Java Quartz Update Trigger Example
In this example, we will learn how to update a trigger in Quartz.
1. Introduction
Quartz is a richly featured, open-source job scheduling library that can be integrated within virtually any Java application – from the smallest stand-alone application to the largest e-commerce system. Quartz can be used to create simple or complex schedules for executing tens, hundreds, or even tens-of-thousands of jobs; jobs whose tasks are defined as standard Java components that may execute virtually anything you may program them to do.
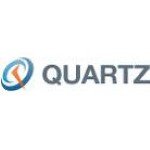
Quartz can run embedded within another free-standing application. Quartz can be instantiated within an application server (or servlet container), and participate in XA transactions. Quartz can run as a stand-alone program (within its own Java Virtual Machine), to be used via RMI. Quartz can be instantiated as a cluster of stand-alone programs (with load-balance and fail-over capabilities) for the execution of jobs.
2. Code
In this section, we will see a working example of updating a trigger. First, we will create a simple job. Every Quartz Job needs to implement the org.quartz.Job
interface. The instance of this Job
must have a public no-argument constructor.
ExampleJob.java
package org.javacodegeeks; import org.quartz.Job; import org.quartz.JobExecutionContext; public class ExampleJob implements Job { public void execute(JobExecutionContext jobExecutionContext) { try { Thread.sleep(3000); } catch (InterruptedException e) { e.printStackTrace(); } } }
Now let’s see how we can schedule this job to run at a specific time. First, we need to create a Scheduler
instance using the SchedulerFactory
.
SchedulerFactory schedulerFactory = new StdSchedulerFactory(); Scheduler scheduler = schedulerFactory.getScheduler();
SchedulerFactory
provides a mechanism for obtaining client-usable handles to Scheduler instances. A Scheduler maintains a registry of JobDetails and Triggers. Once registered, the Scheduler is responsible for executing Jobs when they are associated Triggers
fire (when their scheduled time arrives).
Scheduler
instances are produced by a SchedulerFactory
. A scheduler that has already been created/initialized can be found and used through the same factory that produced it. After a Scheduler
has been created, it is in “stand-by” mode, and must have its start() method called before it will fire any Jobs
.
Jobs
are to be created by the ‘client program’, by defining a class that implements the Job
interface. JobDetail
objects are then created (also by the client) to define an individual instance of the Job
. JobDetail
instances can then be registered with the Scheduler
via the scheduleJob(JobDetail, Trigger)
or addJob(JobDetail, boolean)
method.
Triggers
can then be defined to fire individual Job instances based on given schedules. SimpleTriggers
are most useful for one-time firings, or firing at an exact moment in time, with N
repeats with a given delay between them. CronTriggers
allow scheduling based on time of day, day of week, day of month, and month of year.
Jobs
and Triggers
have a name and group associated with them, which should uniquely identify them within a single Scheduler
. The ‘group’ feature may be useful for creating logical groupings or categorizations of Jobs
and Triggers
. If you don’t have need for assigning a group to a given Jobs
of Triggers
, then you can use the DEFAULT_GROUP constant defined on this interface.
Now let’s create out Job
instance:
JobDetail job = newJob(ExampleJob.class).withIdentity("MyJobName", "MyJobGroup").build();
Now let’s create the Trigger
instance:
Date date = Date.from(LocalDateTime.now().plusSeconds(2).atZone(ZoneId.systemDefault()).toInstant()); Trigger trigger = newTrigger().withIdentity("MyTriggerName", "MyTriggerGroup").startAt(date).build();
Now let’s add a value in the job data map:
trigger.getJobDataMap().put("triggerUpdated", false);
Let’s schedule the job:
scheduler.scheduleJob(job, trigger);
We can check the value of the attribute set:
scheduler.getTrigger(trigger.getKey()).getJobDataMap().getBoolean("triggerUpdated")
Now let’s update the value:
trigger.getJobDataMap().put("triggerUpdated", true);
Let’s re-schedule the job:
scheduler.rescheduleJob(trigger.getKey(), trigger);
Let’s check the value of the attribute again:
scheduler.getTrigger(trigger.getKey()).getJobDataMap().getBoolean("triggerUpdated")
Below is the full source code of the scheduler class:
UpdateTriggerExample.java
package org.javacodegeeks; import org.quartz.*; import org.quartz.impl.StdSchedulerFactory; import java.time.LocalDateTime; import java.time.ZoneId; import java.util.Date; import static org.quartz.JobBuilder.newJob; import static org.quartz.TriggerBuilder.newTrigger; public class UpdateTriggerExample { public static void main(String[] args) { UpdateTriggerExample deleteJobExample = new UpdateTriggerExample(); deleteJobExample.run(); } private void run() { // First we must get a reference to a scheduler SchedulerFactory schedulerFactory = new StdSchedulerFactory(); try { Scheduler scheduler = schedulerFactory.getScheduler(); // define the job and tie it to our HelloJob class JobDetail job = newJob(ExampleJob.class).withIdentity("MyJobName", "MyJobGroup").build(); // Trigger the job to run after 3 seconds Date date = Date.from(LocalDateTime.now().plusSeconds(2).atZone(ZoneId.systemDefault()).toInstant()); Trigger trigger = newTrigger().withIdentity("MyTriggerName", "MyTriggerGroup").startAt(date).build(); trigger.getJobDataMap().put("triggerUpdated", false); // Tell quartz to schedule the job using our trigger scheduler.scheduleJob(job, trigger); System.out.println("Trigger Updated: " + scheduler.getTrigger(trigger.getKey()).getJobDataMap().getBoolean("triggerUpdated")); trigger.getJobDataMap().put("triggerUpdated", true); scheduler.rescheduleJob(trigger.getKey(), trigger); System.out.println("Trigger Updated: " + scheduler.getTrigger(trigger.getKey()).getJobDataMap().getBoolean("triggerUpdated")); // Start up the scheduler (nothing can actually run until the scheduler has been started) scheduler.start(); try { Thread.sleep(4*1000); } catch (Exception e) { } // Shutdown the scheduler scheduler.shutdown(true); } catch (SchedulerException e) { e.printStackTrace(); } } }
When you will run this example you will see the output as below:
Trigger Updated: false Trigger Updated: true
3. Summary
In this example, we discussed the Quartz scheduler. We learned what we can do with the scheduler and especially how we can update the trigger. We discussed that if we want to update the trigger we will need to re-schedule the job. We also discussed some of the important classes which is used in scheduling a simple job.
4. Download the source code
You can download the full source code of this example here: Java Quartz Update Trigger Example