Java Primitive Types Tutorial
This is a tutorial about Java Primitive Data Types. Primitive types are the most basic data types available in any programming language. Being an object-oriented language Java supports both primitive types and objects as its data types. In this article, we are going to see all the supported Java primitive types.
Java is a strongly typed language and every variable should have a type definition. They can be either primitive or of any class type. Java supports 8 primitive types: boolean
, byte
, char
, short
, int
, long
, float
and double
. They form the basic blocks of data manipulation in Java.
Table Of Contents
1. Java Data Types
In this section, we have a brief introduction to the Java type system. Being a strongly typed language Java supports both primitive types and objects. Hierarchy of Java type systems can be viewed as below:
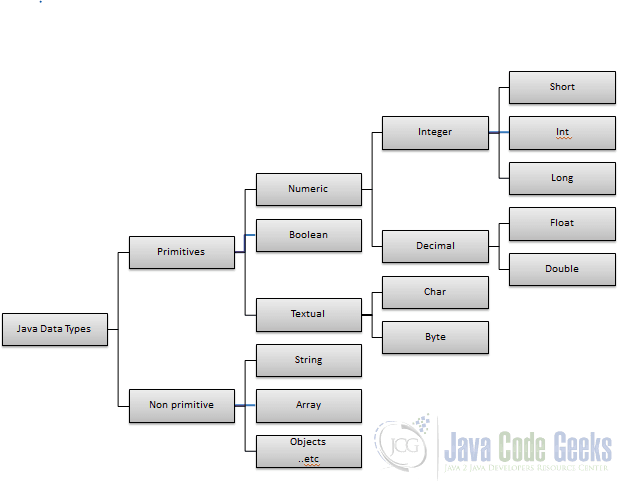
Data types specify the kind of values held by a variable. Java data types can be broadly classified as Primitive types and Non-primitive types.
Primitive types are the most basic types and they and support for them are inbuilt in the language, they are created by language creators.
Non-primitive types are complex data structures and are often created by the programmers (or users of the language).
The scope of this article is limited to primitive types.
2. Java Primitives
Java primitives are the basic building blocks of data manipulation. Java primitives are fixed in size. Thus, they are fixed to a range of values.
Java primitives can be classified as Numeric types, Textual types, and Boolean types.
2.1. Numeric types
Java numeric types are used to represent numbers. These include both whole numbers and decimal numbers. Java supports various type of systems like decimal, binary, octal, hexadecimal and so on. By default, all numbers are represented by base-10 (decimal).
2.2. Textual types
Java doesn’t have a string as a primitive type. String is represented as a sequence of characters. Even though the character is internally represented as of integer type, they are used to represent textual values.
2.3. Boolean types
Boolean values are single-bit values and they are used to represent boolean values true or false. Sometimes even null is classified into boolean value as null evaluates to false.
3. Java primitive types Examples
In the following section, I will provide an example for each of the primitive types.
3.1 Byte
Bytes
are the smallest integer type and they are 8 bit signed two’s complement integers. The maximum value of a byte
variable is 127 and the minimum value is -128. A byte
can be used in the place of integers where we are sure that value is not exceeding 127, this can save memory significantly. Byte variables can be declared as in the below snippet,
//Valid byte byte positiveByte = 120; byte negativeByte = -110; //Unassigned byte byte unAssignedByte;
When a byte
variable is set with a value higher than 127, data will overflow and the program won’t compile. Any unassigned byte
variable defaults to zero. When you try to compile the program with an invalid byte, then output is as below (This invalid output applies to every other primitive data type),
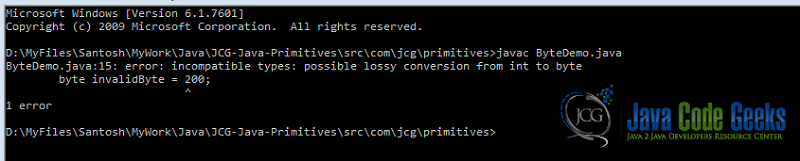
3.2 short
The short
type is a 16 digit 2’s complement integer. It can store values in the range of -32,768 to 32,767. Like byte
, it can also be used to save memory. Short variables can be declared and defined as in the below code snippet,
short positiveShort = 12000; short negativeShort = -11045; //Unassigned byte short unAssignedShort;
Any unassigned short
variable defaults to zero.
3.3 integers
Next primitive type we are seeing is int
or integer
. An integer is the 32 bit 2’s complement number and is used to store whole numbers in Java. Integers can hold values in the range of -2,147,483,648 to 2,147,483,647. Below code snippet shows how to declare integers,
int positiveInt = 123456; int negativeInt = -674532; //Unassigned integer int unAssignedInt;
With Java 8 it is possible to store unsigned integers up to 2^32- 1.
The default value of integer without an assignment is zero.
Integer supports almost all the arithmetic operations and any resulting decimal will be rounded to the whole number.
3.4 long
Long is another primitive type to represent whole numbers. He is like big brother of int. Long can store really large values. its values range from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807. A long variable occupies 64 bits in memory and it is memory intensive. need to be used with care. An example code snippet is as below,
long positiveLong = 12345623456L; long negativeLong = -6745321234L; //Unassigned long long unAssignedLong;
Long supports all the arithmetic operations like an integer and it defaults to zero when no value is assigned.
3.5 float
Float is the basic data type used to represent fractional numbers. Float data type is single-precision 32 bit IEEE 754 floating-point number. Float can be used for saving memory in place of doubles. Any floating-point number becomes less accurate once it passes 6 decimal points. Hence, it is not recommended to use float in high precision calculations like one’s involving currency.
A floating-point number can store values in the range of ±3.40282347E+38F.
All unassigned floating-point numbers have 0.0 as the default value. It is important to note that all floating-point numbers in Java have the suffix f. Otherwise, the decimal number is treated as double by default.
Below is the code snippet demonstrating declaration of a floating-point number,
float positiveFloat = 1.234F; float negativeFloat = -6.7F; //Unassigned float float unAssignedFloat;
3.6 double
Double data types stores double precision 64 bit IEEE 754 floating-point numbers. It is not recommended to use double data type numbers for high precision mathematical operations.
Any unassigned double number has a default value of 0.0
Value range – approximately ±1.79769313486231570E+308
All decimal values in Java are of the double data type. Double values can literally save all numbers.
Below is the code snippet showing how to declare double variables,
double positiveDouble = 1.234; double negativeDouble = -6.788989; //Unassigned double float unAssignedDouble;
3.7 char
Characters are single 16-bit Unicode characters. It has a minimum value of \u0000
(or 0) and a maximum value of \uffff
(or 65,535 inclusive). The character data type is used to store any character.
Character variables can be declared as shown in the below code snippet,
char myChar = 'A'; //unassigned char char unAssignedChar;
3.8 boolean
The boolean data type can have only two possible values true or false. This data type represents one bit of information, but its size isn’t defined precisely.
By default boolean ahs false value unless specified.
A boolean variable can be declared as in the below code snippet,
boolean boolValue = true; //Unassigned boolean boolean unAssignedBool;
4. Java primitives: Summary
Data type | Keyword | Syntax | Size in Bytes | Value range |
Boolean | boolean | boolean myVar = false; | 1 bit | true false |
Byte | byte | byte myVar = 123; | 1 byte | -128 to 127 |
Short | short | short myVar = 12345; | 2 bytes | -32768 to 32767 |
Integer | int | int myVar = 2345; | 4 bytes | -2147483648 to 2147483647 |
Long | long | long myVar = 12345678; | 8 bytes | -9223372036854775808 to 9223372036854775807 |
Float | float | float myVar = 12.23F; | 4 bytes | Approximately ±3.40282347E+38F |
Double | double | double myVar = 12345.6789; | 8 bytes | Approximately ±1.79769313486231570E +308 |
Character | char | char myVar = ‘A’; | 2 bytes | 0 to 65536 (unsigned) |
5. Data conversion (casting)
Typecasting is the operation of converting the unintended data type to the expected data type, such as float when you need an int. Casting allows us to convert from one type to another.
Though casting can be applied to both primitive and non-primitive types, in this article we are going to discuss only with respect to primitive types.
It is very common to cast numeric values from one type to another. It is important to note that boolean
cannot be used in casting operations.
It is straight forward to cast smaller types to larger types like byte
to an int
or an int
to a long
or float since the
destination can hold larger values than the source. When we cast smaller type to larger type precision isn’t lost.
A character can easily casted to an integer because each character has a numeric code representing its position in the character set. For example, character A is associated with integer value 65.
Whenever any smaller type is casted to a higher type, no need to specify the destination type. However, when a larger type has to be casted to smaller type then, the destination type needs to be specified explicitly as in (typename) value
.
typename is the data type to which the value is being converted to. Ex: int result = (int) (x/y);
Division operation results in a double
value and it is explicitly converted to an integer value in the above expression. In the casting operation, java operator precedence rules apply. If we don’t use parenthesis around the expression, the only x is converted to int type.
When a higher data type is typecast to lower data type, number precision is lost. In case of converting a floating-point number to an integer, decimal points are lost and only the whole number portion is retained in the result.
Below program demonstrates each of the use cases presented above,
package com.jcg.primitives; /** * @author Santosh Balgar Sachchidananda */ public class JavaTypeCastDemo { public static void main(String[] args) { System.out.println("******** Java primitives type casting *********"); //Automatic type conversion //Any smaller type can automatically converted to higher type. //This doesn't lead to any precision loosing as higher order primitive //can accommodate smaller ones. System.out.println("--------------- Automatic type conversion -----------------"); byte myByte = 100; //As short has lager range of values, it doesn't need any explicit type casting byte myShort = myByte; System.out.println("Byte value = " + myByte + " to Short value = " + myShort); int myInt = 20000; long myLong = myInt; System.out.println("Int value = " + myInt + " to Long value = " + myLong); //Characters are internally represented as integers. They can be converted //to integer type without explicit conversion char myChar = 'A'; int charToInt = myChar; System.out.println("Character value = " + myChar + " to Integer value = " + charToInt); //Explicit type conversion //When larger data types are casted to smaller ones we need explicit type //conversion. Normally these kind of conversion will oftern result in loss //of precision if not done carefully. System.out.println("--------------- Explicit type conversion -----------------"); long myLongVal = 1234567890123456L; //When long value is converted to int, if it is not in the range it looses precision //It needs explicit type casting as int is a smaller type int myIntVal = (int) myLongVal; System.out.println("Long value = " + myLongVal + " to Int value = " + myIntVal); double myDoubleVal = 234.343; myIntVal = (int) myDoubleVal; System.out.println("Double value = " + myDoubleVal + " to Int value = " + myIntVal); //Only valid integers can be converted to characters int myIntToConvert = 107; char myCharFromInt = (char) myIntToConvert; System.out.println("Integerer value = " + myIntToConvert + " to Character value = " + myCharFromInt); } }
The output from typecasting demo program:
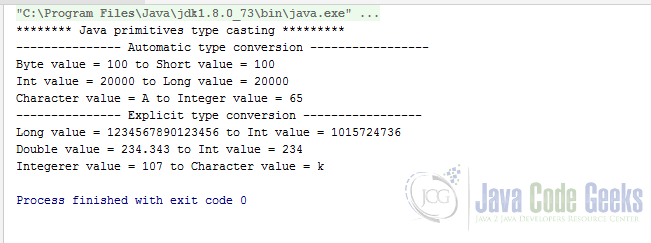
6. Download the Source Code
In this Java Primitive Data Types tutorial, I have created all the demo programs using the IntelliJ Idea editor.
You can download the full source code of this example here: Java Primitive Types Example
line 16 – the datatype should be “short”. I assume you had a little omission there for the conversion.
Hello Femi,
You are right. It is supposed to be short.
Thank you for bringing it up.
— Santosh B S
Hi,
Use
short myShort = myByte;
in line 16 of JavaTypeCastDemo java program.— Santosh B S