JSF Form Example
With this example, we are going to demonstrate how to use JSF Form in JavaServer Faces (JSF) to develop a Web Application.
1. What is Java Server Faces (JSF)?
JavaServer Faces (JSF) is used as a Component-based web framework that implements a Model-View-Controller design pattern for developing web applications.
2. Overview of JavaServer Faces (JSF)
- It uses Facelets as its default templating System.
- It implements the MVC Design Pattern.
- It uses Expression Language that allows JSF Pages to access and manipulate Managed Beans and bind them to UI components.
- It has Navigation Model explains when and how JSF Page navigation should happen in a web application.
- It supports HTML 5 and Ajax.
- It is easy and can be used for rapid web development.
- It supports Internationalization to create web applications in different languages and regions.
- It provides Managed Bean and Backing Bean to offer Inversion of Control (IoC) or Dependency Injection.
- It handles Data Conversion using Faces Converter System and Validation using Faces validation System.
- It provides Event-based Programming model.
- It can be integrated with Spring Framework and JDBC.
- It can use other User Interface Component library (Primefaces) for creating User Interface Screens.
- It uses Core Component Tags Library for validations, converting input values, and loading resource bundles.
- It uses an HTML Component Tags Library to create and render HTML components.
3. What is Standard JSF Library?
The Standard JSF library consists of four parts:
- The Core library
- The HTML library
- The Facelet Templating tag library
- The Composite Component tag library
The Core library is associated with the f: namespace and provides common application development utilities in the areas of validation, conversion, internationalization, and overall application development.
The HTML library is associated with the h: namespace and is specifically for HTML clients and provides a set of widgets rendered in HTML that are common in most Web applications, such as text fields, buttons, checkboxes, and so on.
h: form tag represents an Html Form component of HTML library, The Html form component renders as an Input form. The inner tags of the form receive the data that will be submitted with the form. It displays an HTML element with an action attribute set to the URL that defines the view containing the form and a method attribute set to “post”. When the form is submitted, only components that are children of the submitted form are processed.
The Facelet Templating library, which is associated with the ui: namespace and adds template/layout functionality.
The Composite component tag library, which is associated with the cc: namespace and adds the ability to define a usage contract for Facelet Composite Components.
4. JSF Form Example – Employee Information System
This JSF Form Example shows you how to store and retrieve the values for an Employee Information System using HTML Form Component (h:form) of HTML Library of the Standard JSF Library.
Employee Information System allows getting the values from the User for Employee data such as Employee name, Gender, Date of Birth, Marital Status, Address, Email Address, Mobile Number, Designation, Department, Status of Employment (Permanent or Temporary). It stores the Employee information after getting confirmation about the employee data from the User.
This Employee Information System will have the following files: EmployeeBean.java
, employee.xhtml
, confirm.xhtml
, success.xhtml
, and web.xml.
4.1 Software Requirements
- Java SE 8
- Eclipse Java EE IDE for Web Developers (Version: 2018-09 (4.9.0))
- Apache-tomcat-9.0.13
- JSF 2.0
4.2 How to create a Project
Open Eclipse, Click File > New > Dynamic Web Project as shown below:
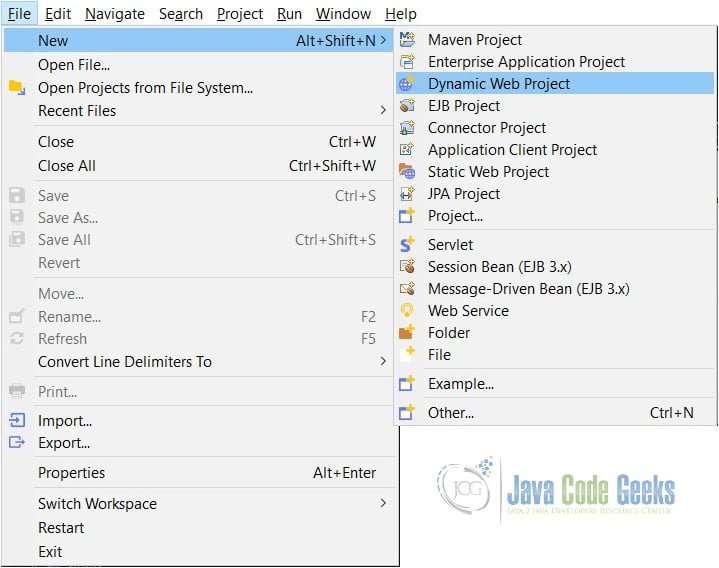
Enter Project Name and Select “Apache Tomcat v9.0” as Target Runtime, as shown below and then Click Next Button.
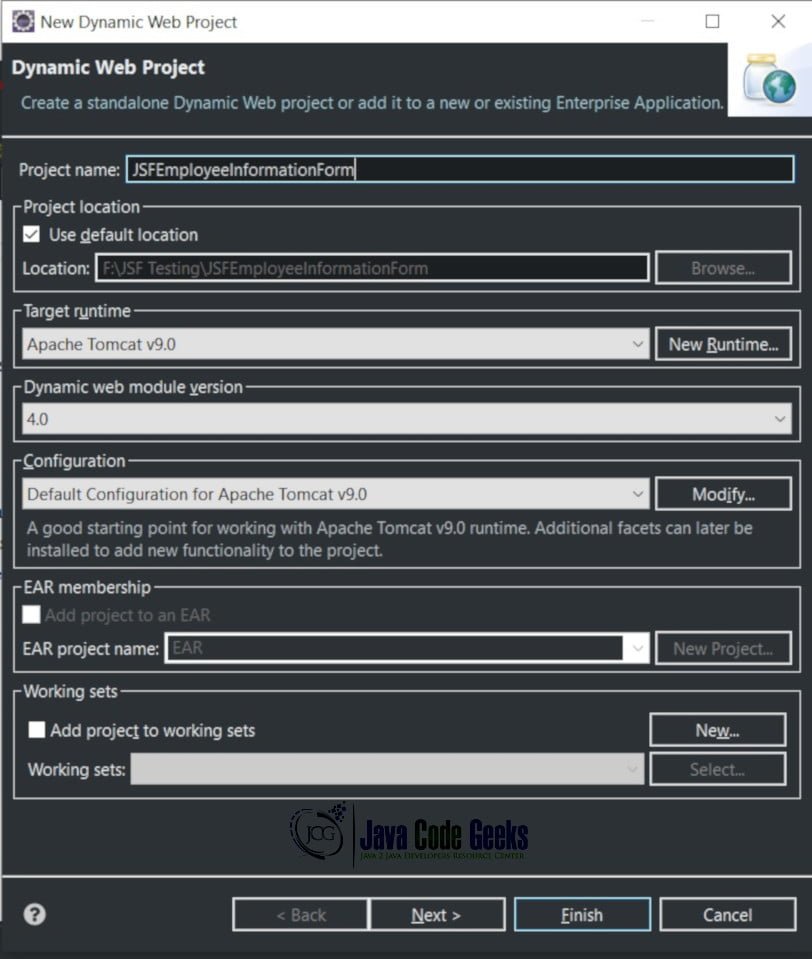
Click Next Button as shown below:
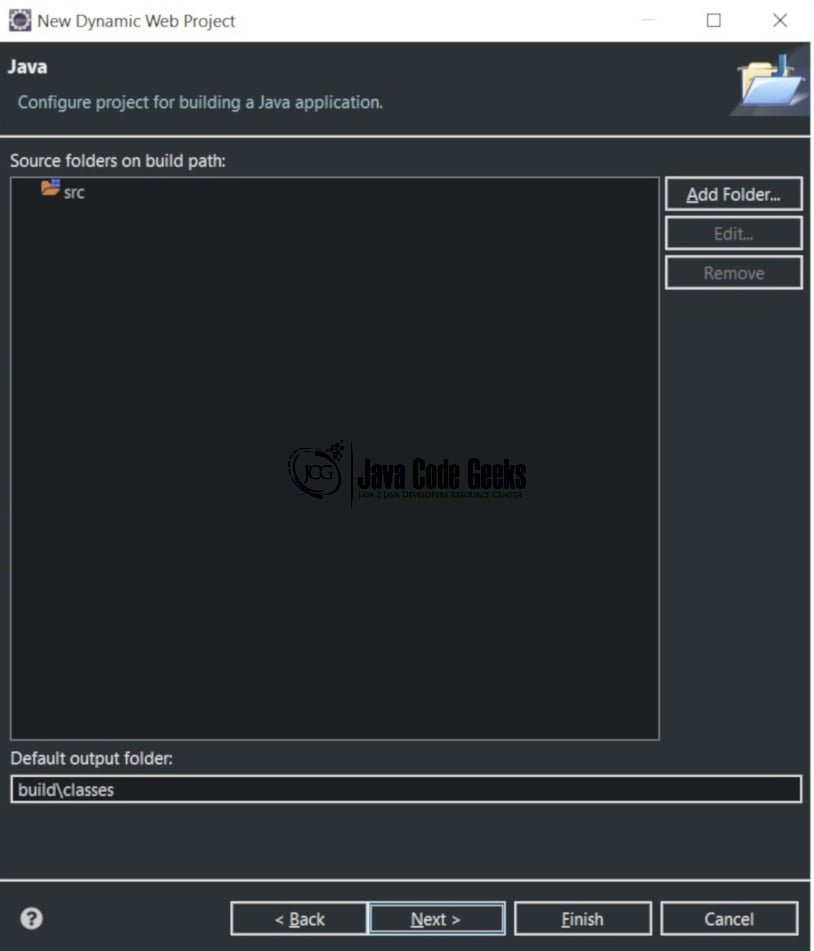
Select the Checkbox (Generate web.xml
deployment descriptor and then Click Finish Button
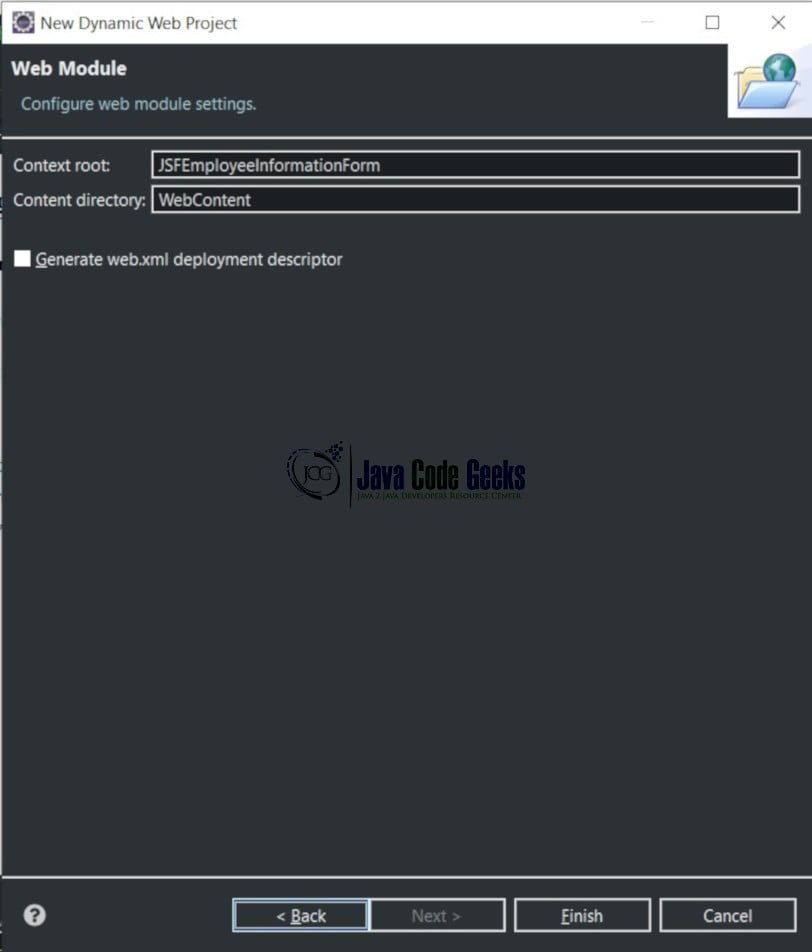
4.3 Project Folder Structure
The following Project Folder Structure will be generated :
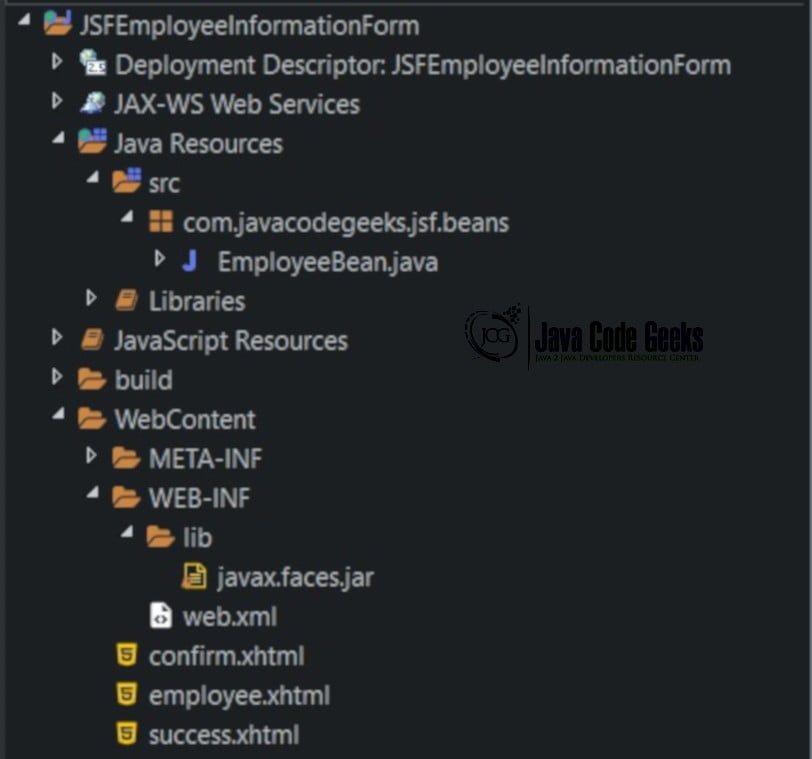
4.4 Outputs
Employee Information System containing JSF Form will have the following outputs as shown below:
The below screen shows the data entry screen for Employee Information System:
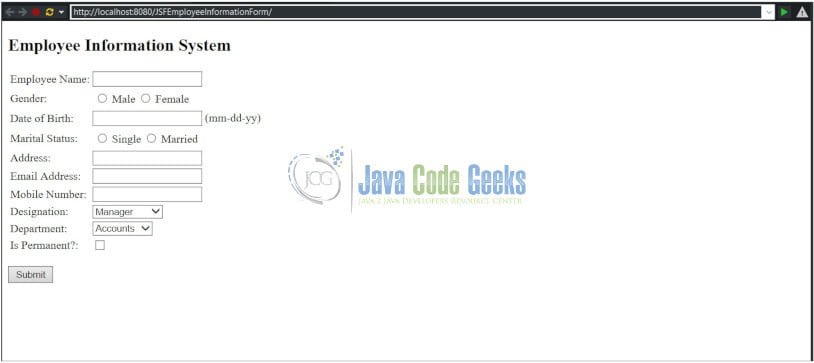
The below screen displays error messages if the user clicks on submit button without entering valid employee data.
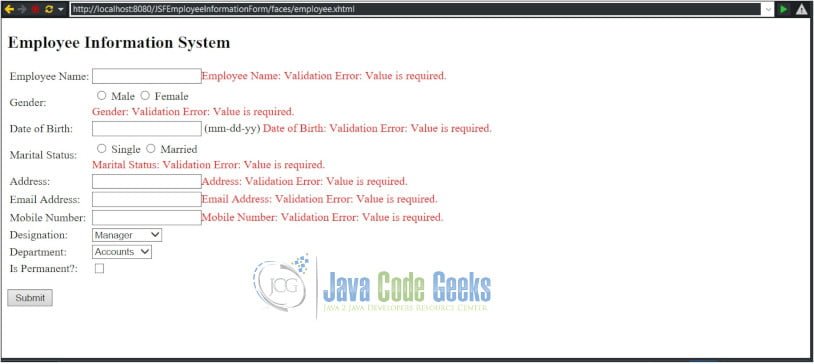
The below screen shows that user enters valid data and trying to submit it.
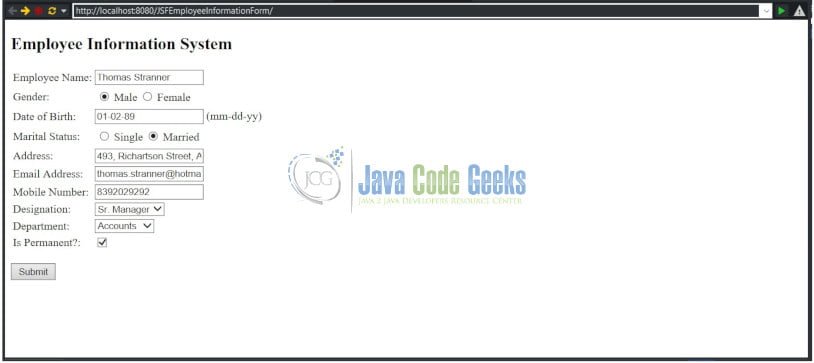
The below screen shows the valid data entered by the user, prompting the user either to click on the cancel button to edit the employee data or to click on the confirm button to accept the employee data.
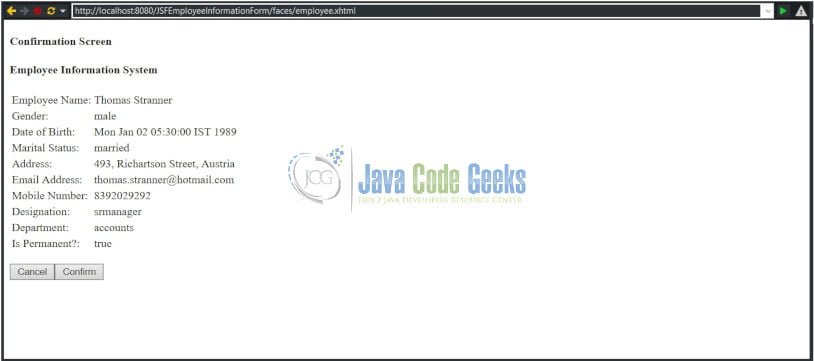
The below screen allows the user to enter new employee data or modify the already entered data and allow to click on submit button for further processing.
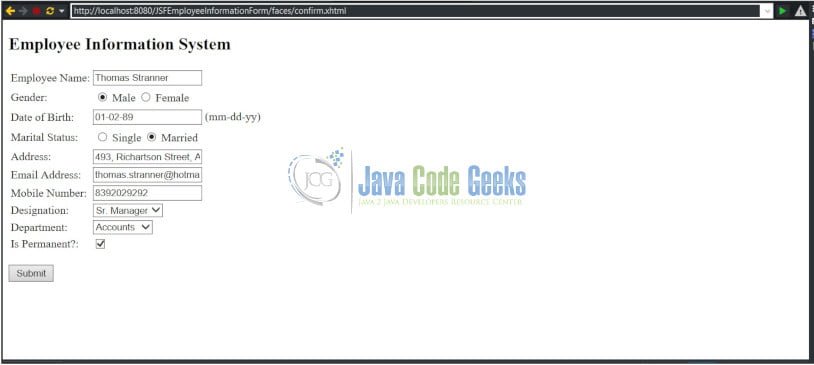
The below screen shows that the User modified the already entered data and clicking on submit button.
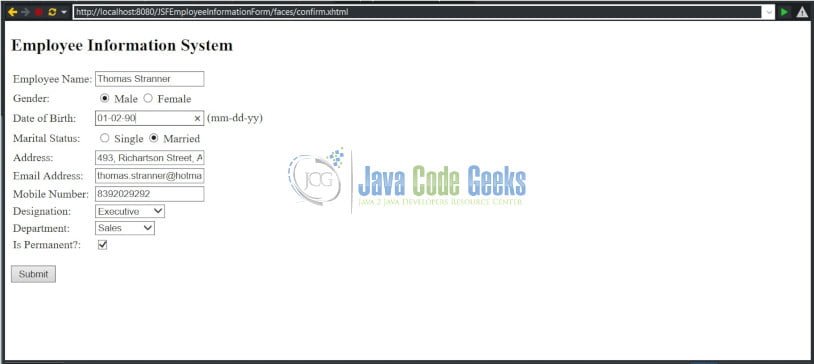
The below screen displaying the submitted data, prompting the user either to click on the cancel button to edit the data again or to click on the confirm button to accept the employee data entered by the user. The user has clicked on the Confirm button to accept the data.
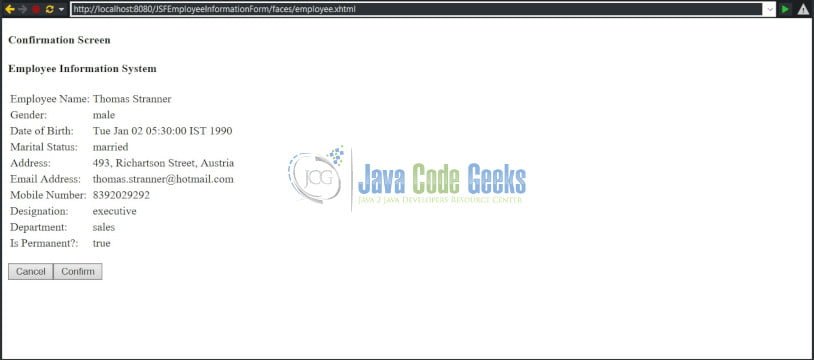
The below screen displays the accepted and confirmed employee data entered by the User.
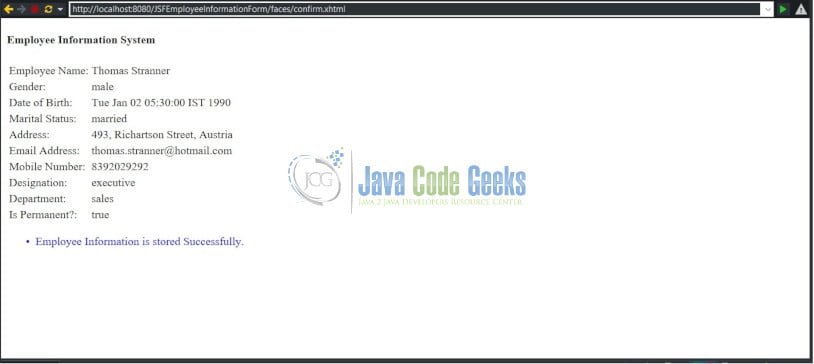
4.5 Source Code
The following are the Source Code used for Employee Information System explaining about JSF Form Example:
JSF application consists of Configuration files and Web content files. The key configuration files required are faces-config.xml
and a web.xml
. Web content files can be composed of Facelet and/or general HTML content such as HTML pages, images, and cascading style sheets (CSS).
employeebean.java
package com.javacodegeeks.jsf.beans; import java.util.Date; import javax.faces.application.FacesMessage; import javax.faces.component.UIComponent; import javax.faces.context.FacesContext; import javax.faces.bean.ManagedBean; import javax.faces.bean.SessionScoped; import javax.faces.validator.ValidatorException; @ManagedBean @SessionScoped public class EmployeeBean { // Member Variables private String empName; private String gender; private Date dob; private String address; private String emailAddress; private String mobileNumber; private String maritalStatus; private String designation; private String department; private boolean employeeType; // Member Methods public String getEmpName() { return empName; } public void setEmpName(String empName) { this.empName = empName; } public String getGender() { return gender; } public void setGender(String gender) { this.gender = gender; } public Date getDob() { return dob; } public void setDob(Date dob) { this.dob = dob; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } public String getEmailAddress() { return emailAddress; } public void setEmailAddress(String emailAddress) { this.emailAddress = emailAddress; } public String getMobileNumber() { return mobileNumber; } public void setMobileNumber(String mobileNumber) { this.mobileNumber = mobileNumber; } public String getMaritalStatus() { return maritalStatus; } public void setMaritalStatus(String maritalStatus) { this.maritalStatus = maritalStatus; } public String getDesignation() { return designation; } public void setDesignation(String designation) { this.designation = designation; } public String getDepartment() { return department; } public void setDepartment(String department) { this.department = department; } public boolean isEmployeeType() { return employeeType; } public void setEmployeeType(boolean employeeType) { this.employeeType = employeeType; } // Validate Email public void validateEmail(FacesContext context, UIComponent toValidate, Object value) throws ValidatorException { String emailStr = (String) value; if (-1 == emailStr.indexOf("@")) { FacesMessage message = new FacesMessage("Email Address is Valid"); throw new ValidatorException(message); } } // Action Methods public String storeEmployeeInfo() { boolean stored = true; FacesMessage message = null; String outcome = null; if (stored) { message = new FacesMessage("Employee Information is stored Successfully."); outcome = "success"; } else { message = new FacesMessage("Employee Information is NOT stored Successfully."); outcome = "employee"; } FacesContext.getCurrentInstance().addMessage(null, message); return outcome; } }
EmployeeBean.java
is a Java class that is used as a “managed bean” to temporarily store the employee information provided by the User. It accepts the employee data such as Employee name, Gender, Date of Birth, Marital Status, Address, Email Address, Mobile Number, Designation, Department, Status of Employment (Permanent or Temporary) from the User.
It also contains a simple validation method for verifying the e-mail address and action method storeEmployeeInfo. Employee Bean is a Managed Bean registered with a Session scope that will be stored on the HTTP session. This means that the values in the managed bean will persist beyond a single HTTP request for a single user.
employee.xhtml
<?xml version="1.0" encoding="ISO-8859-1" ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:f="http://xmlns.jcp.org/jsf/core" xmlns:h="http://xmlns.jcp.org/jsf/html"> <h:head> <title>Employee Information System</title> </h:head> <h:body> <h:form> <h2>Employee Information System</h2> <table> <tr> <td>Employee Name:</td> <td> <h:inputText label="Employee Name" id="ename" value="#{employeeBean.empName}" required="true"/> <h:message for="ename" style="color: red"/> </td> </tr> <tr> <td>Gender:</td> <td> <h:selectOneRadio label="Gender" id="gender" value="#{employeeBean.gender}" required="true"> <f:selectItem itemLabel="Male" itemValue="male" /> <f:selectItem itemLabel="Female" itemValue="female" /> </h:selectOneRadio> <h:message for="gender" style="color: red"/> </td> </tr> <tr> <td>Date of Birth:</td> <td> <h:inputText label="Date of Birth" id="dob" value="#{employeeBean.dob}" required="true"> <f:convertDateTime pattern="MM-dd-yy" /> </h:inputText> (mm-dd-yy) <h:message for="dob" style="color: red"/> </td> </tr> <tr> <td>Marital Status:</td> <td> <h:selectOneRadio label="Marital Status" id="mstatus" value="#{employeeBean.maritalStatus}" required="true"> <f:selectItem itemLabel="Single" itemValue="single" /> <f:selectItem itemLabel="Married" itemValue="married" /> </h:selectOneRadio> <h:message for="mstatus" style="color: red"/> </td> </tr> <tr> <td>Address:</td> <td> <h:inputText label="Address" id="address" value="#{employeeBean.address}" required="true"/> <h:message for="address" style="color: red"/> </td> </tr> <tr> <td>Email Address:</td> <td> <h:inputText label="Email Address" id="email" value="#{employeeBean.emailAddress}" required="true" validator="#{employeeBean.validateEmail}"/> <h:message for="email" style="color: red"/> </td> </tr> <tr> <td>Mobile Number:</td> <td> <h:inputText label="Mobile Number" id="mobileno" value="#{employeeBean.mobileNumber}" required="true"> </h:inputText> <h:message for="mobileno" style="color: red"/> </td> </tr> <tr> <td>Designation:</td> <td> <h:selectOneMenu label="Designation" value="#{employeeBean.designation}"> <f:selectItem itemLabel="Manager" itemValue="manager" /> <f:selectItem itemLabel="Executive" itemValue="executive" /> <f:selectItem itemLabel="Sr. Manager" itemValue="srmanager" /> </h:selectOneMenu> </td> </tr> <tr> <td>Department:</td> <td> <h:selectOneMenu label="Department" value="#{employeeBean.department}"> <f:selectItem itemLabel="Accounts" itemValue="accounts" /> <f:selectItem itemLabel="Sales" itemValue="sales" /> <f:selectItem itemLabel="Marketing" itemValue="marketing" /> </h:selectOneMenu> </td> </tr> <tr> <td>Is Permanent?:</td> <td> <h:selectBooleanCheckbox value="#{employeeBean.employeeType}" /> </td> </tr> </table> <p><h:commandButton value="Submit" action="confirm" /></p> </h:form> </h:body> </html>
employee.xhtml
accepts the employee data entered by the User such as Employee name, Gender, Date of Birth, Marital Status, Address, Email Address, Mobile Number, Designation, Department, Status of Employment (Permanent or Temporary) for further processing after the user clicks on Submit button.
confirm.xhtml
<?xml version="1.0" encoding="ISO-8859-1" ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:f="http://xmlns.jcp.org/jsf/core" xmlns:h="http://xmlns.jcp.org/jsf/html"> <h:head> <title>JSF Form Example</title> </h:head> <h:body> <h4>Confirmation Screen</h4> <h:form> <h4>Employee Information System</h4> <table> <tr> <td>Employee Name:</td> <td> <h:outputText value="#{employeeBean.empName}" /> </td> </tr> <tr> <td>Gender:</td> <td> <h:outputText value="#{employeeBean.gender}"/> </td> </tr> <tr> <td>Date of Birth:</td> <td> <h:outputText value="#{employeeBean.dob}" /> </td> </tr> <tr> <td>Marital Status:</td> <td> <h:outputText value="#{employeeBean.maritalStatus}" /> </td> </tr> <tr> <td>Address:</td> <td> <h:outputText value="#{employeeBean.address}" /> </td> </tr> <tr> <td>Email Address:</td> <td> <h:outputText value="#{employeeBean.emailAddress}" /> </td> </tr> <tr> <td>Mobile Number:</td> <td> <h:outputText value="#{employeeBean.mobileNumber}" /> </td> </tr> <tr> <td>Designation:</td> <td> <h:outputText value="#{employeeBean.designation}" /> </td> </tr> <tr> <td>Department:</td> <td> <h:outputText value="#{employeeBean.department}" /> </td> </tr> <tr> <td>Is Permanent?:</td> <td> <h:outputText value="#{employeeBean.employeeType}" /> </td> </tr> </table> <p><h:commandButton value="Cancel" action="employee" /> <h:commandButton value="Confirm" action="#{employeeBean.storeEmployeeInfo}" /></p> </h:form> </h:body> </html>
This confirm.xhtml
page displays the validated user-entered employee data with two buttons providing the options either to Cancel or Confirm. Clicking on the Cancel button will send the user back to the Employee form, whereas clicking on the Confirm button confirms the user input and redirects to the final “Success” page.
success.xhtml
<?xml version="1.0" encoding="ISO-8859-1" ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:f="http://xmlns.jcp.org/jsf/core" xmlns:h="http://xmlns.jcp.org/jsf/html"> <h:head> <title>A Simple JavaServer Faces Registration Application</title> </h:head> <h:body> <h4>Employee Information System</h4> <table> <tr> <td>Employee Name:</td> <td><h:outputText value="#{employeeBean.empName}" /></td> </tr> <tr> <td>Gender:</td> <td><h:outputText value="#{employeeBean.gender}" /></td> </tr> <tr> <td>Date of Birth:</td> <td><h:outputText value="#{employeeBean.dob}" /></td> </tr> <tr> <td>Marital Status:</td> <td><h:outputText value="#{employeeBean.maritalStatus}" /></td> </tr> <tr> <td>Address:</td> <td><h:outputText value="#{employeeBean.address}" /></td> </tr> <tr> <td>Email Address:</td> <td><h:outputText value="#{employeeBean.emailAddress}" /></td> </tr> <tr> <td>Mobile Number:</td> <td><h:outputText value="#{employeeBean.mobileNumber}" /></td> </tr> <tr> <td>Designation:</td> <td><h:outputText value="#{employeeBean.designation}" /></td> </tr> <tr> <td>Department:</td> <td><h:outputText value="#{employeeBean.department}" /></td> </tr> <tr> <td>Is Permanent?:</td> <td><h:outputText value="#{employeeBean.employeeType}" /></td> </tr> </table> </h:body> </html>
This success.xhtml
page indicates that the user information was successfully submitted along with a final display of the confirmed user information.
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5"> <display-name>JSF Form Example</display-name> <description>Employee Information System</description> <!-- Change to "Production" when you are ready to deploy --> <context-param> <param-name>javax.faces.PROJECT_STAGE</param-name> <param-value>Development</param-value> </context-param> <!-- Welcome page --> <welcome-file-list> <welcome-file>faces/employee.xhtml</welcome-file> </welcome-file-list> <!-- JSF Faces Servlet Mapping --> <servlet> <servlet-name>Faces Servlet</servlet-name> <servlet-class>javax.faces.webapp.FacesServlet</servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>Faces Servlet</servlet-name> <url-pattern>/faces/*</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>Faces Servlet</servlet-name> <url-pattern>*.jsf</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>Faces Servlet</servlet-name> <url-pattern>*.faces</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>Faces Servlet</servlet-name> <url-pattern>*.xhtml</url-pattern> </servlet-mapping> </web-app>
web.xml
– The Faces Controller is implemented as a servlet that responds to all the User requests conforming to a specified URL pattern, such as /faces/*, as defined in the web.xml
file. A request from the User that uses the appropriate Faces URL pattern can be considered a “Faces request,” and when received by the Faces Controller, it processes the request by preparing an object known as the JSF context, which contains all the application data and routes the User to the appropriate View component (JSF page).
The context param whose name is javax.faces.PROJECT_STAGE
. The value shown here is Development. This setting causes the JSF runtime to generate additional page aids when common developer mistakes are detected. Other values are Production, SystemTest, and UnitTest. The welcome-file url-pattern for the Faces Servlet, any request to the root of the application, such as http://localhost:8080/JSFEmployeeInformationForm/
will automatically take the user to the front page of the application, which is http://localhost:8080/JSFBackingBeanExample1/faces/employee.xhtml
.
5. Download the complete Source Code
You can download the full source code of this example here: JSF Form Example
/newjsf.xhtml @15,121 value=”#{mBeanjoyeria.nombre}”: Target Unreachable, identifier ‘mBeanjoyeria’ resolved to null
I Have this problem