Java Abstract Class Example
In this tutorial, we will discuss abstraction in java through examples. We are also going to talk about abstract class vs interface implementation in Java.
Data abstraction is the process of hiding certain details and showing only essential information to the user. We can have abstraction with using either abstract
classes or interfaces.
An abstract class is a class that is declared using the abstract keyword and it cannot be instantiated. It can be used only as a super-class for those classes that extend the abstract
class. The default functionality of the class still exists, with its fields, methods and constructors being accessed in the same way as with the other classes.
Moreover, an abstract
class may contain methods without any implementation, called abstract methods. The declaration of an abstract method starts with the abstract keyword and ends with a semicolon, instead of the method’s body. If a class contains an abstract
method, either declared or inherited, it must be declared as an abstract
class.
A class that extends an abstract
class must implement all its abstract
methods (if any). Otherwise, the sub-class must be declared as abstract
as well. Finally, any implementation of an abstract
method can be overridden by additional sub-classes.
You can also check this tutorial in the following video:
The last things to mention are that abstract
classes can also implement methods, despite providing just their signature and that an abstract
class may have static fields and static methods.
In this example, we use the following tools on a Windows 7 platform:
- Eclipse Kepler Service Release 1
- Java 1.7.0_51
1. Using a Java Abstract class
The purpose of an abstract
class is to specify the default functionality of an object and let its sub-classes to explicitly implement that functionality. Thus, it stands as an abstraction layer that must be extended and implemented by the corresponding sub-classes.
A sample example of using an abstract
class is the following. We declare an abstract class, called Instrument
:
Instrument.java
1 2 3 4 5 | abstract class Instrument { protected String name; abstract public void play(); } |
As we can observe, an Instrument
object contains a field name and a method called play, that must be implemented by a sub-class.
Next, we define a sub-class called StringedInstrument
that extends the Instrument
class and adds an extra field called numberOfStrings
:
StringedInstrument.java
1 2 3 | abstract class StringedInstrument extends Instrument { protected int numberOfStrings; } |
Finally, we add two more classes that implement the functionality of a StringedIntrument
, called ElectricGuitar
and ElectricBassGuitar
accordingly. The definition of these newly added classes is shown below:
ElectricGuitar.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 | public class ElectricGuitar extends StringedInstrument { public ElectricGuitar() { super (); this .name = "Guitar" ; this .numberOfStrings = 6 ; } public ElectricGuitar( int numberOfStrings) { super (); this .name = "Guitar" ; this .numberOfStrings = numberOfStrings; } @Override public void play() { System.out.println( "An electric " + numberOfStrings + "-string " + name + " is rocking!" ); } } |
ElectricBassGuitar.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 | public class ElectricBassGuitar extends StringedInstrument { public ElectricBassGuitar() { super (); this .name = "Bass Guitar" ; this .numberOfStrings = 4 ; } public ElectricBassGuitar( int numberOfStrings) { super (); this .name = "Bass Guitar" ; this .numberOfStrings = numberOfStrings; } @Override public void play() { System.out.println( "An electric " + numberOfStrings + "-string " + name + " is rocking!" ); } } |
Finally, we create a new class called Execution
that contains a single main method:
Execution.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 | import main.java.music.ElectricBassGuitar; import main.java.music.ElectricGuitar; public class Execution { public static void main(String[] args) { ElectricGuitar guitar = new ElectricGuitar(); ElectricBassGuitar bassGuitar = new ElectricBassGuitar(); guitar.play(); bassGuitar.play(); guitar = new ElectricGuitar( 7 ); bassGuitar = new ElectricBassGuitar( 5 ); guitar.play(); bassGuitar.play(); } } |
In this example, we create two different instances of an ElectricGuitar
and an ElectricBassGuitar
classes and we call their play methods. A sample execution of the aforementioned main method is shown below:
An electric 6-string Guitar is rocking!
An electric 4-string Bass Guitar is rocking!
An electric 7-string Guitar is rocking!
An electric 5-string Bass Guitar is rocking!
1.1 The Purpose of Abstract Classes
The purpose of abstract
classes is to function as base classes which can be extended by subclasses to create a full implementation.
Due to this feature, an Abstract Class is being used in scenarios where a complete implementation can be further broken down into a set of repetitive steps with few unique steps.
One such example will be, of URL Processor
application which extract the HTML of the website hosted at the URL.
URLProcessorBase.java
package URLProcessor;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.net.URLConnection;
public abstract class URLProcessorBase {
public void process(URL url) throws IOException {
URLConnection urlConnection = url.openConnection();
InputStream input = urlConnection.getInputStream();
try {
processURLData(input);
} finally {
input.close();
}
}
protected abstract void processURLData(InputStream input)
throws IOException;
}
This is a base class that contains the methods used for the implementation of the URL processor.
Now we can have the multiple subclasses of this base class based on the kind of processing that needs to be done on the website hosted at the URL provided as input.
In our example code, we are extracting the HTML passed as the response when we call the URL.
URLProcessorImpl.java
package URLProcessor;
import java.io.IOException;
import java.io.InputStream;
public class URLProcessorImpl extends URLProcessorBase {
@Override
protected void processURLData(InputStream input) throws IOException {
int data = input.read();
while (data != -1) {
System.out.print((char) data);
data = input.read();
}
}
}
Now the main driver code.
Driver.java
package URLProcessor;
import java.io.IOException;
import java.net.URL;
public class Driver {
public static void main(String[] args) throws IOException {
System.out.println("Driver Code for URL Processor Application using Abstract Class Method");
System.out.println("++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
System.out.println();
URLProcessorImpl urlProcessor = new URLProcessorImpl();
urlProcessor.process(new URL("https://abhiit89.github.io/"));
System.out.println();
System.out.println("++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
}
}
The output of the driver program is shown below.
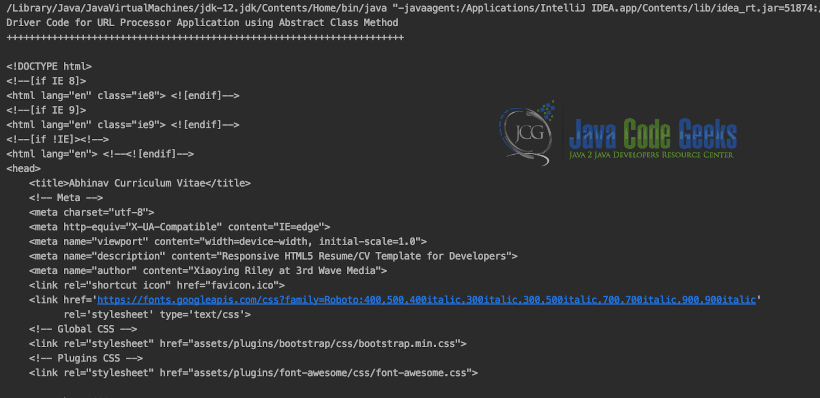
If we apply a new implementation of the URLProcessorBase
class, as shown below.
URLProcessorImplToExtractDataLength.java
package URLProcessor;
import java.io.IOException;
import java.io.InputStream;
public class URLProcessorImplToExtractDataLength extends URLProcessorBase {
@Override
protected void processURLData(InputStream input) throws IOException {
byte[] data = input.readAllBytes();
System.out.print(data.length);
}
}
URLProcessorImplToExtractDataLength.java
is another implementation of the base class URLProcessorBase.java
which calculates the length alone of the retrieved data. This implementation in a way enforces the SRP (Single Responsibility Principle).
We can override the original driver class to include this new implementation, but for the sake of clarity, a new driver class called the LengthDriver.java
is created.
LengthDriver.java
package URLProcessor;
import java.io.IOException;
import java.net.URL;
public class LengthDriver {
public static void main(String[] args) throws IOException {
System.out.println("Driver Code for URL Processor Application to Extract length of Retrieved Data using Abstract Class Method");
System.out.println("++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
System.out.println();
URLProcessorImplToExtractDataLength metaProcessor = new URLProcessorImplToExtractDataLength();
metaProcessor.process(new URL("https://abhiit89.github.io/"));
System.out.println();
System.out.println("++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
}
}
The output of LengthDriver.java is shown in the snapshot below.
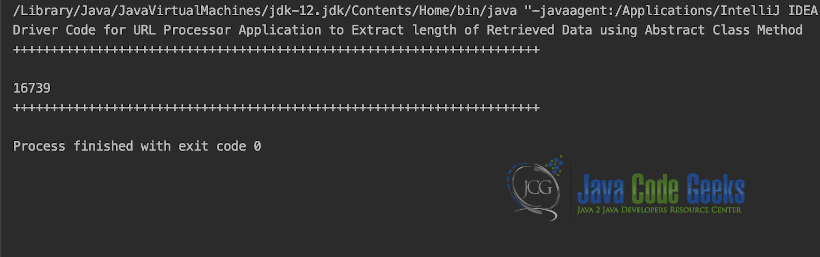
This covers the examples related to the Abstract Class.
2. Java Abstract Class vs Interface
In this section, we are going to talk about abstract class vs interface implementation. Java provides and supports the creation of abstract classes and interfaces.
As we stated at the beginning, we can achieve the abstraction by using either abstract
classes or interfaces. Both implementations share some common features, but they differ in the following features:
- All methods in an interface are implicitly abstract. On the other hand, an abstract class may contain both abstract and non-abstract methods.
- A class may implement a number of Interfaces but can extend only one abstract class.
- In order for a class to implement an interface, it must implement all its declared methods. However, a class may not implement all declared methods of an abstract class. Though, in this case, the subclass must also be declared as abstract.
- Abstract classes can implement interfaces without even providing the implementation of interface methods.
- Variables declared in a Java interface is by default final. An abstract class may contain non-final variables.
- Members of a Java interface are public by default. A member of an abstract class can either be private, protected or public.
- An interface is absolutely abstract and cannot be instantiated. An abstract class also cannot be instantiated but can be invoked if it contains the main method.
You can learn more about the abstract class vs interface implementation by reading this example.
3. Download the Eclipse Project
That was a tutorial about the Java Abstract
Class.
The Eclipse project of the Java Abstract Class Example: Java Abstract Class Example
Last updated on Mar. 18th, 2020
“In order for a class to implement an interface, it must implement all its declared methods. However, a class may not implement all declared methods of an abstract class. Though, in this case, the sub-class must also be declared as abstract.”
We still can declare method or methods default in interface and we dont need to implement those methods , would that be not the case ?
Looking at Java from historical perspective of view, we can find out that default methods in interfaces appeared since Java8.
So, sticking to the design of the language, it is more appropriate to use default methods in the inerfaces for refactoring the legacy code, keeping the compatibility with old client part.
When we compose the new code, it’s better to put a default part to the abstract class.