Python Dictionary with Examples
1. Introduction
This is an article about dictionaries in Python. A dictionary is a collection of unique key-value pairs.
2. Dictionary – Python
2.1 Prerequisites
For windows or non-Windows operating systems requires an above version of 3.X. Pycharm is a popular IDE (Integrated Development Environment) that facilitates the development of python scripts with such features as Intellisense or auto-complete and allows for syntax checking and script execution. Other IDEs include Microsoft Visual Code and Eclipse.
2.2 Download
Python 3.6.8 can be downloaded from https://www.python.org/downloads/. Pycharm is available at https://www.jetbrains.com/pycharm/download.
2.3 Setup
2.3.1 Python Setup
In order to install Python you need to execute the package or the executable.
2.4 IDE
2.4.1 Pycharm Setup
Pycharm package or installable needs to be executed to install Pycharm.
2.5 Launching IDE
2.5.1 Pycharm
Launch the Pycharm and start creating a pure python project named HelloWorld. The screen shows the project creation.
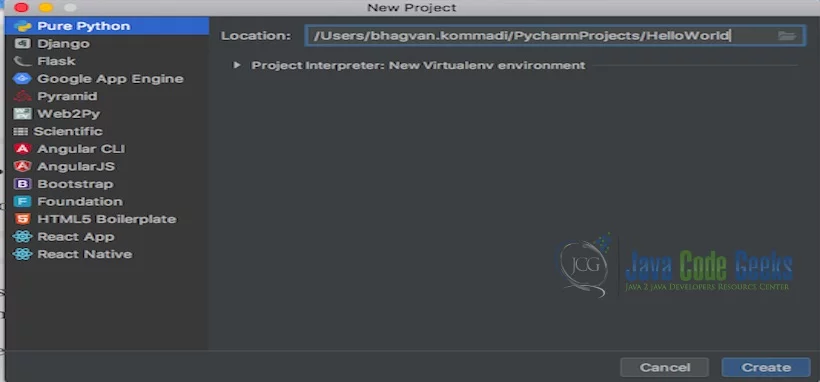
The project settings are set on the next screen as shown below.
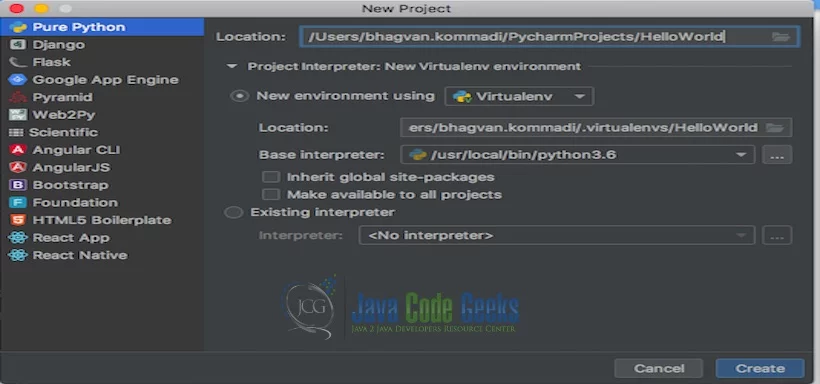
The welcome screen of the Pycharm application comes up with the progress as indicated below.
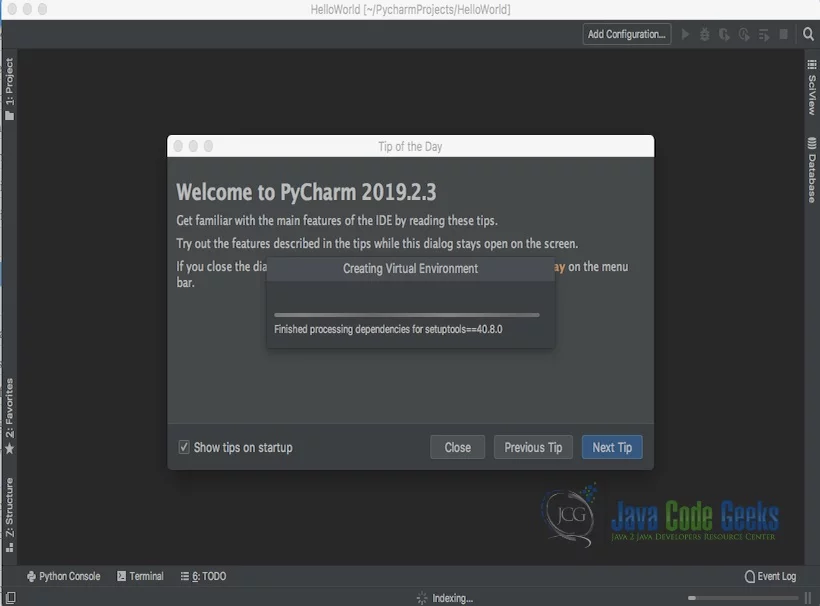
You can create a Hello.py and execute the python file by selecting the Run menu.
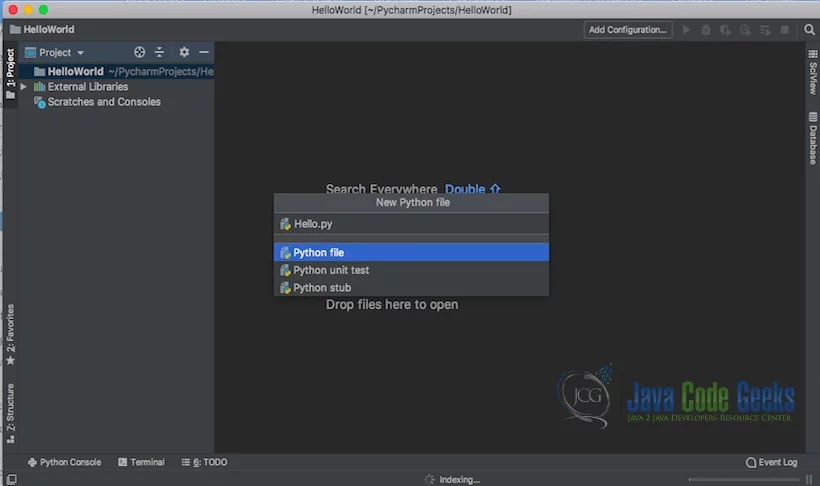
The next script prints “Hello World” when the Python script file runs.
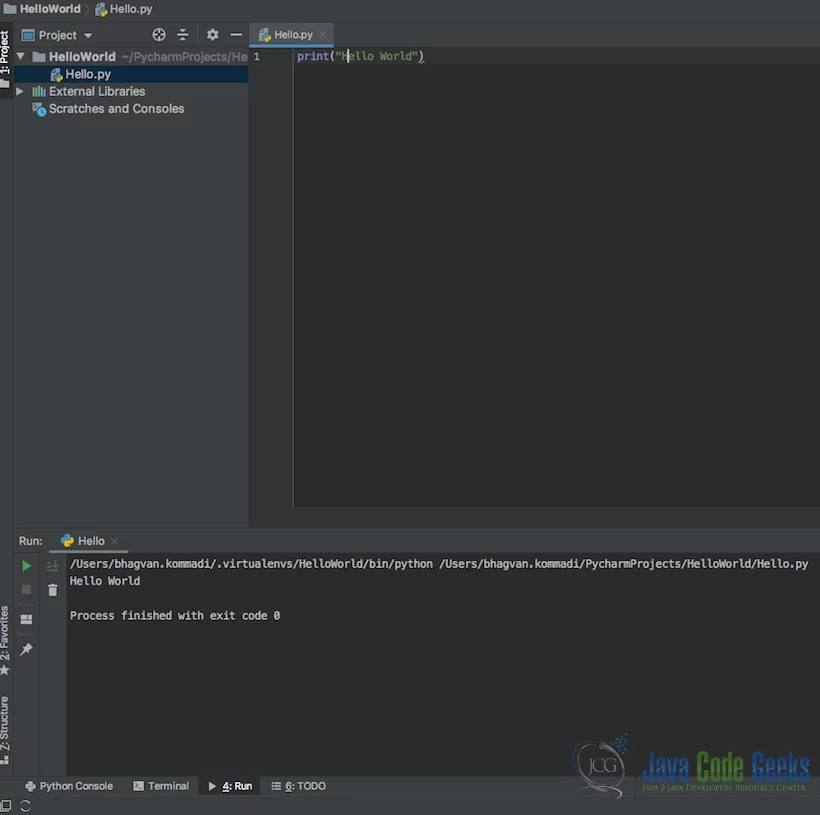
2.6 What is a dictionary?
You can use a dictionary for storing a set of data items. It has keys that are used to associate items with it.
- Given a key, the dictionary will retrieve the associated item.
- These keys can be of any data type: strings, integers, or objects.
- Where we need to sort a list, an element value can be found using its key.
- Add, remove, modify, and lookup operations are possible on this collection.
- A dictionary is similar to other data structures, such as hash function and hashmap.
- The key/value store is used in distributed caching and in-memory databases.
- Dictionary data structures are used in the following streams like Phone directories, Router tables in networking, Pagetables in operating systems, Symbol tables in compilers, and Genome maps in biology.
2.7 Defining a Dictionary
You can define the dictionary by using the dict() function or curly braces {}.
Defining Dictionary in Python
Example_Dict = {} print("Empty Example_Dictionary: ") print(Example_Dict)
You can execute the above code using the command below:
Execution Command
python3 Example_Dict.py
The output of the above code have this output:
Execution Output
apples-MacBook-Air:python_data_structures bhagvan.kommadi$ python3 Example_Dict.py Empty Example_Dictionary: {}
2.8 Dictionary comprehensive
The adjective form for the word comprehensive, stands from the meaning that, this will include everything that is needed or is relevant. As a result a comprehensive form means a compact style of writing some code for iterating/filtering/transforming a dictionary or a list.
Using comprehensive style it will be easy for the developers to read your code and will help you to keep it simple. The most important benefits of using a comprehensive style is to replace the for loops and lambda functions.
# defining a comprehensive syntax
new_dict = [expression for item in iterable <multiple if condition == True>]
# defining a dictionary
Example_Dict = {0: 'JavaCodeGeeks', 1: 'Like', 2: 'Dictionary', 3: 'comprehensive', 4: 'Follow', 5: 'next',
6: 'examples'}
print('Accessing all keys from dictionary:')
print(Example_Dict.keys())
print('Accessing all values from dictionary:')
print(Example_Dict.values())
print('Accessing all items from dictionary:')
dict1_items = {k: v for (k, v) in Example_Dict.items()}
print(dict1_items)
print('Change the value from dictionary through comprehensive style:')
dict1_items = {k: v*2 for (k, v) in Example_Dict.items()}
print(dict1_items)
Output for the above code:
Accessing all keys from dictionary:
dict_keys([0, 1, 2, 3, 4, 5, 6])
Accessing all values from dictionary:
dict_values(['JavaCodeGeeks', 'Like', 'Dictionary', 'comprehensive', 'Follow', 'next', 'examples'])
Accessing all items from dictionary:
{0: 'JavaCodeGeeks', 1: 'Like', 2: 'Dictionary', 3: 'comprehensive', 4: 'Follow', 5: 'next', 6: 'examples'}
Change the value from dictionary through comprehensive style:
{0: 'JavaCodeGeeksJavaCodeGeeks', 1: 'LikeLike', 2: 'DictionaryDictionary', 3: 'comprehensivecomprehensive', 4: 'FollowFollow', 5: 'nextnext', 6: 'examplesexamples'}
Process finished with exit code 0
Comprehensive syntax with if, if else statements:
# defining a comprehensive syntax
new_dict = [<value> if 'true' else <another value> for item in iterable <multiple if condition == True>]
# adding if condition in the comprehensive style
Example_Dict = {0: 'JavaCodeGeeks', 1: 'Like', 2: 'Dictionary', 3: 'comprehensive', 4: 'Follow', 5: 'next',
6: 'examples'}
dict1_items = {k: v for (k, v) in Example_Dict.items() if v.startswith('c')}
print('Print elements from dictionary where value starts with \'c\' letter')
print(dict1_items)
dict1_items = {k: v for (k, v) in Example_Dict.items() if v.startswith('J') if v.endswith('s')}
print('Print elements from dictionary where value starts with \'J\' and ends with \'s\' letter')
print(dict1_items)
dict1_items = {k: ('ends with a vowel' if v.endswith('e') else 'not ends with a vowel')
for (k, v) in Example_Dict.items()}
print('Print elements from dictionary where value ends with vowel \'e\'')
print(dict1_items)
Output for the above code:
Print elements from dictionary where value starts with 'c' letter
{3: 'comprehensive'}
Print elements from dictionary where value starts with 'J' and ends with 's' letter
{0: 'JavaCodeGeeks'}
Print elements from dictionary where value ends with vowel 'e'
{0: 'not ends with a vowel', 1: 'ends with a vowel', 2: 'not ends with a vowel', 3: 'ends with a vowel', 4: 'not ends with a vowel', 5: 'not ends with a vowel', 6: 'not ends with a vowel'}
Process finished with exit code 0
2.9 Accessing Dictionary Values
You can access the dictionary values by key name. You can use [] square brackets to access the value of any key as shown below:
Accessing Dictionary Values in Python
Example_Dict = {} print("Empty Example_Dictionary: ") print(Example_Dict) Example_Dict[0] = 'JavaCodeGeeks' Example_Dict[2] = 'Like' Example_Dict[3] = 2 print("\nExample_Dictionary will be like below after adding 3 elements: ") print(Example_Dict) print(Example_Dict[3])
You can execute the above code using the command below:
Execution Command
python3 Example_Dict.py
The output of the above code have this output:
Execution Output
apples-MacBook-Air:python_data_structures bhagvan.kommadi$ python3 Example_Dict.py Empty Example_Dictionary: {} Example_Dictionary will be like below after adding 3 elements: {0: 'JavaCodeGeeks', 2: 'Like', 3: 2} 2
2.10 Dictionary Keys vs. List Indices
Dictionary values can be accessed by keys. Key-value pairs are saved in the dictionary. A list is a collection of ordered elements that are used to persist a list of items. Unlike array lists, these can expand and compress dynamically. You can use Lists as a base for other data structures, such as stack and queue. You can also use them to store lists of users, car parts, ingredients, to-do items, and various other such elements. Lists are the commonly data structures that developers are using. They were first introduced in the lisp programming language.
Defining Dictionary in Python
Example_Dict = {} print("Empty Example_Dictionary: ") print(Example_Dict) Example_Dict[0] = 'JavaCodeGeeks' Example_Dict[2] = 'Like' Example_Dict[3] = 2 print("\nExample_Dictionary will be like below after adding 3 elements: ") print(Example_Dict) Example_Dict['Value_set'] = 4, 5, 7 print("\nExample_Dictionary will be like below after adding 3 elements: ") print(Example_Dict) Example_Dict[2] = 'Hello' print("\nUpdated key value for second element: ") print(Example_Dict[2]) # List Definition Example_List = [] print("Initial blank Example_List: ") print(Example_List) Example_List.append(1) Example_List.append(2) Example_List.append(7) print("\nExample_List after Addition of Three elements: ") print(Example_List) print(Example_List[1])
You can execute the above code using the command below:
Execution Command
python3 Example_Dict_List.py
The output of the above code is:
Dictionary vs List
apples-MacBook-Air:python_data_structures bhagvan.kommadi$ python3 Example_Dict.py Empty Example_Dictionary: {} Example_Dictionary will be like below after adding 3 elements: {0: 'JavaCodeGeeks', 2: 'Like', 3: 2} Example_Dictionary will be like below after adding 3 elements: {0: 'JavaCodeGeeks', 2: 'Like', 3: 2, 'Value_set': (4, 5, 7)} Updated key value for second element: {0: 'JavaCodeGeeks', 2: 'Hello', 3: 2, 'Value_set': (4, 5, 7)} Hello Initial blank Example_List: [] Example_List after Addition of Three elements: [1, 2, 7] 2
2.11 Example: Use of Continue Statement in processing dictionary
The following is an example of using a continue Statement in the processing of a dictionary, in this case, ages. The continue statement will allow the key (along with its value) of the dictionary to bypass processing the remainder of the for loop if it is not in a desired key list ( lines 40-42 ).
from datetime import datetime data = [{"sport": "hockey", "Player Name": "Joseph Offsider", "birthdate": "10/10/1996"}, {"sport": "soccer", "Player Name": "Derek CornerKick", "birthdate": "10/13/1995"}, {"sport": "hockey", "Player Name": "William Puck", "birthdate": "2/19/1986"}, {"sport": "hockey", "Player Name": "Max Goalie", "birthdate": "8/9/1992"}, {"sport": "baseball", "Player Name": "Simon Hoops", "birthdate": "4/19/1996"}, {"sport": "hockey", "Player Name": "Larry Icing", "birthdate": "1/25/1980"}, {"sport": "basketball", "Player Name": "Carl Foul", "birthdate": "3/12/1978"}, {"sport": "golf", "Player Name": "Jack ParMeister", "birthdate": "12/30/1978"}, {"sport": "tennis", "Player Name": "Sarah SetMatch", "birthdate": "8/25/1977"}, {"sport": "football", "Player Name": "David Touchdown", "birthdate": "5/29/1977"}, {"sport": "hockey", "Player Name": "Wayne Red Wings", "birthdate": "1/24/1982"}, {"sport": "soccer", "Player Name": "Miles Midfielder", "birthdate": "1/17/1985"}, {"sport": "basketball", "Player Name": "Aaron Dunker", "birthdate": "5/20/1986"}, {"sport": "football", "Player Name": "Peter Sackmeister", "birthdate": "7/24/1998"}, {"sport": "golf", "Player Name": "Frank Bogie", "birthdate": "9/22/1992"}, {"sport": "hockey", "Player Name": "Franz FaceoffArtist", "birthdate": "9/3/1987"}, {"sport": "baseball", "Player Name": "Benny TriplePlayer", "birthdate": "12/1/1987"}, {"sport": "hockey", "Player Name": "Colin Powerplay", "birthdate": "12/12/1995"}, {"sport": "tennis", "Player Name": "Sylvia Doubles", "birthdate": "10/2/1982"}, {"sport": "basketball", "Player Name": "Steve AssistLeader", "birthdate": " 7/27/1997"}, {"sport": "golf", "Player Name": "Calem Eagle", "birthdate": " 1/10/1992"}, ] today = datetime.now() ages = {"hockey": [0, 0], "baseball": [0, 0], "basketball": [0, 0], "football": [0, 0], "golf": [0, 0], "soccer": [0, 0]} for item in data: if item['sport'] in ages: parts = item['birthdate'].split('/') yearDiff = today.year - int(parts[2]) if int(parts[0]) > today.month: yearDiff -= 1 monthDiff = today.month + 12 - int(parts[0]) else: monthDiff = int(parts[0]) - today.month ages[item['sport']][0] += yearDiff * 12 + monthDiff ages[item['sport']][1] += 1 for key in ages: if key not in ['baseball', 'basketball', 'football', 'hockey']: continue averageAgeInMonths = round(ages[key][0] / ages[key][1]) print(f'Average age for {ages[key][1]} {key} players is {averageAgeInMonths // 12} years {averageAgeInMonths % 12} months')
2.12 Restrictions on Dictionary Keys
Dictionary keys cannot be polymorphic. You will not allow to have duplicates key. They are immutable. Its keys are case-sensitive. Key with different cases but same spelling are different.
2.13 Operators and Built-in Functions
Python has the following operators for Dictionary:
- dict[key]: This operator is used to get the value based on the given key from the dictionary.
- dict[key] = pairvalue: You can use this operator to set the value based on the given key from the dictionary.
- del dict[key]: This operator is used to delete the key and its paired value from the dictionary.
The table below shows the built-in functions for Dictionary:
Function | Usage |
---|---|
clear() | This function removes all items from the dictionary. |
copy() | This function returns a shallow copy of the dictionary. |
fromkeys(seq[, v]) | This function returns a new dictionary with keys from seq and value equal to v (defaults to None ). |
get(key[,d]) | This function returns the value of the key. If the key does not exist, returns d (defaults to None ). |
items() | This function returns a new object of the dictionary’s items in (key, value) format. |
keys() | This function returns a new object of the dictionary’s keys. |
pop(key[,d]) | This function removes the item with the key and returns its value or d if the key is not found. If d is not provided and the key is not found, it raises KeyError . |
popitem() | This function removes and returns a key-value pair. It raises KeyError if the dictionary is empty. |
setdefault(key[,d]) | This function returns the corresponding value if the key is in the dictionary. If not, it inserts the key with a value of d and returns d (defaults to None ). |
update([other]) | This function updates the dictionary with the key-value pairs from other, overwriting existing keys. |
values() | This function returns a new object of the dictionary’s values |
2.14 Order vs Unorder
In Python we have two types of data structure in which we can store the information.
These types are: order and unorder. This means that the information that is in the lists are in the order we insert.
Order data structures in Python are:
- Tuples
- String
- List
Unorder data structures in Python are:
- Set
- Dictionary (with a note that for Python version above 3.6, keeps the inserted order)
Examples of data structure with order vs unorder:
import sys
letters = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'
string_result = str(letters)
list_result = list(letters)
tuples_result = tuple(letters)
sets_result = set(letters)
dict_result = {value: value for value in list_result}
print('Print python version')
print(sys.version)
print('Printing the result for each data structure')
print('Print the string letters')
print(string_result)
print('Print list from the string letters')
print(list_result)
print('Print tuples from string letters')
print(tuples_result)
print('Print sets from string letters')
print(sets_result)
print('Print dictionary from string letters')
print(dict_result)
Output for the above code:
Print python version
3.9.1 (tags/v3.9.1:1e5d33e, Dec 7 2020, 17:08:21) [MSC v.1927 64 bit (AMD64)]
Printing the result for each data structure
Print the string letters
abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ
Print list from the string letters
['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z']
Print tuples from string letters
('a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z')
Print sets from string letters
{'l', 'u', 'A', 'E', 'c', 'j', 'k', 'q', 'v', 'w', 'K', 'F', 'U', 'W', 'C', 'R', 'p', 'o', 'e', 'r', 't', 'B', 'L', 'N', 'm', 'd', 'J', 'a', 'H', 'O', 'g', 'i', 'n', 'P', 'V', 's', 'x', 'D', 'y', 'M', 'f', 'b', 'I', 'S', 'Y', 'T', 'G', 'X', 'h', 'Z', 'Q', 'z'}
Print dictionary from string letters
{'a': 'a', 'b': 'b', 'c': 'c', 'd': 'd', 'e': 'e', 'f': 'f', 'g': 'g', 'h': 'h', 'i': 'i', 'j': 'j', 'k': 'k', 'l': 'l', 'm': 'm', 'n': 'n', 'o': 'o', 'p': 'p', 'q': 'q', 'r': 'r', 's': 's', 't': 't', 'u': 'u', 'v': 'v', 'w': 'w', 'x': 'x', 'y': 'y', 'z': 'z', 'A': 'A', 'B': 'B', 'C': 'C', 'D': 'D', 'E': 'E', 'F': 'F', 'G': 'G', 'H': 'H', 'I': 'I', 'J': 'J', 'K': 'K', 'L': 'L', 'M': 'M', 'N': 'N', 'O': 'O', 'P': 'P', 'Q': 'Q', 'R': 'R', 'S': 'S', 'T': 'T', 'U': 'U', 'V': 'V', 'W': 'W', 'X': 'X', 'Y': 'Y', 'Z': 'Z'}
Process finished with exit code 0
As we can see in the above output, some of the data structures keep the initial order of insertion. The only difference we can see is the dictionary data structure. This is an ordered structure based on the insertion order for the running Python version.
3. More articles
4. Download the Source Code
You can download the full source code for this article here: Python Dictionary with Examples
Last updated on April 11th, 2022