Gradle Build System Tutorial
In this tutorial, we will see how to use gradle build in detail with examples.
1. Overview
Gradle is a popular build management system. The Gradle framework has features for the automatic download and configuration of the libraries which are required for the build. The library dependencies can be downloaded from Maven and Ivy repositories. Gradle can handle multiple projects and multiple artifact-based builds.
Table Of Contents
- 1. Overview
- 2. Gradle Build System Tutorial
-
- 2.1. Prerequisites
- 2.2. Download
- 2.3. Setup
- 2.4. Running Gradle
- 2.5. Gradle Projects
- 2.6. Gradle Tasks
- 2.7. Gradle Plugins
- 2.8. Gradle Java Project
- 2.9. Gradle Wrapper
- 2.10. Gradle Custom Tasks
- 2.11. Gradle Testing
- 2.12. Gradle Deployment
- 2.13. Gradle Custom Plugins
- 2.14. Gradle Eclipse Integration
- 2.15. Gradle build scans
- 3. Download the Source Code
2. Gradle Build System Tutorial
2.1 Prerequisites
Java 8 is required on the Linux, windows, or Mac operating systems. Gradle 5.4.1 version can be used for building Gradle projects.
2.2 Download
You can download Java 8 from the Oracle web site. Likewise, Gradle 5.4.1 can be downloaded from this website.
2.3 Setup
2.3.1 Java Setup
You can set the environment variables for JAVA_HOME and PATH. They can be set as shown below.
Java Environment Setup
JAVA_HOME="/desktop/jdk1.8.0_73" export JAVA_HOME PATH=$JAVA_HOME/bin:$PATH export PATH
2.3.2 Gradle Setup
The environment variables for Gradle are set as below:
Gradle Setup
GRADLE_HOME="/opt/gradle/gradle-5.4.1/bin" export GRADLE_HOME=$GRADLE_HOME/bin/ export PATH=$PATH:$GRADLE_HOME
2.4 Running Gradle
You can check the version of the Gradle by using the command Gradle –-version. The command for running Gradle is as below:
Command
gradle --version
The output of the executed Gradle command is shown below.
Gradle Version
apples-MacBook-Air:~ bhagvan.kommadi$ gradle --version Welcome to Gradle 5.5.1! Here are the highlights of this release: - Kickstart Gradle plugin development with gradle init - Distribute organization-wide Gradle properties in custom Gradle distributions - Transform dependency artifacts on resolution For more details see https://docs.gradle.org/5.5.1/release-notes.html ------------------------------------------------------------ Gradle 5.5.1 ------------------------------------------------------------ Build time: 2019-07-10 20:38:12 UTC Revision: 3245f748c7061472da4dc184991919810f7935a5 Kotlin: 1.3.31 Groovy: 2.5.4 Ant: Apache Ant(TM) version 1.9.14 compiled on March 12 2019 JVM: 1.8.0_101 (Oracle Corporation 25.101-b13) OS: Mac OS X 10.12.6 x86_64
2.4.1 Gradle Hello World
Let us look at Gradle Hello World. You can create a task as shown below in build.gradle
:
Setup
task helloworld { doLast { println 'Hello World' } }
The command to execute above task is shown below:
Command
gradle helloworld
The output of the command executed is as below:
Command
apples-MacBook-Air:gradlesystem bhagvan.kommadi$ gradle helloworld Starting a Gradle Daemon (subsequent builds will be faster) > Task :helloworld Hello World BUILD SUCCESSFUL in 8s 1 actionable task: 1 executed
2.5 Gradle Projects
Every Gradle project has tasks. A Gradle task is a unit of work to execute a build. The compilation of source code and generation of Javadoc are examples of Gradle tasks. The project name is mentioned in settings.gradle
as shown below.
settings.gradle
rootProject.name ='org.javacodegeeks.gradle.saygreetings'
build.gradle
is written as below:
build.gradle
description =""" Example project for a Gradle build Project name: ${project.name} More detailed information here... """ task saygreetings { doLast { println 'Greetings' } }
The command to execute above task is shown below:
Command
gradle saygreetings
The output of the command executed is as below:
Output
apples-MacBook-Air:project bhagvan.kommadi$ gradle saygreetings > Task :saygreetings Greetings BUILD SUCCESSFUL in 1s 1 actionable task: 1 executed
An enterprise application will have multiple projects to be built. The Gradle framework has a root project which can have multiple sub-projects. build. Gradle file has the root project. The file settings.gradle will have the subprojects information.
For example, you can have the project structure as below:
- base_project
- auth
- usermgmt
- utils
- settings.gradle
Based on the project structure, you can have the settings.gradle.
build.gradle
include 'auth', 'usermgmt', 'utils' #include 'auth' #include 'usermgmt' #include 'utils'
2.6 Gradle Tasks
The Gradle task is used to create tasks such as jar creation and archive publishing. The Gradle framework is extensible. Tasks are the core part of the framework. A Gradle task can be created for moving data from a directory to the other directory. A task can have a dependency on another task. It can have an input and the output. Gradle has introspection related tasks.
For example, the tasks command shows the available tasks for a project. This command shows the base tasks when you do not have a build.gradle
file.
Tasks command
gradle -q tasks
The output of the command executed is as below:
Tasks command output
apples-MacBook-Air:gradlesystem bhagvan.kommadi$ gradle -q tasks ------------------------------------------------------------ Tasks runnable from root project ------------------------------------------------------------ Build Setup tasks ----------------- init - Initializes a new Gradle build. wrapper - Generates Gradle wrapper files. Help tasks ---------- buildEnvironment - Displays all buildscript dependencies declared in root project 'gradlesystem'. components - Displays the components produced by root project 'gradlesystem'. [incubating] dependencies - Displays all dependencies declared in root project 'gradlesystem'. dependencyInsight - Displays the insight into a specific dependency in root project 'gradlesystem'. dependentComponents - Displays the dependent components of components in root project 'gradlesystem'. [incubating] help - Displays a help message. model - Displays the configuration model of root project 'gradlesystem'. [incubating] projects - Displays the sub-projects of root project 'gradlesystem'. properties - Displays the properties of root project 'gradlesystem'. tasks - Displays the tasks runnable from root project 'gradlesystem'. To see all tasks and more detail, run gradle tasks --all To see more detail about a task, run gradle help --task
Gradle has helped the task to give information related to the other tasks such as the init task. The command executed for help task related to init is shown below:
Help for Init task
gradle -q help --task init
The output of the command executed is as below:
Help for Init task output
apples-MacBook-Air:gradlesystem bhagvan.kommadi$ gradle -q help --task init Detailed task information for init Path :init Type InitBuild (org.gradle.buildinit.tasks.InitBuild) Options --dsl Set the build script DSL to be used in generated scripts. Available values are: groovy kotlin --package Set the package for source files. --project-name Set the project name. --test-framework Set the test framework to be used. Available values are: junit junit-jupiter kotlintest scalatest spock testng --type Set the type of project to generate. Available values are: basic cpp-application cpp-library groovy-application groovy-gradle-plugin groovy-library java-application java-gradle-plugin java-library kotlin-application kotlin-gradle-plugin kotlin-library pom scala-library Description Initializes a new Gradle build. Group Build Setup
2.7 Gradle Plugins
Gradle has an extension for a plugin for preconfigured tasks. It has a base set of plugins and developers can add custom plugins. build.gradle file has plugin specified using the statement apply plugin ‘plugin-name’.
You can add the entry apply plugin: 'com.android.application'
which sets the Android plug-in available for a Gradle build. Gradle has a registry of plugins which can be accessed at site.
2.8 Gradle Java Project
The Gradle framework has features for java projects. You can create a new Gradle based Java project for JUnit Jupiter.
build.gradle
gradle init --type java-library --test-framework junit-jupiter
The output of the command executed is as below:
build.gradle
apples-MacBook-Air:gradlesystem bhagvan.kommadi$ gradle init --type java-library --test-framework junit-jupiter Select build script DSL: 1: Groovy 2: Kotlin Enter selection (default: Groovy) [1..2] 1 Project name (default: gradlesystem): junit Source package (default: junit): junit > Task :init Get more help with your project: https://docs.gradle.org/5.5.1/userguide/java_library_plugin.html BUILD SUCCESSFUL in 50s 2 actionable tasks: 2 executed
Java code generated related to the command above is presented below:
Library class
/* * This Java source file was generated by the Gradle 'init' task. */ package junit; public class Library { public boolean someLibraryMethod() { return true; } }
The code generated for Junit Test is shown below:
Junit class
/* * This Java source file was generated by the Gradle 'init' task. */ package junit; import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.*; class LibraryTest { @Test void testSomeLibraryMethod() { Library classUnderTest = new Library(); assertTrue(classUnderTest.someLibraryMethod(), "someLibraryMethod should return 'true'"); } }
You can execute the build using the command below:
build command
gradle build
The output of the command executed is presented below:
build command output
apples-MacBook-Air:junit bhagvan.kommadi$ gradle build BUILD SUCCESSFUL in 31s 4 actionable tasks: 4 up-to-date
The command for executing the junit test is shown below:
build command
gradle test
The output of the command executed is presented below:
build command output
apples-MacBook-Air:junit bhagvan.kommadi$ gradle test BUILD SUCCESSFUL in 35s 3 actionable tasks: 3 up-to-date
2.9 Gradle Wrapper
The Gradle wrapper is used for executing the build with a predefined Gradle version and settings. Gradle version download happens when the Gradle wrapper is executed. The Gradle wrapper can be created using command Gradle wrapper
gradlew
is created for mac and Unix systems. gradlew.batis created for window systems. These files are executed when the Gradle command is executed. Gradle wrapper version can be specified in a Gradle task. When the task is executed, Gradle wrapper is created and it downloads the Gradle based on the version. Gradle wrapper version can be specified as shown below:
Gradle wrapper
wrapper { gradleVersion = '4.9' }
Gradle options can be specified in the gradlew
or gradlew.bat
file.
gradle wrapper options
#!/usr/bin/env bash DEFAULT_JVM_OPTS="-Xmx1024m"
2.10 Gradle Custom Tasks
A gradle custom task can be created and it can be derived from other tasks. For example, CopyTask can be created to copy files.
A copyTask can be created in a build.gradle
file as shown below:
custom task
task copyTask(type: Copy) { from 'src' into 'dest' }
You can create an src folder inside this project and add an example.txt text file to this folder. copy task will copy the example.txt
file to a new dest folder.
The command to execute above task is shown below:
custom task run command
gradle copyTask
The output of the command executed is presented below:
custom task output
apples-MacBook-Air:customtask bhagvan.kommadi$ gradle copyTask BUILD SUCCESSFUL in 4s 1 actionable task: 1 up-to-date
2.11 Gradle Testing
Gradle 6.0 has features for unit testing with Junit 5. you can add dependencies in the build.gradle
file as shown below.
custom task
dependencies { testImplementation(enforcedPlatform("org.junit:junit-bom:5.4.0")) testImplementation("org.junit.jupiter:junit-jupiter") }
Gradle test task helps in finding the compiled classes in the project source folder.
2.12 Gradle Deployment
Gradle provides support for deploying build artifacts to artifact repositories, such as Artifactory or Sonatype Nexus. You can use a maven-publish plugin for publishing build artifacts.
2.13 Gradle Custom Plugins
A gradle custom plugin can be created to have a plugin with custom logic. The build file will have simple and straightforward tasks. The build needs to have declarative logic to have better maintenance.
2.14 Gradle – Eclipse Integration
You can find the source code for installing Gradle Plugin using the Grails project in this javacodegeeks article.
You need to also ensure that the Buildship Gradle Integration plugin is installed. The snapshot below shows the installed Gradle version.
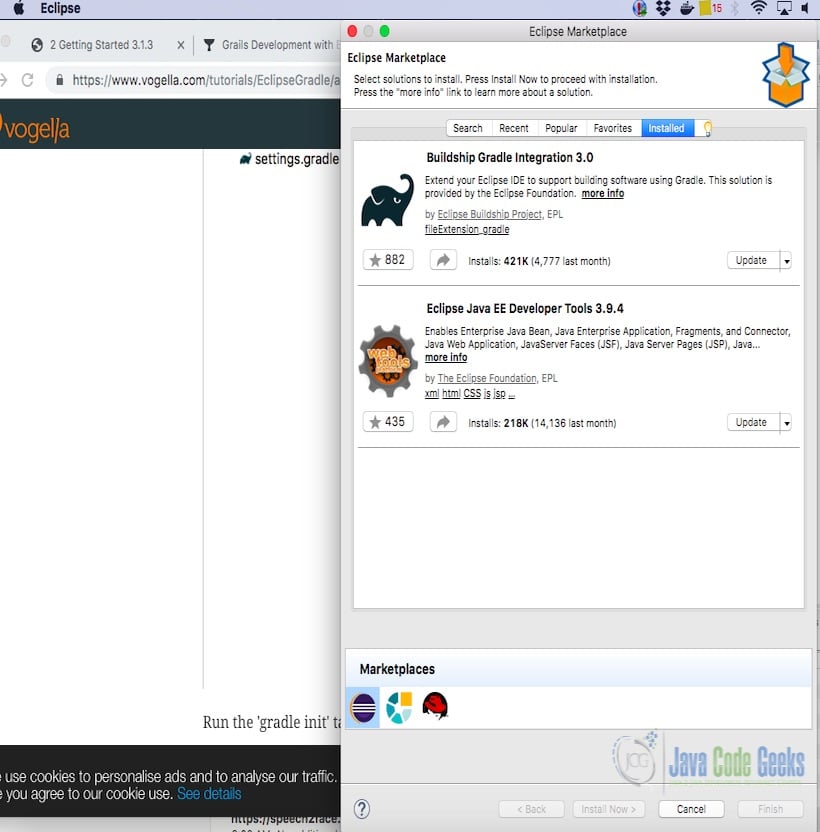
2.14.1 Building with Gradle – Eclipse
You can import the project HelloWorld which was a Gradle project created. The snapshot below shows the import wizard from the Eclipse menu File-> Import.
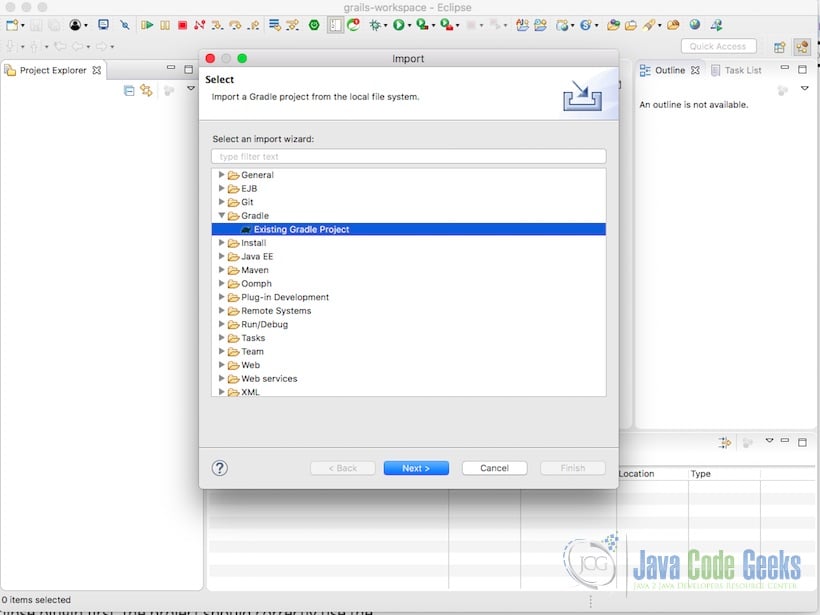
After the import, the Gradle Grails project can be viewed in the eclipse. The screen shot below shows the imported project.
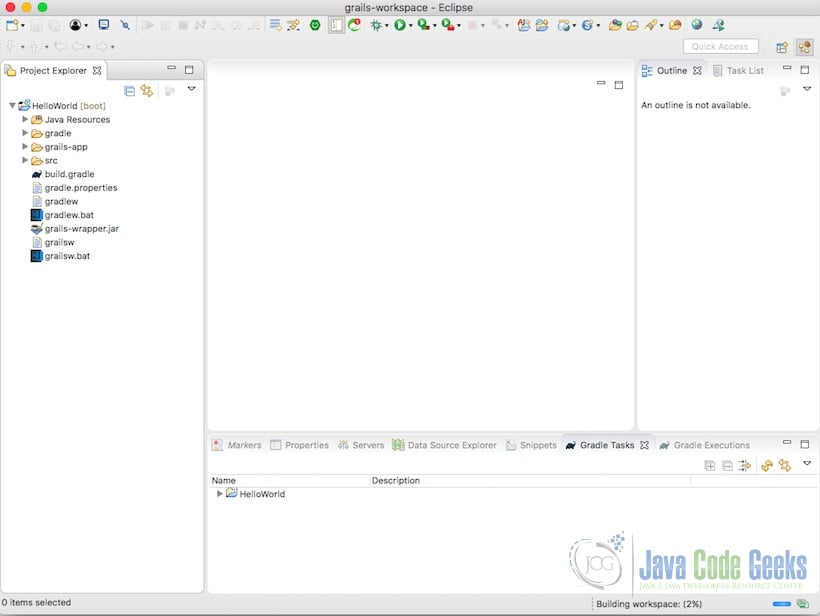
From the Gradle tasks view, You can see all the Gradle tasks. To execute the grails-app, click on bootRun. The screenshot below shows the Gradle tasks view.
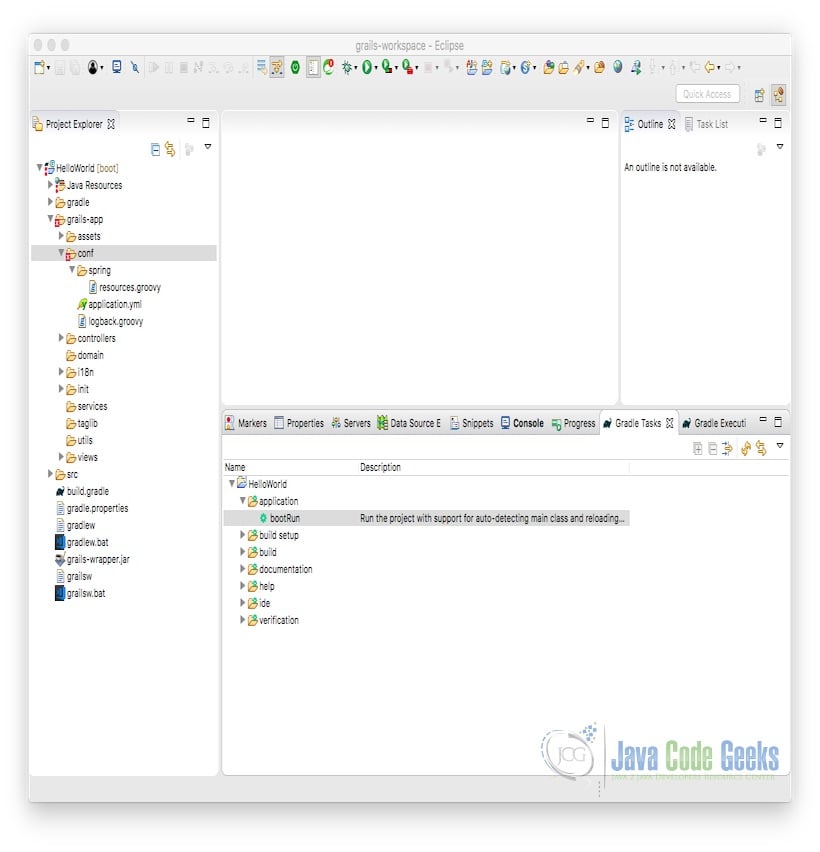
The grails app can be accessed at http://localhost:8080 when the gradle runs the Grails app on eclipse. The snapshot of the Grails app and Gradle task execution is shown below.
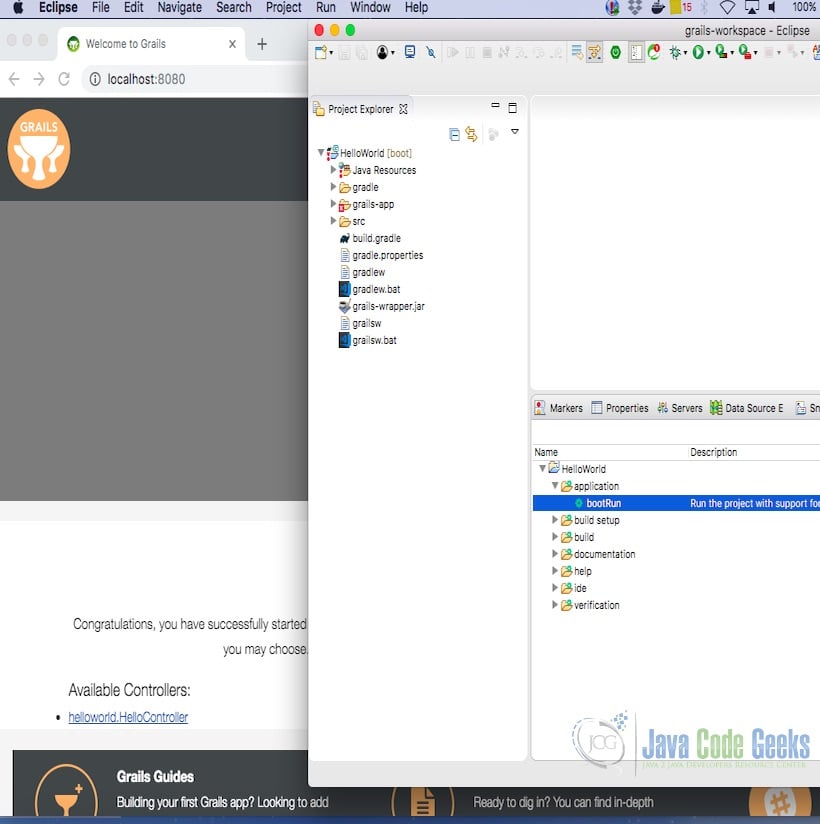
The HelloController
can be accessed and the page renders to show the “Greetings” message. The rendered page is shown below:
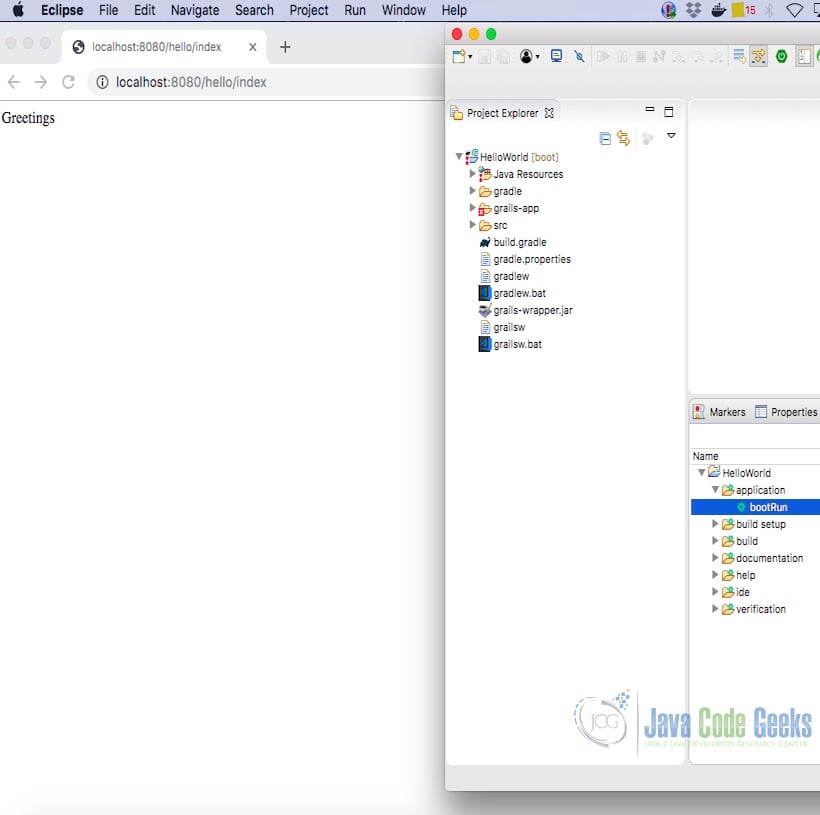
Gradle provides several plugins for analyzing the code base of a Gradle project.
2.15 Gradle Build Scans
The Gradle project can have a build scanner. A build scan has incidents and details of a Gradle build project. The Gradle remote server will have the build scans which are published. Gradle init is used for creating a project. The command is shown below:
gradle project create command
gradle init
You can use build scan option to publish the build scan as shown below:
build scan
gradlew build --scan
The output of the above command executed is shown below:
gradle project create command
apples-MacBook-Air:build_scan bhagvan.kommadi$ ./gradlew build --scan BUILD SUCCESSFUL in 40s 7 actionable tasks: 7 executed Publishing a build scan to scans.gradle.com requires accepting the Gradle Terms of Service defined at https://gradle.com/terms-of-service. Do you accept these terms? [yes, no] yes Gradle Terms of Service accepted. Publishing build scan... https://gradle.com/s/n7m73v5szsjxg
The above command publishes the Gradle project. The scan can be accessed by the link provided in the output. The link takes you to the website as shown below.
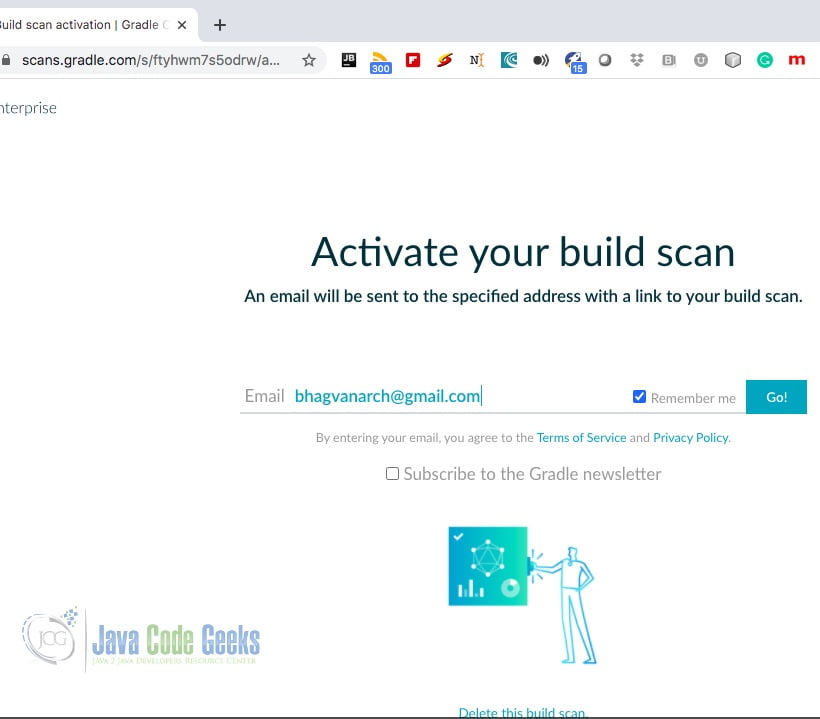
You can type your email address and an email will be sent to you as shown in the message below.
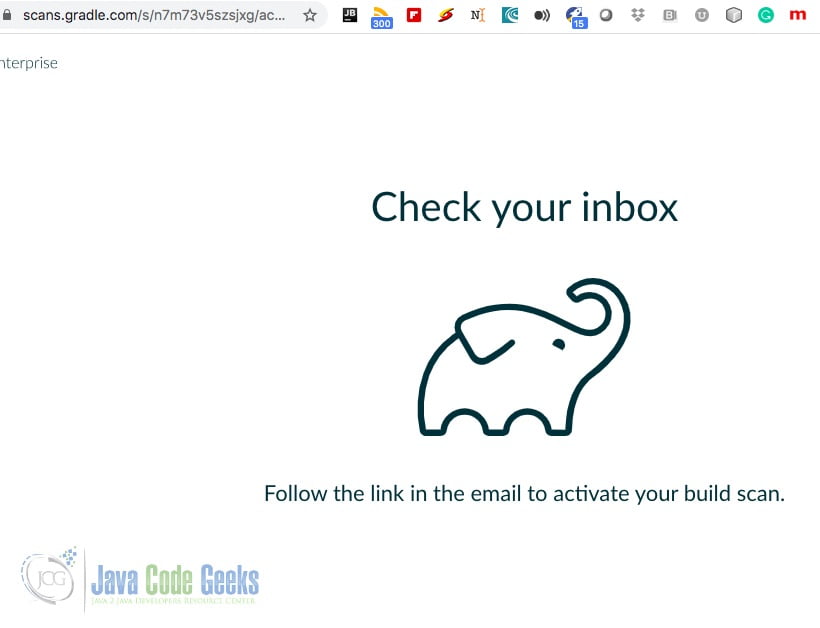
When you click on the link. The email will be like in the picture below.
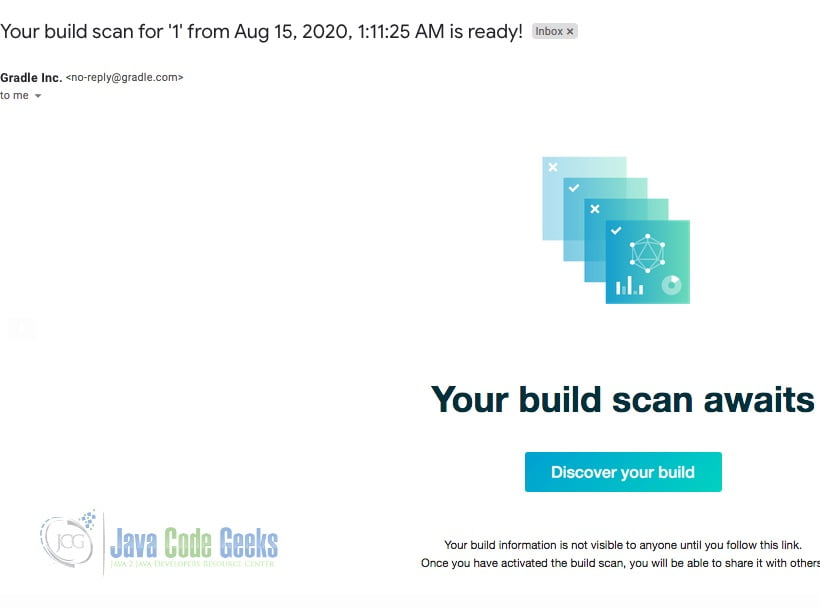
When you click on the build, the link takes you to the build scan.
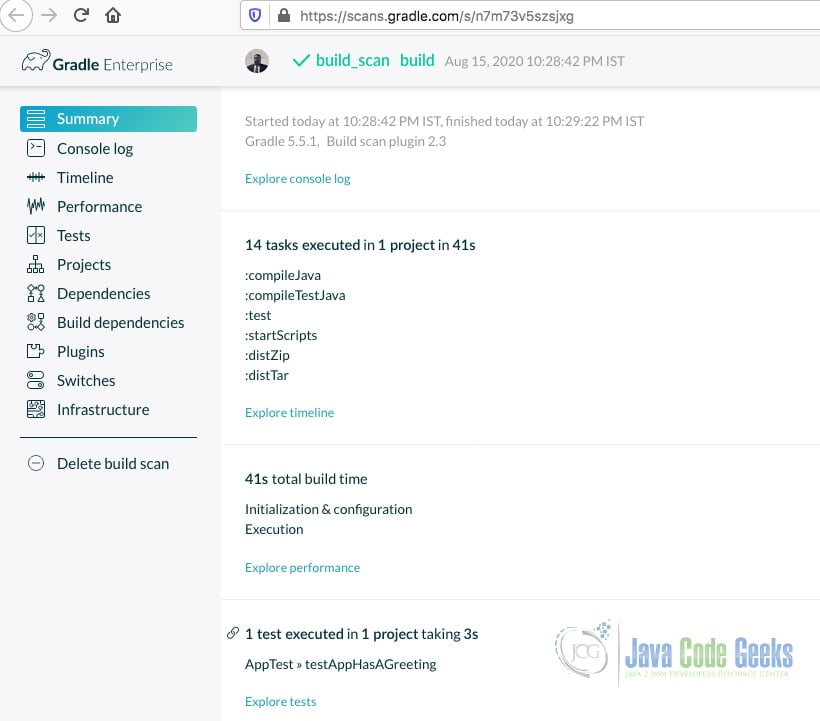
3.Download the Source Code
You can download the full source code of this example here: Gradle Build System Tutorial