Java Calendar Example (with video)
In this tutorial, we will explain the Calendar class in Java – java.util.Calendar using an example.
1. Introduction
The Calendar
is an abstract class that provides methods for converting between time and calendar fields. Also, the class provides fields and methods for implementing a concrete calendar system.
The Calendar
field values can be set by calling the following methods: set, add and roll. In addition, the Calendar
has two modes for interpreting its fields, lenient and non-lenient. The lenient mode accepts a wider range of field values than it produces. The non-lenient mode is more strict and throws an exception in incorrect cases.
You can also check the Java Date and Calendar Tutorial in the following video:
2. Technologies used
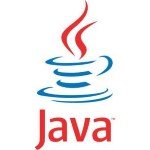
The example code in this article was built and run using:
- Java 1.8.231(1.8.x will do fine)
- Eclipse IDE for Enterprise Java Developers-photon
3. Calendar constructors
We can see two different ways that we can build constructor.
- protected Calendar(): The constructor creates a Calendar with the default time zone and locale.
- protected Calendar(TimeZone x, Locale y): The constructor creates a Calendar with the specified time zone and locale.
4. Java Calendar methods
Here you can see the methods of a calendar class:
abstract void add(int field, int amount)
: This method adds the amount of time to the given calendar field.boolean before(Object a):
This method returns whether this Calendar represents a time before the time represented by the specified Object.void clear()
: This method sets all the calendar field values and the time value of this Calendar undefined.void clear(int a):
This method sets the given calendar field value and the time value of this Calendar undefined.int compareTo(Calendar b)
: Compares the time values which are represented by two Calendar objects.protected void complete()
: This method fills in any unset fields in the calendar fields.protected abstract void ComputeTime()
: Converts the current calendar field values in fields[] to the millisecond time value time.boolean after(Object b)
: This method returns whether this Calendar represents a time after the time represented by the specified Object.boolean equals(Object b)
: Compares this Calendar to the specified Object.Int getActualMaximum(int f)
: Returns the maximum value that the specified calendar field could have given the time value of this Calendar.-
Int getActualMinimum(int f)
: Returns the minimum value that the specified calendar field could have given the time value of this Calendar. static Locale[] getAvailableLocales():
Returns an array of all locales for which the getInstance methods of this class can return localized instances.String getDisplayName(int f, int s, Locale l)
: Returns the string representation of the calendar field (f) value in the given style (s) and locale (l).String toString()
: Return the string representation of a calendar.-
void set(int f, int v)
: Sets the given calendar field (f) to the given value (v). void set(int y, int m, int d)
: sets the values for the calendar fields year(y), month(m), and day of the month (d). We can see the same method with more values such as the hours of a day , minute and second.Void setMinimalDaysInFirstWeek(int value)
: Sets what the minimal days required in the first week of the year are.void setTime(Date d)
: Sets the Calendar’s time with the given Date(d).void setTimeInMillis(long m)
: Sets the Calendar’s current time from the given long value.void setTimeZone(TimeZone v)
: Sets the time zone with the given time zone value.void setFirstDayOfWeek(int v)
: Sets what the first day of the week.void setLenient(boolean l)
: Specifies whether or not date/time interpretation is to be lenient.Map<String, Integer>getDisplayNames(int f, int s, Locale l)
: Returns a Map which contains all names of the calendar field in the given style and locale and their corresponding field values.int getFirstDayOfWeek()
: This method give as the first day of the week.abstract int getGreatestMinimum(int f)
: Returns the highest minimum value of a calendar field(f) of this Calendar instance.Static Calendar getInstance()
: Gets a calendar using the default time zone and locale. We can see this method with some parameters such as the time zone and locale.abstract int getLeastMaximum(int f)
: Returns the lowest maximum value for the given calendar field(f) of this Calendar instance.abstract int getMaximum(int f)
: Returns the maximum value for the given calendar field(f) of a Calendar instance.-
abstract int getMinimum(int f)
: Returns the minimum value for the given calendar field of a Calendar instance. int getMinimalDaysInFirstWeek(
): Gets what the minimal days required in the first week of the year are.Date getTime()
: Returns a Date object representing a Calendar’s time value.long getTimeInMillis()
: Returns a Calendar’s time value in milliseconds.TimeZone getTimeZone()
:Gets the time zone.int hashCode()
: Returns a hash code for the calendar.boolean isLenient()
: Tells whether date/time interpretation is to be lenient.boolean isSet(int field)
: Determines if the given calendar field has a value set, including cases that the value has been set by internal fields calculations triggered by a get method call.void roll(int f, int v)
: Sets the given calendar field(f) to the given value(v).
5. Create an Instance
The Calendar
provides a class method, called getInstance()
, which returns a general object of this type, whose calendar fields have been initialized with the current date and time. For example:
1 | Calendar calendar = Calendar.getInstance(); |
The Calendar
provides getter and setter methods for its fields:
public final int get (int field)
public final void set (int field, int value)
A subset of the Calendar
fields are shown below:
Calendar.YEAR
Calendar.MONTH
Calendar.DAY_OF_MONTH
Calendar.HOUR
Calendar.MINUTE
Calendar.SECOND
A sample example that uses an instance of the Calendar
class is shown below:
CalendarExample.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | import java.text.SimpleDateFormat; import java.util.Calendar; public class CalendarExample { public static void main(String[] args) { // Get an instance of a Calendar, using the current time. SimpleDateFormat dateFormat = new SimpleDateFormat( "yyyy-MM-dd HH:mm:ss" ); Calendar calendar = Calendar.getInstance(); System.out.println(dateFormat.format(calendar.getTime())); // Printing some information... System.out.println( "ERA: " + calendar.get(Calendar.ERA)); System.out.println( "YEAR: " + calendar.get(Calendar.YEAR)); System.out.println( "MONTH: " + calendar.get(Calendar.MONTH)); System.out.println( "WEEK_OF_YEAR: " + calendar.get(Calendar.WEEK_OF_YEAR)); System.out.println( "WEEK_OF_MONTH: " + calendar.get(Calendar.WEEK_OF_MONTH)); System.out.println( "DATE: " + calendar.get(Calendar.DATE)); System.out.println( "DAY_OF_MONTH: " + calendar.get(Calendar.DAY_OF_MONTH)); System.out.println( "DAY_OF_YEAR: " + calendar.get(Calendar.DAY_OF_YEAR)); System.out.println( "DAY_OF_WEEK: " + calendar.get(Calendar.DAY_OF_WEEK)); System.out.println( "DAY_OF_WEEK_IN_MONTH: " + calendar.get(Calendar.DAY_OF_WEEK_IN_MONTH)); System.out.println( "AM_PM: " + calendar.get(Calendar.AM_PM)); System.out.println( "HOUR: " + calendar.get(Calendar.HOUR)); System.out.println( "HOUR_OF_DAY: " + calendar.get(Calendar.HOUR_OF_DAY)); System.out.println( "MINUTE: " + calendar.get(Calendar.MINUTE)); System.out.println( "SECOND: " + calendar.get(Calendar.SECOND)); System.out.println( "MILLISECOND: " + calendar.get(Calendar.MILLISECOND)); } } |
A sample execution is shown below:
2014-02-06 17:33:40
ERA: 1
YEAR: 2014
MONTH: 1
WEEK_OF_YEAR: 6
WEEK_OF_MONTH: 2
DATE: 6
DAY_OF_MONTH: 6
DAY_OF_YEAR: 37
DAY_OF_WEEK: 5
DAY_OF_WEEK_IN_MONTH: 1
AM_PM: 1
HOUR: 5
HOUR_OF_DAY: 17
MINUTE: 33
SECOND: 40
MILLISECOND: 692
Notice that the output will be different in each execution, because of the Calendar
is initialized using the current time.
6. Java Calendar and Date
The Calendar
class can be used with the java.util.Date class, in order to create dates of a specific format. A sample example is given below:
CalendarDateExample.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 | import java.text.SimpleDateFormat; import java.util.Calendar; import java.util.GregorianCalendar; public class CalendarDateExample { public static void main(String[] args) { // Create an instance of a GregorianCalendar Calendar calendar = new GregorianCalendar( 2014 , 1 , 06 ); System.out.println( "Year: " + calendar.get(Calendar.YEAR)); System.out.println( "Month: " + (calendar.get(Calendar.MONTH) + 1 )); System.out.println( "Day: " + calendar.get(Calendar.DAY_OF_MONTH)); // Format the output. SimpleDateFormat date_format = new SimpleDateFormat( "yyyy-MM-dd" ); System.out.println(date_format.format(calendar.getTime())); } } |
A sample execution is shown below:
Year: 2014
Month: 2
Day: 6
2014-02-06
7. Gregorian Calendar
The only implementation of a Calendar that is provided by Java is the GregorianCalendar class. This class provides a standard calendar system that supports both the Julian and Gregorian calendar systems.
A sample example that uses a GregorianCalendar is shown below:
GregorianCalendarExample.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | import java.text.SimpleDateFormat; import java.util.Calendar; import java.util.GregorianCalendar; public class GregorianCalendarExample { public static void main(String[] args) { // Create an instance of a GregorianCalendar, using the current time. SimpleDateFormat dateFormat = new SimpleDateFormat( "yyyy-MM-dd HH:mm:ss" ); Calendar calendar = new GregorianCalendar(); System.out.println(dateFormat.format(calendar.getTime())); // Printing some information... System.out.println( "ERA: " + calendar.get(Calendar.ERA)); System.out.println( "YEAR: " + calendar.get(Calendar.YEAR)); System.out.println( "MONTH: " + calendar.get(Calendar.MONTH)); System.out.println( "WEEK_OF_YEAR: " + calendar.get(Calendar.WEEK_OF_YEAR)); System.out.println( "WEEK_OF_MONTH: " + calendar.get(Calendar.WEEK_OF_MONTH)); System.out.println( "DATE: " + calendar.get(Calendar.DATE)); System.out.println( "DAY_OF_MONTH: " + calendar.get(Calendar.DAY_OF_MONTH)); System.out.println( "DAY_OF_YEAR: " + calendar.get(Calendar.DAY_OF_YEAR)); System.out.println( "DAY_OF_WEEK: " + calendar.get(Calendar.DAY_OF_WEEK)); System.out.println( "DAY_OF_WEEK_IN_MONTH: " + calendar.get(Calendar.DAY_OF_WEEK_IN_MONTH)); System.out.println( "AM_PM: " + calendar.get(Calendar.AM_PM)); System.out.println( "HOUR: " + calendar.get(Calendar.HOUR)); System.out.println( "HOUR_OF_DAY: " + calendar.get(Calendar.HOUR_OF_DAY)); System.out.println( "MINUTE: " + calendar.get(Calendar.MINUTE)); System.out.println( "SECOND: " + calendar.get(Calendar.SECOND)); System.out.println( "MILLISECOND: " + calendar.get(Calendar.MILLISECOND)); } } |
A sample execution is shown below:
2014-02-06 17:24:50
ERA: 1
YEAR: 2014
MONTH: 1
WEEK_OF_YEAR: 6
WEEK_OF_MONTH: 2
DATE: 6
DAY_OF_MONTH: 6
DAY_OF_YEAR: 37
DAY_OF_WEEK: 5
DAY_OF_WEEK_IN_MONTH: 1
AM_PM: 1
HOUR: 5
HOUR_OF_DAY: 17
MINUTE: 24
SECOND: 50
MILLISECOND: 934
Notice that the output will be different in each execution because the GregorianCalendar
is initialized using the current time.
8. More articles
- Java LocalDate Example
- Java Tutorial for Beginners (with video)
- Java Constructor Example (with video)
- Printf Java Example (with video)
- Java Set Example (with video)
- Java random number generator Example
- Java API Tutorial
9. Download the Source Code
In this example, we explained the Calendar class in Java.
You can download the full source code of this example here: Java Calendar Example
Last updated on Jul. 23rd, 2021
can you please provide me the code for displaying whole calendar(12 months with dates ) of particular year.(only year entered by user like 2018)