Java Tuple Example
Hello readers, in this tutorial, we will learn about Tuple collection in Java.
1. Introduction
A Tuple
in Java is an ordered collection of different types of objects. The objects individually do not have an existing but collectively they have a meaning. Java in its real form but does not provide a Tuple functionality although there are different ways to achieve such kind of functionality like creating a class that works like a Tuple. Let us see the sample Tuple snippets –
Sample Examples
1 2 3 | [Chloe, Decker, 28 ] [John, Mathew, 40 , Senior Vice President] [ 1 , Jane, Doe, 50000.50 ] |
1.1 Maven Library
To have the Tuple in your application, developers need to include the following library in their project.
Employee.java
1 2 3 4 5 6 | <!-- java tuples library --> < dependency > < groupId >org.javatuples</ groupId > < artifactId >javatuples</ artifactId > < version >1.2</ version > </ dependency > |
1.2 Tuple vs. Lists/Array
- A Tuple contains the different set of type while Lists/Array store elements of the same type
- Tuples consume less memory as compared in Collection(s) in Java language
- A Tuple is immutable in Java while Array and List are mutable in nature
- Tuples are fixed in size and are effective when the collection will change only once in a lifetime
1.3 Classes supported by Tuples
Tuple support classes of size up to 10
i.e.
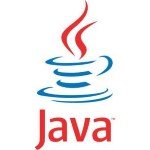
- Unit (One element)
- Pair (Two elements)
- Triplet (Three elements)
- Quartet (Four elements)
- Quintet (Five elements)
- Sextet (Six elements)
- Septet (Seven elements)
- Octet (Eight elements)
- Ennead (Nine elements)
- Decade (Ten elements)
2. Java Tuple Example
Let us understand the different tuples and various methods in the tuple library of Java through the sample code.
- Creating a tuple
- Inserting/Removing/Modifying tuple
- Fetching value(s) from tuple
- Iterating a tuple
Tuples.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 | package com.java.tuple; import java.util.Arrays; import java.util.List; import org.javatuples.Pair; import org.javatuples.Quartet; import org.javatuples.Triplet; import org.javatuples.Unit; public class Tuples { public static void main(String[] args) { // Create a tuple. // Creating a tuple through "with" factory method. final Pair<String, String> pair = Pair.with( "Pair1" , "Value1" ); System.out.println( "Pair= " + pair); System.out.println( "========================================" ); // Creating a tuple through collection. final List<String> names = Arrays.asList( "John" , "Mary" , "Jane" , "Sharon" ); // Creating a quartet from the collection. // Always remember the number of elements in the collection should match the tuple type. final Quartet<String, String, String, String> quartet = Quartet.fromCollection(names); System.out.println( "Quartet= " + quartet); System.out.println( "========================================" ); // Insert element to a tuple. final Pair<String, Integer> nameAgePair = Pair.with( "Derek" , 20 ); // Adding the job profession information to the pair using triplet. final Triplet<String, Integer, String> triplet = nameAgePair.add( "Accountant" ); System.out.println( "Name age pair= " + nameAgePair); System.out.println( "Using triplet= " + triplet); System.out.println( "========================================" ); // Exploring the addAtx() method to add a value at the particular index. final Quartet<String, String, Integer, String> quartet2 = triplet.addAt1( "Mathew" ); System.out.println( "Exploring the addAt()= " + quartet2); System.out.println( "========================================" ); // Getting a value from a tuple and iterating it. final Quartet<String, Integer, String, Double> quartet3 = Quartet.with( "Abc" , 30 , "Pqr" , 23000.5 ); // Getting a value from the tuple. System.out.println( "Name= " + quartet3.getValue0()); System.out.println( "Age= " + quartet3.getValue1()); System.out.println( "Address= " + quartet3.getValue2()); System.out.println( "Sal= " + quartet3.getValue3()); // Getting value from the index. final int position = 0 ; System.out.println( "[Get value from an index] Name= " + quartet3.getValue(position)); // Iteration. quartet3.forEach((value) -> System.out.println( "Value= " + value)); System.out.println( "========================================" ); // Modifying a tuple. final Pair<String, String> originalPair = Pair.with( "Chloe" , "Decker" ); System.out.println( "Original pair= " + originalPair); final Pair<String, String> modifiedPair = originalPair.setAt1( "Lucifer" ); System.out.println( "Modified pair= " + modifiedPair); System.out.println( "========================================" ); // Removing from a tuple. // Note - The usage of removex() method is not proper as internally removing from 1 it removed the element from 0 position // and returns it. final Unit<String> removedUnit = modifiedPair.removeFrom1(); System.out.println( "Removed value= " + removedUnit.getValue0()); } } |
Output
Pair= [Pair1, Value1] ======================================== Quartet= [John, Mary, Jane, Sharon] ======================================== Name age pair= [Derek, 20] Using triplet= [Derek, 20, Accountant] ======================================== Exploring the addAt()= [Derek, Mathew, 20, Accountant] ======================================== Name= Abc Age= 30 Address= Pqr Sal= 23000.5 [Get value from an index] Name= Abc Value= Abc Value= 30 Value= Pqr Value= 23000.5 ======================================== Original pair= [Chloe, Decker] Modified pair= [Chloe, Lucifer] ======================================== Removed value= Chloe
That is all for this tutorial and I hope the article served you with whatever you were looking for. Happy Learning and do not forget to share!
3. Summary
In this tutorial, we learned the following,
- Introduction to Tuple and sample examples
- How to use Tuple in an application
- Difference between Tuple and Lists/Arrays
- Different class types supported by Tuple, and
- A simple example to understand the basic functionality of Tuples
Developers can download the sample class from the Downloads section.
4. More articles
- Java Tutorial for Beginners (with video)
- Java Collections Tutorial
- ArrayList Java Example – How to use ArrayList (with video)
- Java List Example
- Java Array – java.util.Arrays Example (with Video)
5. Download the Eclipse Project
This was an example of Tuples in Java.
You can download the full source code of this example here: Java Tuple Example
Last updated on Jun. 15th, 2021