Java Syntax Rules
In this tutorial for beginners, we will explain the basics of Java and its Syntax Rules.
Firstly, we will talk about the simple program syntax and we will analyze a simple program. After that, we will explicate the modifiers, enums, and comments. Last but not least we will talk about what keywords are allowed to use as classes, variables, or methods.
You can learn about the basics of Java syntax in the following Java Tutorial for Beginners video:
1. Java Syntax – Introduction
A Java program is a simple way to communicate with a computer in order for the computer to do a task for us. But how we create a program and what are the restrictions of the code that we must follow to have the right result without syntax errors?
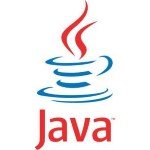
2. Technologies Used
The example code in this article was built and run using:
- Java 1.8.231(1.8.x will do fine)
- Eclipse IDE for Enterprise Java Developers-Photon
3. Simple program
At first, we must know the basics of the structure of a program. The four most important elements of a Java program are:
- Object: Objects are known to possess states and behaviors. For example, a dog has a name, color, and behavior.
- Class: Class is a template that is used to describe the states that are being supported by the objects.
- Instance variables: An instance variable is a variable that is a member of an instance of a class.
- Methods: The methods are created in classes and are used for the executions of the actions of the program.
Here we can see an example:
Hello_world.java
public class Hello_world { public static void main(String []args) { System.out.println("Hello World"); } }
You can also take a look at this Java Hello World Example for more practise.
4. Case Sensitivity
Java is a case-sensitive language. This means that if you declare two variables with the same name but the one has a capital character then the code understands that are two different variables. For example:
int value=1; int Value=1;//These variables are different
5. Java Conventions
Here are some naming conventions that we need to follow at java programming for good maintenance and readability of the code. In Java, we use the CamelCase to write the name of methods variables, classes, constants.
- Classes and Interfaces: The name of a class must be a noun and the first letter of each name should be capitalized. It’s recommended that the name should be a whole word. For example:
class Dog implements Animal
- Methods: The name of the method must be a verb with the first letter lowercase and the first letter of each internal word capitalized.For example:
void pauseTime(int time);
- Variables: The variable names should not start with a dollar(‘$’) or underscore(‘_’).One character variable name must be avoided and should be mnemonic. For example:
int number=8;
- Constant variables: The name of a constant variable must have all the letters capital and if it has more words at name should be separated by an underscore(‘_’). For example:
public static final float MAX_NUMBER= 100;
6. Brackets
In Java, we use brackets for many reasons. The first reason is to collect statements in blocks with curly brackets (‘{}’). We can see these brackets when we open or close classes, interfaces, methods, if and loop commands. The second reason is to index into an array with square brackets(‘[]’).For example:
Square brackets:
String[] animals= {"dog", "cat", "horse", "lion"};
Curly brackets:
public static int Number(int j) { int i = 0; i=i*j; return i; }
7. Semicolons
The semicolon(‘;’) is a character that we see almost at every line of code. The use of the semicolon is that it indicates an end of the statement. Some statements have a special characteristic which is that they are self-close. This commands are if(){}, for(){}, while(){} and functions(){}.
8. Arrays syntax in Java
An array is a reference type that can store a collection of values of a specific type. The declaration of an array is:
type[] identifier = new type[length];
- Type: The type can be primitive or reference.
- Identifier: The name of the array.
- Length: The number of items that we will insert.
For example:
int[] numbers=new int[30];
In Java, the array indexes start at zero. To insert an item in array we use the variable name and its index:
numbers[0] = 1; numbers[1] = 2;
9. Modifiers
Modifier: Is the access type of methods, classes, etc.. There are two categories of modifiers:
Access Modifiers:
- private: These methods can not be accessed by anywhere outside the class in which are declared.
- public: These methods are visible to any class in the program whether these classes are in the same or another package.
- protected: These methods can be accessed only by subclasses in other packages and the by all classes on the same package.
- default: This modifier is used when no access modifier is present and these methods can be accessed by classes in the same package.
Non-access Modifiers:
- final: A final variable can be explicitly initialized only once.
- abstract: An abstract class can never be instantiated.
- Static: The static keyword is used to create variables that will exist independently of any instances created for the class.
10. Java Enums
Enums in java are used to restrict a variable to have specific values.With enums its easier to reduce the number of bugs in your code. For example:
Enums.java
public class Enums { enum difficulty { easy, normal, hard } public static void main(String[] args) { difficulty dif = difficulty.hard; System.out.println(dif); } }
The output is:
hard
11. Comments syntax in Java
Comments can be used to explain Java code, and to make it more readable. It can also be used to prevent execution when testing an alternative code. there are 2 different ways to make a comment:
- One-line comments: It starts with two slashes (‘//’)
- Multi-line comments: Multi-line comments start with /* and ends with */.
Here is an example:
/*this * is * the * multi-line comment. */ System.out.println("Hi"); //this is one-line comment
You can take a look at this article related to Java Comments.
12. Keywords
There are some words that are reserved in Java and we cannot use them for variable names or identifiers names. These words are:
- abstract
- assert
- boolean
- break
- byte
- case
- catch
- char
- class
- const
- continue
- default
- do
- double
- else
- enum
- extends
- final
- finally
- float
- for
- goto
- if
- implements
- import
- instanceof
- int
- interface
- long
- native
- new
- package
- private
- protected
- public
- return
- short
- static
- strictfp
- super
- switch
- synchronized
- this
- throw
- throws
- transient
- try
- void
- volatile
- while
13. Download the Source Code
That was a tutorial for beginners. We explained the basics of Java and its Syntax Rules.
You can download the full source code of this example here: Java Syntax Rules