Encapsulation Java Example
In this tutorial we will discuss about Encapsulation in Java. Encapsulation is the mechanism for restricting access to an object’s components. It aims for high maintenance and handling of the application’s code. Also, the encapsulation mechanism protects the members of a class from external access, in order to be protected against unauthorized access.
You can also check this tutorial in the following video:
Encapsulation can be described as a protective barrier that prevents the code and data being randomly accessed by other code defined outside the class. Also, encapsulation provides the ability to modify our implemented code without breaking the code of others who use our code. Thus, using encapsulation we can achieve maintainability, flexibility and extensibility of our code.
1. Encapsulation Java Example
Java provides three keywords in order to define the scope and the access permissions of a class member or method: public
, private
and protected
.
- public member or method can be accessed from any other class.
- private member or method is accessible only within its own class.
- protected member is accessible within its class, its sub-classes and in all classes that reside in the same package.
2. Advantage of Encapsulation in Java
As we already mentioned, the encapsulation mechanism aims for controlling the access over the fields or methods of a class and for providing flexibility. Specifically, by using encapsulation while coding our applications, we:
- Combine the data of our application and its manipulation in one place.
- Allow the state of an object to be accessed and modified through behaviors.
- Hide the implementation details of an object.
- Achieve data hiding in Java because other class will not be able to access the data through the private data members.
- Reduce the coupling of modules and increase the cohesion inside them.
- Create immutable classes that can be used in multi-threaded environments.
- Can make the class read-only or write-only by providing only a setter or getter method
- Have control over the data. Suppose you want to set the value of id which should be greater than 0 only, you can write the logic inside the setter method. You can write the logic not to store the negative numbers in the setter methods.
- Can test the encapsulate class very easily. So, it is better for unit testing.
Finally, we must not confuse the encapsulation mechanism with the abstraction mechanism: the latter aims to specify what an object can do, while the former aims to specify how an object implements its functionality.
3. Examples of Encapsulation in Java
3.1 Simple encapsulate class
In encapsulationExample01 we haveh a Java class in which is a fully encapsulated class. It has one field with its setter and getter methods.
Employee.java
public class Employee { //private data member private String name; //getter method for name public String getName(){ return name; } //setter method for name public void setName(String name){ this.name=name; } }
Now here is a Java class to test the encapsulated class:
Test.java
public class Test { public static void main(String[] args) { //creating instance of the encapsulated class Employee e = new Employee(); //setting value in the name member e.setName("John"); //getting value of the name member System.out.println(e.getName()); } }
The output is: John
3.2 Read-Only encapsulate class
Here we have a Java class which has only getter methods.
Employee.java
public class Employee { //private data member private String department="Data Science"; //getter method for department public String getDepartment(){ return department; } }
Now, you can’t change the value of the department
data member which is “Data Science”.
e.setDepartment("Business Analysis");//will render compile time error
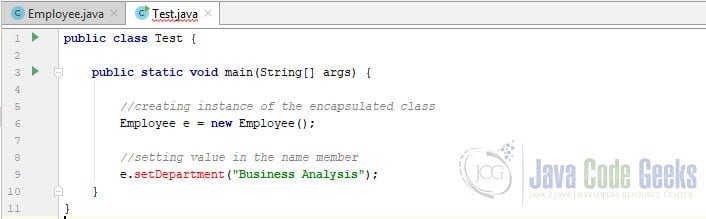
3.3 Write-Only encapsulate class
In encapsulationExample03 we have a Java class which has only setter methods.
Employee.java
public class Employee { //private data member private String department; //setter method for department public void setDepartment(String department) { this.department = department; } }
Now, you can’t get the value of the department
, you can only change the value of department
data member.
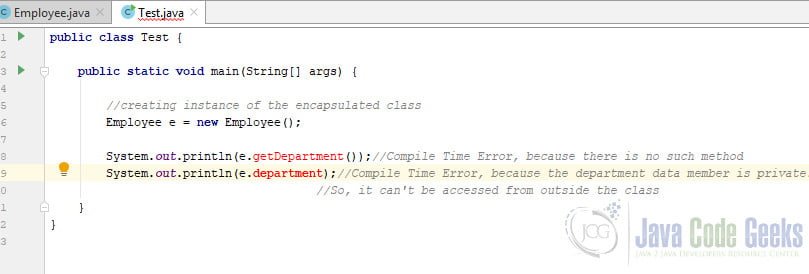
3.4 Another Example of Encapsulation in Java
Let’s see another sample example of encapsulation in Java, encapsulationExample04:
User.java:
public class User { private String username = null; private String password = null; private String firstname = null; private String lastname = null; private String email = null; public User(String username, String password, String firstname, String lastname, String email) { this.username = username; this.password = password; this.firstname = firstname; this.lastname = lastname; this.email = email; } // Define all setter and getter methods for each member field. public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public String getFirstname() { return firstname; } public void setFirstname(String firstname) { this.firstname = firstname; } public String getLastname() { return lastname; } public void setLastname(String lastname) { this.lastname = lastname; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } @Override public String toString() { return "<" + username + ", " + password + ", " + email + ", " + firstname + ", " + lastname + ">"; } }
We can create a fully encapsulated class in Java by making all the data members of the class private. Now we can use setter and getter methods to set and get the data in it. In this example, we created a User
class that contains the username, password, email, first and last names of a user of our application. As we observe, all members of the User
class are declared as private. For each member we provide a setter and a getter method, in order to change and retrieve the value of a member respectively.
The functionality of a User instance is implemented inside its class. A very important feature of encapsulation is the ability to change the implementation of a method, without changing the provided API. Also, if we want to alter or extend the functionality of a User, all changes will be applied in this class only. Thus, the extensibility and maintainability of our code increase.
A sample main method that creates and handles instances of the User class is shown below:
Test.java
public class Test { public static void main(String[] args) { User user1 = new User("StathisJCG", "JavaCodeGeeks", "myemail", "Stathis", "Maneas"); System.out.println("User: " + user1.toString() + "\n"); user1.setUsername("JCG"); System.out.println("My new username is: " + user1.getUsername() + "\n"); System.out.println("My password is: " + user1.getPassword()); System.out.println("I am about to change my password...\n"); user1.setPassword("JavaCodeGeeks!"); System.out.println("My new password is: " + user1.getPassword()); } }
A sample execution of the main method is shown below:
User: <StathisJCG, JavaCodeGeeks, Maneas, myemail, Stathis> My new username is: JCG My password is: JavaCodeGeeks I am about to change my password... My new password is: JavaCodeGeeks!
4. Design Patterns and Encapsulation
The encapsulation mechanism is used by very popular design patterns, in order to provide a specific functionality. For example, the Factory Pattern aims for creating objects, without exposing the implementation logic to the client. On the other hand, the Singleton Pattern is used to ensure that at most one instance of a class will be created. Both these patterns are based on the encapsulation, in order to implement their functionality.
5. Download the Source Code
You can download the full source code of this example here: Encapsulation Java Example
Last updated on Aug. 22, 2019
Very good material for junior developer to understand encapsulation.