Convert int to string Java Example (with video)
Integer
to String
conversion is a basic task in many Java projects. There are many ways to convert int to string in java, where some of them are very simple.
In this example, we will show all the possible ways via an example on Java convert int to string.
You can also check this tutorial in the following video:
1. Syntax of Integer.toString() method
There are two different expressions for Integer.toString()
method:
The parameters of this method are:
i
: the integer to be converted.radix
: the used base – number system in order to represent the string.
The radix value is optional and if it is not set, the default value is 10, for the decimal base system.
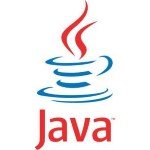
The returned value for both expressions is a String
that represents the i
argument. If radix
parameter is used, the returned string is specified by the respective radix.
2. Int to String with String.valueOf() method
String.valueOf()
is another static utility method. It is expressed:
where the parameter i
is the converted int.
This method returns the string that is represented of the int argument.
3. Int to String with String.format() method
Another use case for Integer
to String
conversion is format()
method. There are two different expressions:
public static String format(Locale l, String format, Object... args)
public static String format(String format, Object... args)
The arguments for this method are:
l
: theLocal
to be addressed during the formatting.format
: the format string, which includes at least oneformat specifier
and sometimes a fixed text.args
: arguments that refer to theformat specifiers
we set in theformat
parameter.
The returned value of this method is a formatted string, specified by the arguments. It is important to mention that this is a new method because it is introduced in JDK 1.5.
4. StringBuilder Class
A more complex way for converting int to string is the use of StringBuilder
class. StringBuilder
object represent String
object that can be modified and is treated as an array with a sequence of characters. To add a new argument to the end of the string, StringBuilder instance implements append() method. In the end it is important to call toString()
method, in order to take the string representation of the data in this sequence.
5. Int to string Java conversion
Create a java class with the name IntToStringTest.java
and paste the following code.
IntToStringTest.java:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 | package com.javacodegeeks.javabasics.inttostring; import java.util.IllegalFormatException; public class IntToStringTest { public static void main(String args[]) { int mainInt = 123456789 ; // add + operator String string1 = "" + mainInt; System.out.println( "With + operator: string1 = " + string1); // into the "" string String string2 = "123" ; System.out.println( "Directly in the String: string2 = " + string2); // use Integer.toString() with and without radix String string3 = Integer.toString(mainInt); String string4 = Integer.toString(mainInt, 16 ); System.out.println( "With toString method: string3(10 base system) = " + string3 + ", string4(16 base system) = " +string4); // String.valueOf() method String string5 = String.valueOf(mainInt); System.out.println( "With valueOf method: string5 = " + string5); try { // use format() method String string6 = String.format( "%d" , mainInt); System.out.println( "With format method: string6 = " + string6); } catch (IllegalFormatException e1) { System.err.println( "IllegalFormatException: " + e1.getMessage()); } catch (NullPointerException e2) { System.err.println( "NullPointerException: " + e2.getMessage()); } // StringBuilder instance StringBuilder sb = new StringBuilder(); sb.append(mainInt); String string7 = sb.toString(); System.out.println( "With StringBuilder class: string7 = " + string7); } } |
As you can see in the code above, the most easy and simple way of int to string conversion is the +
operator and/or the direct definition of the int into the string declaration. string1
and string2
show these situations. Notice that we defined %d
as format specifier into the String.format()
, in order to specify the integer. Also, this method throws IllegalFormatException
to catch illegal syntax of the format string and NullPointerException
if the format
argument is null
.
Below you can see the output of the execution.
Output:
With + operator: string1 = 123456789
Directly in the String: string2 = 123
With toString method: string3(10 base system) = 123456789, string4(16 base system) = 75bcd15
With valueOf method: string5 = 123456789
With format method: string6 = 123456789
With StringBuilder class: string7 = 123456789
Notice that for string3
there is a signed decimal representation of the string because we didn’t set a radix into the Integer.toString()
method. On the other hand, string4
represents the hex of the integer, because we defined radix
to 16.
6. More articles
7. Download the source code
This was an example of Java convert int to string.
Download the source code of this example: Convert int to string Java Example
Last updated on May 13th, 2021