Java Quartz Get Job Status Example
In this article, we will discuss how to check the status of a Quartz job. Quartz is a richly featured, open-source job scheduling library that can be integrated with any Java application.
1. Introduction
Quartz can be used to create simple or complex schedules for executing tens, hundreds, or even tens-of-thousands of jobs; jobs whose tasks are defined as standard Java components that may execute virtually anything we may program them to do.
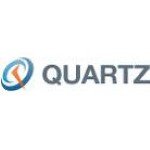
Quartz can run embedded within another free-standing application. It can be instantiated within an application server (or servlet container), and participate in XA transactions. It can run as a stand-alone program (within its own Java Virtual Machine), to be used via RMI
2. Quartz Job Execution
Jobs can be any Java class that implements the simple interface, leaving infinite possibilities for the work your Jobs can perform. Job
class instances can be instantiated by Quartz, or by your application’s framework.
When a Trigger
occurs, the scheduler notifies zero or more Java objects implementing the JobListener
and TriggerListener
interfaces (listeners can be simple Java objects, or EJBs, or JMS publishers, etc.). These listeners are also notified after the Job
has executed.
As Jobs are completed, they return a JobCompletionCode
which informs the scheduler of success or failure. They can also instruct the scheduler of any actions it should take based on the success/fail code – such as immediate re-execution of the Job.
3. Code
In this section, we will see the coding part. First, we will create a simple job. To create a job we need to implement the org.quartz.Job interface. The instance of these jobs must have a no-argument constructor. This interface has one method: execute(JobExecutionContext)
. All the jobs need to implement this method.
ExampleJob.java
package org.javacodegeeks; import org.quartz.Job; import org.quartz.JobExecutionContext; public class ExampleJob implements Job { public void execute(JobExecutionContext jobExecutionContext) { System.out.println("Waiting for 3 seconds"); try { Thread.sleep(3000); } catch (InterruptedException e) { e.printStackTrace(); } } }
As we can see this is a very simple job which just waits for 3 seconds before finishing.
Now we will create the scheduler class. First, we will get the Scheduler
instance using the SchedulerFactory
:
Scheduler scheduler = schedulerFactory.getScheduler();
Now we will create a new Job
using the utility method provided in org.quartz.JobBuilder
class.
JobDetail job = newJob(ExampleJob.class).withIdentity("MyJobName", "MyJobGroup").build();
Now we will create a new Trigger
and will schedule the job to be run after 5 seconds:
Date date = Date.from(LocalDateTime.now().plusSeconds(5).atZone(ZoneId.systemDefault()).toInstant()); Trigger trigger = newTrigger().withIdentity("MyTriggerName", "MyTriggerGroup").startAt(date).build();
Now we will tell quartz to schedule the job using our trigger:
scheduler.scheduleJob(job, trigger);
Now lets start the scheduler:
scheduler.start();
We can get the trigger state from schedule by using the getTriggerState()
method:
scheduler.getTriggerState(trigger.getKey())
QuartzExample.java
package org.javacodegeeks; import org.quartz.*; import org.quartz.impl.StdSchedulerFactory; import java.time.LocalDateTime; import java.time.ZoneId; import java.util.Date; import static org.quartz.JobBuilder.newJob; import static org.quartz.TriggerBuilder.newTrigger; public class QuartzExample { public static void main(String[] args) { QuartzExample quartzExample = new QuartzExample(); quartzExample.run(); } private void run() { // First we must get a reference to a scheduler SchedulerFactory schedulerFactory = new StdSchedulerFactory(); try { Scheduler scheduler = schedulerFactory.getScheduler(); // define the job and tie it to our HelloJob class JobDetail job = newJob(ExampleJob.class).withIdentity("MyJobName", "MyJobGroup").build(); // Trigger the job to run after 5 seconds Date date = Date.from(LocalDateTime.now().plusSeconds(5).atZone(ZoneId.systemDefault()).toInstant()); Trigger trigger = newTrigger().withIdentity("MyTriggerName", "MyTriggerGroup").startAt(date).build(); // Tell quartz to schedule the job using our trigger scheduler.scheduleJob(job, trigger); System.out.println(job.getKey() + " will run at: "+ date); System.out.println(String.format("Trigger %s state: %s", trigger.getKey().getName(), scheduler.getTriggerState(trigger.getKey()))); // Start up the scheduler (nothing can actually run until the scheduler has been started) scheduler.start(); // wait long enough so that the scheduler has an opportunity to run the job! System.out.println("Waiting for 5 seconds"); try { Thread.sleep(5*1000); } catch (Exception e) { } // Shutdown the scheduler scheduler.shutdown(true); } catch (SchedulerException e) { e.printStackTrace(); } } }
4. Summary
In this example, we saw what Quartz jobs are, how to check their status, and how we can use them. We also saw how we can get the trigger state using the scheduler.
You can check more of our Quartz tutorials here.
5. Download the source code
That was an example of the Java Quartz Get Job Status.
You can download the full source code of this example here: Java Quartz Get Job Status Example