Java Map Example
In this post, we feature a comprehensive Java Map Example. We will discuss about Maps
in Java.
A Map
is an interface that maps keys to values. The keys are unique and thus, no duplicate keys are allowed. A map can provide three views, which allow the contents of the map to be viewed as a set of keys, collection of values, or set of key-value mappings. In addition, the order of the map is defined as the order in which, the elements of a map are returned during iteration.
You can also check the Hashmap Java Example in the following video:
The Map interface is implemented by different Java classes, such as HashMap
, HashTable
, and TreeMap
. Each class provides different functionality and can be either synchronized or not. Also, some implementations prohibit null keys and values, and some have restrictions on the types of their keys.
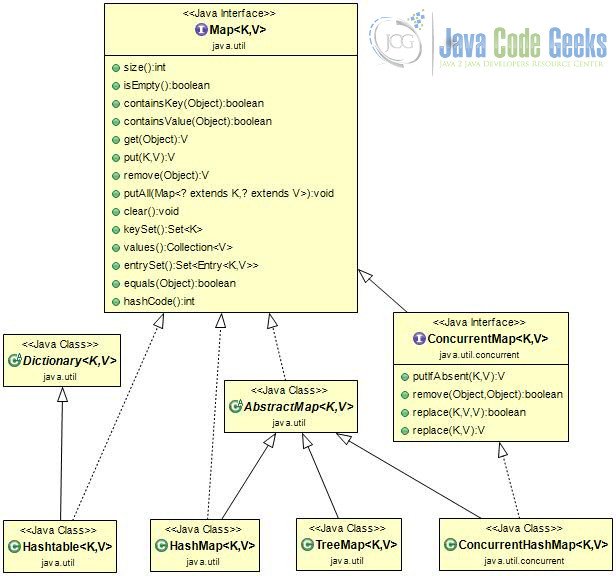
1. Basic Methods
A map has the form Map <K, V>
where:
- K: specifies the type of keys maintained in this map.
- V: defines the type of mapped values.
Furthermore, the Map
interface provides a set of methods that must be implemented. In this section, we will discuss the most famous methods:
- clear: Removes all the elements from the map.
- containsKey: Returns true if the map contains the requested key.
- containsValue: Returns true if the map contains the requested value.
- equals: Compares an Object with the map for equality.
- get: Retrieve the value of the requested key.
- entrySet: Returns a Set view of the mappings contained in this map.
- keySet: Returns a Set that contains all keys of the map.
- put: Adds the requested key-value pair in the map.
- remove: Removes the requested key and its value from the map, if the key exists.
- size: Returns the number of key-value pairs currently in the map.
2. Examples of Map
There are many classes that implement the Java Map
interface. In this chapter, we will present the most common and used.
2.1 HashMap
The most common class that implements the Map
interface is the Java HashMap
. A HashMap
is a hash table-based implementation of the Map interface. It permits null keys and values. Also, this class does not maintain any order among its elements and especially, it does not guarantee that the order will remain constant over time. Finally, a HashMap
contains two fundamental parameters: initial capacity and performance. The capacity is defined as the number of buckets in the hash table, while the load factor is a measure that indicates the maximum value the hash table can reach, before being automatically increased.
A simple example that uses a HashMap
is shown below:
HashMapExample.java:
package jcg.zheng.demo.data; import java.util.HashMap; import java.util.Map; public class HashMapExample { public static void main(String[] args) { Map<String, Integer> vehicles = new HashMap<>(); // Add some vehicles. vehicles.put("BMW", 5); vehicles.put("Mercedes", 3); vehicles.put("Audi", 4); vehicles.put("Ford", 10); System.out.println("Total vehicles: " + vehicles.size()); // Iterate over all vehicles, using the keySet method. for (String key : vehicles.keySet()) System.out.println(key + " - " + vehicles.get(key)); System.out.println(); String searchKey = "Audi"; if (vehicles.containsKey(searchKey)) System.out.println("Found total " + vehicles.get(searchKey) + " " + searchKey + " cars!\n"); // Clear all values. vehicles.clear(); // Equals to zero. System.out.println("After clear operation, size: " + vehicles.size()); } }
A sample execution is shown below:
Total vehicles: 4 Audi - 4 Ford - 10 BMW - 5 Mercedes - 3 Found total 4 Audi cars! After clear operation, size: 0
2.2 HashTable
The HashTable
class implements a hash table and maps keys to values. However, neither the key nor the value can be null. This class contains two fundamental parameters: initial capacity and performance, with the same definitions as the HashMap
class.
A simple example that uses a HashTable
is shown below:
HashTableExample.java:
package jcg.zheng.demo.data; import java.util.Hashtable; import java.util.Map; public class HashTableExample { public static void main(String[] args) { Map<String, Integer> vehicles = new Hashtable<>(); // Add some vehicles. vehicles.put("BMW", 5); vehicles.put("Mercedes", 3); vehicles.put("Audi", 4); vehicles.put("Ford", 10); System.out.println("Total vehicles: " + vehicles.size()); // Iterate over all vehicles, using the keySet method. for (String key : vehicles.keySet()) System.out.println(key + " - " + vehicles.get(key)); System.out.println(); String searchKey = "Audi"; if (vehicles.containsKey(searchKey)) System.out.println("Found total " + vehicles.get(searchKey) + " " + searchKey + " cars!\n"); // Clear all values. vehicles.clear(); // Equals to zero. System.out.println("After clear operation, size: " + vehicles.size()); // The next statements throw a NullPointerException, if uncommented. // vehicles.put("Nissan", null); // vehicles.put(null, 6); } }
A sample execution is shown below:
Total vehicles: 4 Audi - 4 Ford - 10 BMW - 5 Mercedes - 3 Found total 4 Audi cars! After clear operation, size: 0
2.3 TreeMap
The TreeMap
is a Red-Black tree implementation that is sorted according to the natural ordering of its keys, or by a Comparator
provided at the creation time. Also, this class maintains order on its elements. Finally, this class is not synchronized and thus, if an application uses multiple threads, the map must be synchronized externally.
A simple example that uses a TreeMap
is shown below:
TreeMapExample.java:
package jcg.zheng.demo.data; import java.util.Map; import java.util.TreeMap; public class TreeMapExample { public static void main(String[] args) { Map<String, Integer> vehicles = new TreeMap<>(); // Add some vehicles. vehicles.put("BMW", 5); vehicles.put("Mercedes", 3); vehicles.put("Audi", 4); vehicles.put("Ford", 10); System.out.println("Total vehicles: " + vehicles.size()); // Iterate over all vehicles, using the keySet method. for (String key : vehicles.keySet()) System.out.println(key + " - " + vehicles.get(key)); System.out.println(); System.out.println("Highest key: " + ((TreeMap) vehicles).lastKey()); System.out.println("Lowest key: " + ((TreeMap) vehicles).firstKey()); System.out.println("\nPrinting all values:"); for (Integer val : vehicles.values()) System.out.println(val); System.out.println(); // Clear all values. vehicles.clear(); // Equals to zero. System.out.println("After clear operation, size: " + vehicles.size()); } }
A sample execution is shown below:
Total vehicles: 4 Audi - 4 BMW - 5 Ford - 10 Mercedes - 3 Highest key: Mercedes Lowest key: Audi Printing all values: 4 5 10 3 After clear operation, size: 0
As you can observe, the elements of the map are printed in a strict lexicographic order, which does not appear in the previous examples of HashMap
and HashTable
.
2.4 ConcurrentHashMap
The class is a hash table that supports the full concurrency of retrievals. Thus, this structure is safe to use in case of multiple threads. Finally, this class allows neither keys nor values to be null.
A simple example that uses a ConcurrentHashMap
is shown below:
ConcurrentHashMapExample.java:
package jcg.zheng.demo.data; import java.util.Enumeration; import java.util.Map; import java.util.concurrent.ConcurrentHashMap; public class ConcurrentHashMapExample { public static void main(String[] args) { Map<String, Integer> vehicles = new ConcurrentHashMap<>(); // Add some vehicles. vehicles.put("BMW", 5); vehicles.put("Mercedes", 3); vehicles.put("Audi", 4); vehicles.put("Ford", 10); System.out.println("Total vehicles: " + vehicles.size()); // Iterate over all vehicles, using the keySet method. for (String key : vehicles.keySet()) System.out.println(key + " - " + vehicles.get(key)); System.out.println(); String searchKey = "Audi"; if (vehicles.containsKey(searchKey)) System.out.println("Found total " + vehicles.get(searchKey) + " " + searchKey + " cars!\n"); Enumeration<Integer> elems = ((ConcurrentHashMap) vehicles).elements(); while (elems.hasMoreElements()) System.out.println(elems.nextElement()); System.out.println(); Integer val = (Integer) vehicles.putIfAbsent("Audi", 9); if (val != null) System.out.println("Audi was found in the map and its value was updated!"); val = (Integer) vehicles.putIfAbsent("Nissan", 9); if (val == null) System.out.println("Nissan wasn't found in map, thus a new pair was created!"); System.out.println(); // The next statements throw a NullPointerException, if uncommented. // vehicles.put("Nissan", null); // vehicles.put(null, 6); // Clear all values. vehicles.clear(); // Equals to zero. System.out.println("After clear operation, size: " + vehicles.size()); } }
A sample execution is shown below:
Total vehicles: 4 BMW - 5 Mercedes - 3 Audi - 4 Ford - 10 Found total 4 Audi cars! 5 3 4 10 Audi was found in the map and its value was updated! Nissan wasn't found in map, thus a new pair was created! After clear operation, size: 0
3. More articles
- Hashmap Java Example
- Hashset Java Example
4. Download The Eclipse Project
This was a tutorial about Maps
in Java.
You can download the full source code of this example here: Java Map Example
Last updated on Feb. 24th, 2022
for(String key: I got: Error: java: incompatible types: java.lang.Object cannot be converted to java.lang.String
for(Object key: vehicles.keySet())