OOP Python Tutorial
1. Introduction
This is an article about oop in python. Python can be used for developing software and executing the code. Python is a programming language that has dynamic types. Python started off in a research firm based at Netherlands. The goal of this language was to reduce the gap between the shell commands syntax and C. The motivation of the language comes from different languages such as Algol68, Pascal and ABC.
2. OOP Python Tutorial
2.1 Prerequisites
Python 3.6.8 is required on windows or any operating system. Pycharm is needed for python programming.
2.2 Download
Python 3.6.8 can be downloaded from the website. Pycharm is available at this link.
2.3 Setup
2.3.1 Python Setup
To install python, the download package or executable needs to be executed.
2.4 IDE
2.4.1 Pycharm Setup
Pycharm package or installable needs to be executed to install Pycharm.
2.5 Launching IDE
2.5.1 Pycharm
Launch the Pycharm and start creating a pure python project named HelloWorld. The screen shows the project creation.
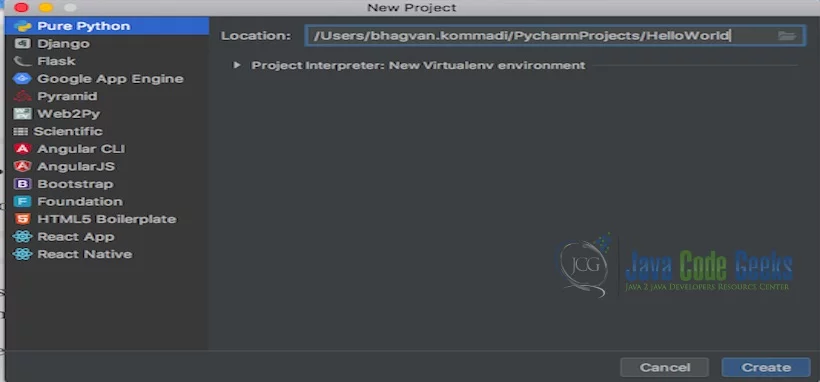
The project settings are set in the next screen as shown below.
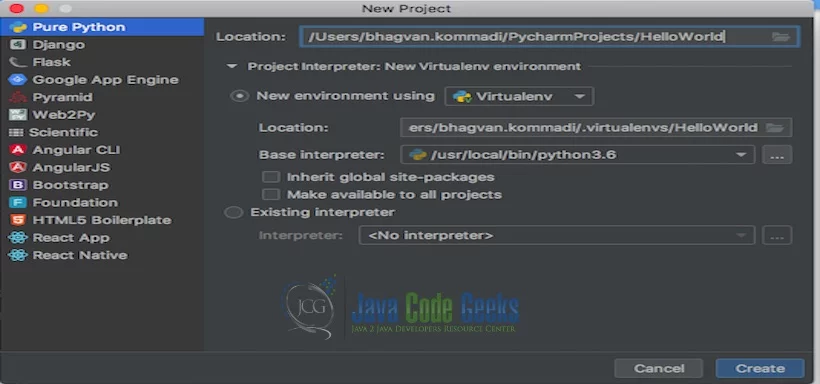
Pycharm welcome screen comes up shows the progress as indicated below.
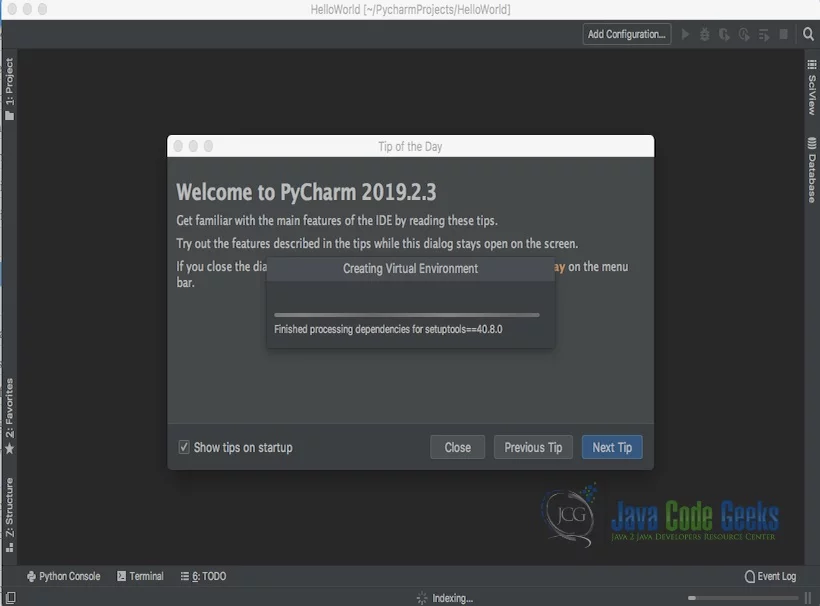
You can create a Hello.py and execute the python file by selecting the Run menu.
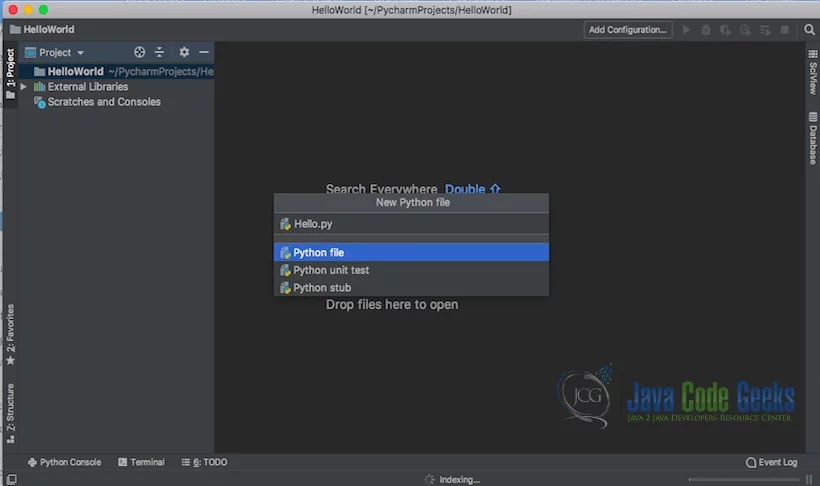
The output message “Hello World” is printed when the python file is Run.
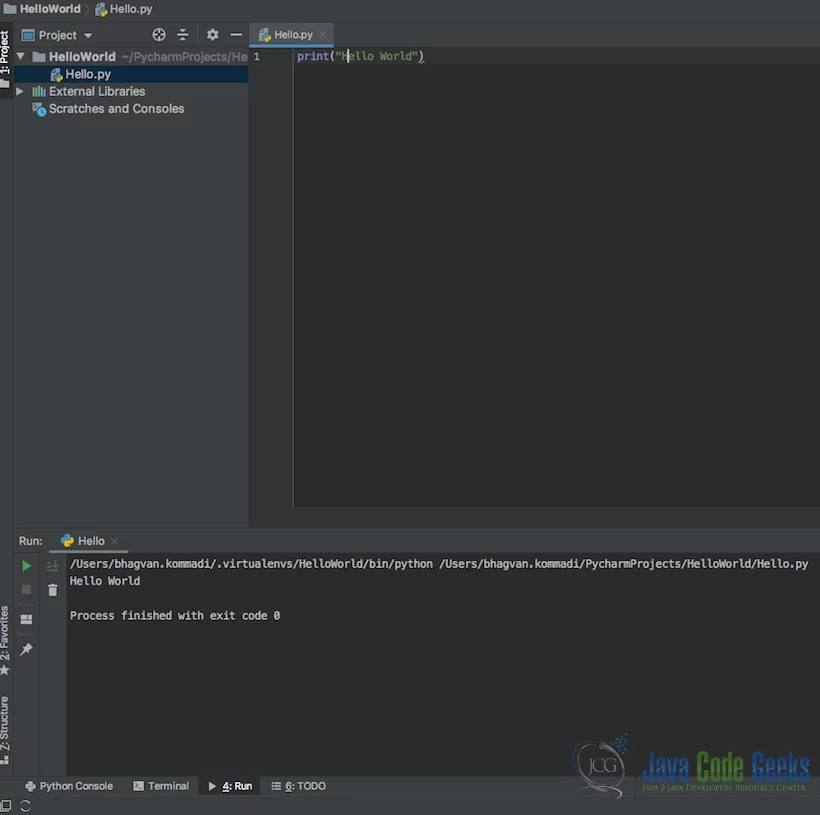
2.6 What Is Object-Oriented Programming in Python?
Python support multiple programming paradigms. Object oriented programming is one of the paradigms supported by the language. Object have attributes and behavior. Now let us look at an example, Truck has attributes such as name, model, color, and speed. It has behaviors like speeding and driving. Reusability is one of the aspects of Python. Don’t repeat Yourself (DRY) is a very popular concept based on reusability.
2.7 Defining a Class
In python, a class represents the objects’ blueprint. Truck is a class of objects which have name, model, color and speed. Truck is an object. Class can be defined as below:
Class definition
class Truck: pass
An empty class Truck is defined using the class keyword. We can have instances created by using the class. You can perform operations on the class after instantiating it.
2.8 Instantiate an Object
An instance of a class is an object. Class Truck is instantiated as below:
Class instantiation
truck = Truck()
In the above example, truck object is created using Truck class constructor.
2.9 Inherit From Other Classes
You can have in python inheritance modeled using the base class, parent class, and derived class. Vehicle, Machine, and Truck class examples are shown. The truck can inherit from Vehicle and Machine as shown below:
Class Inheritance
class Vehicle: def __init__(self): self.velocity = 50 def getVelocity(): return self.velocity class Machine: def __init__(self): self._distanceTravelled = 100 def getDistance(): return self.distanceTravelled class Truck(Vehicle, Machine): def __init__(self, velocity,time): Vehicle.__init__(self) Machine.__init__(self) self.velocity = velocity self.time = time def getVelocity(): return self.distanceTravelled/self.time def getDistance(): return self.velocity * time
2.10 What are Instance Methods and how do we use them?
You can have instance methods which are inside the body of a python class. Methods represent the behaviors of an object.
Methods are functions defined inside the body of a class. They are used to define the behaviors of an object.
Class Instance Methods
class Truck: def __init__(self, name, color): self.name = name self.color = color def speed(self, speed): return "{} speeding at {}".format(self.name, speed) truck = Truck("Wagon","Red") print(truck.speed(20))
3. Download the Source Code
You can download the full source code of this example here: OOP Python Tutorial: Object Oriented Programming