Java Pointers (References) Example
1. Overview
In this article, we will take a look at the comparison of Java Pointers (References ) with C++ Pointers. Java has four types of references which are strong, weak, soft, and phantom references. In C++, you can use references and java pointer.
2. Java Pointers (References)
2.1 Prerequisites
Java 8 is required on the Linux, windows or mac operating system. Eclipse Oxygen can be used for this example. Eclipse C++ is necessary on the operating system in which you want to execute the code.
2.2 Download
You can download Java 8 from the Oracle web site . Eclipse Oxygen can be downloaded from the eclipse web site. Eclipse C++ is available at this link.
2.3 Setup
2.3.1 Java Setup
Below is the setup commands required for the Java Environment.
Setup
JAVA_HOME="/desktop/jdk1.8.0_73" export JAVA_HOME PATH=$JAVA_HOME/bin:$PATH export PATH
2.3.2 C++ Setup
Eclipse C++ installation sets the environment for C++ development and project building.
2.4 IDE
2.4.1 Eclipse Oxygen Setup
The ‘eclipse-java-oxygen-2-macosx-cocoa-x86_64.tar’ can be downloaded from the eclipse website. The tar file is opened by double click. The tar file is unzipped by using the archive utility. After unzipping, you will find the eclipse icon in the folder. You can move the eclipse icon from the folder to applications by dragging the icon.
2.4.2 Eclipse C++ Setup
The ‘eclipse-cpp-2019-06-R-macosx-cocoa-x86_64.dmg’ can be downloaded from the eclipse C/C++ website. After installing the application on macos, you will find the eclipse icon in the folder. You can move the eclipse icon from the folder to applications by dragging the icon.
2.5 Launching IDE
2.5.1 Eclipse Java
Eclipse has features related to language support, customization, and extension. You can click on the eclipse icon to launch eclipse. The eclipse screen pops up as shown in the screen shot below:
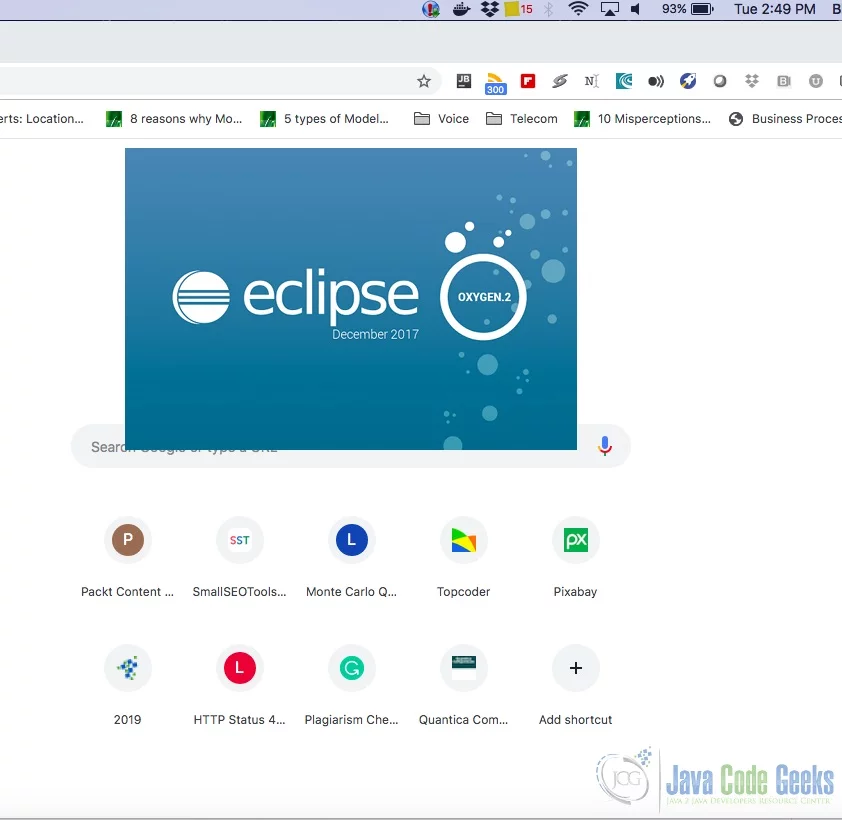
You can select the workspace from the screen which pops up. The attached image shows how it can be selected.
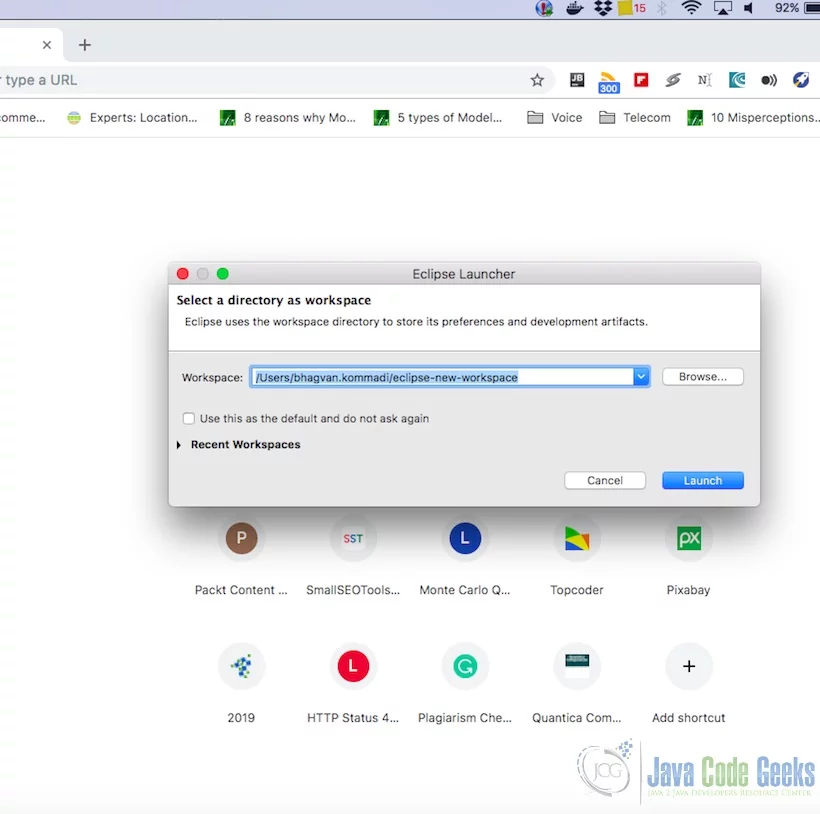
You can see the eclipse workbench on the screen. The attached screen shot shows the Eclipse project screen.
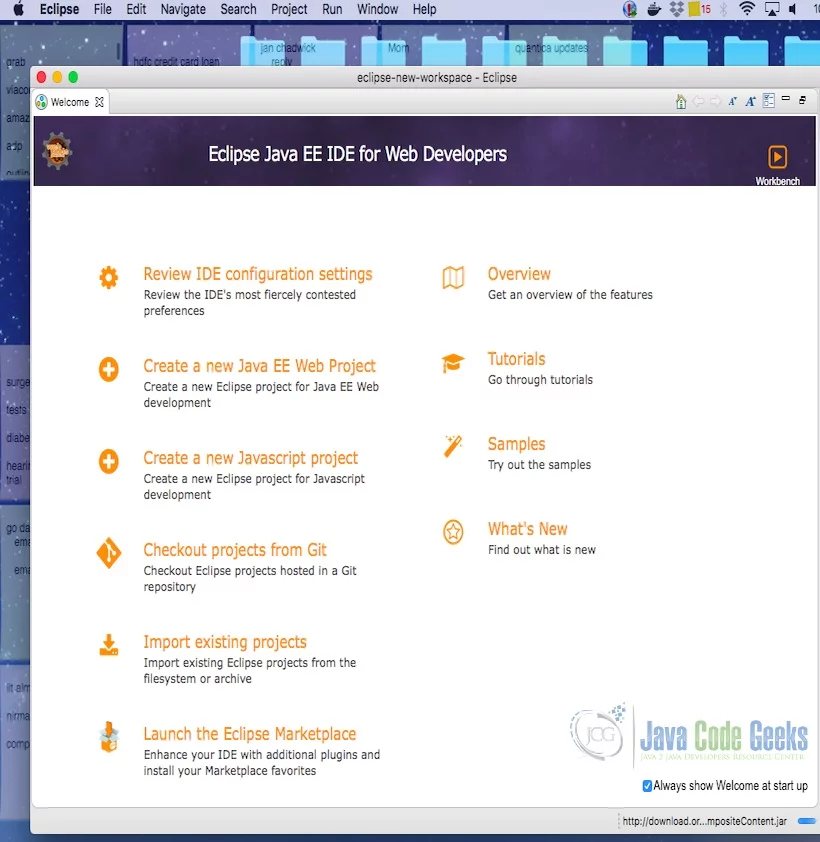
Java Hello World
class prints the greetings. The screenshot below is added to show the class and execution on eclipse.
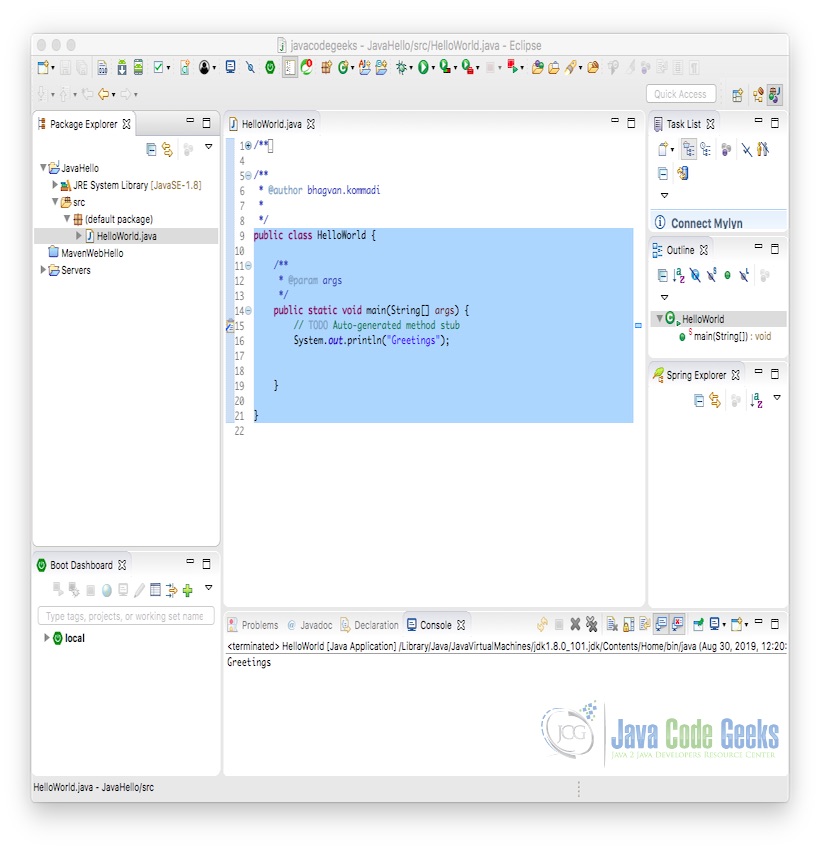
2.5.1 Eclipse C++
C++ code is created to print “Hello World” and executed on Eclipse C++. The screenshot below shows the Hello World in C++ and the printed output.
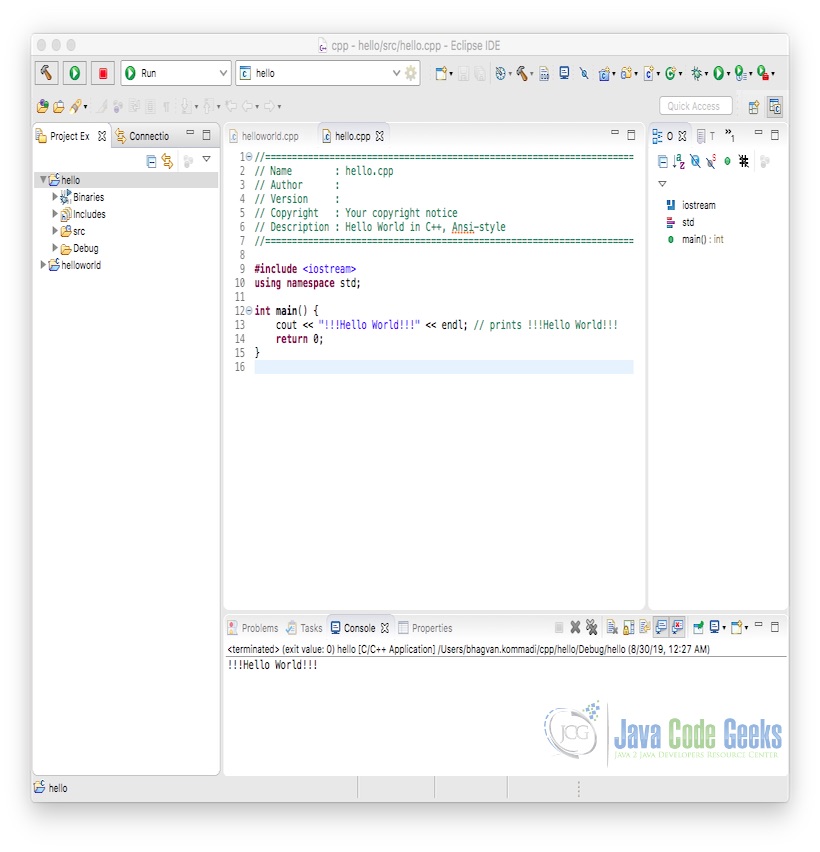
2.6 What is a reference in Java?
In java, Objects can be modified by references. The properties of the object are references. Sample code is shown for a Location
class whose properties are x coordinate xcoord
and y coordinate ycoord
.
Java Reference
/** * */ /** * @author bhagvan.kommadi * */ public class Location { public int xcoord; public int ycoord; public Location(int x, int y) { this.xcoord = x; this.ycoord = y; } public static void setLocations(Location loc1, Location loc2) { loc1.xcoord = 30; loc1.ycoord = 40; Location buf = loc1; loc1 = loc2; loc2 = buf; } /** * @param args */ public static void main(String[] args) { Location loc1 = new Location(20,10); Location loc2 = new Location(10,50); System.out.println("Location 1 X Coordinate: " + loc1.xcoord + " Y Coordinate: " +loc1.ycoord); System.out.println("Location 2 X Coorodinate: " + loc2.xcoord + " Y Coordinate: " +loc2.ycoord); System.out.println(" "); setLocations(loc1,loc2); System.out.println("Location 1 X Coordinate: " + loc1.xcoord + " Y Coordinate: " +loc1.ycoord); System.out.println("Location 2 X Coorodinate: " + loc2.xcoord + " Y Coordinate: " +loc2.ycoord); System.out.println(" "); } }
The Location
class has a constructor to set the x and y coordinates. The static method setLocations
method which sets the properties of loc1
and tries to swap the loc1
and loc2
objects. The references are used to modify the properties. loc1 object will have the properties changed. loc2 does not change after the call on setLocations method.
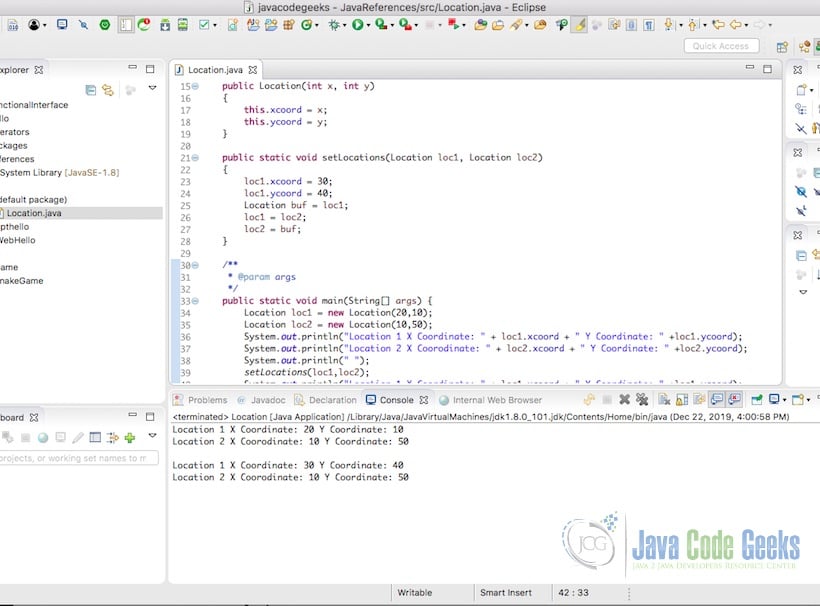
2.7 Comparison between java reference and c++ pointer
In C++, pointers are used for executing tasks and managing the memory dynamically. A pointer to a variable is related to the memory address.’ &’ operator is used for accessing the memory address of the pointer. ‘*’ operator is used for getting the value of the variable at the pointer location.
C++ Pointer
#include using namespace std; int main(int argc, char **argv) { int i; char str[20]; cout << "Address of i - integer variable: "; cout << &i << endl; cout << "Address of str - char array variable: "; cout << &str << endl; return 0; }
The output of the above code when executed in eclipse is shown below:
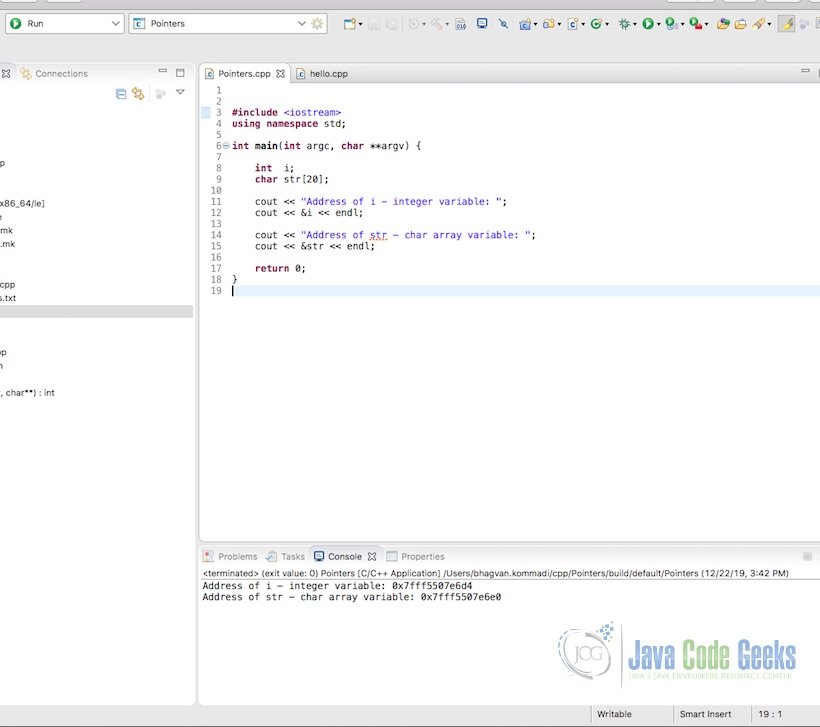
Let us look at another example where you can see pointers and references in C++.
C++ Pointers And Refrences
/* * References.cpp * * Created on: Dec 22, 2019 * Author: bhagvan.kommadi */ #include using namespace std; int main(int argc, char **argv) { int intvar = 30; int *intpointer; intpointer = &intvar; cout << "Value of integer var variable: "; cout << intvar << endl; cout << "Address stored in integer pointer variable: "; cout << intpointer << endl; cout << "Value of *intpointer variable: "; cout << *intpointer << endl; int intval = 30; int& intref = intval; intref = 40; cout << "intval is " << intval << endl ; intval = 50; cout << "intpointer is " << intpointer << endl ; return 0; }
In the above example, you can see the pointer intpointer
defined and set to an integer variable. Similarly, a reference is defined intref
pointing to intval. The output of the above code when executed in eclipse is shown below:
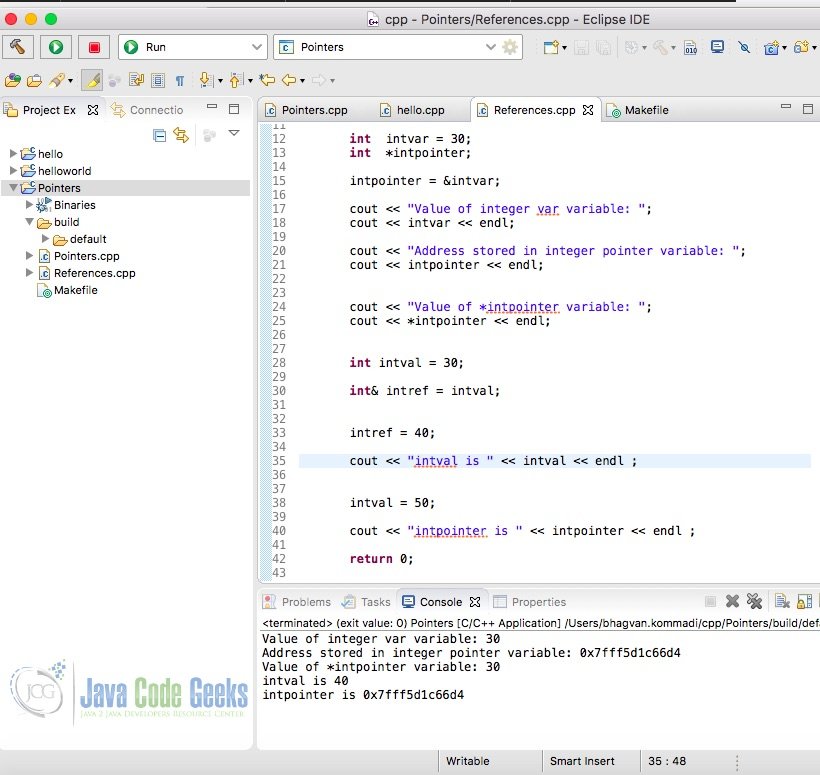
In java, let us look at Flower
object and how references are used to modify the properties of the Flower
. The code example below shows the properties are changed using the references.
Java Reference
/** * */ /** * @author bhagvan.kommadi * */ public class Flower { private String color; public Flower(){} public Flower(String color){ this.color= color; } public String getColor() { return color; } public void setColor(String color) { this.color = color; } private static void setProperties(Flower flower) { flower.setColor("Red"); flower = new Flower("Green"); flower.setColor("Blue"); } public static void swapObjects(Object obj1, Object obj2){ Object buff = obj1; obj1=obj2; obj2=buff; } /** * @param args */ public static void main(String[] args) { Flower rose = new Flower("Red"); Flower iris = new Flower("Blue"); swapObjects(rose, iris); System.out.println("rose color="+rose.getColor()); System.out.println("iris color="+iris.getColor()); setProperties(iris); System.out.println("iris="+iris.getColor()); } }
setProperties
method changes the color
property of the iris flower to Red. SwapObjects
method does not change the flower objects and their properties.
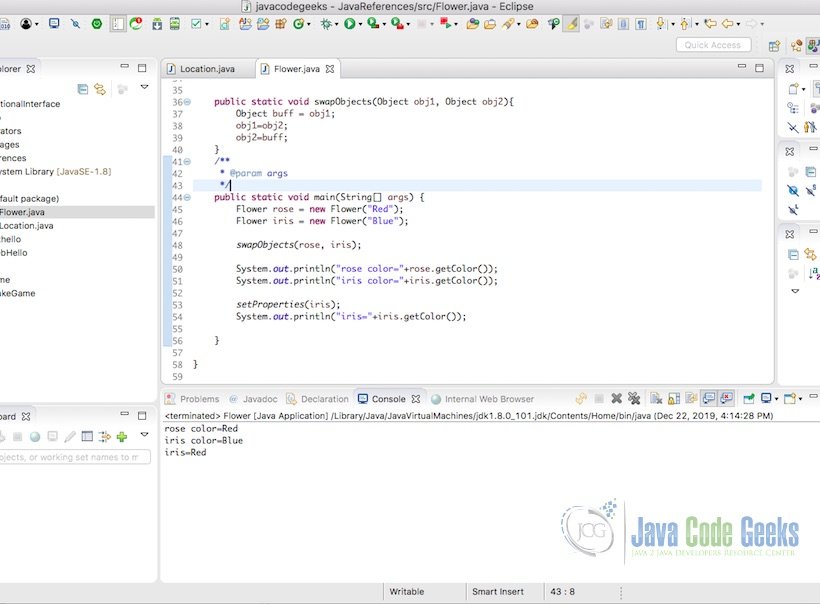
2.8 Example using Java references
In the sections below, different types of references are shown in the examples.
2.8.1 Strong References
Strong references are the default type of References. Objects which are strong references are not candidates for garbage collection. If the object is set to null, it is garbage collected. The code sample below shows how strong references are used.
Strong Reference
public class StrongReference { /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub StrongReference reference = new StrongReference(); reference = null; } }
The output of the above code when executed in eclipse is shown below:
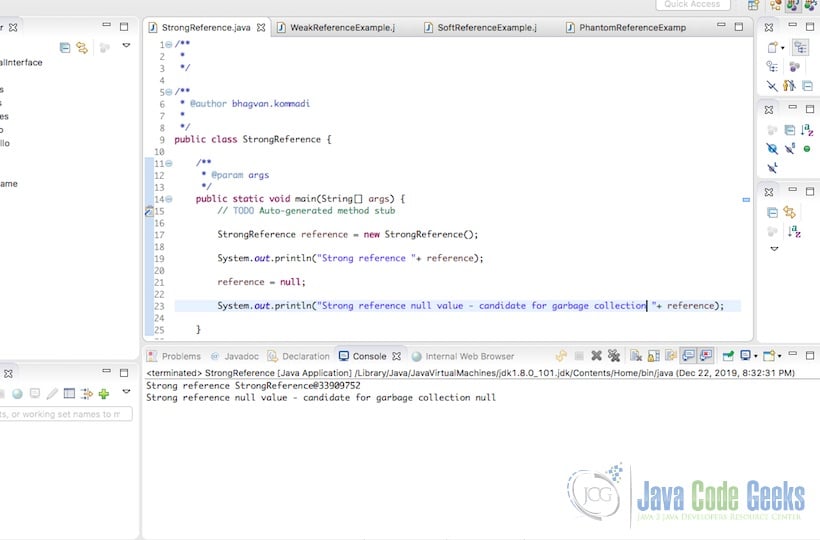
2.8.2 Weak References
Weak references are used in WeakHashMap
for referencing the entries. java.lang.ref.WeakReference
class is used to create this type of reference. Weak objects are marked for garbage collection.
Weak Reference
import java.lang.ref.WeakReference; /** * @author bhagvan.kommadi * */ public class WeakReferenceExample { /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub WeakReferenceExample reference = new WeakReferenceExample(); WeakReference weakReference = new WeakReference(reference); reference = weakReference.get(); } }
The output of the above code when executed in eclipse is shown below:
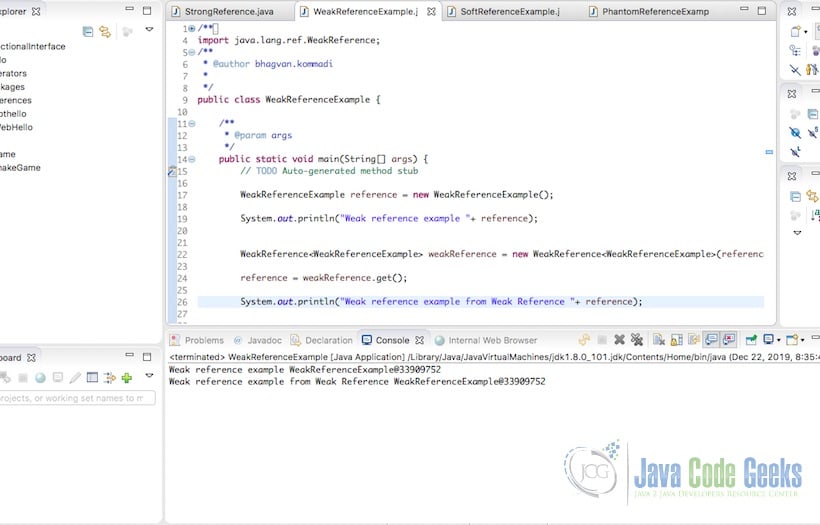
2.8.3 Soft References
java.lang.ref.SoftReference
class is used to create soft references. This type of objects are not garbage collected, even if they are free.
Soft Reference
/** * */ import java.lang.ref.SoftReference; /** * @author bhagvan.kommadi * */ public class SoftReferenceExample { /** * @param args */ public static void main(String[] args) { SoftReferenceExample reference = new SoftReferenceExample(); SoftReference softReference = new SoftReference(reference); reference = softReference.get(); } }
The output of the above code when executed in eclipse is shown below:
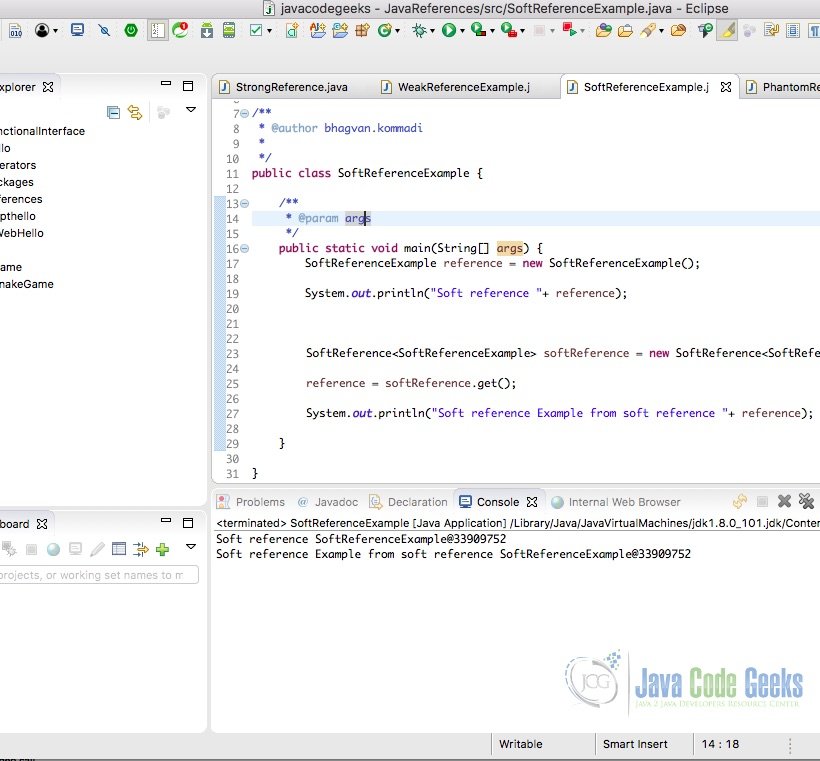
2.8.4 Phantom References
java.lang.ref.PhantomReference
class is used for creating phantom references. They are good candidates for garbage collection.
Phantom Reference
import java.lang.ref.PhantomReference; import java.lang.ref.ReferenceQueue; /** * */ /** * @author bhagvan.kommadi * */ public class PhantomReferenceExample { /** * @param args */ public static void main(String[] args) { PhantomReferenceExample reference = new PhantomReferenceExample(); ReferenceQueue queue = new ReferenceQueue(); PhantomReference phantomReference = new PhantomReference(reference,queue); reference = phantomReference.get(); } }
The output of the above code when executed in eclipse is shown below:
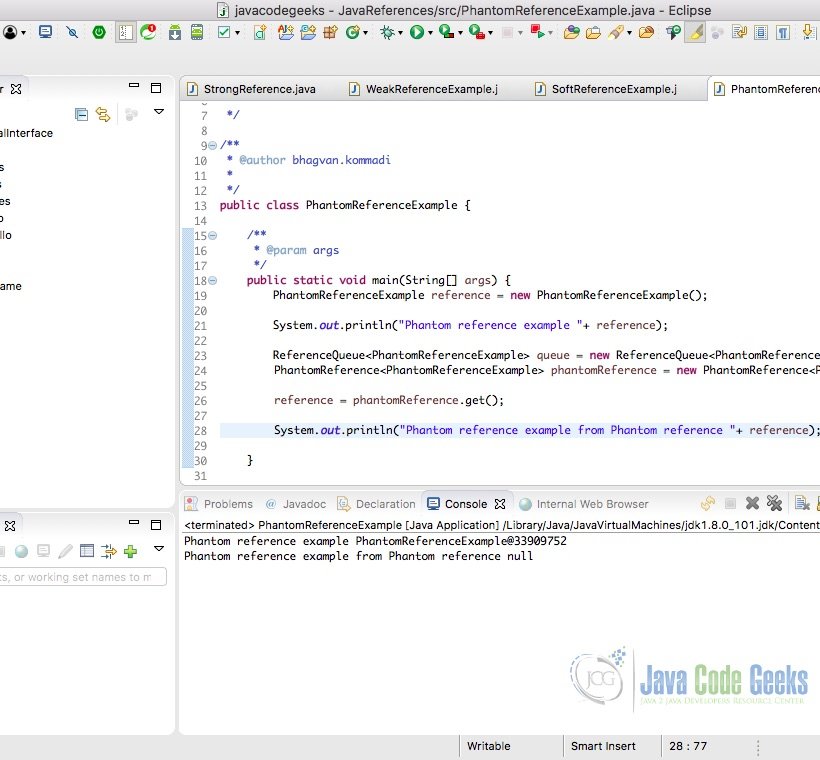
3. Summary
Java references are useful to modify the properties of the class. Java pointers have better benefits compared to C++ pointers. In C++, pointers can be modified which is not a good practice. In Java, references can be pointed to an object or null but cannot be modified. Types can be changed in C++ for pointers. In java, types cannot be changed or reinterpreted. Pointers in C++ might cause issues related to security.
4. More articles
- ArrayList Java Example – How to use ArrayList (with video)
- Java Tutorial for Beginners (with video)
- Java Array – java.util.Arrays Example (with Video)
- LinkedList Java Example (with video)
- Java Stack Example (with video)
- Java Queue Example (with video)
- Java List Example
- Hashmap Java Example (with video)
5. Download the Source Code
You can download the full source code of this example here: Java Pointers (References) Example
Last updated on Aug. 10th, 2021