Python Basics
In this article, we will explain some of the Python Basics.
1. What is Python?
Python is a popular programming language. It was created by Guido van Rossum, and released in 1991.
It is used for:
- web development (server-side)
- software development
- mathematics
- system scripting
You can also check this tutorial in the following video:
2. What can Python do?
- Python can be used on a server to create web applications.
- Python can be used alongside software to create workflows.
- Python can connect to database systems. It can also read and modify files.
- Python can be used to handle big data and perform complex mathematics.
- Python can be used for rapid prototyping, or for production-ready software development
- Not to mention, Python is the number 1 language used for Data Science, Machine learning and AI.
3. Why Python?
- Python works on different platforms (Windows, Mac, Linux, Raspberry Pi, etc).
- Python has a simple syntax similar to the English language.
- Python has syntax that allows developers to write programs with fewer lines than some other programming languages.
- Python runs on an interpreter system, meaning that code can be executed as soon as it is written. This means that prototyping can be very quick.
- Python can be treated in a procedural way, an object-oriented way or a functional way.
4. Python Installation
To check if you have python installed on a Windows PC, search in the start bar for Python or run the following on the Command Line (cmd.exe):
C:\Users\Your Name>python –version
If you find that you do not have python installed on your computer, then you can download it for free from the python website.
However, If you are using one of the methods below then Python will come with it.
4.1 Anaconda and Jupyter Notebook install
Anaconda Individual Edition is the world’s most popular Python distribution platform with over 25 million users worldwide. You can trust in our long-term commitment to supporting the Anaconda open-source ecosystem, the platform of choice for Python data science.
Download the distribution from here for your operating system
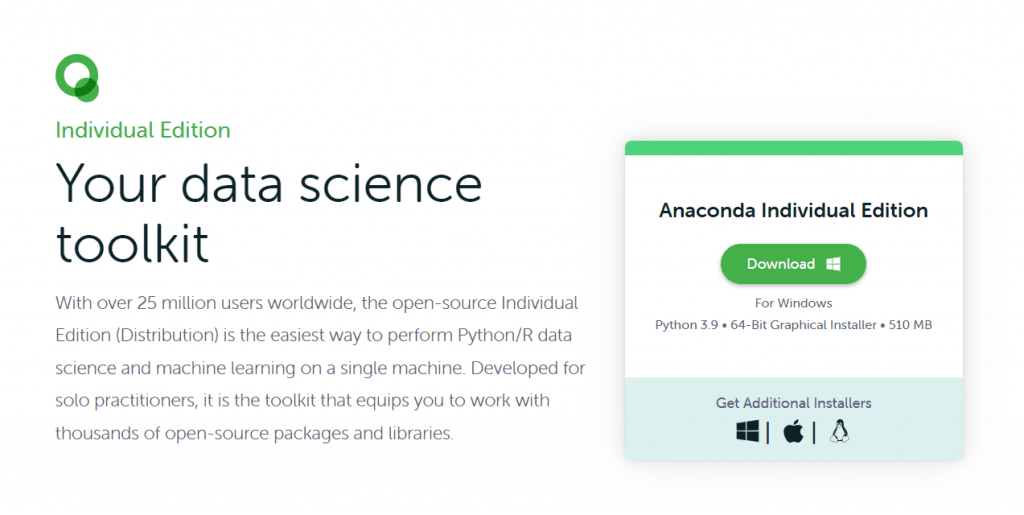
Install the distribution by opening the downloaded package and clicking through without changing any options.
After installation, you will see the following screen
Launch Jupyter notebooks from here.
4.2 PyCharm Install
Download PyCharm from here
5. Python Data Types
Data types are categorizations of data items. Python data types are meant to store different types of data such as numbers, numbers with decimals, characters, true/false values, a list of numbers or characters, etc. Python supports several data types. One neat thing about Python is that we don’t have a need to declare the type of any variable. Python will infer it from the value assigned to it.
The below-mentioned datatypes are called fundamental data types.
- Integers
- Floats
- Strings
- Boolean
- Lists
- Dictionaries
- Tuples
5.1 Integers
This is the most basic data type and the most frequently used. This data type is used to represent whole numbers e.g. 1, 2, 500, etc.
Declaring an Integer variable is very simple
x= 3
The above code creates a variable named x and assigns it a value of 3. The variable x will automatically have “int” as its data type. The code to check the data type can be very easily written as:
print(type(x))
Output : class 'int'
5.2 Floats
This is also one of the most basic data types and the most frequently used. This data type is used to represent decimal numbers e.g. 1.3, 2.45, etc.
Declaring a float variable is very simple
y= 3.3
floats can also be represented using scientific notation as follows:
x= 4.2e2
Any number bigger than 1.8 x 10308 is treated as infinity
Any number less than 5.0 x 10-324 is treated as zero
5.3 Booleans
This data type is used to represent true/false values. The most frequent use of this variable is in the loops for testing a given condition
Declaring a boolean variable is very simple
y= True
x= False
5.3.1 Truthy/Falsy values
In addition to the “True” and “False” values, Python also supports something called as “Truthy” and “Falsy” values. Lets look at these in a bit more detail.
5.3.1.1 Falsy Values
The following when tested in a condition will result in a “False” value
Sequences and Collections:
- Empty lists
[]
- Empty tuples
()
- Empty dictionaries
{}
- Empty sets
set()
- Empty strings
""
- Empty ranges
range(0)
Numbers
- Zero of any numeric type.
- Integer:
0
- Float:
0.0
- Complex:
0j
Constants
None
False
my_list = [] my_tuple = () my_set = {} my_dict = {} empty_str = "" x = 0 y = 0.0 z = None a = False if(my_list): print("This should not print") else: print("This should print") Output : This should print
In the above code my_list is empty and therefore it is a Falsy value and so evaluates to “False”. This is the reason the code runs the “else” block. The code will print the same result if the “if” condition is changed to use any of the other variables.
5.3.1.2 Truthy Values
Truthy values include:
- Non-empty sequences or collections (lists, tuples, strings, dictionaries, sets).
- Numeric values that are not zero.
True
5.4 Strings
Represents a series of characters aka Strings. Always enclosed in single ‘’ or double “ ” quotes e.g., “Python” or ‘Python’. This topic is discussed more in its separate chapter later. It is recommended to use one style and avoid mixing both styles in a given Python program.
Strings are the most heavily used Python data type.
Declaring a string variable is very simple
y= "Hello World"
5.5 Lists
List is one of the Python data types that is used to store more than one value in a single variable. Until this point, all the data types we have discussed are capable of storing only one value at a time.
List is a collection of any data objects. We can store multiple values of varying data types inside a single List.
List is defined using a square bracket [ ] and all the elements are separated using a comma “,”
my_list = [1, 2.5, "Python", True] print(vars) print(type(vars)) Output: [1, 2.5, 'Python', True] class 'list'
5.5.1 Accessing List elements
Lists are zero-based and ordered. They can be sliced to create sub-lists from a given list as shown below. The below code creates a list and then accesses all the elements using indices.
my_list = [1, "Python", 3.4, True] print(my_list[0]) print(my_list[1]) print(my_list[2]) print(my_list[3]) Output: 1 Python 3.4 True
5.5.2 Slicing a list
my_list = [1, "Python", 3.4, True] print(my_list[1:3]) print(my_list[0:3]) print(my_list[-4:-1]) Output: ['Python', 3.4] [1, 'Python', 3.4] [1, 'Python', 3.4]
my_list[1:3] : This prints the elements at positions 1 and 2.
my_list[0:3] : This prints the elements at positions 0, 1 and 2.
my_list[-4:-1] : This prints the elements at positions 0,1 and 2.
The end index is not included.
lists can also be accessed using negative indices. The last element is always at position -1 and the position of the other elements can be deduced by counting backward e.g. -4, -3, -2, -1
5.6 Tuples
Tuples are ordered collections of elements. Tuples are immutable. We cannot change the value of an element inside a tuple once assigned. Tuples are represented using circular brackets ( ). Indexing and Slicing operations are the same as the lists.
my_tuple= (1, "Python", 3.4, True) print(my_tuple[1:3]) print(my_tuple[0:3]) print(my_tuple[-4:-1]) Output: ['Python', 3.4] [1, 'Python', 3.4] [1, 'Python', 3.4]
We will get the following error if we try to change the value of an item inside a tuple.
my_tuple= (1, "Python", 3.4, True) my_tuple [1] = 2 # Try to change the value of the first element Output: my_tuple [1] = 2 TypeError: 'tuple' object does not support item assignment
5.7 Sets
Sets are a special case of lists where the elements can only be unique. Sets are not ordered and therefore cannot be indexed. Sets are immutable too.
Sets are represented by curly brackets { }
my_set= {1, "Python", 3.4, True} print(my_set) Output: {'Python', 1, 3.4}
If we try to include duplicate items in a Set, it will silently ignore the duplicates as shown below.
my_set= {"Python", "Python", 3.4, True} print(my_set) Output: {True, 3.4, 'Python'}
Note: The order of the elements when printed can be different than how it was declared.
5.8 Dictionaries
Dictionaries are a unique data type in Python. It represents data in a key-value pair structure. The keys inside a dictionary have to be unique. This is an unordered data type. Dictionaries are also represented by curly brackets { }.
Each key-value pair is separated by a comma “,”
my_dict= { 1: 1, 2: "Python", 3: 3.4, 4: True } print(my_dict) Output: {1: 1, 2: 'Python', 3: 3.4, 4: True}
In the above example, 1,2,3,4 are the keys, and data on the right side of “:” are the values.
5.8.1 Accessing using key
my_dict= { "a": 1, "b": "Python", "c": 3.4, "d": True } print(my_dict["b"]) print(my_dict["c"]) Output: Python 3.4
6. Python Control Flow
In Python, control flows are used to control the order in which the statements in a python program are executed.
This is achieved using Conditional statements, loops, and function calls.
By default, the statements in the script are executed sequentially from the first to the last. If the processing logic requires, the sequential flow can be altered in two ways:
- Conditional Execution : a block of one or more statements will be executed if a certain expression is true.
- Loops : a block of one or more statements will be executed repetitively as long as a certain expression is true.
6.1 Control Flow : if-else-elif statement
These statements are used to check a certain condition and execute a certain block of code if the condition is true and another block if the condition is not true. The syntax for the if statement is
if: #block of code #block of code #block of code elif: #block of code #block of code #block of code else: #block of code #block of code #block of code
The keyword if is followed by condition to be tested followed by “:”
The block of the code that is indented in relation to the if statement will get executed if the condition is true.
The elif keyword is used to specify an alternative condition. The block of the code that is indented in relation to the elif statement will get executed if the alternative condition is true.
The else block is used to specify a block of code that gets executed if none of the conditions above it are true.
A simple example is shown below
age = 13 if age > 17: print("You are an adult") elif 13 <= age <= 17: print("You are a teenager") else: print("You are a child") Output : You are a teenager
6.2 Loops
Iteration means executing the same block of code over and over again. A programming structure that implements iteration is called a loop.
In programming, there are 2 types of iteration, indefinite and definite:
- With indefinite iteration, the designated block is executed repeatedly as long as some condition is met.
- With definite iteration, the designated block is executed repeatedly fora fixed number of times. This number is explicitly at the time the loop starts
6.2.1 For Loops
This loop is generally used with sequence types such as a list, tuple, set and strings. The body of the for loop is executed for each member element of the sequence. The syntax for the “for” loop is
for x in sequence: #code block #code block #code block
A simple example of the for loop is
language = "Python" for x in language: print(x) Output: P y t h o n
In the above code the loop iterates “for” as many times as the number of characters in the word “Python” and then for each character the loop executes the print statement and prints each character. We can also use set, tuple or any other sequence instead of a string.
6.2.2 While Loops
A while loop is used to execute a block of code repeatedly and indefinitely as long as a given condition is true. The syntax for the while loop is
while : #code block #code block #code block
A simple example of the while loop is
num = 0 while num < 10: print(num) num = num+1 Output: 0 1 2 3 4 5 6 7 8 9
7. Python Functions
Functions are programming constructs that take some input, perform some operations on the input data, and may return an output. Having functions reduces the duplication of code in programs. It also eliminates the need to make changes in several places, should a change be required at a later stage.
A function can have one or more inputs or no inputs at all. These inputs are called function parameters. Generally, it is expected that a function will then process the parameters and return the result. While legal, it will be silly to define a function with parameters and not use them inside the function
There are 3 parts of a function definition
7.1 Function Headers
Python uses the keyword “def” to define the function. A function can have 0 or more inputs and/or outputs. The following is an example of a function header definition
def multiply(x,y):
The above defines a function named multiply that takes two input parameters named x and y.
We can also define functions in a way that does not require the caller to pass in an argument. Let’s say a function takes 3 parameters, we can declare one(any number actually) of those parameters to be optional. See the example below
def multiply(x,y,z=1):
In the above function z is the optional input. If the caller does not pass z, then z will be assumed to have a value of 1.
While legal. It will be silly to define a function such that all inputs are optional.
7.2 Function Body
The function body is indented in a code block, like loops and conditional statements. We can return one or more outputs using the return statement.
def multiply(x,y,z=1): return x*y*z
7.3 Function Naming
Function names:
- Can’t use spaces or special characters
- Can’t use Python keywords or built-in function names
- Should be descriptive and appropriate
7.4 Calling a Function
A function has to be defined before it can be used. The following code is illegal:
response = multiply(3,4) print(response) # Defines the function. def multiply(num1, num2): result = num1 * num2 return result #The correct code is # Defines the function def multiply(num1, num2): result = num1 * num2 return result # This is how a function is called and the result is stored in a variable response = multiply(3, 4) print(response)
8. Summary
In this article, we covered some very basics of the Python language. The content of this article is a must-know for every python beginner. We learned what python language is, what it is used for.
Then we looked at how to download and install some of the popular IDEs used for python coding. We also looked at some of the basic building blocks of the language such as basic data types and how to declare them.
This article also introduced the concept of loops and helps understand the difference between definite and indefinite loops. In that, we looked at for and while loops.
Finally, we understood how to define a function, how to name a function, and how to call a function.
9. Download the Source Code
You can download the source code discussed in this article from this section. Please comment and uncomment as needed to run specific code snippets in the main.py file.
You can download the full source code of this example here: Python Basics