fetch API (get method) in React.js
Welcome readers, in this tutorial, we will implement a fetch api (get method) in a react application.
1. Introduction
React is a flexible javascript library responsible for building reusable UI components. It is an open-source component-based frontend library responsible only for the view part of an application and works in a virtual document object model. But before that let us take a look at the differences between angular and react –
Angular | React |
Complete framework | Library |
Object oriented programming | Extension to javascript library and its internal architecture is based on jsx |
Based on model view controller and real document object model | Based on virtual document object model |
To understand a practical implementation of the react library let us go through some hands-on exercises.
1.1 Setting up Node.js
To set up Node.js on windows you will need to download the installer from this link. Click on the installer (also include the NPM package manager) for your platform and run the installer to start with the Node.js setup wizard. Follow the wizard steps and click on Finish when it is done. If everything goes well you can navigate to the command prompt to verify if the installation was successful as shown in Fig. 1.
1.1.1 Setting up reactjs project
Once the Nodejs setup is successful we will use the below command to install the react library and set up the project. Do note that the npx
package is available with the npm 5.2 version and above.
Create project structure
npx create-react-app get-fetch-app
The above command will take some time to install the react library and create a new project named – get-fetch-app
as shown below.
2. fetch API (get method) in react app
To set up the application, we will need to navigate to a path where our project will reside and I will be using Visual Studio Code as my preferred IDE.
2.1 Setting up backend project
To understand the fetch api call (get method) in react application I will be using a separate nodejs application that exposes the get endpoint to fetch the users from the postgresql database. Since the nodejs application is out of the scope of this tutorial hence I am skipping it for brevity. However, you can download the source code from the Downloads section.
2.2 Setting up the react code
2.2.1 Creating the implementation file
Add the below code to the app.js
file responsible to handle the implementation. We will use the fetch(api)
method to hit the backend application endpoint running on the nodejs server and get the data. Once the data is retrieved we will create an html table in the react application to show it to the user. Remove the existing changes from the app.js
file and replace them with the ones below.
App.js
import "./App.css"; import React, { useEffect, useState } from "react"; function App() { // a separate nodejs application is running in backend // get all users endpoint const url = "http://localhost:9500/all"; let [data, setData] = useState([]); // always call api inside useEffect as it is a functional component. useEffect(() => { fetch(url).then((result) => { result.json().then((response) => { setData(response.data); }); }); }, []); // [] helps to run only 1 time. // console.log("api result", data); return ( <div className="App"> <h1>Get API call</h1> <table border="1"> <thead> <tr> <td>Id</td> <td>Name</td> <td>Email</td> <td>Age</td> </tr> </thead> <tbody> {data.map((item, i) => ( <tr key={i}> <td>{item.id}</td> <td>{item.name}</td> <td>{item.email}</td> <td>{item.age}</td> </tr> ))} </tbody> </table> </div> ); } export default App;
3. Run the setup
To run the application navigate to the project directory and enter the following command as shown below in the terminal.
Run command
$ npm run start
The application will be started on the default port. In case the application does not get started on the default port you can change the port number as per your requirement. I have changed the default port to 2000
.
4. Demo
The application will be started in the default browser as shown below and will hit the backend endpoint to get the user details and display the below.
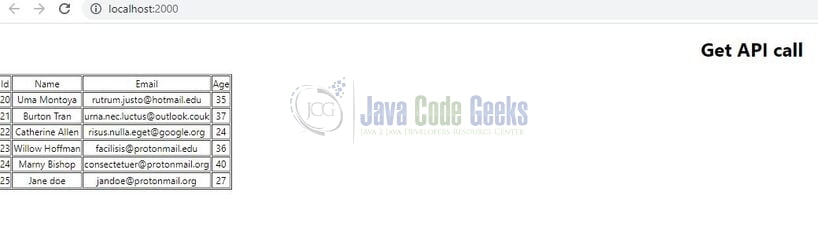
If the table is empty check your backend application as it may not be running or the code to fetch the users from the database might have thrown some error. That is all for this tutorial and I hope the article served you with whatever you were looking for. Happy Learning and do not forget to share!
5. Summary
In this tutorial, we created a react application and understood the fetch api call to get the data from the backend application. You can download the source code from the Downloads section.
6. Download the Project
This was a tutorial to understand fetch api in a react application.
You can download the full source code of this example here: fetch API (get method) in React.js