PyGame: Game Programming in Python
1. Introduction
This is an article about PyGame and Game programming in Python.
Pygame has modules to develop games. The modules are related to sound, graphics, io, drawing, and other utilities in python. It was developed by Pete Shinners. Initially, it was PySDL. You can create games as a standalone package based on client-side apps.
You can also check this tutorial in the following video:
2. PyGame Examples
2.1 Prerequisites
Python 3.6.8 is required on windows or any operating system. Pycharm is needed for python programming. Pygame 2.1.0 needs to be installed.
2.2 Download
Python 3.6.8 can be downloaded from the website. Pycharm is available at this link.
2.3 Setup
2.3.1 Python Setup
To install python, the download package or executable needs to be executed.
2.3.2 PyGame Setup
You can install Pygame by using the command below:
PyGame installation
pip3 install pygame==2.1.0
2.4 IDE
2.4.1 Pycharm Setup
Pycharm package or installable needs to be executed to install Pycharm.
2.5 Launching IDE
2.5.1 Pycharm
Launch the Pycharm and start creating a pure python project named HelloWorld. The screen shows the project creation.
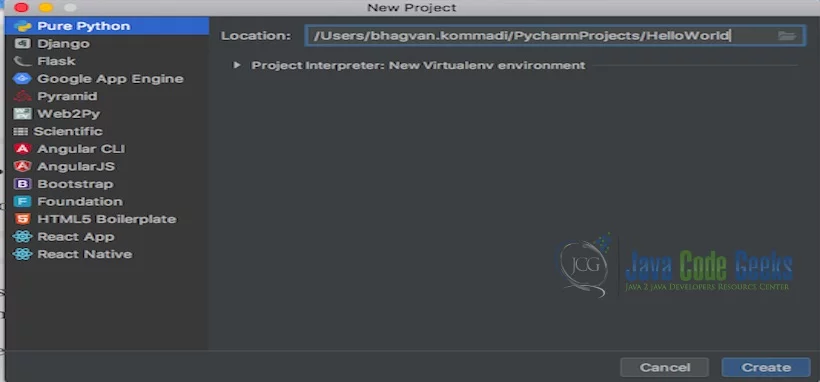
The project settings are set in the next screen as shown below.
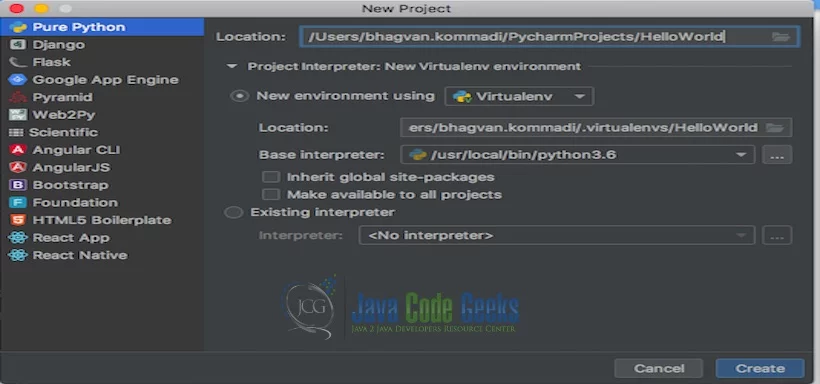
Pycharm welcome screen comes up shows the progress as indicated below.
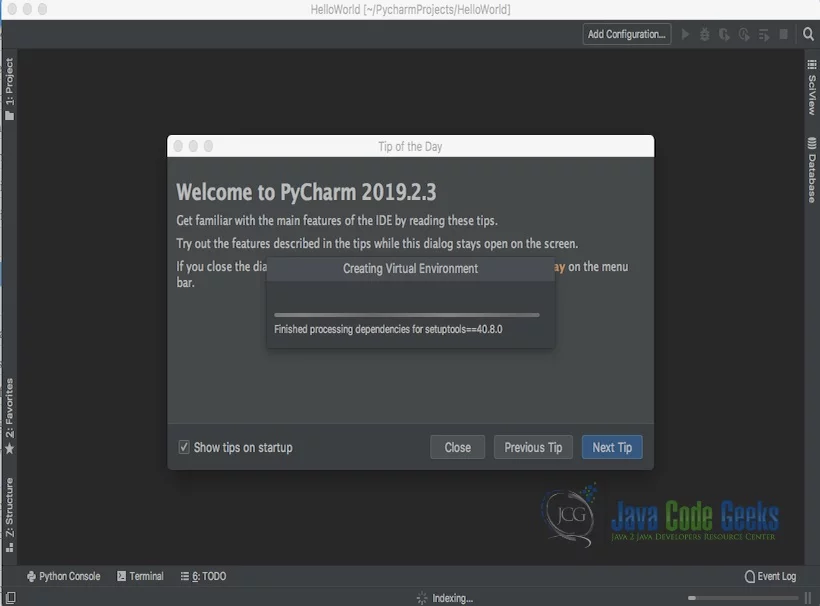
You can create a Hello.py and execute the python file by selecting the Run menu.
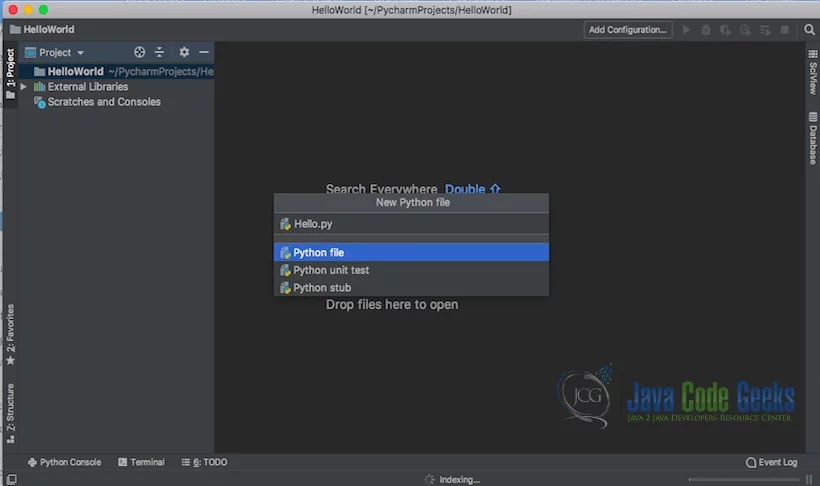
The output message “Hello World” is printed when the python file is Run.
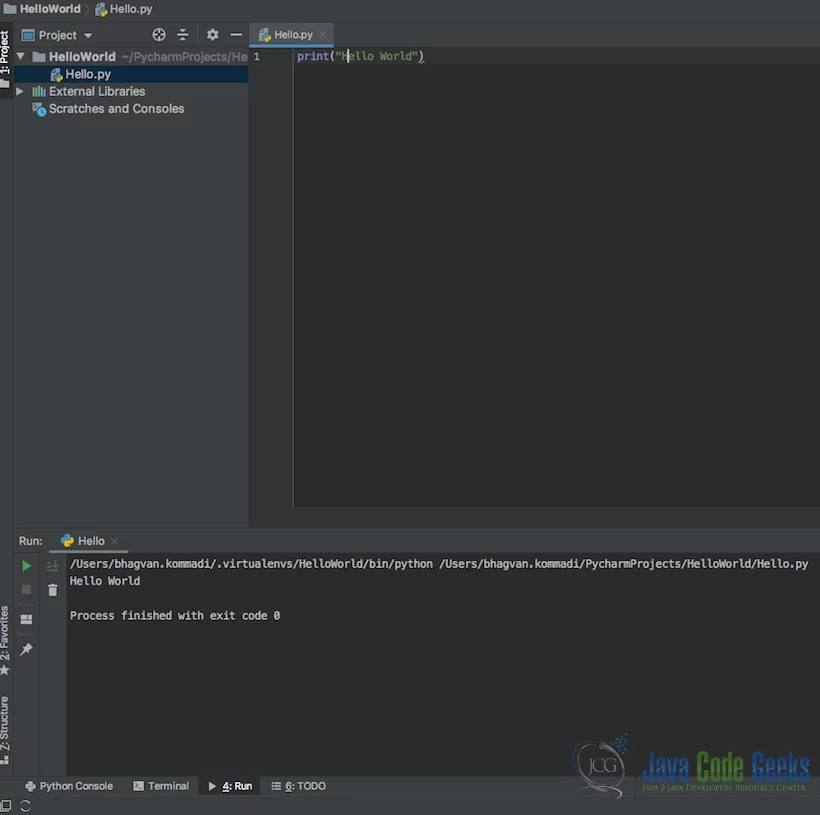
2.6 PyGame Background
PyGame was developed in python based on the Simple DirectMedia Layer (SDL) package. Simple DirectMedia Layer package is used for developing apps based on multimedia hardware libraries. Hardware libraries will give access to joystick, keyboard, mouse, video, and mouse. PyGame replaced PySDL which was initially written for game development. PySDL went dormant. You can develop multimedia applications using PyGame.
2.7 Basic PyGame Program
Let us start off writing a Game which has a window. The back ground color will be white and a circle with red color will be drawn in the middle.
PyGame Hello World
import pygame as pyg pyg.init() window = pyg.display.set_mode([500, 500]) loop = True while loop: for event in pyg.event.get(): if event.type == pyg.QUIT: loop = False window.fill((255, 255, 255)) pyg.draw.circle(window, (255, 0, 0), (250, 250), 75) pyg.display.flip() pyg.quit()
You can execute the above code using the command below:
PyGame Hello WorldCode Compilation
python3 pygame_hello_world.py
The output of the code executed is shown below:
2.8 Basic Game Design
Let us now look at the game design. Pygame framework has the functions for the pygame. The lifecycle functions like init(), display(), event.get(), and quit() are provided by the pygame module. You can use init for game initialization. The window’s display and setting the size are done using the display. display.flip() is used for getting updates.Event handling is done by event.get(). You can use quit() for exiting the game. If you have a new game in mind, you need to start creating a goal. There will be multiple stages with hurdles to overcome. The game player always starts from the screen left corner. The hurdles come from the right side and go straight in the left direction. Game players can move in directions like left, right, upside, and downside to overcome hurdles. The player loses if hit by the hurdle. If the player overcomes, he goes to the next stage. The game player is bound by the screen. The other features in a game are listed below:
- multiple lives
- scorekeeping
- player attack capabilities
- advancing levels
- Head characters
2.9 Importing and Initializing PyGame
Let us now look at importing and initialization of the pygame. The code below shows the steps.
Initialization – Pygame
import pygame as pyg from pyg.locals import ( K_UP, K_DOWN, K_LEFT, K_RIGHT, K_ESCAPE, KEYDOWN, QUIT, ) pyg.init()
2.10 Setting Up the Display
You can create the window by using pygame.display.set_mode(). WINDOW_WIDTH and WINDOW_HEIGHT are the dimensions of the window. The window has the borders and the title. You can see the code below on how to set up the display.
Display- Pygame
import pygame as pyg from pyg.locals import ( K_UP, K_DOWN, K_LEFT, K_RIGHT, K_ESCAPE, KEYDOWN, QUIT, ) pyg.init() WINDOW_WIDTH = 800 WINDOW_HEIGHT = 600 window = pyg.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
2.11 Setting Up the Game Loop
Now let us look at the Game loop. The game loop helps in controlling the game. The game loop can handle user input, state updates, display updates, audio output updates, and the speed of the game. The frame is referred to an iteration in a game. Game speed is controlled by the frame. The game will continue if the goal is not met by the player. If the game player is hit when crossing the hurdle, it is referred to as collision detection. Collision detection happens, the game ends. If the game player closes the screen, the game ends. Game player input is processed in the game loop to move the player in the window. Pygame provides an event framework to process the player inputs.
Game Loop
loop = True while loop: for event in pyg.event.get(): if event.type == KEYDOWN: if event.key == K_ESCAPE: loop = False elif event.type == QUIT: loop = False
2.12 Drawing on the Screen
Now let us look at the drawing on the game screen or window. The background is set using fill(). You can draw a circle using draw.circle() method. The surface also provides capabilities to draw.
Drawing Screen
window = pyg.display.set_mode([500, 500]) window.fill((255, 255, 255)) surface = pyg.Surface((50, 50)) surface.fill((0, 0, 0)) rectangle = surface.get_rect()
2.13 Sprites
A sprite is a two-dimensional form of the game window. You can use the Sprite class to display a game object on the window. You can create your own Custom Class which extends Sprite.
Player
class GamePlayer(pyg.sprite.Sprite): def __init__(self): super(GamePlayer, self).__init__() self.surf = pyg.Surface((75, 25)) self.surf.fill((255, 255, 255)) self.rect = self.surf.get_rect()
2.14 Players
You can create a Game Player by extending Sprite Class. You can create an init method which calls Sprite superclass method init. You can have a rectangular box created within a surface.
Player
import pygame as pyg from pyg.locals import ( K_UP, K_DOWN, K_LEFT, K_RIGHT, K_ESCAPE, KEYDOWN, QUIT, ) WINDOW_WIDTH = 800 WINDOW_HEIGHT = 600 class GamePlayer(pygame.sprite.Sprite): def __init__(self): super(GamePlayer, self).__init__() self.surface = pyg.Surface((75, 25)) self.surface.fill((0, 0, 0)) self.rect = self.surface.get_rect() pyg.init() window = pyg.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT)) gamePlayer = GamePlayer() loop = True while loop: for event in pyg.event.get(): if event.type == KEYDOWN: if event.key == K_ESCAPE: loop = False elif event.type == QUIT: loop = False window.fill((0, 0, 0)) window.blit(player.surf, (WINDOW_WIDTH/2, WINDOW_HEIGHT/2)) pyg.display.flip()
2.15 User Input
Now let us look at how user input is handled. Pygame has event framework methods like get() to get a list of events. The list has event types. You can get different events using the framework. The code below shows how to get all the events related to keys in every frame.
User Input
pressed_keys = pygame.key.get_pressed() class GamePlayer(pygame.sprite.Sprite): def __init__(self): super(GamePlayer, self).__init__() self.surface = pyg.Surface((75, 25)) self.surface.fill((255, 255, 255)) self.rect = self.surface.get_rect() def update(self, pressed_keys): if pressed_keys[K_UP]: self.rectangle.move_ip(0, -5) if pressed_keys[K_DOWN]: self.rectangle.move_ip(0, 5) if pressed_keys[K_LEFT]: self.rectangle.move_ip(-5, 0) if pressed_keys[K_RIGHT]: self.rectangle.move_ip(5, 0) while loop: for event in pyg.event.get(): if event.type == KEYDOWN: if event.key == K_ESCAPE: loop = False elif event.type == QUIT: loop = False pressed_keys = pyg.key.get_pressed() gamePlayer.update(pressed_keys) window.fill((0, 0, 0))
2.16 Sprite Groups and Custom Events
Now let us look at Sprite Groups. Sprite Group consists of a set of Sprite Objects. You can use Sprite Group when you want to track a set of Sprite Objects. In a scenario, like Game Player is hit by the hurdle, the updates need to happen to multiple objects as it is an exit scenario. In this scenario, Sprite groups will be created for Enemy hurdles and another for all Sprites in the game. Every time an enemy hurdle gets created, Sprite group – Enemy hurdle needs to be updated by adding this. Custom Event can be created for updating when an enemy hurdle is added. You can see how custom events are created in the code below.
Sprite Groups and Custom Events
gamePlayer = GamePlayer() enemyHurdles = pyg.sprite.Group() all_sprites = pyg.sprite.Group() all_sprites.add(gamePlayer) window.fill((0, 0, 0)) for entity in all_sprites: window.blit(entity.surf, entity.rect) pyg.display.flip() window = pyg.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT)) ADDENEMYHURDLE = pyg.USEREVENT + 1 pyg.time.set_timer(ADDENEMYHURDLE, 250) loop = True while loop: for event in pygame.event.get(): if event.type == KEYDOWN: if event.key == K_ESCAPE: running = False elif event.type == QUIT: running = False elif event.type == ADDENEMY: new_enemy = Enemy() enemies.add(new_enemy) all_sprites.add(new_enemy) pressed_keys = pygame.key.get_pressed() player.update(pressed_keys) enemies.update()
2.17 Collision Detection, Sprite Images and Sound Effects
Collision detection is needed when the Game Player is hit by the enemy hurdle. PyGame framework has spritecollideany on Sprite class which is used to check every object in the group intersects with the .rect of the Sprite. Regarding sound effects, a mixer module is used. You can use the Music sub module for handling sound files with formats like MP3, Ogg, and Mod.
Collision Detection, Sprite Images and Sound Effects
pyg.mixer.init() pyg.init() clock = pyg.time.Clock() pyg.mixer.music.load("sound_of_music.mp3") pyg.mixer.music.play(loops=-1) move_up_sound = pyg.mixer.Sound("Rising_Sun.ogg") move_down_sound = pyg.mixer.Sound("Falling_Water.ogg") collision_sound = pyg.mixer.Sound("Batman.ogg")
2.18 Pygame – Game Example
Let us now create a small game with two cubes – one as a player and the other as the enemy to be killed. The code sample is attached below:
PyGame Example
import pygame as pyg import sys class Sprite(pyg.sprite.Sprite): def __init__(self, pos): pyg.sprite.Sprite.__init__(self) self.image = pyg.Surface([20, 20]) self.image.fill((255, 0, 255)) self.rect = self.image.get_rect() self.rect.center = pos def main(): pyg.init() clock = pyg.time.Clock() fps = 50 bg = [255, 255, 255] size =[300, 300] window = pyg.display.set_mode(size) gamePlayer = Sprite([40, 50]) gamePlayer.move = [pyg.K_LEFT, pyg.K_RIGHT, pyg.K_UP, pyg.K_DOWN] gamePlayer.vx = 5 gamePlayer.vy = 5 wall = Sprite([100, 60]) wall_group = pyg.sprite.Group() wall_group.add(wall) gamePlayer_group = pyg.sprite.Group() gamePlayer_group.add(gamePlayer) while True: for event in pyg.event.get(): if event.type == pyg.QUIT: return False key = pyg.key.get_pressed() for i in range(2): if key[gamePlayer.move[i]]: gamePlayer.rect.x += gamePlayer.vx * [-1, 1][i] for i in range(2): if key[gamePlayer.move[2:4][i]]: gamePlayer.rect.y += gamePlayer.vy * [-1, 1][i] window.fill(bg) collision = pyg.sprite.spritecollide(gamePlayer, wall_group, True) if collision: gamePlayer.image.fill((0, 0, 0)) gamePlayer_group.draw(window) wall_group.draw(window) pyg.display.update() clock.tick(fps) pyg.quit() sys.exit if __name__ == '__main__': main()
You can execute the above code using the command below:
PyGame Example Code Compilation
python3 pygame_example.py
The output of the code executed is shown below:
3. Download the Source Code
You can download the full source code of this example here: PyGame: Game Programming in Python
Last updated on Jan. 17th, 2022