Spring Boot Docker Image Debugging
In this article, we will explain how to debug the spring boot application running in the docker image. We will see a sample spring boot application and debug it in the docker container.
1. Create a spring boot application
Create a simple spring-boot application using any IDE(in my case, I am using IntelliJ IDEA) of your choice with basic configuration and dependency. I am using gradle for the dependencies. You can use maven as well. Find the build.gradle file as shown below
build.gradle
plugins { id 'org.springframework.boot' version '2.4.0' id 'io.spring.dependency-management' version '1.0.10.RELEASE' id 'java' } group = 'com.jcg' version = '1.0' sourceCompatibility = '1.8' repositories { mavenCentral() } dependencies { implementation 'org.springframework.boot:spring-boot-starter-web' testImplementation 'org.springframework.boot:spring-boot-starter-test' } test { useJUnitPlatform() }
It has a simple controller with a simple greeting message as shown below:
DockerController
package com.jcg.sampledocker; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController; @RestController public class DockerController { @RequestMapping("/") public String greetings(@RequestParam String name) { String message = " Hello " +name.toUpperCase() + ", from JCG"; return message; } }
Run the following command to build the application jar file.
./gradlew clean build
This will compile, build the application and generate the executable jar file in the build/libs folder. This jar file is used in the next steps
2. Create a docker file
Create the docker file and add the following line as shown below
Dockerfile
FROM openjdk:9-jre ADD build/libs/sample-docker-1.0.jar . EXPOSE 8080 5005 CMD java -jar sample-docker-1.0.jar
Here we are using the base image as openjdk:9-jre to build our container. It will copy the jar file into the docker image so that it’s available within the container. We are exposing two of the ports, as shown above. One is the default one (8080), and the other is for debugging (5005). Finally, we have the command to execute once the docker container runs.
3. Create a docker-compose file
Now create a docker compose file to setup the application. Add the following code into the docker-compose file. It basically specifies the ports and agent for remote debug as shown below
docker-compose.yml
services: web: build: . ports: - "8080:8080" - "5005:5005" command: java -agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=*:5005 -jar sample-docker-1.0.jar
Here in the command section we configure the JVM to allow debugging. We enable the agent Java Debug Wire Protocol (JDWP) inside the JVM. And the for the debug connections is set as 5005.
4. Running the application
4.1 Setup the remote debug
Inorder to debug the application first attach the remote debugger. You can do this in any IDE in the run configurations section. Please find the screenshot below
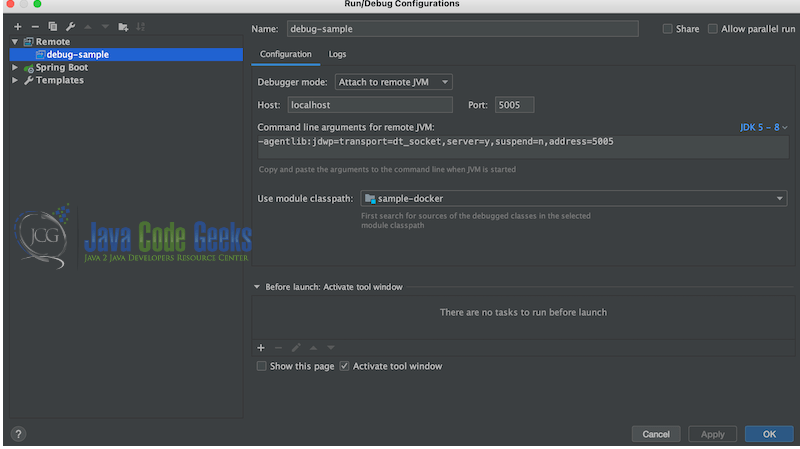
Here as shown in the image above we are using address as 5005 to listen to debug connections
4.2 Running the application
We will run the application using the docker-compose as shown below
docker-compose up
Once you run this you can see the server is listening to the debug connection in the port 5005 as shown
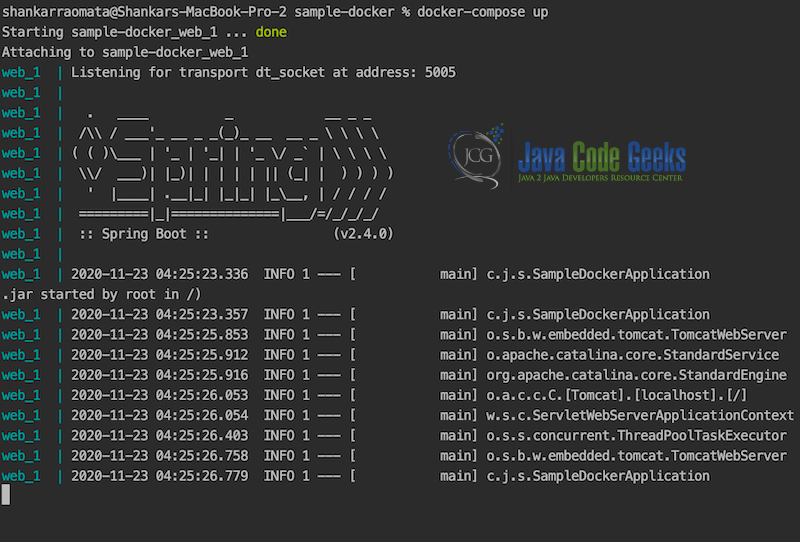
5. Debugging the application
Now once the server has started running, go and click the remote debugger which you have set in the step 4.1. And then add a breakpoint in the controller and enter the url localhost:8080?name=abc into the browser. You will see the control stopping at the breakpoint as shown below.
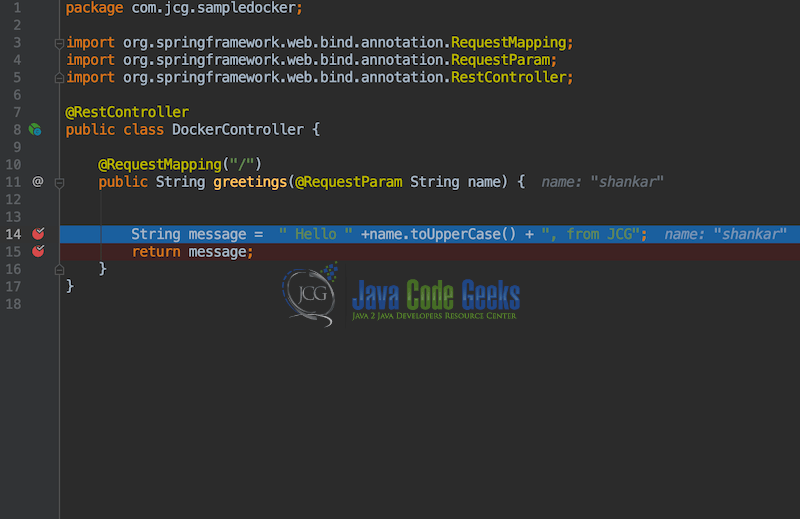
6. Summary
In this article we discussed about the debugging spring boot application in the docker image. We saw a sample application and configured the same for the debugging in the container.
You can find more Spring boot tutorials here.
7. Download the source code
This was an article of how to debug the spring boot application running in the docker image.
You can download the full source code of this example here: Spring Boot Docker Image Debugging