Java Cheat Sheet
In this post, you will find an easy syntax cheat sheet for Java programming. We will see the Java features and the API classes of Java in detail.
1. Overview
We will look at the features of Java and its basic syntax.
Java is used for developing software and executing the code. Java code is converted into bytecode after compilation. The java interpreter runs the bytecode and the output is created.
Table Of Contents
- 1. Overview
- 2. Java Cheat Sheet
- 2.1. Prerequisites
- 2.2. Download
- 2.3. Setup
- 2.4. IDE
- 2.5. Launching IDE
- 2.6.Hello World Program
- 2.7.Data Types – Declare Variable
- 2.8. Explanation
- 2.9. Operators
- 2.10. If else – switch
- 2.11.For loop-While loop,do while – break -continue
- 2.12. Arrays
- 2.13. Objects
- 2.14.Inheritance
- 2.15.Encapsulation
- 2.16.Important keywords in Java
- 2.17.Classes
- 2.18.Annotations
- 2.19.File Operations
- 2.20.Polymorphism
- 2.21.Typecasting
- 2.22.Abstract Class
- 2.23.Comments
- 3. Download the Source Code
2. Java Syntax Cheat Sheet
Java language is an object-oriented language. It is platform-independent and architectural neutral. Cheat sheets about Java syntax are useful for beginners. They help in saving time and achieving the specified work. Cheat sheets help in improving productivity.
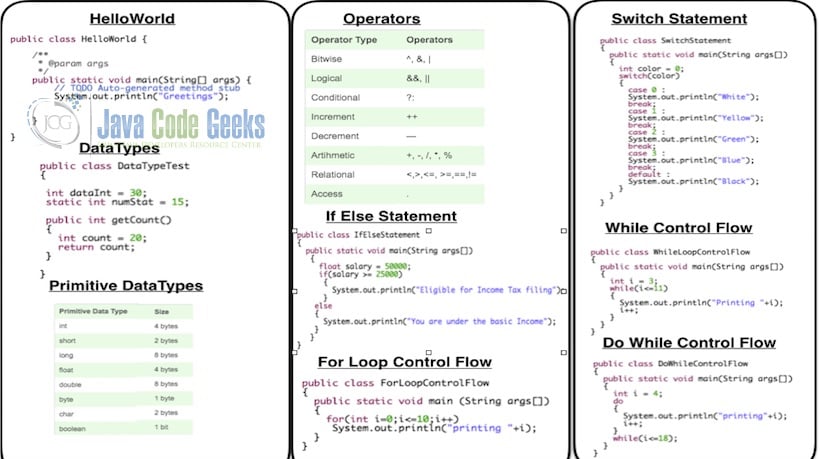
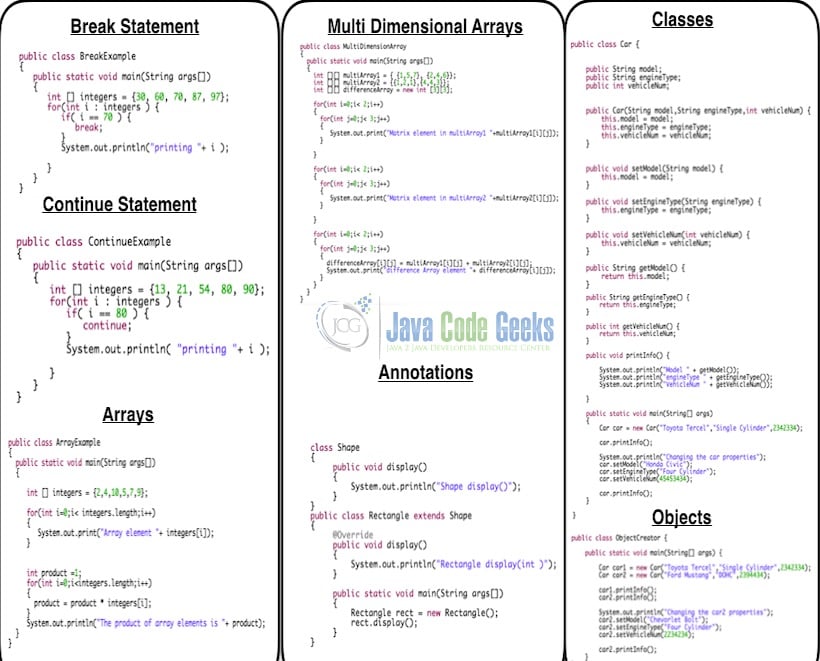
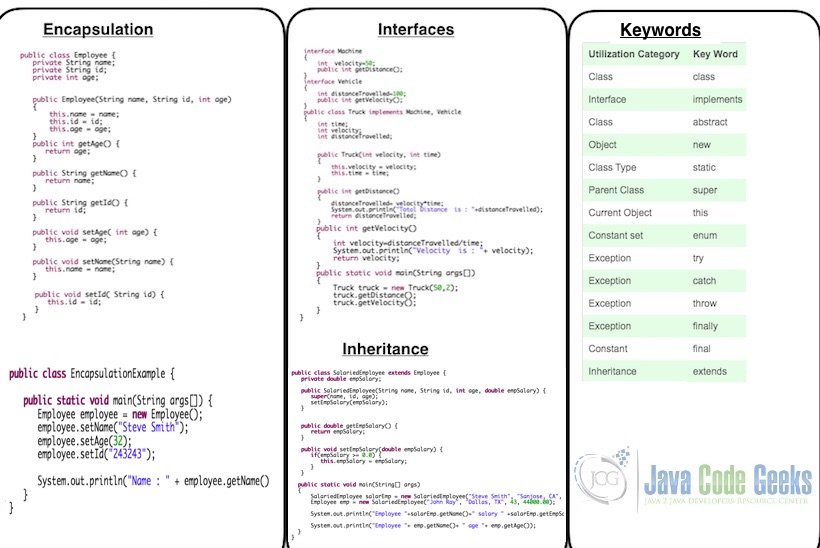

You can learn more about the basic syntax of Java in this article.
2.1 Prerequisites
Java 8 is required on the linux, windows or mac operating system. Eclipse Oxygen can be used for this example.
2.2 Download
You can download Java 8 from the Oracle web site . Eclipse Oxygen can be downloaded from the eclipse web site.
2.3 Setup
Below is the setup commands required for the Java Environment.
Java Setup
JAVA_HOME=”/jboss/jdk1.8.0_73″ export JAVA_HOME PATH=$JAVA_HOME/bin:$PATH export PATH
2.4 IDE
The ‘eclipse-java-oxygen-2-macosx-cocoa-x86_64.tar’ can be downloaded from the eclipse website. The tar file is opened by double click. The tar file is unzipped by using the archive utility. After unzipping, you will find the eclipse icon in the folder. You can move the eclipse icon from the folder to applications by dragging the icon.
2.5 Launching IDE
Eclipse has features related to language support, customization, and extension. You can click on the eclipse icon to launch eclipse. You can select the workspace from the screen which pops up. You can see the eclipse workbench on the screen.
2.6 Hello World Program
Java Hello World
program code is presented below. The class has main
method which prints out the greetings message. System.out.println
is used for printing the messages.
Hello World
public class HelloWorld { /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub System.out.println("Greetings"); } }
You can also check our Java Hello World Example.
2.7 Data Types – Declare Variable
Java language has primitive and object types. Java has features related to autoboxing which converts the types automatically. The java.lang.Object
class is the base class for all the classes and Java follows the single root chain of command. The code below shows the instantiation of primitive data type int. The method in the class DataTypeTest
returns the int value.
Data Types
public class DataTypeTest { int dataInt = 30; static int numStat = 15; public int getCount() { int count = 20; return count; } }
You can also check this Java Data Types Example.
2.8 Explanation of Primitive Data Types
Java language has primitive data types such as int, short, long, float, char, boolean, double, and byte data types.
The table shows the various primitive data types and their size values.
Primitive Data Type | Size |
int | 4 bytes |
short | 2 bytes |
long | 8 bytes |
float | 4 bytes |
double | 8 bytes |
byte | 1 byte |
char | 2 bytes |
boolean | 1 bit |
You can also check our Java Primitive Types Tutorial.
2.9 Operators
The table below shows the operators for different operator types.
Operator Type | Operators |
Bitwise | ^, &, | |
Logical | &&, || |
Conditional | ?: |
Increment | ++ |
Decrement | — |
Artihmetic | +, -, /, *, % |
Relational | <,>,<=, >=,==,!= |
Access | . |
You can also check our article related to the Basic Java Operators.
2.10 If else – switch
If else statement checks the condition. When the condition is true, the block under the if statement gets executed. Otherwise, the block under the else gets executed. The sample code below shows the If Else statement example.
If Else Statement
public class IfElseStatement { public static void main(String args[]) { float salary = 50000; if(salary >= 25000) { System.out.println("Eligible for Income Tax filing"); } else { System.out.println("You are under the basic Income"); } } }
The condition under the switch statement is checked. When the condition passes a specific case, the block under the case is executed. The case conditions which are left out are not executed. After the condition is met, the code under the switch loop is stopped.
Switch Statement
public class SwitchStatement { public static void main(String args[]) { int color = 0; switch(color) { case 0 : System.out.println("White"); break; case 1 : System.out.println("Yellow"); break; case 2 : System.out.println("Green"); break; case 3 : System.out.println("Blue"); break; default : System.out.println("Black"); } } }
Check this Simple if else Java Example to learn more.
2.11 For loop, While loop, do while, break ,continue
In the For loop, the block of code is iterated for a given number of times till the condition in the for statement is true. The sample code shows the execution of the code in the for loop.
For Loop
public class ForLoopControlFlow { public static void main (String args[]) { for(int i=0;i<=10;i++) System.out.println("printing "+i); } }
The block of code within the while loop is executed till the condition is true in the while statement. The sample code shows the while loop example.
While Loop
public class WhileLoopControlFlow { public static void main(String args[]) { int i = 3; while(i<=11) { System.out.println("Printing "+i); i++; } } }
The loop code block under the do statement gets executed till the while condition is true. The code presented below shows the do while statement usage.
Do While Statement
public class DoWhileControlFlow { public static void main(String args[]) { int i = 4; do { System.out.println("printing"+i); i++; } while(i<=18); } }
Break statement is executed within a loop and the loop gets stopped. The control flow starts at the next statement after the loop. The code below shows the example for the usage of the break statement.
Break Statment
public class BreakExample { public static void main(String args[]) { int [] integers = {30, 60, 70, 87, 97}; for(int i : integers ) { if( i == 70 ) { break; } System.out.println("printing "+ i ); } } }
continue statement in the control flow moves the control to the update statement. The sample code below shows the continue statement usage.
Continue
public class ContinueExample { public static void main(String args[]) { int [] integers = {13, 21, 54, 80, 90}; for(int i : integers ) { if( i == 80 ) { continue; } System.out.println( "printing "+ i ); } } }
2.12 Arrays
Array is related to a set of same type instances. Array has continuous memory. Array can have primitive data values and objects. The data can be sorted efficiently and the access can be random. In array, the size of the elements is fixed. The sample code shows the usage of one dimensional Arrays.
Array Example
public class ArrayExample { public static void main(String args[]) { int [] integers = {2,4,10,5,7,9}; for(int i=0;i< integers.length;i++) { System.out.print("Array element "+ integers[i]); } int product =1; for(int i=0;i<integers.length;i++) { product = product * integers[i]; } System.out.println("The product of array elements is "+ product); } }
The sample code below shows the usage of Multi Dimensional Arrays.
MultiDimension Array
public class MultiDimensionArray { public static void main(String args[]) { int [][] multiArray1 = { {1,5,7}, {2,4,6}}; int [][] multiArray2 = {{1,2,1},{4,4,3}}; int [][] differenceArray = new int [3][3]; for(int i=0;i< 2;i++) { for(int j=0;j< 3;j++) { System.out.print("Matrix element in multiArray1 "+multiArray1[i][j]); } } for(int i=0;i< 2;i++) { for(int j=0;j< 3;j++) { System.out.print("Matrix element in multiArray2 "+multiArray2[i][j]); } } for(int i=0;i< 2;i++) { for(int j=0;j< 3;j++) { differenceArray[i][j] = multiArray1[i][j] + multiArray2[i][j]; System.out.print("difference Array element "+ differenceArray[i][j]); } } } }
You can also check our Java Array – java.util.Arrays Example.
2.13 Objects
Objects are units of data and used to modify the data. A collection of objects is referred to as a class if they are of the same type. Classes are used to represent entities and objects are instances of the entity type. Let us look at the Car
Class implementation below:
Car Class
public class Car { public String model; public String engineType; public int vehicleNum; public Car(String model,String engineType,int vehicleNum) { this.model = model; this.engineType = engineType; this.vehicleNum = vehicleNum; } public void setModel(String model) { this.model = model; } public void setEngineType(String engineType) { this.engineType = engineType; } public void setVehicleNum(int vehicleNum) { this.vehicleNum = vehicleNum; } public String getModel() { return this.model; } public String getEngineType() { return this.engineType; } public int getVehicleNum() { return this.vehicleNum; } public void printInfo() { System.out.println("Model " + getModel()); System.out.println("engineType " + getEngineType()); System.out.println("VehicleNum " + getVehicleNum()); } public static void main(String[] args) { Car car = new Car("Toyota Tercel","Single Cylinder",2342334); car.printInfo(); System.out.println("Changing the car properties"); car.setModel("Honda Civic"); car.setEngineType("Four Cylinder"); car.setVehicleNum(45453434); car.printInfo(); } }
Now, we can look at object creation by instantiating the Car
class below:
Object Creator
public class ObjectCreator { public static void main(String[] args) { Car car1 = new Car("Toyota Tercel","Single Cylinder",2342334); Car car2 = new Car("Ford Mustang","DOHC",2394434); car1.printInfo(); car2.printInfo(); System.out.println("Changing the car2 properties"); car2.setModel("Chevorlet Bolt"); car2.setEngineType("Four Cylinder"); car2.setVehicleNum(2234234); car2.printInfo(); } }
2.14 Inheritance
Inheritance is related to the derivation of the properties of a subclass from the parent class. It helps in making the classes reusable. The sample code shows the Employee class implementation.
Employee Class
public class Employee { private String name; private String id; private int age; public Employee(String name, String id, int age) { this.name = name; this.id = id; this.age = age; } public int getAge() { return age; } public String getName() { return name; } public String getId() { return id; } public void setAge( int age) { this.age = age; } public void setName(String name) { this.name = name; } public void setId( String id) { this.id = id; } }
Now, let us look at Salaried Employee class which extends the Employee class.
Salaried Employee Class
public class SalariedEmployee extends Employee { private double empSalary; public SalariedEmployee(String name, String id, int age, double empSalary) { super(name, id, age); setEmpSalary(empSalary); } public double getEmpSalary() { return empSalary; } public void setEmpSalary(double empSalary) { if(empSalary >= 0.0) { this.empSalary = empSalary; } } public static void main(String[] args) { SalariedEmployee salarEmp = new SalariedEmployee("Steve Smith", "Sanjose, CA", 33, 56000.00); Employee emp = new SalariedEmployee("John Ray", "Dallas, TX", 43, 44000.00); System.out.println("Employee "+salarEmp.getName()+" salary " +salarEmp.getEmpSalary()); System.out.println("Employee "+ emp.getName()+ " age "+ emp.getAge()); } }
Multiple inheritance is not supported in java. Each class can extend only on one class but can implement more than one interfaces. Truck class can implement multiple interfaces Machine and Vehicle.
Truck Class
interface Machine { int velocity=50; public int getDistance(); } interface Vehicle { int distanceTravelled=100; public int getVelocity(); } public class Truck implements Machine, Vehicle { int time; int velocity; int distanceTravelled; public Truck(int velocity, int time) { this.velocity = velocity; this.time = time; } public int getDistance() { distanceTravelled= velocity*time; System.out.println("Total Distance is : "+distanceTravelled); return distanceTravelled; } public int getVelocity() { int velocity=distanceTravelled/time; System.out.println("Velocity is : "+ velocity); return velocity; } public static void main(String args[]) { Truck truck = new Truck(50,2); truck.getDistance(); truck.getVelocity(); } }
Check our Java Inheritance Example to learn more.
2.15 Encapsulation
Encapsulation is related to data wrapping and methods enclosure into a unit. It is also called data hiding. Let us look at the Employee
class implementation below:
Employee Class
public class Employee { private String name; private String id; private int age; public Employee(String name, String id, int age) { this.name = name; this.id = id; this.age = age; } public int getAge() { return age; } public String getName() { return name; } public String getId() { return id; } public void setAge( int age) { this.age = age; } public void setName(String name) { this.name = name; } public void setId( String id) { this.id = id; } }
Now look at the encapsulation example below. The properties Name
, Age
and Id
are set through setter methods and accessed through getter methods.
Encapsulation Example
public class EncapsulationExample { public static void main(String args[]) { Employee employee = new Employee(); employee.setName("Steve Smith"); employee.setAge(32); employee.setId("243243"); System.out.println("Name : " + employee.getName() + " Age : " + employee.getAge()+ " Id : " + employee.getId()); } }
You can check our Encapsulation Java Example for further knowledge.
2.16 Important keywords in Java
The table below shows the key words in java.
Utilization Category | Key Word |
Class | class |
Interface | implements |
Class | abstract |
Object | new |
Class Type | static |
Parent Class | super |
Current Object | this |
Constant set | enum |
Exception | try |
Exception | catch |
Exception | throw |
Exception | finally |
Constant | final |
Inheritance | extends |
2.17 Classes
A class is related to a set of objects which have similar properties and can be created by using a defined prototype. The methods of the class are used to identify the behavior of the objects. Let us look at the Car
class implementation.
Car Class
public class Car { public String model; public String engineType; public int vehicleNum; public Car(String model,String engineType,int vehicleNum) { this.model = model; this.engineType = engineType; this.vehicleNum = vehicleNum; } public void setModel(String model) { this.model = model; } public void setEngineType(String engineType) { this.engineType = engineType; } public void setVehicleNum(int vehicleNum) { this.vehicleNum = vehicleNum; } public String getModel() { return this.model; } public String getEngineType() { return this.engineType; } public int getVehicleNum() { return this.vehicleNum; } public void printInfo() { System.out.println("Model " + getModel()); System.out.println("engineType " + getEngineType()); System.out.println("VehicleNum " + getVehicleNum()); } public static void main(String[] args) { Car car = new Car("Toyota Tercel","Single Cylinder",2342334); car.printInfo(); System.out.println("Changing the car properties"); car.setModel("Honda Civic"); car.setEngineType("Four Cylinder"); car.setVehicleNum(45453434); car.printInfo(); } }
Java API has collection classes that implement the Collection interface and the other subinterfaces. These classes implement Map, List, Set, Queue, Serializable, Cloneable, and Iterator interfaces. Stronger type checking is provided by the generic collections in java.
You can also check our Java Classes and Objects Tutorial.
2.18 Annotations
Annotations help in providing additional information about the code. They start with the symbol ‘@’. Annotations do not modify the compiled code behavior. They associate the information of the code elements with the elements such as classes, properties, methods, and constructors. Below is the sample which shows the Rectangle class over riding the display method with an annotation.
Rectangle Class
class Shape { public void display() { System.out.println("Shape display()"); } } public class Rectangle extends Shape { @Override public void display() { System.out.println("Rectangle display(int )"); } public static void main(String args[]) { Rectangle rect = new Rectangle(); rect.display(); } }
You can also check our Java Annotations Example.
2.19 File Operations
File is an abstract data type in java. You can perform different file operations such as:
- creating a new File
- getting information about File
- writing into a File
- reading from a File
- deleting a File.
In Java, Stream and File are important classes. Stream consists of set of data in sequence. Stream is of two types which are ByteStream and CharacterStream. ByteStream is related to byte type. CharacterStream is used for Character data. Below is the code for different file operations.
File Operations
import java.io.File; import java.io.FileWriter; import java.io.FileNotFoundException; import java.io.IOException; import java.util.Scanner; class FileOperations { public static void main(String[] args) { try { File file = new File("input.txt"); Scanner dataReader = new Scanner(file); FileWriter fwrite = new FileWriter("output.txt"); while (dataReader.hasNextLine()) { String fileData = dataReader.nextLine(); System.out.println(fileData); fwrite.write(fileData+System.lineSeparator()); } dataReader.close(); fwrite.close(); System.out.println("output file is written"); File fileD = new File("checkDelete.txt"); if (fileD.delete()) { System.out.println(fileD.getName()+ " file is deleted "); } else { System.out.println("Unexpected exception"); } } catch (FileNotFoundException exception) { System.out.println(" exception occurred - file is not found"); exception.printStackTrace(); } catch (IOException exception) { System.out.println("unable to write to a file"); exception.printStackTrace(); } } }
2.20 Polymorphism
Polymorphism is related to having same method which can operate on different types. In Java, an interface is used to have define methods to handle different types. There are two types of polymorphism which are Method overloading and Method overriding
You can see the example below where Vehicle interface has the method getVelocity. Truck and Plane implements the Vehicle Interface and the method increases the velocity to the appropriate velocity related to the vehicle type. Below is the example code :
Plane and Truck Class
interface Machine { int distanceTravelled=100; public int getDistance(); } interface Vehicle { int velocity=50; public int getVelocity(); } class Plane implements Machine, Vehicle { int time; int velocity; int distanceTravelled; public Plane(int velocity, int time) { this.velocity = velocity; this.time = time; } public int getDistance() { distanceTravelled= velocity*time; System.out.println("Total Distance is : "+distanceTravelled); return distanceTravelled; } public int getVelocity() { int velocity=distanceTravelled/time; System.out.println("Velocity is : "+ velocity); return velocity; } } public class Truck implements Machine, Vehicle { int time; int velocity; int distanceTravelled; public Truck(int velocity, int time) { this.velocity = velocity; this.time = time; } public int getDistance() { distanceTravelled= velocity*time; System.out.println("Total Distance is : "+distanceTravelled); return distanceTravelled; } public int getVelocity() { int velocity=distanceTravelled/time; System.out.println("Velocity is : "+ velocity); return velocity; } public static void main(String args[]) { Truck truck = new Truck(50,2); truck.getDistance(); truck.getVelocity(); Plane plane = new Plane(1000,3); plane.getDistance(); plane.getVelocity(); } }
2.20 Typecasting
Type casting is converting a data type to another datatype. Widening and Narrowing are two types of type casting.
Widening is converting in this direction lower to higher. byte -> short -> char -> int -> long -> float -> double Widening is safe and there is no data loss. Narrowing is conversion in other direction higher to lower. double -> float -> long -> int -> char -> short -> byte . Below is the sample code shown for typecasting
TypeCasting Example
public class TypeCasting { public static void main(String[] args) { int x = 6; long y = x; float z = y; System.out.println("Before conversion, integer value "+x); System.out.println("After conversion, long value "+y); System.out.println("After conversion, float value "+z); double doub = 256.76; long lon = (long)doub; int intValue = (int)lon; System.out.println("Before conversion: "+doub); System.out.println("After conversion long type: "+lon); System.out.println("After conversion int type: "+intValue); } }
2.21 Abstract Class
Abstract classes are used for separation of instances from concepts. They do not have the implementation details. They help in separating behavior from the implementation. Below is the abstract class Example, Animal is the abstract class with behavior eat, Mobile is the interface, and Tiger is the instance.
Abstract Class
interface Mobile{ void move(); void jump(); void leap(); void eat(); } abstract class Animal implements Mobile{ public void eat(){System.out.println("living on other animals");} } class Tiger extends Animal{ public void move(){System.out.println("moving");} public void jump(){System.out.println("jumping");} public void leap(){System.out.println("leaping");} } class AbstractClassExample{ public static void main(String args[]){ Mobile animal =new Tiger(); animal.move(); animal.jump(); animal.leap(); animal.eat(); } }
2.22 Comments
Comments are used in java to make the program readable. There are three types of comments which are single line, multi line, and documentation. They help in code maintenance and explaining the code. Single line commenting is done two forward slashes //. Multi line commenting is done using /* and */ between the lines. Documentation commenting is done using /** and */ between the textual comments using javadoc tags. Comments example is shown below:
Comments Example
/** *demonstrating coments
* This program implements shows different types of comments ** Note: Comments help the developer to read the code * * @author Bhagvan Kommadi * @version 1.0 * @since 2021-17-10 */ public class CommentDemo { /* static public main method */ public static void main(String[] args) { int intValue=11; // integer with value 11 System.out.println(intValue); //printing the integer variable } }
3. Download the Source Code
In this article, we saw an easy syntax cheat sheet for Java programming.
You can download the full source code of this example here: Java Cheat Sheet
Last updated on Oct. 19th, 2021