastIndexOf("Bob"));
Result
Index of Andrews is: 0 Index of Andrews is: 2 Index of Andrews is: -1
Removing Index of element from LinkedList
LinkedList<String> linkedList = new LinkedList<String>(Arrays.asList("Alex", "John", "Martin", "Google", "Andrews", "Elizabeth", "Andrews")); System.out.println("Innitial Linked list: " + linkedList); System.out.println("removing the head (first element) of list."); linkedList.remove(); System.out.println("Updated Linked list: " + linkedList); System.out.println("removing the element at index 1 in this list."); linkedList.remove(1); System.out.println("Updated Linked list: " + linkedList); System.out.println("removing the first occurrence of the specified element(Elizabeth) from this list, if it is present."); linkedList.remove("Elizabeth"); System.out.println("Updated Linked list: " + linkedList); System.out.println("removing the first element from this list."); linkedList.removeFirst(); System.out.println("Updated Linked list: " + linkedList); System.out.println("removing the last element from this list."); linkedList.removeLast(); System.out.println("Updated Linked list: " + linkedList); System.out.println( "removing the first occurrence of the specified element(Google) in this list (when traversing the list from head to tail)."); linkedList.removeFirstOccurrence("Google"); System.out.println("Updated Linked list: " + linkedList); System.out.println( "removing the last occurrence of the specified element(Andrews) in this list (when traversing the list from head to tail)."); linkedList.removeLastOccurrence("Andrews"); System.out.println("Updated Linked list: " + linkedList);
Result
Innitial Linked list: [Alex, John, Martin, Google, Andrews, Elizabeth, Andrews] removing the head (first element) of list. Updated Linked list: [John, Martin, Google, Andrews, Elizabeth, Andrews] removing the element at index 1 in this list. Updated Linked list: [John, Google, Andrews, Elizabeth, Andrews] removing the first occurrence of the specified element(Elizabeth) from this list, if it is present. Updated Linked list: [John, Google, Andrews, Andrews] removing the first element from this list. Updated Linked list: [Google, Andrews, Andrews] removing the last element from this list. Updated Linked list: [Google, Andrews] removing the first occurrence of the specified element(Google) in this list (when traversing the list from head to tail). Updated Linked list: [Andrews] removing the last occurrence of the specified element(Andrews) in this list (when traversing the list from head to tail). Updated Linked list: []
Setting value of an element in LinkedList
LinkedList<String> linkedList = new LinkedList<String>(Arrays.asList("Alex", "John", "Martin")); System.out.println("Innitial Linked list: " + linkedList); System.out.println("Updating John(at index 1) to Elizabeth"); linkedList.set(1, "Elizabeth"); System.out.println("Updated Linked list: " + linkedList);
Result
Innitial Linked list: [Alex, John, Martin] Updating John(at index 1) to Elizabeth Updated Linked list: [Alex, Elizabeth, Martin]
2.3.3 Vector
Vector
is a synchronized implementation of a growable array of objects which can be accessed using an integer index. We can create a Vector class object in the following ways.
Creating Vector Objects
// Creates an empty vector so that its internal data array has size 10 and zero capacity increment. Vector<String> vector = new Vector<String>(); // Creates a vector containing the elements of the specified collectionCreates Vector<String> vectorWithAnotherCollection = new Vector<String>(Arrays.asList("Alex", "Bob")); // Constructs an empty vector with the specified initial capacity and zero capacity increment. Vector<String> vectorWithDefaultCapicity = new Vector<String>(10); // Creates an empty vector with the specified initial capacity and capacity increment. Vector<String> vectorWithDefaultCapicityAndIncrementFactor = new Vector<String>(10, 5);
Adding Element to Vector Objects
Vector<String> vector = new Vector<String>(Arrays.asList("Devid", "Bob")); System.out.println("Initial Vector: " + vector); System.out.println("Appending the John to the end of Vector."); vector.add("John"); System.out.println("Updated Vector: " + vector); System.out.println("Inserting the Alex 2nd index in Vector."); vector.add(2, "Alex"); System.out.println("Updated Vector: " + vector); System.out.println("Appending all of the elements in the Collection(\"Martin\", \"Steave\") to the end of this Vector"); vector.addAll(Arrays.asList("Martin", "Steave")); System.out.println("Updated Vector: " + vector); System.out.println("Inserts all of the elements in the Collection(\"Jack\", \"Jill\") into Vector 1st position onwards."); vector.addAll(1, Arrays.asList("Jack", "Jill")); System.out.println("Updated Vector: " + vector); System.out.println("Adding the specified Element to the end of this vector and increasing its size by one."); vector.addElement("Zin"); System.out.println("Updated Vector: " + vector);
Result
Initial Vector: [Devid, Bob] Appending the John to the end of Vector. Updated Vector: [Devid, Bob, John] Inserting the Alex 2nd index in Vector. Updated Vector: [Devid, Bob, Alex, John] Appending all of the elements in the Collection("Martin", "Steave") to the end of this Vector Updated Vector: [Devid, Bob, Alex, John, Martin, Steave] Inserts all of the elements in the Collection("Jack", "Jill") into Vector 1st position onwards. Updated Vector: [Devid, Jack, Jill, Bob, Alex, John, Martin, Steave] Adding the specified Element to the end of this vector and increasing its size by one. Updated Vector: [Devid, Jack, Jill, Bob, Alex, John, Martin, Steave, Zin]
2.3.4 Vector
Stack
is a LIFO(Last In First Out) implementation of Vector class with 5 additional methods that allow a vector to be treated as a stack. These methods are push()
, pop()
, peek()
, search()
and empty()
. Stack
has only one constructor i.e. default constructor. We can create Stack
Objects and use all five methods as follows.
Using Stack
// Creating Stack Stack<String> stack = new Stack<String>(); // Adding Stack Element stack.push("item1"); stack.push("item2"); stack.push("item3"); System.out.println("Initial stack is: " + stack); // Getting top (peek) element System.out.println("Top item is: " + stack.peek()); // Finding 1 based index from top of the stack for an element System.out.println("Finding item3: found at index " + stack.search("item1")); // Deleting top element System.out.println("Deleting top item"); stack.pop(); System.out.println("Updated stack is: " + stack);
Result
Initial stack is: [item1, item2, item3] Top item is: item3 Finding item3: found at index 3 Deleting top item Updated stack is: [item1, item2]
2.4 ArrayList vs LinkedList
Features Compared | ArrayList | LinkedList |
Data structure | Dynamic (a.k.a. growable, resizable) array | Doubly-linked list |
Random Access with index (get() ) | O(1) | O(n) |
Insertion (add() ) / removal at the back | amortized O(1) | O(1) |
Insertion / removal at the front | O(n) | O(1) |
One step of iteration through an Iterator | O(1) | O(1) |
Insertion / removal in the middle through an Iterator / ListIterator | O(n) | O(1) |
Insertion/removal in the middle through the index | O(n) | O(n) |
Search contains() / removal remove() by object | O(n) | O(n) |
2.5 Which one to use ArrayList vs LinkedList
As per the comparison between ArrayList
and linkedList
in the previous section, we conclude the following points
- If we want to create a list which is going to be used heavily for lookup and search operation then we should go for
ArrayList
. - If we want to create a list which is going to be used heavily for data manipulation operation like insert/remove/update we should choose
LinkedList
.
3. More articles
- ArrayList Java Example – How to use arraylist
- LinkedList Java Example
- Java Array – java.util.Arrays Example
- Java Queue Example
- Java Stack Example
4. Download the Source Code
This was an example of Java List.
You can download the full source code of this example here: Java List Example
Last updated on Feb. 24th, 2022