Python Development with Visual Studio
1. Overview
In this article, we look at python development with visual studio.
The integrated development environment has an editor, compiler, interpreter, and debugger. They can be used from a user interface for developing applications for the web and mobile. Visual studio has a python extension that needs to be installed to look at the code execution steps during debugging and check the values of the variables on the path of execution. Visual Studio has support for python 3.4 and higher. It can support python 2.7. The other features are code completion, linting, debugging support, code snippets, unit testing support, automatic use of condo and virtual environments, and code editing in Jupyter notebooks.
2. Visual Studio – Python Development
2.1 Prerequisites
Visual study code is necessary on the operating system in which you want to execute the code.
2.2 Download
You can download Visual studio code from the website.
2.3 Setup
2.3.1 Visual Studio Code Setup
The visual studio code.dmg can be downloaded as the macOS Disk Image file. You can mount the disk image file as a disk in the Mac. Ensure that you have copied the Visual Studio Code to the Applications folder
2.4 Launching IDE
2.4.1. Visual Studio
Visual studio code can be launched by clicking on the application icon. The visual studio starts up as shown below:
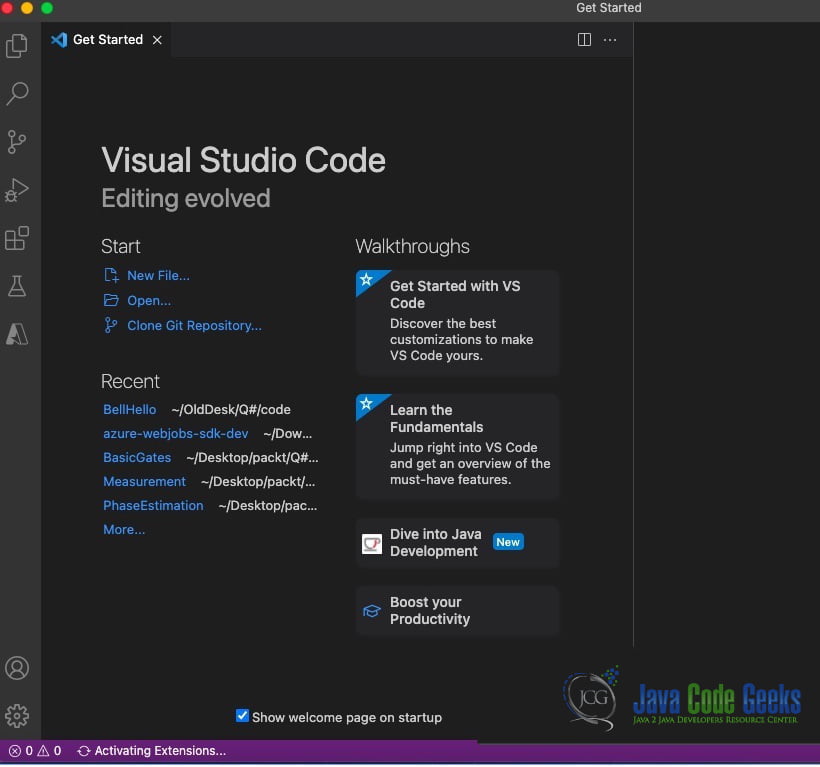
You can select the language in which you can develop. The screen looks as below:
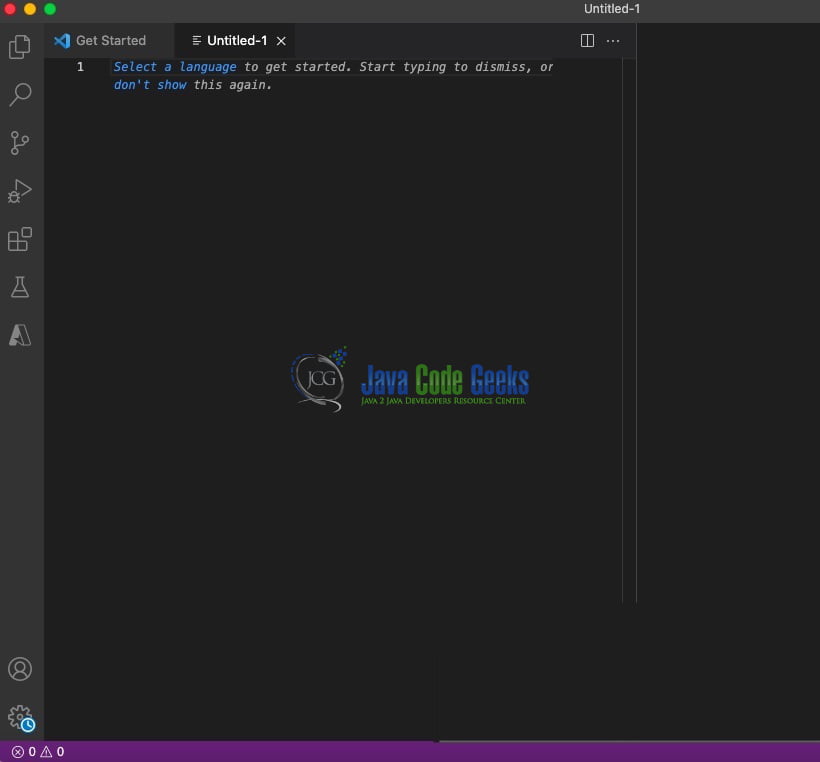
2.5 Configuring Visual Studio Code for Python Development
You can select the language as python and install the python extension. Before installing, user settings, workspace settings need to be set. Workspace settings can be saved as .json files in a folder with extension .vscode.
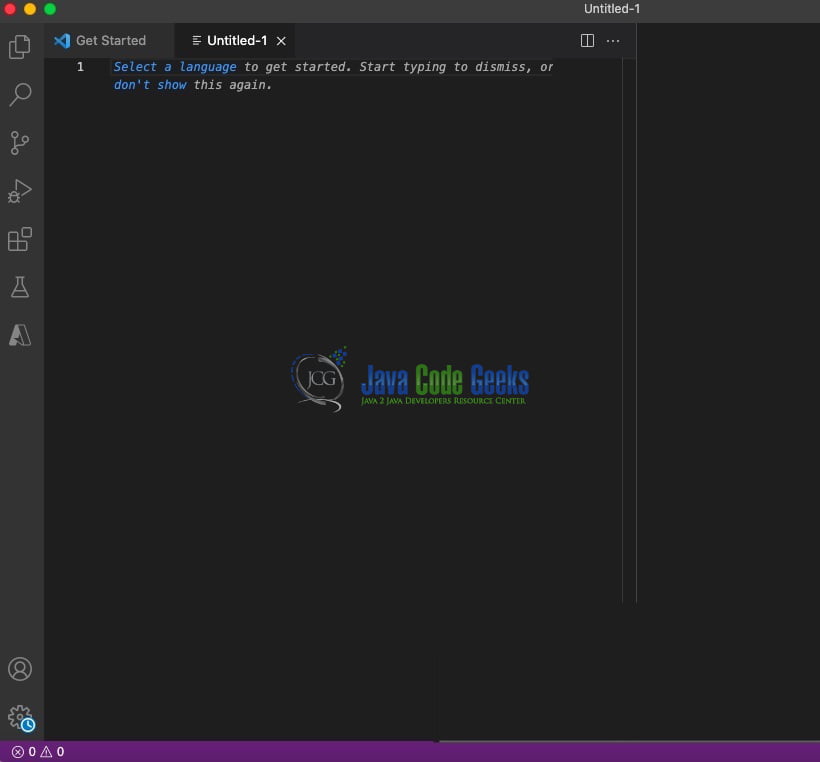
You can click on the install button. The below screen comes up as shown below:
Click on the install button. Installing in progress comes as shown in the image below:
After the installation is complete, you can set the python version.
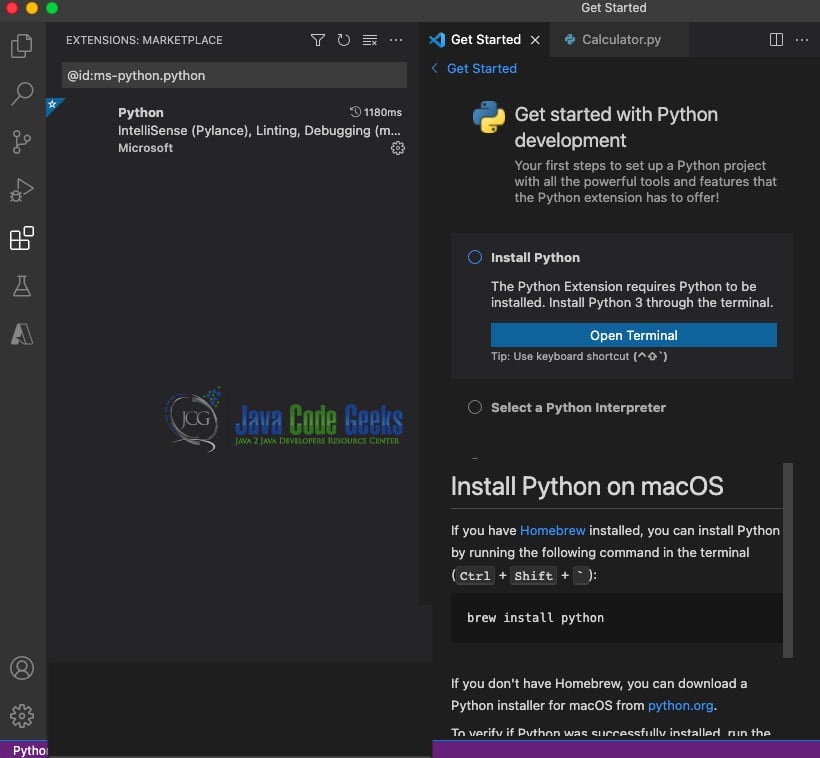
2.6 Start a New Python program
You can start creating a new python program using the code below:
New Python Program
class Calculator: def __init__(self): self.data = [] def sum(self,int1,int2): self.data.append(int1); self.data.append(int2); return int1+int2; def random(intarr1): from random import randint rand = randint(0,len(intaar1)-1) output = intarr1[rand] return output print("executing main") calculator = Calculator() sum = calculator.sum(3,4) print(str(sum))
You can use the code above to create a python file Calculator.py using the editor.
2.7 Running Python program
After saving the file, you can execute the code using Run Python File in Terminal by right-clicking on the editor window. Code gets executed as shown in the image below:
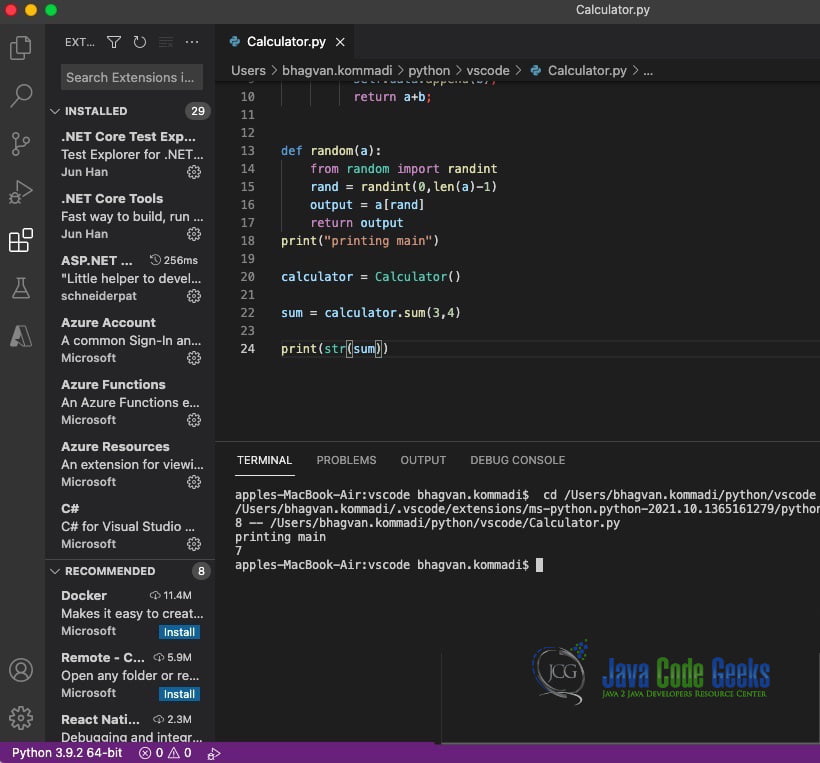
2.8 How to edit an Existing Python Project
You can download the existing python projects from this GitHub repository link. You can open the project after downloading and start editing the file reverse.py from the reverse folder. You can modify the code as shown below:
reverse.py
words = ['magnificent', 'world', 'hello', 'python'] words.sort(reverse=True) words = [ w[::-1] for w in words ] print(words) words.reverse() print("reverse order", words)
You can execute the code after modification. The executed code looks like as shown in the image below:
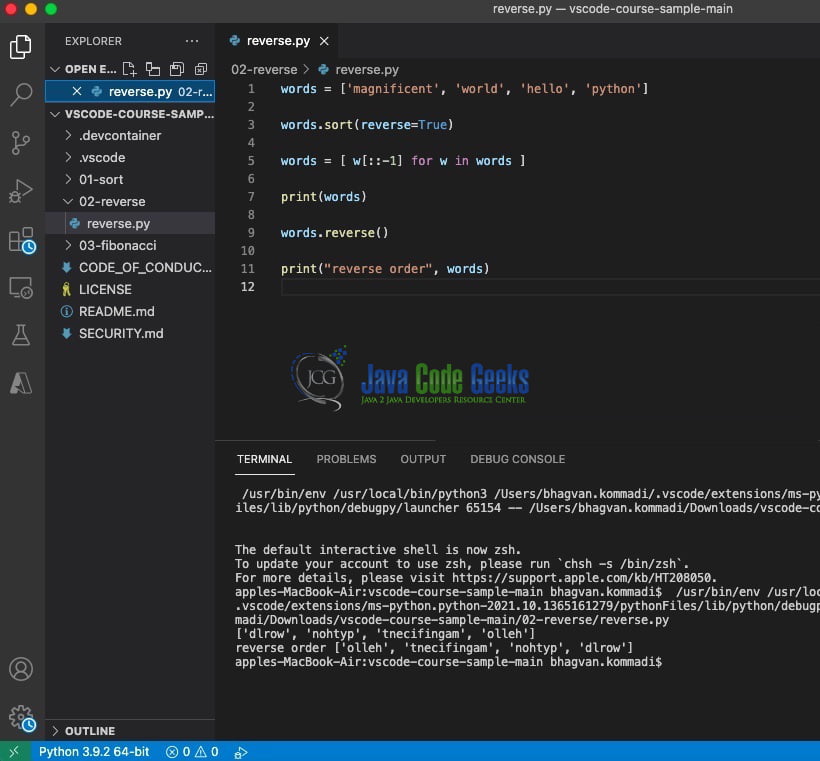
2.9 Testing support and Debugging support
Visual Studio Code supports testing support. You can write python tests using Pytest/Noise frameworks. Unit tests can be executed by selecting the Run Current Unit Test File. The test files are saved with extension _test.py. The configuration can be saved as settings.json in the .vscode folder. You can develop the test suite and execute them by selecting the Run Tests from the status bar.
You can also debug the code using the debugger. The other features of the debugger are automatic variable tracking, watch expressions, breakpoints, and call stack inspection. You can access these features from the Debug view on the activity bar.
3. Download the Source Code
You can download the full source code of this example here: Python Development with Visual Studio