Java Data Types Example
In this article, we will learn about the Java data types. We will see examples with Java Primitive Data Types, like Number, Floating-point, Boolean, and Character, and examples with non-primitive Types, like String, Object, Interface, and Array.
Table Of Contents
1. Introduction
The Java programming language is statically-typed, which means that all variables must first be declared before they can be used. This involves stating the variable’s type and name.
int age = 10;
Doing so tells your program that a field named age exists, holds numerical data, and has an initial value of 10. A variable’s data type determines the values it may contain, plus the operations that may be performed on it.
2. Data Type
In this section, we will look into the different types of data types
available in Java. In Java, Data Types
are divided into two broad categories: Primitive data types
and Non-primitive data types
.
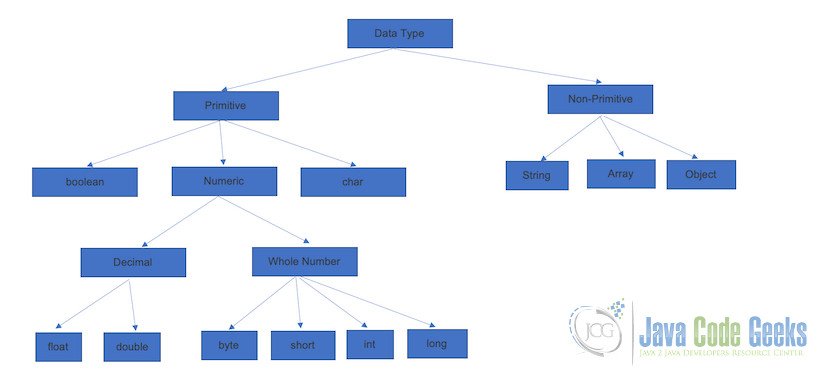
2.1 Primitive Data Type
A primitive type is predefined by the language and is named by a reserved keyword. It specifies the size and type of variable values, and it has no additional methods. Primitive values do not share state with other primitive values. There are eight primitive data types
in Java: byte
, short
, int
, long
, float
, double
, boolean
and char
Data Type | Size | Description | Default Value |
boolean | 1 bit | Has values as true or false | false |
byte | 1 byte | Stores whole numbers from -128 to 127 | 0 |
char | 2 bytes | Stores a single character/letter or ASCII values | ‘\u0000’ |
short | 2 bytes | Stores whole numbers from -32,768 to 32,767 | 0 |
int | 4 bytes | Stores whole numbers from -2,147,483,648 to 2,147,483,647 | 0 |
long | 8 bytes | Stores whole numbers from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 | oL |
float | 4 bytes | Stores fractional numbers. Sufficient for storing 6 to 7 decimal digits | 0.0f |
double | 8 bytes | Stores fractional numbers. Sufficient for storing 15 decimal digits | 0.0d |
2.1.1 Numbers
Primitive number types are divided into two groups:
Integer types stores whole numbers, positive or negative (such as 999 or -999), without decimals. Valid types are byte
, short
, int
and long
. Which type you should use, depends on the numeric value.
Floating-point types represent numbers with a fractional part, containing one or more decimals. There are two types: float
and double
.
Byte
The byte
data type is an 8-bit signed two’s complement integer. The byte
data type can store whole numbers from -128
to 127
. This can be used instead of int
or other integer types to save memory when you are certain that the value will be within -128
and 127
. The byte
data type is used to save memory in large arrays where the memory saving is most required. It saves space because a byte is 4 times smaller than an integer
byte myByte = 125; System.out.println(myByte);
Short
The short
data type is a 16-bit signed two’s complement integer. Its value-range lies between -32,768
to 32,767
(inclusive). Its default value is 0. The short
data type can also be used to save memory just like byte
data type. A short data type is 2 times smaller than an integer.
short myShort = -32765; System.out.println(myShort);
Int
The int
data type is a 32-bit signed two’s complement integer. Its value-range lies between - 2,147,483,648
(-2^31
) to 2,147,483,647
(2^31 -1
) (inclusive). Its default value is 0.The int
data type is generally used as a default data type for integral values unless if there is no problem about memory.
int myInt = 2147483647; System.out.println(myInt);
Long
The long
data type can store whole numbers from -9223372036854775808
to 9223372036854775807
. This is used when int
is not large enough to store the value. Note that you should end the value with an L
.
long myLong = -9223372036854775808L; System.out.println(myLong);
2.1.2 Floating-point
Use floating-point type data-type when the number is in decimal.
Float
The float
data type can store fractional numbers from 3.4e−038
to 3.4e+038
. Note that you should end the value with an f
:
float myFloat = 1234567.75f; System.out.println(myFloat);
Double
The double
data type can store fractional numbers from 1.7e−308
to 1.7e+308
. Note that you should end the value with a d
:
double myDouble = 123456789012.75234d; System.out.println(myDouble);
Running the above code will output 1.2345678901275233E11
. The precision of a floating point value indicates how many digits the value can have after the decimal point. The precision of float
is only six or seven decimal digits, while double
variables have a precision of about 15 digits. Therefore it is safer to use double for most calculations.
2.1.3 Boolean
A boolean data type is declared with the boolean keyword and can only take the values true or false. They are mostly used for conditional testing.
boolean myBoolean = true; System.out.println(myBoolean);
2.1.4 Character
The char
data type is used to store a single character. The character must be surrounded by single quotes, like ‘A’ or ‘a’.You can also use ASCII values to display certain characters:
char myChar = 'a'; System.out.println(myChar); char A = 65; System.out.println(A);
2.2 Non-Primitive Data Type
Non-primitive data types are called reference types because they refer to objects. The main difference between primitive and non-primitive data types are:
- Primitive types are predefined (already defined) in Java. Non-primitive types are created by the programmer and is not defined by Java (except for String).
- Non-primitive types can be used to call methods to perform certain operations, while primitive types cannot.
- A primitive type has always a value, while non-primitive types can be
null
. - A primitive type starts with a lowercase letter, while non-primitive types starts with an uppercase letter.
- The size of a primitive type depends on the data type, while non-primitive types have all the same size.
2.2.1 String
The String
data type is used to store a sequence of characters (text). String values must be surrounded by double quotes:
String str = "Java Code Geeks"; System.out.println(str);
A String
in Java is actually a non-primitive data type, because it refers to an object. The String
object has methods that are used to perform certain operations on strings. Strings are constant; their values cannot be changed after they are created.
The Java language provides special support for the string concatenation operator ( +
), and for conversion of other objects to strings. String
concatenation is implemented through the StringBuilder
(or StringBuffer
) class and its append
method. String conversions are implemented through the method toString
, defined by Object
and inherited by all classes in Java.
2.2.2 Object
Java objects are analogous to objects in the real world. In the real world, an object is an entity having both state and behaviour. It can be physical (tangible) , such as a house, car, etc., or logical (intangible), such as a Customer. In Java, all objects are intangible in the sense they only exist as a collection of data and programming in computer memory. A Java object is a representation of an entity and an implementation of its behaviours.
All Java objects have three characteristics:
- Identity is a unique ID used to identify the specific object from all other objects of the same kind. This ID is used internally by the Java system to reference and track an object and is not directly changeable by the programmer or user.
- State is determined by a group of data values associated with the object.
- Behavior is a series of methods that implement the object’s functionality.
Objects and classes are intertwined. A class is a description of a group of objects that have common properties and behaviour. It’s a template or blueprint from which specific objects are created. An object is a specific instance of class with its own state.
2.2.3 Interface
Interface is a way of achieving abstraction in Java. To access the interface methods, the interface must be implemented by another class with the implements
keyword.
Like abstract classes, interfaces cannot be used to create objects. On implementation of an interface, you must override all of its methods. Interface methods are by default abstract and public. Interface attributes are by default public, static and final. An interface cannot contain a constructor (as it cannot be used to create objects).
Java does not support “multiple inheritance” (a class can only inherit from one superclass). However, it can be achieved with interfaces, because the class can implement multiple interfaces.
2.2.4 Array
Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. To declare an array, define the variable type with square brackets:
int[] arrays = new int[10];
The above declared variable arrays
can hold 10 values of int
type.
int[] initializedArrays = new int[]{50, 2, 44};
The above code initializes the array while declaring it. Please note that we don’t explicitly provide the size in the second case as it is determined based on the number of values provided in the curly braces. You access an array element by referring to the index number:
System.out.println("Second element in the array: " + initializedArrays[1]);
The above code will print the second element of the array. To change the value of a specific element, refer to the index number:
int[] initializedArrays = new int[]{50, 2, 44}; System.out.println("Second element in the array: " + initializedArrays[1]); initializedArrays[1] = 100; System.out.println("New Second element in the array: " + initializedArrays[1]);
3. Java Data Types – Summary
In Java, Data Types
are divided into two broad categories Primitive types and Non-primitive data types
. In this article, we discussed the various data types
used in Java programming language. First, we looked into the primitive data types and discussed what their minimum and maximum values can be and what they are used for. Then we looked at some of the most commonly used non-primitive data types e.g. String, Object, etc.
4. Download the source code
You can download the full source code of this example here: Java Data Types Example