Selenium with Python Example
1. Introduction
This is an article about using selenium with python. Selenium is used for functional testing. It supports python scripting.
2. Selenium with Python
2.1 Prerequisites
Python 3.6.8 is required on windows or any operating system. Pycharm is needed for python programming. Selenium and eclipse need to be installed for test script execution.
2.2 Download
Python 3.6.8 can be downloaded from the website. Pycharm is available at this link. Eclipse can be downloaded from this link.
2.3 Setup
2.3.1 Python Setup
To install python, the download package or executable needs to be executed.
2.3.2 Selenium Setup
You can install Selenium using the command below:
Selenium Setup
pip3 install Selenium
2.4 IDE
2.4.1 Pycharm Setup
Pycharm package or installable needs to be executed to install Pycharm.
2.5 Launching IDE
2.5.1 Pycharm
Launch the Pycharm and start creating a pure python project named HelloWorld. The screen shows the project creation.
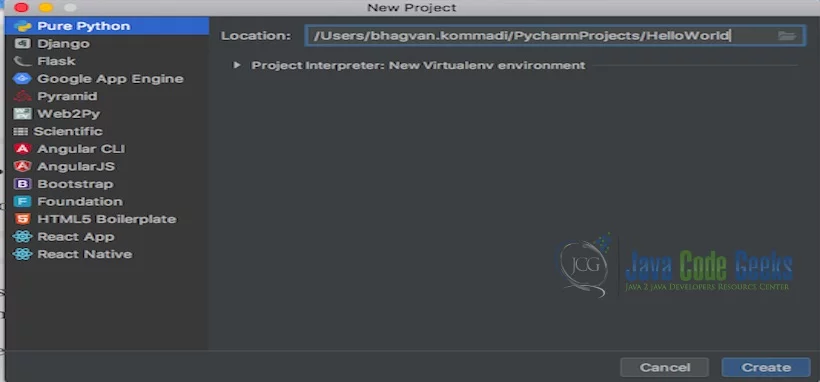
The project settings are set in the next screen as shown below.
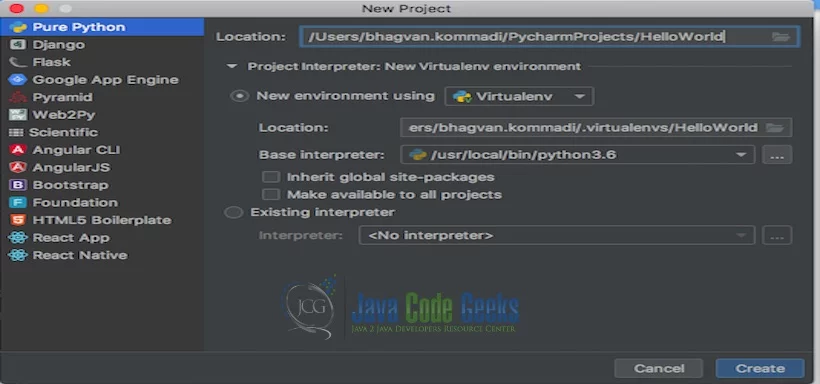
Pycharm welcome screen comes up shows the progress as indicated below.
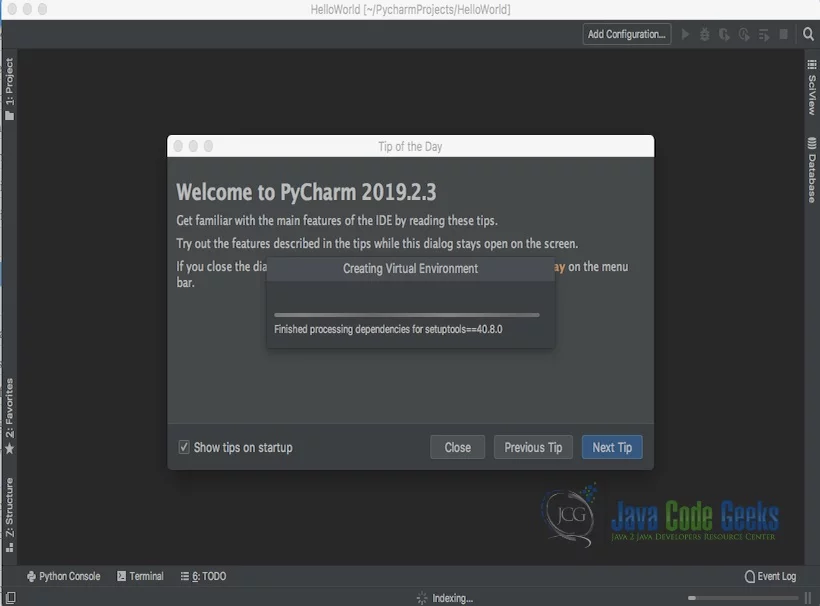
You can create a Hello.py and execute the python file by selecting the Run menu.
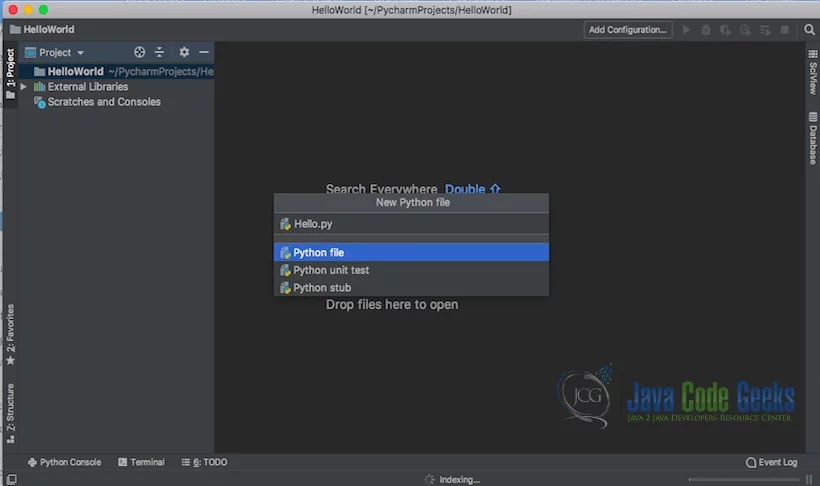
The output message “Hello World” is printed when the python file is Run.
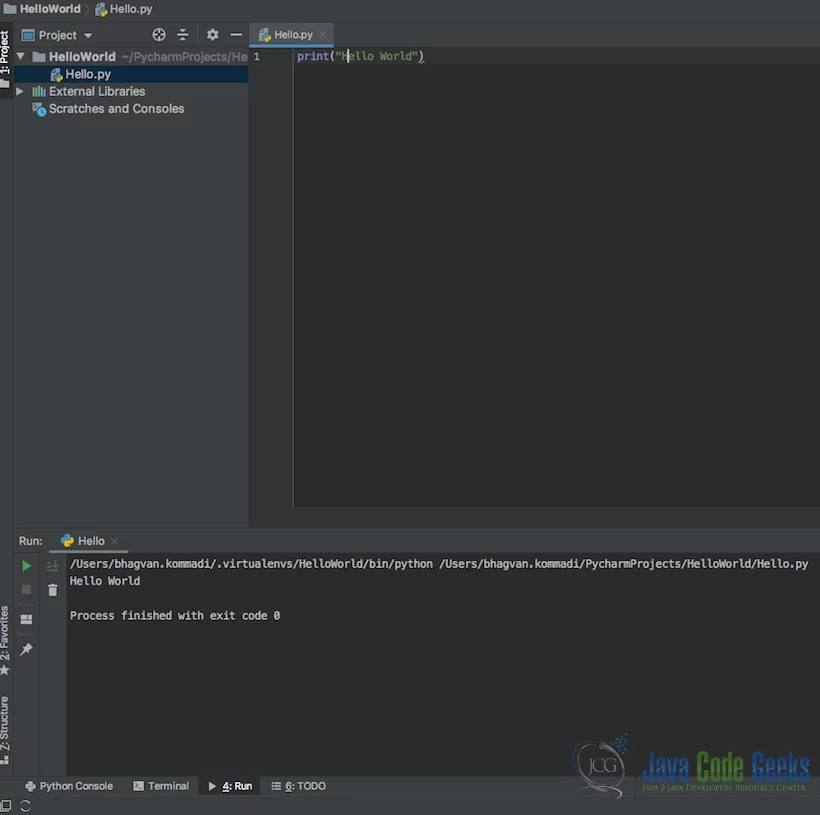
2.6 What is Python?
Python is a programming language used for developing software and web applications. It has Object-oriented programming constructs to create applications. Python is opensource. It is a scripting language that has an interpreter. Python helps in creating software applications quickly and debugging them.
2.7 What is Selenium?
Selenium is used for automation testing. It is used for creating automation tests from manual test cases. Selenium has support for Java, C#, Ruby, Perl, and Python. The test scripts can be executed with Selenium on Windows, Linux, and Mac OS. The test scripts can be executed on various browsers such as Chrome, Firefox, Edge, Safari, and others. Testing tools like TestNG and Junit can be integrated with Selenium test for test suite management and reports generation. Selenium has support for Maven, Jenkins, and Docker testing tools.
2.8 Why choose Python over Java in Selenium?
Python has better support for multiple programming paradigms. It has built-in testing frameworks like Pytest and Robot. Compared to java, python has better debugging and faster workflow. Python is a cross-platform language and can be used with other programming languages like C, C++, and Java. Selenium test scripts can be developed using PyDev in Eclipse and Selenium configured with Python. Selenium can be configured with python by installing selenium packages. Pycharm IDE can be used for Selenium Test scripts development and execution.
2.9 How to Install and Configure PyDev in Eclipse?
PyDev can be installed in Eclipse for creating Selenium test scripts. PyDev is installed by accessing the marketplace and searching in PyDev.
PyDev can be installed by clicking on the install button. The screen shot below shows the next screen.
You can create a new project by clicking on create a new project from the menu as shown below:
You can configure the interpreter for the PyDev project.
You can create a new package after creating the PyDev Project from the menu as shown below:
You can create a PyDev module by selecting the PyDev module from the menu:
2.10 How to Create Test Scripts in Selenium with Python
To execute the test scripts we will use the flask application created in this article. Flask App is executed as shown below:
Flask App
apples-MacBook-Air:flask bhagvan.kommadi$ source flaskenv/bin/activate (flaskenv) apples-MacBook-Air:flask bhagvan.kommadi$ ls Customer.py customers.db flaskexample.py templates CustomerData.py flaskenv history.txt (flaskenv) apples-MacBook-Air:flask bhagvan.kommadi$ python3 Customer.py * Serving Flask app "Customer" (lazy loading) * Environment: production WARNING: This is a development server. Do not use it in a production deployment. Use a production WSGI server instead. * Debug mode: on * Running on http://127.0.0.1:5000/ (Press CTRL+C to quit) * Restarting with stat * Debugger is active! * Debugger PIN: 981-329-817 127.0.0.1 - - [30/Mar/2021 12:14:55] "GET / HTTP/1.1" 200 - 127.0.0.1 - - [30/Mar/2021 12:16:32] "GET /add HTTP/1.1" 200 - 127.0.0.1 - - [30/Mar/2021 12:19:23] "GET / HTTP/1.1" 200 - 127.0.0.1 - - [30/Mar/2021 12:19:23] "GET /favicon.ico HTTP/1.1" 404 - 127.0.0.1 - - [30/Mar/2021 12:19:24] "GET /add HTTP/1.1" 200 - 127.0.0.1 - - [30/Mar/2021 12:22:22] "GET / HTTP/1.1" 200 - 127.0.0.1 - - [30/Mar/2021 12:22:23] "GET /favicon.ico HTTP/1.1" 404 - 127.0.0.1 - - [30/Mar/2021 12:22:23] "GET /add HTTP/1.1" 200 - 127.0.0.1 - - [30/Mar/2021 12:25:59] "GET / HTTP/1.1" 200 - 127.0.0.1 - - [30/Mar/2021 12:25:59] "GET /favicon.ico HTTP/1.1" 404 - 127.0.0.1 - - [30/Mar/2021 12:25:59] "GET /add HTTP/1.1" 200 - 127.0.0.1 - - [30/Mar/2021 12:26:08] "POST /savedetails HTTP/1.1" 200 - 127.0.0.1 - - [30/Mar/2021 12:26:41] "GET / HTTP/1.1" 200 - 127.0.0.1 - - [30/Mar/2021 12:26:41] "GET /favicon.ico HTTP/1.1" 404 - 127.0.0.1 - - [30/Mar/2021 12:26:41] "GET /add HTTP/1.1" 200 -
The below test script is used in PyDev project for execution.
Selenium Test Script
''' Created on Mar 30, 2021 @author: bhagvan.kommadi ''' from selenium import webdriver import time from selenium.webdriver.common.keys import Keys from webdriver_manager.chrome import ChromeDriverManager driver = webdriver.Chrome(ChromeDriverManager().install()) print("test case starting now") driver.maximize_window() driver.delete_all_cookies() driver.get("http://localhost:5000") driver.find_element_by_xpath("//a[contains(text(),'Add Customer')][1]").click() time.sleep(4) driver.find_element_by_xpath("//input[@type='text'][@name='name'][1]").send_keys("Jack Hill") time.sleep(2) driver.find_element_by_xpath("//input[@type='email'][@name='email'][1]").send_keys("jack@gmail.com") time.sleep(2) driver.find_element_by_xpath("//input[@type='text'][@name='address'][1]").send_keys("345 Hill Drive, Sanjose 30489") time.sleep(2) driver.find_element_by_xpath("//input[@type='submit'][@value='Submit'][1]").click() time.sleep(2) driver.close() print("Customer creation")
Chrome driver is downloaded into the project from this link. Webdrivermanager is installed using the command below:
Web Driver Manager
pip3 install webdriver-manager
The code is executed as shown below:
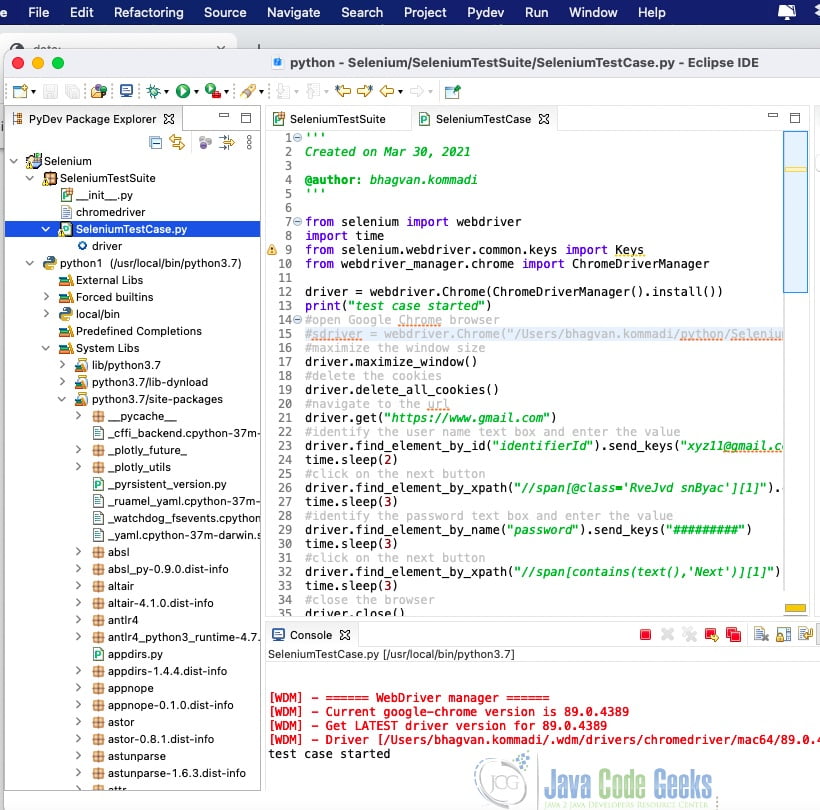
The output is shown in the next set of images below.

The next page is reached by the selenium by clicking on the link – Add Customer.

The next page comes when script clicks on the submit button. The screen shot below shows the last page.

3. More articles
- Python Tutorial for Beginners
- Python Random Module Tutorial
- Python input() method Tutorial
- Queue in Python
- Python RegEx Tutorial
- Python JSON Example
- Introduction to the Flask Python Web App Framework
4. Download the Source Code
You can download the full source code of this example here: Selenium with Python Example
Last updated on May 31st, 2021