Java Quartz Cancel Job Example
In this article we will learn how to cancel a quartz job.
1. Introduction
Quartz is a richly featured, open-source job scheduling library that can be integrated within virtually any Java application – from the smallest stand-alone application to the largest e-commerce system. Quartz can be used to create simple or complex schedules for executing tens, hundreds, or even tens-of-thousands of jobs; jobs whose tasks are defined as standard Java components that may execute virtually anything you may program them to do.
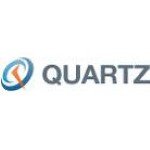
Quartz can run embedded within another free-standing application. Quartz can be instantiated within an application server (or servlet container), and participate in XA transactions. Quartz can run as a stand-alone program (within its own Java Virtual Machine), to be used via RMI. Quartz can be instantiated as a cluster of stand-alone programs (with load-balance and fail-over capabilities) for the execution of jobs.
2. Code
First, we will see how to schedule a Job. We will create a very simple job. To create a Quartz job we need to implements the org.quartz.Job
interface.
ExampleJob.java
package org.javacodegeeks; import org.quartz.Job; import org.quartz.JobExecutionContext; public class ExampleJob implements Job { public void execute(JobExecutionContext jobExecutionContext) { System.out.println("Job execution started - waiting for 3 seconds"); try { Thread.sleep(3000); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("Job Completed"); } }
First, we need to get the Scheduler
instance. For this we will make use of the getScheduler()
method of SchedulerFactory the class:
SchedulerFactory schedulerFactory = new StdSchedulerFactory(); Scheduler scheduler = schedulerFactory.getScheduler();
StdSchedulerFactory
is an implementation of org.quartz.SchedulerFactory
that does all the work of creating QuartzScheduler
instance.
Now we will create a Job
instance. For this, we will make use of the org.quartz.JobBuilder
:
JobDetail job = newJob(ExampleJob.class).withIdentity("MyJobName", "MyJobGroup").build();
Now we will create a Trigger
instance which will execute the Job
in 3 seconds:
Trigger trigger = newTrigger().withIdentity("MyTriggerName", "MyTriggerGroup").startAt(date).build();
Now we will tell quartz to schedule the job using our trigger:
scheduler.scheduleJob(job, trigger);
Now lets start the job:
scheduler.start();
2.1. Delete job
In this section we will see how to delete an existing Job
. The Scheduler
provides a deleteJob()
method which deletes the identitfied Job
from the Scheduler
and any associated Triggers
.
scheduler.deleteJob(job.getKey());
The method returns true if the Job
was found and deleted.
DeleteJobExample.java
package org.javacodegeeks; import org.quartz.*; import org.quartz.impl.StdSchedulerFactory; import java.time.LocalDateTime; import java.time.ZoneId; import java.util.Date; import static org.quartz.JobBuilder.newJob; import static org.quartz.TriggerBuilder.newTrigger; public class DeleteJobExample { public static void main(String[] args) { DeleteJobExample deleteJobExample = new DeleteJobExample(); deleteJobExample.run(); } private void run() { // First we must get a reference to a scheduler SchedulerFactory schedulerFactory = new StdSchedulerFactory(); try { Scheduler scheduler = schedulerFactory.getScheduler(); // define the job and tie it to our HelloJob class JobDetail job = newJob(ExampleJob.class).withIdentity("MyJobName", "MyJobGroup").build(); // Trigger the job to run after 3 seconds Date date = Date.from(LocalDateTime.now().plusSeconds(3).atZone(ZoneId.systemDefault()).toInstant()); Trigger trigger = newTrigger().withIdentity("MyTriggerName", "MyTriggerGroup").startAt(date).build(); // Tell quartz to schedule the job using our trigger scheduler.scheduleJob(job, trigger); System.out.println(job.getKey() + " will run at: "+ date); // Start up the scheduler (nothing can actually run until the scheduler has been started) scheduler.start(); System.out.println("Deleting the job" + job.getKey()); scheduler.deleteJob(job.getKey()); // wait long enough so that the scheduler has an opportunity to run the job! System.out.println("Waiting for 4 second"); try { Thread.sleep(4*1000); } catch (Exception e) { } // Shutdown the scheduler scheduler.shutdown(true); } catch (SchedulerException e) { e.printStackTrace(); } } }
When you will run above code you will see output as below:
MyJobGroup.MyJobName will run at: Thu Oct 01 20:58:18 BST 2020 Deleting the jobMyJobGroup.MyJobName Waiting for 4 second
2.2 Unschedule Job
We can unschedule a Job
by calling the unschedule()
method of the Scheduler
class and passing the TriggerKey
. If the related job does not have any other triggers, and the job is not durable, then the job will also be deleted.
3. Summary
In this example, we talked about how to schedule a Quartz Job and how to delete one. We saw that there are two ways we can achieve this. The first one is by unscheduling the job. This will remove the indicated Trigger from the Scheduler. The second way is to delete the job. This also deletes any associated trigger.
4. Download the source code
That was a Java Quartz Cancel Job Example.
You can download the full source code of this example here: Java Quartz Cancel Job Example