Microservices Implementation using Spring Boot and Cloud
Welcome readers, in this tutorial, we will see the theoretical concept of implementing Microservices using Spring Boot and Cloud.
1. Introduction
In recent years, Microservices architecture (popularly known as MSA) has been the favored choice for developing an application due to its independent development plus deployment and the resiliency of the application. So here, in this tutorial, I will try to explain the concept of implementing Microservices using Spring Boot and Cloud. Let us take a look at each component.
1.1 What is Spring boot?
- Spring boot is a module that provides rapid application development feature to the spring framework including auto-configuration, standalone-code, and production-ready code
- It creates applications that are packaged as jar and are directly started using embedded servlet container (such as Tomcat, Jetty or Undertow). Thus, no need to deploy the war files
- It simplifies the maven configuration by providing the starter template and helps to resolve the dependency conflicts. It automatically identifies the required dependencies and imports them in the application
- It helps in removing the boilerplate code, extra annotations, and XML configurations
- It provides a powerful batch processing and manages the rest endpoints
- It provides an efficient JPA-starter library to effectively connect the application with the relational databases
- It offers a Microservice architecture and cloud configuration that manages all the application related configuration properties in a centralized manner.
In the following diagram, I explain how the microservices are built using Spring boot and are managed using Spring Cloud.
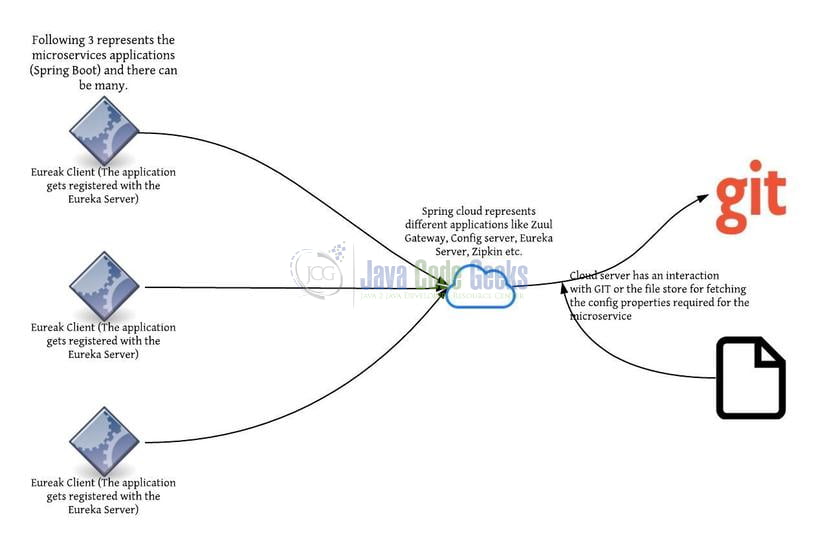
1.2 Zuul Gateway
Zuul Gateway is a front door for all requests coming to a backend application. It enables dynamic routing, monitoring, security, and resiliency to an application. It is a router that provides a single-entry point to our application without managing the CORS (Cross-origin Resource Sharing) and authentication for each microservice of an application. It easily interacts with other cloud components like Eureka server for service discovery, Hystrix for fault tolerance mechanism, and Config server for configuration details. The following diagram quickly summarizes the Zuul Gateway.
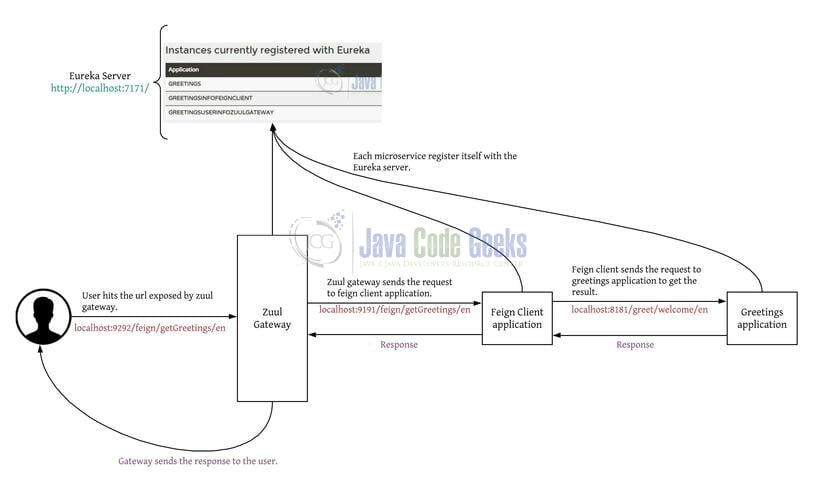
You can take a look at this tutorial under the practical implementation of Spring Cloud Zuul gateway.
1.3 Config Server
During the application development in a microservice-based architecture, many configurations are present for the different environments (i.e. DEV, TEST, PROD). These configurations help to achieve a faster development approach where the application in itself knows about which configuration file to pick based on the hosting environment. To achieve this type of setup there has to be a central place that allows this quick way of working things (i.e. changing the configuration at a single place and voila, the appropriate microservice adapts to it). The following diagram quickly summarized the Config Server.
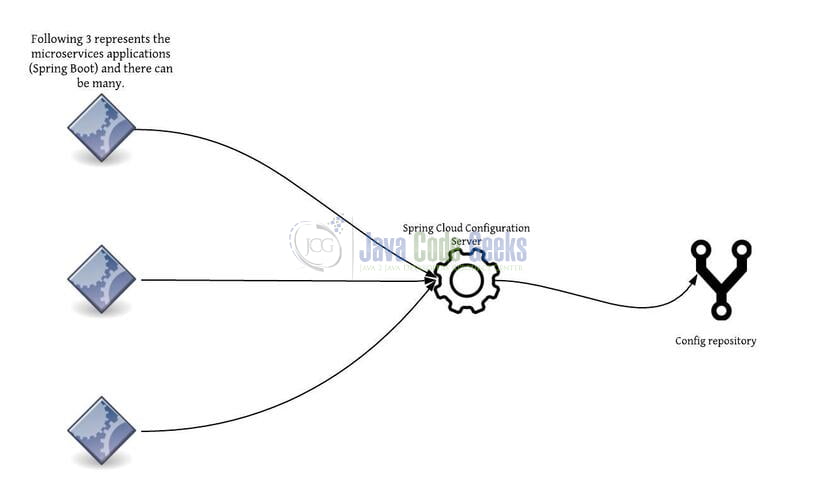
You can take a look at this tutorial under the practical implementation of the Spring Cloud Config Server.
1.4 Service Registry
The Service Registry is divided into two parts i.e. Eureka Server and Eureka Client. Let us understand some basic points about the Eureka Server –
- It is a Service Registration and Discovery application that holds the information about all the other microservices and is popularly known a Discovery server
- Every Microservice registers itself into the Eureka server and is known as Discovery client
- Eureka server knows the client microservices running status, port number, and IP address
These days the Eureka Client plays an important role as mostly the microservices are getting deployed on the containerized environment where the number of service instances and their location changes dynamically. The following diagram quickly summarized the Service Registry.
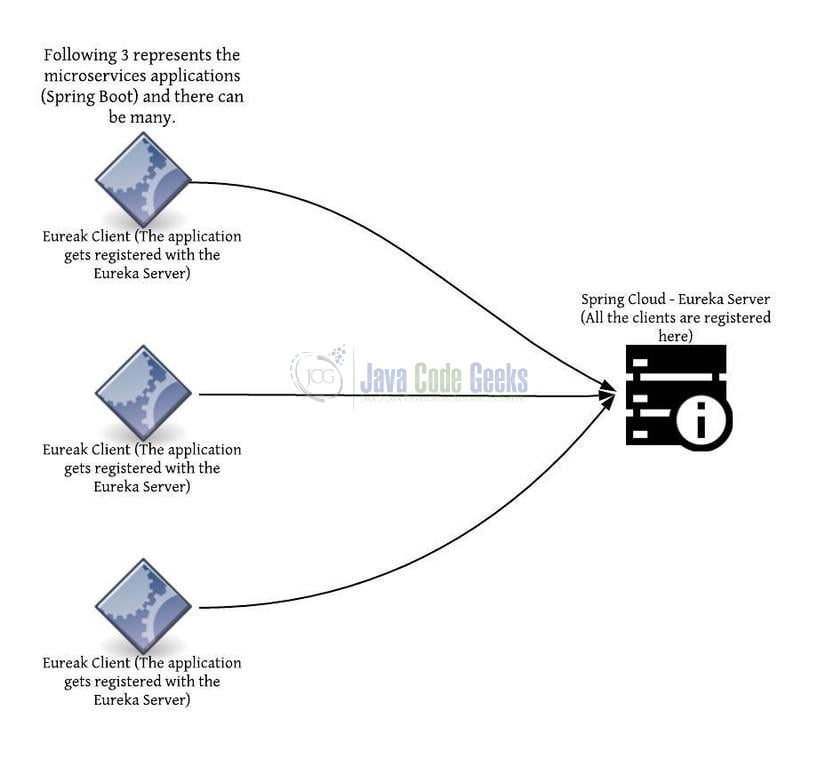
You can take a look at this tutorial under the practical implementation of Service Registry in Microservice architecture.
1.5 Zipkin Server
Spring Cloud Sleuth and Zipkin are useful tools when one microservice talks to another microservice hosted in a different location (i.e. each service has its log). This approach requires the extensive use of correlation id which helps to map the request chaining.
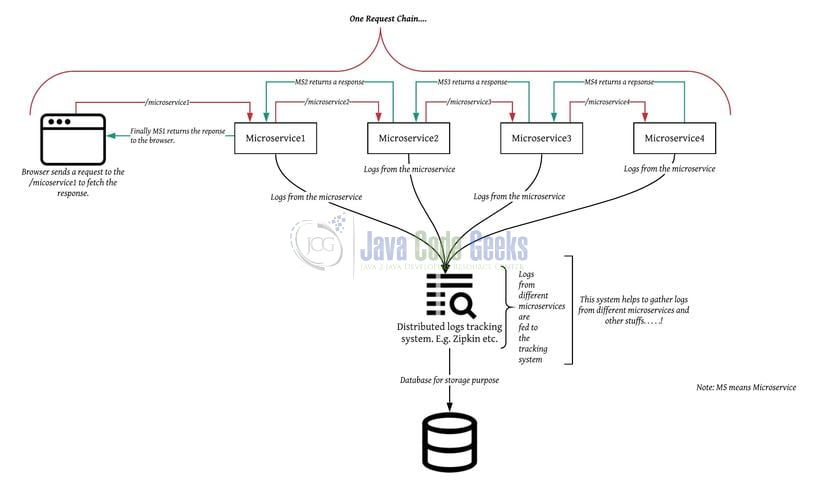
Getting back to the discussion, to resolve issues like distributed log tracing, latency problems, etc. Sleuth and Zipkin came into the picture.
- Here the Cloud Sleuth generates the attach the trace id, span id to the logs so that it can be used by tools like Zipkin and ELK for storage and analysis
- Later Zipkin which is a distributed tracing system gathers this data and helps to troubleshoot the latency problems presents in the Microservices architecture
You can take a look at this tutorial under the practical implementation of Zipkin Server in Microservice architecture.
1.6 Circuit Breaker
Netflix Hystrix or Circuit Breaker is a commonly used component in the Microservice architecture for handling the fault tolerance of a microservice. The following diagram quickly summarizes the circuit breaker pattern.
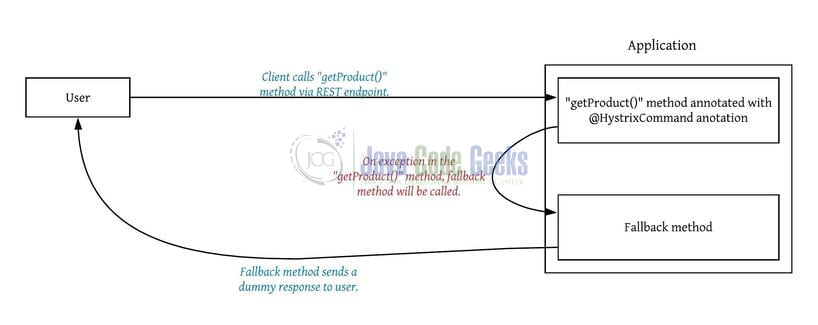
You can at a look at this tutorial under the practical implementation of Circuit Breaker in Microservice architecture.
1.7 Feign Client
Netflix Feign Client is a client binder for implementing the declarative REST client in a microservices architecture. The following diagram quickly summarizes the Feign Client.
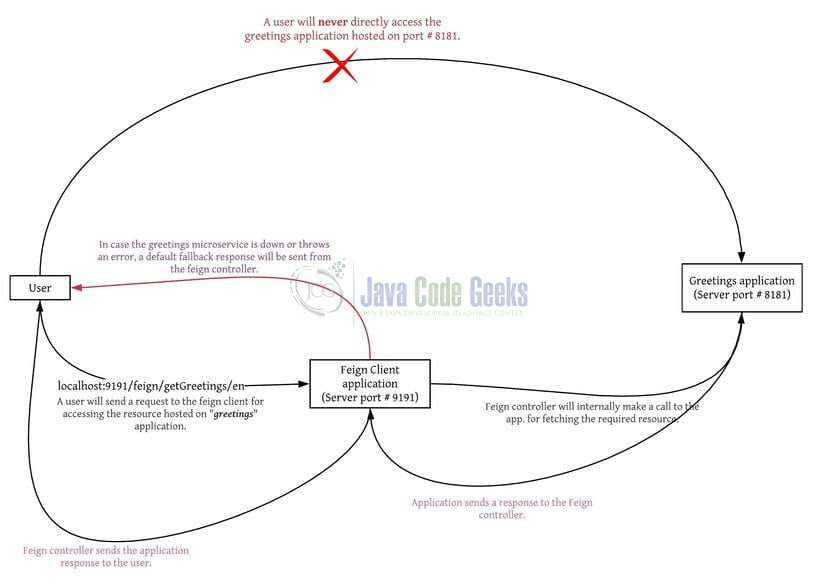
You can at a look at this tutorial under the practical implementation of Feign Client in Microservice architecture.
That is all for this tutorial and I hope the article served you whatever you were looking for. Happy Learning and do not forget to share!
2. Summary
In this section, we learned:
- An extract to Microservices and its components
- Introduction to Spring Boot, Zuul Gateway, Config Server, Service Registry, Zipkin Server, Circuit Breaker, and Feign Client
You can either navigate to the links shared above or you can download the sample application as an Eclipse project in the Downloads section.
3. Download the Eclipse Project
This was an example of implementing Microservices using Spring Boot and Cloud.
You can download the full source code of this example here: Microservices Implementation using Spring Boot and Cloud