Spring Boot add filter Example
In this article, we will see how to configure Filter using Spring Boot. Also, we will see how to configure multiple filters.
1. What are Filters?
Filter is an interface available in javax.servlet package which use to perform filtering task on request to a resource (a servlet or static content), or on the response from a resource, or both . In fact it is an object used to intercept the HTTP requests and responses of your application.
By using filter, we can perform two operations:
- Before sending the request to the controller
- Before sending a response to the client
2. Filter methods
Spring Boot provides few options to register custom filters in the Spring Boot application. We can define our custom filter implementing this interface. Since Filter interface contains three methods (all of them are invoked by container), our custom filter need to override these methods.
Filter interface methods are:
- void init(FilterConfig filterConfig) throws ServletException
This method tells this filter has been placed into service. It is invoked only one time. If it throws ServletException other tasks will not be performed. - void doFilter(ServletRequest servletRequest, ServletResponse ServletResponse, FilterChain filterChain) throws IOException, ServletException
It is also invoked each time whenever the client sends a request or server sends a response. In this method, we can check our request is proper or not and even we can modify request and response data. - void destroy()
It indicates to a filter that it is being taken out of service.
3. Spring Boot add filter – Examples
Here we will some examples of Spring filters:
3.1 Example01: Real-time use of filter
Suppose we want to allow some specific request URI, we can restrict by configuring a filter.
Step1: Create a maven project and name it example01.
Update the pom.xml as below:
pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>example01</groupId> <artifactId>example01</artifactId> <version>1.0-SNAPSHOT</version> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.2.RELEASE</version> </parent> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.7.0</version> <configuration> <source>1.9</source> <target>1.9</target> <jdkToolchain> <version>9</version> </jdkToolchain> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-jar-plugin</artifactId> <version>3.0.2</version> </plugin> </plugins> </build> </project>
Step2: Define below packages and classes.
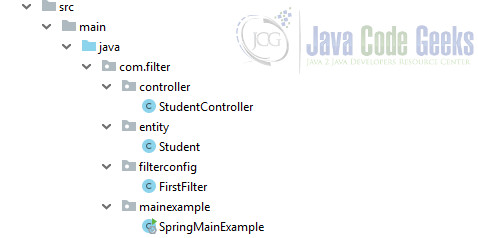
Update the Studend.java class as below:
Student.java
package com.filter.entity; public class Student { int studentId; String studentName; String major; public int getStudentId() { return studentId; } public void setStudentId(int studentId) { this.studentId = studentId; } public String getStudentName() { return studentName; } public void setStudentName(String studentName) { this.studentName = studentName; } public String getMajor() { return major; } public void setMajor(String major) { this.major = major; } }
Now it is time to set the mappings in StudentController.java.
StudentController.java
package com.filter.controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.ResponseBody; import org.springframework.web.bind.annotation.RestController; import com.filter.entity.Student; @RestController @RequestMapping("/student") public class StudentController { @RequestMapping(value = "/getstudent",method = RequestMethod.GET) @ResponseBody public Student getStudent() { Student student = new Student(); student.setStudentId(1); student.setStudentName("John Smith"); student.setMajor("Computer Science"); return student; } }
We will define our filter in FirstFilter.java.
FirstFilter.java
package com.filter.filterconfig; import java.io.IOException; import javax.servlet.Filter; import javax.servlet.FilterChain; import javax.servlet.FilterConfig; import javax.servlet.ServletException; import javax.servlet.ServletRequest; import javax.servlet.ServletResponse; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.springframework.stereotype.Component; @Component public class FirstFilter implements Filter{ //this method will be called by container when we send any request public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException { System.out.println("doFilter() method is invoked"); HttpServletRequest httpServletRequest = (HttpServletRequest)request; HttpServletResponse httpServletResponse = (HttpServletResponse)response; System.out.println("Context path is " + httpServletRequest.getContextPath()); chain.doFilter(httpServletRequest, httpServletResponse); System.out.println("doFilter() method is ended"); } // this method will be called by container while deployment public void init(FilterConfig config) throws ServletException { System.out.println("init() method has been get invoked"); System.out.println("Filter name is "+config.getFilterName()); System.out.println("ServletContext name is"+config.getServletContext()); System.out.println("init() method is ended"); } public void destroy() { //do some stuff like clearing the resources } }
Filters perform filtering in the doFilter
method. Every Filter has access to a FilterConfig object from which it can obtain its initialization parameters, a reference to the ServletContext which it can use, for example, to load resources needed for filtering tasks. In order for Spring to be able to recognize a filter, we needed to define it as a bean with the @Component
annotation.
SpringMainExample.java
package com.filter.mainexample; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.context.ConfigurableApplicationContext; @SpringBootApplication(scanBasePackages={"com.filter.*"}) public class SpringMainExample { public static void main(final String[] args) { final ConfigurableApplicationContext configurableApplicationContext = SpringApplication .run(SpringMainExample.class, args); } }
Now run the SpringMainExample.java
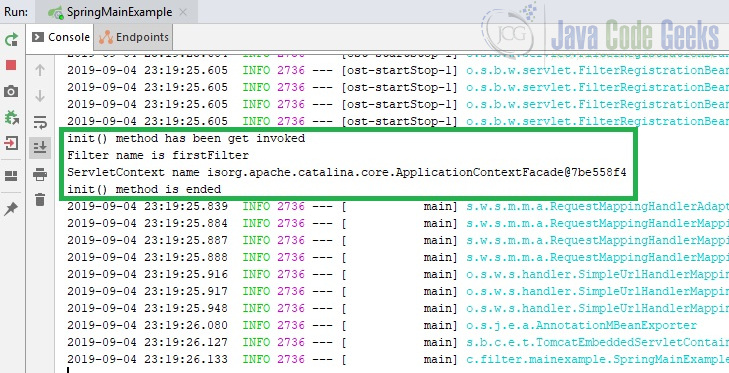
As you see init()
method has been invoked while tomcat start, but doFilter()
method has not been invoked. If we hit the below URI from postman then doFilter()
method will be invoked.
” http://localhost:8080/student/getstudent”
The URL has been defined in the controller class.
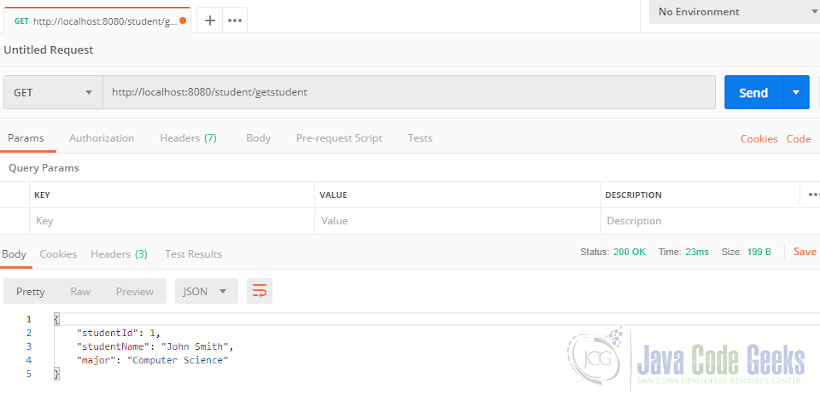
In console we have content of doFilter()
method it will always get invoked with each new request.
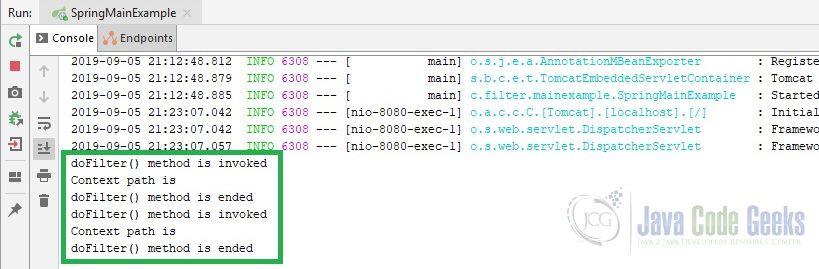
3.2 Example02:
The following example shows the code for reading the remote host and remote address from the ServletRequest object before sending the request to the controller.
MyFilter.java
package com.java.code.geeks; import java.io.IOException; import javax.servlet.Filter; import javax.servlet.FilterChain; import javax.servlet.FilterConfig; import javax.servlet.ServletException; import javax.servlet.ServletRequest; import javax.servlet.ServletResponse; import org.springframework.stereotype.Component; @Component public class MyFilter implements Filter { @Override public void destroy() {} @Override public void doFilter(ServletRequest request, ServletResponse response, FilterChain filterchain) throws IOException, ServletException { System.out.println("Remote Host:"+request.getRemoteHost()); System.out.println("Remote Address:"+request.getRemoteAddr()); filterchain.doFilter(request, response); } @Override public void init(FilterConfig filterconfig) throws ServletException {} }
In the Spring Boot main application class file, we have added the simple REST endpoint that returns the “This is Example02!” string.
Application.java
package com.java.code.geeks; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication @RestController public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } @RequestMapping(value = "/") public String hello() { return "This is Example02!"; } }
The pom.xml file is the same as we used in example01.
Build the project using this command:
mvn clean install
Now run the Application.java. You can see the application has started on the Tomcat port 8080.
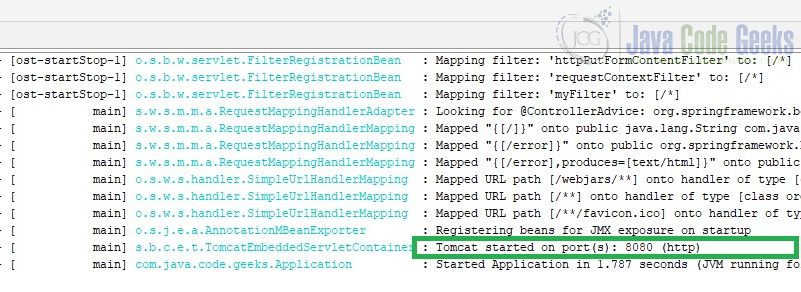
Now hit the URL http://localhost:8080.
As you see the remote address and remote host are displayed:
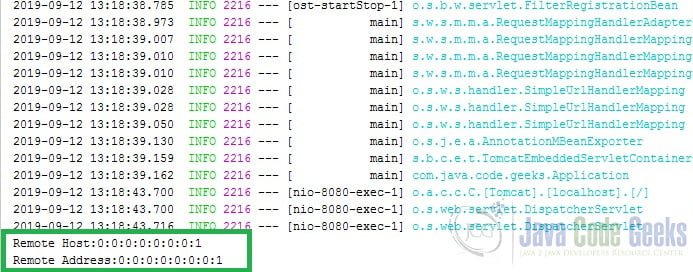
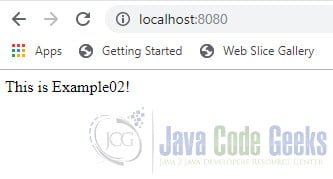
4. Download the Source Code
You can download the full source code of this example here: Spring Boot add filter Example