Java ArrayList Example – How to use ArrayList (with video)
In this example, we will show how to use ArrayList in Java.
The class java.util.ArrayList provides a resizable array, which means that items can be added and removed from the list by using the provided ArrayList methods. It implements the List
interface.
A major question related to arraylists is about when to use them instead of arrays and vice versa. An ArrayList
is a dynamic data structure so it can be used when there is no upper bound on the number of elements.
From the other side, a simple Array
in java is a static data structure, because the initial size of an array cannot be changed, so it can be used only when the data has a known number of elements.
You can also check this tutorial in the following video:
1. ArrayList Java Constructors
The ArrayList
class supports three constructors.
Arraylist()
This constructor builds an empty list.ArrayList(Collection<? extends E> c)
This constructor creates a list containing the elements of the specified collection. Note that E is the notation for the type of an element in a collection.ArrayList(int initialCapacity)
This constructor creates an empty list with the specified initial capacity.
For example, if you want to create an empty array list of Strings then you would do the following:
ArrayList<String> list = new ArrayList<String>();
If you want to create an array list with initial capacity, then you should do the following:
ArrayList<Integer> list = new ArrayList<Integer>(7);
Note: ArrayList
class supports only object types and not primitive types.
2. ArrayList methods
Here are some of the most useful ArrayList methods:
- Adding elements to the list
boolean add(Element e)
Adds the specified element to the end of this list.void add(int index, Element e)
Adds the specified element at the specified position in the list.
- Removing elements from the list
void clear()
Removes all the elements from the list.E remove(int index)
Removes the element at the specified position in the list.protected void removeRange(int start, int end)
Removes from the list all the elements starting from indexstart
(included) until indexend
(not included).
- Getting elements from the list
E get(int index)
Returns the element at the specified position.Object[] toArray()
Returns an array containing all the elements of the list in proper sequence.
- Setting an element
E set(int index, E element)
Replaces the element at the specified position with the specified element.
- Searching elements
boolean contains(Object o)
Returns true if the specified element is found in the list.int indexOf(Object o)
Returns the index of the first occurrence of the specified element in the list. If this element is not in the list, the method returns -1.int lastIndexOf(Object o)
Returns the index of the last occurrence of the specified element in the list. If this element is not in the list, the method returns -1.
- Iterating the arraylist
Iterator iterator()
Returns an iterator over the elements in the list.ListIterator listIterator()
Returns a list iterator over the elements in this list.
- Checking if the list is empty
boolean isEmpty()
Returns true if the list does not contain any element.
- Getting the size of the list
int size()
Returns the length of the list (the number of elements contained in the list).
Those were the most commonly used ArrayList methods. For further details for each method or for other methods that are not mentioned in this section, you can have a look at the official Java API.
3. Examples of using ArrayList in Java
ArrayList, LinkedList, Vector, and Stack implement the List interface.
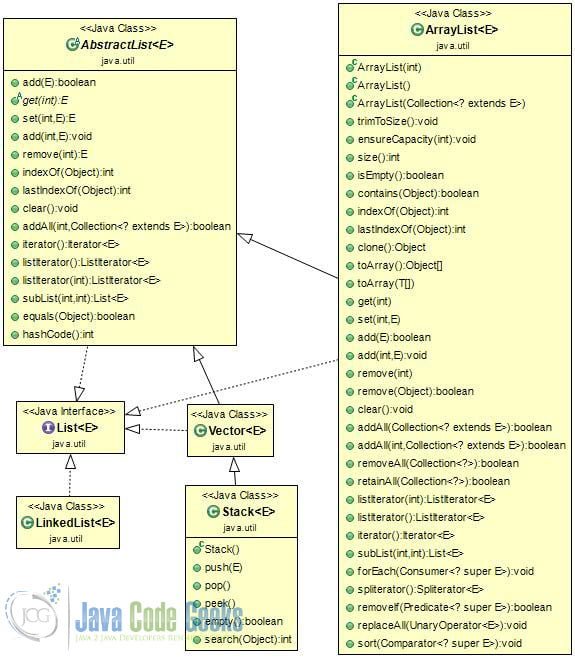
In this step, I will create five Junit test classes to show the usages of ArrayList
as well as LinkedList
, Vector
, and Stack
.
I will include both Junit
and Logback
libraries in the pom.xml
file.
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>jcg.zheng.demo</groupId> <artifactId>java-arraylist-demo</artifactId> <version>0.0.1-SNAPSHOT</version> <build> <sourceDirectory>src</sourceDirectory> <plugins> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.0</version> <configuration> <release>11</release> </configuration> </plugin> </plugins> </build> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> </dependency> <dependency> <groupId>ch.qos.logback</groupId> <artifactId>logback-access</artifactId> <version>1.2.3</version> </dependency> <dependency> <groupId>ch.qos.logback</groupId> <artifactId>logback-classic</artifactId> <version>1.2.3</version> </dependency> <dependency> <groupId>ch.qos.logback</groupId> <artifactId>logback-core</artifactId> <version>1.2.3</version> </dependency> </dependencies> </project>
3.1 ListBaseTest
Create a JUnit class named ListBaseTest.java
with the following code:
ListBaseTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertFalse; import static org.junit.Assert.assertTrue; import java.util.Arrays; import java.util.Iterator; import java.util.List; import org.junit.FixMethodOrder; import org.junit.Test; import org.junit.runners.MethodSorters; import org.slf4j.Logger; @FixMethodOrder(MethodSorters.NAME_ASCENDING) public abstract class ListBaseTest { protected Logger logger; List<String> list; @Test public void test_add() { // Adding items to arrayList list.add("new Item"); assertEquals(5, list.size()); // Display the contents of the array list logger.info("The arraylist contains the following elements: " + list); } @Test public void test_contains() { // Checking if an element is included to the list assertTrue(list.contains("Item1")); assertFalse(list.contains("Item5")); } @Test public void test_get() { assertEquals("Item1", list.get(0)); assertEquals("Item2", list.get(1)); assertEquals("Item3", list.get(2)); assertEquals("Item4", list.get(3)); } @Test public void test_getSize() { // Getting the size of the list assertEquals(4, list.size()); } @Test public void test_indexOf() { // Checking index of an item int pos = list.indexOf("Item2"); assertEquals(1, pos); } @Test public void test_isEmpty() { // Checking if array list is empty assertFalse(list.isEmpty()); } @Test public void test_loop_arraylist_via_for() { // Retrieve elements from the arraylist via foreach logger.info("Retrieving items using foreach loop"); for (String str : list) { logger.info("Item is: " + str); } } @Test public void test_loop_arraylist_via_for_2() { // Retrieve elements from the arraylist via loop using index and size list logger.info("Retrieving items with loop using index and size list"); for (int i = 0; i < list.size(); i++) { logger.info("Index: " + i + " - Item: " + list.get(i)); } } @Test public void test_loop_arrayList_via_foreach() { logger.info("Retrieving items using Java 8 Stream"); list.forEach((item) -> { logger.info(item); }); } @Test public void test_loop_arraylist_via_iterator_for() { // hasNext(): returns true if there are more elements // next(): returns the next element logger.info("Retrieving items using iterator"); for (Iterator<String> it = list.iterator(); it.hasNext();) { logger.info("Item is: " + it.next()); } } @Test public void test_loop_arraylist_via_iterator_while() { Iterator<String> it = list.iterator(); logger.info("Retrieving items using iterator"); while (it.hasNext()) { logger.info("Item is: " + it.next()); } } @Test public void test_remove() { // removing the item in index 0 list.remove(0); assertEquals(3, list.size()); assertEquals("Item2", list.get(0)); assertEquals("Item3", list.get(1)); assertEquals("Item4", list.get(2)); // removing the first occurrence of item "Item3" list.remove("Item3"); assertEquals(2, list.size()); assertEquals("Item2", list.get(0)); assertEquals("Item4", list.get(1)); } @Test public void test_replace() { // Replacing an element list.set(1, "NewItem"); assertEquals("Item1", list.get(0)); assertEquals("NewItem", list.get(1)); assertEquals("Item3", list.get(2)); assertEquals("Item4", list.get(3)); } @Test public void test_toArray() { // Converting ArrayList to Array String[] simpleArray = list.toArray(new String[list.size()]); logger.info("The array created after the conversion of our arraylist is: " + Arrays.toString(simpleArray)); } }
In the above code, we can see that many List
usage cases are covered. Adding elements to the list using two different methods, removing elements, getting the size of the list, checking if the list is empty, checking if a specific element is contained to the list. Also, five different ways are presented for retrieving the elements of a list. Finally, we show how to convert an List
to Array
.
3.2 ArrayListTest
Create a JUnit class named ArrayListTest.java
.
ArrayListTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.ArrayList; import org.junit.Before; import org.slf4j.LoggerFactory; public class ArrayListTest extends ListBaseTest { @Before public void setup_list_with_4_items() { logger = LoggerFactory.getLogger(this.getClass()); // Creating an empty array list list = new ArrayList<String>(); assertTrue(list.isEmpty()); // Adding items to arrayList list.add("Item1"); assertEquals(1, list.size()); list.add("Item2"); assertEquals(2, list.size()); list.add(2, "Item3"); // add Item3 to the third position of array list assertEquals(3, list.size()); list.add("Item4"); assertEquals(4, list.size()); } }
Execute mvn test -Dtest=ArrayListTest
and capture output.
Output
Running jcg.zheng.demo.ArrayListTest 16:01:02.294 [main] INFO jcg.zheng.demo.ArrayListTest - The arraylist contains the following elements: [Item1, Item2, Item3, Item4, new Item] 16:01:02.308 [main] INFO jcg.zheng.demo.ArrayListTest - Retrieving items using Java 8 Stream 16:01:02.310 [main] INFO jcg.zheng.demo.ArrayListTest - Item1 16:01:02.310 [main] INFO jcg.zheng.demo.ArrayListTest - Item2 16:01:02.310 [main] INFO jcg.zheng.demo.ArrayListTest - Item3 16:01:02.310 [main] INFO jcg.zheng.demo.ArrayListTest - Item4 16:01:02.311 [main] INFO jcg.zheng.demo.ArrayListTest - Retrieving items using foreach loop 16:01:02.315 [main] INFO jcg.zheng.demo.ArrayListTest - Item is: Item1 16:01:02.315 [main] INFO jcg.zheng.demo.ArrayListTest - Item is: Item2 16:01:02.315 [main] INFO jcg.zheng.demo.ArrayListTest - Item is: Item3 16:01:02.315 [main] INFO jcg.zheng.demo.ArrayListTest - Item is: Item4 16:01:02.318 [main] INFO jcg.zheng.demo.ArrayListTest - Retrieving items with loop using index and size list 16:01:02.352 [main] INFO jcg.zheng.demo.ArrayListTest - Index: 0 - Item: Item1 16:01:02.352 [main] INFO jcg.zheng.demo.ArrayListTest - Index: 1 - Item: Item2 16:01:02.352 [main] INFO jcg.zheng.demo.ArrayListTest - Index: 2 - Item: Item3 16:01:02.352 [main] INFO jcg.zheng.demo.ArrayListTest - Index: 3 - Item: Item4 16:01:02.353 [main] INFO jcg.zheng.demo.ArrayListTest - Retrieving items using iterator 16:01:02.354 [main] INFO jcg.zheng.demo.ArrayListTest - Item is: Item1 16:01:02.354 [main] INFO jcg.zheng.demo.ArrayListTest - Item is: Item2 16:01:02.354 [main] INFO jcg.zheng.demo.ArrayListTest - Item is: Item3 16:01:02.354 [main] INFO jcg.zheng.demo.ArrayListTest - Item is: Item4 16:01:02.356 [main] INFO jcg.zheng.demo.ArrayListTest - Retrieving items using iterator 16:01:02.357 [main] INFO jcg.zheng.demo.ArrayListTest - Item is: Item1 16:01:02.358 [main] INFO jcg.zheng.demo.ArrayListTest - Item is: Item2 16:01:02.358 [main] INFO jcg.zheng.demo.ArrayListTest - Item is: Item3 16:01:02.358 [main] INFO jcg.zheng.demo.ArrayListTest - Item is: Item4 16:01:02.363 [main] INFO jcg.zheng.demo.ArrayListTest - The array created after the conversion of our arraylist is: [Item1, Item2, Item3, Item4] Tests run: 14, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.486 sec Results : Tests run: 14, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 11.663 s [INFO] Finished at: 2019-07-26T16:01:02-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\java-arraylist-demo>mvn test -Dtest=ArrayListTest
As we see in the output, the results are complied with what we described in the previous section.
3.3 LinkedListTest
Create a JUnit class named LinkedListTest.java
.
LinkedListTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.LinkedList; import org.junit.Before; import org.slf4j.LoggerFactory; public class LinkedListTest extends ListBaseTest { @Before public void setup_list_with_4_items() { logger = LoggerFactory.getLogger(this.getClass()); // Creating an empty linked list list = new LinkedList<String>(); assertTrue(list.isEmpty()); // Adding items to arrayList list.add("Item1"); assertEquals(1, list.size()); list.add("Item2"); assertEquals(2, list.size()); list.add(2, "Item3"); // add Item3 to the third position of array list assertEquals(3, list.size()); list.add("Item4"); assertEquals(4, list.size()); } }
Execute mvn test -Dtest=LinkedListTest
and capture output.
Output
Running jcg.zheng.demo.LinkedListTest 16:03:20.954 [main] INFO jcg.zheng.demo.LinkedListTest - The arraylist contains the following elements: [Item1, Item2, Item3, Item4, new Item] 16:03:20.967 [main] INFO jcg.zheng.demo.LinkedListTest - Retrieving items using Java 8 Stream 16:03:20.968 [main] INFO jcg.zheng.demo.LinkedListTest - Item1 16:03:20.968 [main] INFO jcg.zheng.demo.LinkedListTest - Item2 16:03:20.968 [main] INFO jcg.zheng.demo.LinkedListTest - Item3 16:03:20.969 [main] INFO jcg.zheng.demo.LinkedListTest - Item4 16:03:20.969 [main] INFO jcg.zheng.demo.LinkedListTest - Retrieving items using foreach loop 16:03:20.970 [main] INFO jcg.zheng.demo.LinkedListTest - Item is: Item1 16:03:20.971 [main] INFO jcg.zheng.demo.LinkedListTest - Item is: Item2 16:03:20.971 [main] INFO jcg.zheng.demo.LinkedListTest - Item is: Item3 16:03:20.971 [main] INFO jcg.zheng.demo.LinkedListTest - Item is: Item4 16:03:20.976 [main] INFO jcg.zheng.demo.LinkedListTest - Retrieving items with loop using index and size list 16:03:21.015 [main] INFO jcg.zheng.demo.LinkedListTest - Index: 0 - Item: Item1 16:03:21.015 [main] INFO jcg.zheng.demo.LinkedListTest - Index: 1 - Item: Item2 16:03:21.015 [main] INFO jcg.zheng.demo.LinkedListTest - Index: 2 - Item: Item3 16:03:21.016 [main] INFO jcg.zheng.demo.LinkedListTest - Index: 3 - Item: Item4 16:03:21.017 [main] INFO jcg.zheng.demo.LinkedListTest - Retrieving items using iterator 16:03:21.017 [main] INFO jcg.zheng.demo.LinkedListTest - Item is: Item1 16:03:21.018 [main] INFO jcg.zheng.demo.LinkedListTest - Item is: Item2 16:03:21.018 [main] INFO jcg.zheng.demo.LinkedListTest - Item is: Item3 16:03:21.018 [main] INFO jcg.zheng.demo.LinkedListTest - Item is: Item4 16:03:21.020 [main] INFO jcg.zheng.demo.LinkedListTest - Retrieving items using iterator 16:03:21.022 [main] INFO jcg.zheng.demo.LinkedListTest - Item is: Item1 16:03:21.022 [main] INFO jcg.zheng.demo.LinkedListTest - Item is: Item2 16:03:21.022 [main] INFO jcg.zheng.demo.LinkedListTest - Item is: Item3 16:03:21.022 [main] INFO jcg.zheng.demo.LinkedListTest - Item is: Item4 16:03:21.026 [main] INFO jcg.zheng.demo.LinkedListTest - The array created after the conversion of our arraylist is: [Item1, Item2, Item3, Item4] Tests run: 14, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.492 sec Results : Tests run: 14, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 10.188 s [INFO] Finished at: 2019-07-26T16:03:21-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\java-arraylist-demo>mvn test -Dtest=LinkedListTest
3.4 VectorTest
Create a JUnit class named VectorTest.java
.
VectorTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.Vector; import org.junit.Before; import org.slf4j.LoggerFactory; public class VectorTest extends ListBaseTest { @Before public void setup_list_with_4_items() { logger = LoggerFactory.getLogger(this.getClass()); // Creating an empty vector list = new Vector<String>(); assertTrue(list.isEmpty()); // Adding items to arrayList list.add("Item1"); assertEquals(1, list.size()); list.add("Item2"); assertEquals(2, list.size()); list.add(2, "Item3"); // add Item3 to the third position of array list assertEquals(3, list.size()); list.add("Item4"); assertEquals(4, list.size()); } }
Execute mvn test -Dtest=VectorTest
and capture output.
Output
Running jcg.zheng.demo.VectorTest 16:05:02.444 [main] INFO jcg.zheng.demo.VectorTest - The arraylist contains the following elements: [Item1, Item2, Item3, Item4, new Item] 16:05:02.459 [main] INFO jcg.zheng.demo.VectorTest - Retrieving items using Java 8 Stream 16:05:02.465 [main] INFO jcg.zheng.demo.VectorTest - Item1 16:05:02.466 [main] INFO jcg.zheng.demo.VectorTest - Item2 16:05:02.466 [main] INFO jcg.zheng.demo.VectorTest - Item3 16:05:02.467 [main] INFO jcg.zheng.demo.VectorTest - Item4 16:05:02.469 [main] INFO jcg.zheng.demo.VectorTest - Retrieving items using foreach loop 16:05:02.470 [main] INFO jcg.zheng.demo.VectorTest - Item is: Item1 16:05:02.470 [main] INFO jcg.zheng.demo.VectorTest - Item is: Item2 16:05:02.470 [main] INFO jcg.zheng.demo.VectorTest - Item is: Item3 16:05:02.471 [main] INFO jcg.zheng.demo.VectorTest - Item is: Item4 16:05:02.477 [main] INFO jcg.zheng.demo.VectorTest - Retrieving items with loop using index and size list 16:05:02.527 [main] INFO jcg.zheng.demo.VectorTest - Index: 0 - Item: Item1 16:05:02.527 [main] INFO jcg.zheng.demo.VectorTest - Index: 1 - Item: Item2 16:05:02.528 [main] INFO jcg.zheng.demo.VectorTest - Index: 2 - Item: Item3 16:05:02.528 [main] INFO jcg.zheng.demo.VectorTest - Index: 3 - Item: Item4 16:05:02.529 [main] INFO jcg.zheng.demo.VectorTest - Retrieving items using iterator 16:05:02.530 [main] INFO jcg.zheng.demo.VectorTest - Item is: Item1 16:05:02.531 [main] INFO jcg.zheng.demo.VectorTest - Item is: Item2 16:05:02.531 [main] INFO jcg.zheng.demo.VectorTest - Item is: Item3 16:05:02.531 [main] INFO jcg.zheng.demo.VectorTest - Item is: Item4 16:05:02.532 [main] INFO jcg.zheng.demo.VectorTest - Retrieving items using iterator 16:05:02.533 [main] INFO jcg.zheng.demo.VectorTest - Item is: Item1 16:05:02.534 [main] INFO jcg.zheng.demo.VectorTest - Item is: Item2 16:05:02.534 [main] INFO jcg.zheng.demo.VectorTest - Item is: Item3 16:05:02.534 [main] INFO jcg.zheng.demo.VectorTest - Item is: Item4 16:05:02.537 [main] INFO jcg.zheng.demo.VectorTest - The array created after the conversion of our arraylist is: [Item1, Item2, Item3, Item4] Tests run: 14, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.632 sec Results : Tests run: 14, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 10.325 s [INFO] Finished at: 2019-07-26T16:05:02-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\java-arraylist-demo>mvn test -Dtest=VectorTest
3.5 StackTest
Create a JUnit class named StackTest.java
.
StackTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.Stack; import org.junit.Before; import org.junit.Test; import org.slf4j.LoggerFactory; public class StackTest extends ListBaseTest { @Before public void setup_list_with_4_items() { logger = LoggerFactory.getLogger(this.getClass()); // Creating an empty vector list = new Stack<String>(); assertTrue(list.isEmpty()); // Adding items to arrayList list.add("Item1"); assertEquals(1, list.size()); list.add("Item2"); assertEquals(2, list.size()); list.add(2, "Item3"); // add Item3 to the third position of array list assertEquals(3, list.size()); list.add("Item4"); assertEquals(4, list.size()); } @Test public void test_pop() { String item = ((Stack<String>) list).pop(); assertEquals("Item4", item); } @Test public void test_push() { ((Stack<String>) list).push("newValue"); assertEquals(5, list.size()); } }
Execute mvn test -Dtest=StackTest
and capture output.
Output
Running jcg.zheng.demo.StackTest 16:06:05.112 [main] INFO jcg.zheng.demo.StackTest - The arraylist contains the following elements: [Item1, Item2, Item3, Item4, new Item] 16:06:05.125 [main] INFO jcg.zheng.demo.StackTest - Retrieving items using Java 8 Stream 16:06:05.127 [main] INFO jcg.zheng.demo.StackTest - Item1 16:06:05.127 [main] INFO jcg.zheng.demo.StackTest - Item2 16:06:05.127 [main] INFO jcg.zheng.demo.StackTest - Item3 16:06:05.127 [main] INFO jcg.zheng.demo.StackTest - Item4 16:06:05.128 [main] INFO jcg.zheng.demo.StackTest - Retrieving items using foreach loop 16:06:05.129 [main] INFO jcg.zheng.demo.StackTest - Item is: Item1 16:06:05.129 [main] INFO jcg.zheng.demo.StackTest - Item is: Item2 16:06:05.129 [main] INFO jcg.zheng.demo.StackTest - Item is: Item3 16:06:05.129 [main] INFO jcg.zheng.demo.StackTest - Item is: Item4 16:06:05.130 [main] INFO jcg.zheng.demo.StackTest - Retrieving items with loop using index and size list 16:06:05.185 [main] INFO jcg.zheng.demo.StackTest - Index: 0 - Item: Item1 16:06:05.185 [main] INFO jcg.zheng.demo.StackTest - Index: 1 - Item: Item2 16:06:05.186 [main] INFO jcg.zheng.demo.StackTest - Index: 2 - Item: Item3 16:06:05.187 [main] INFO jcg.zheng.demo.StackTest - Index: 3 - Item: Item4 16:06:05.191 [main] INFO jcg.zheng.demo.StackTest - Retrieving items using iterator 16:06:05.195 [main] INFO jcg.zheng.demo.StackTest - Item is: Item1 16:06:05.197 [main] INFO jcg.zheng.demo.StackTest - Item is: Item2 16:06:05.198 [main] INFO jcg.zheng.demo.StackTest - Item is: Item3 16:06:05.198 [main] INFO jcg.zheng.demo.StackTest - Item is: Item4 16:06:05.200 [main] INFO jcg.zheng.demo.StackTest - Retrieving items using iterator 16:06:05.201 [main] INFO jcg.zheng.demo.StackTest - Item is: Item1 16:06:05.202 [main] INFO jcg.zheng.demo.StackTest - Item is: Item2 16:06:05.202 [main] INFO jcg.zheng.demo.StackTest - Item is: Item3 16:06:05.202 [main] INFO jcg.zheng.demo.StackTest - Item is: Item4 16:06:05.213 [main] INFO jcg.zheng.demo.StackTest - The array created after the conversion of our arraylist is: [Item1, Item2, Item3, Item4] Tests run: 16, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.612 sec Results : Tests run: 16, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 10.466 s [INFO] Finished at: 2019-07-26T16:06:05-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\java-arraylist-demo>mvn test -Dtest=StackTest
4. Summary
ArrayList
is part of Java’s collection framework and implements Java’s List
interface. ArrayList
is used to store a dynamically sized collection of elements. Vector and ArrayList both use Array internally as a data structure. The main difference is that Vector
‘s methods are synchronized and ArrayList
‘s methods are not synchronized. Click here for more details.
The performance differences between ArrayList
and LinkedList
are as the following:
Performance | remove(int idx) | get(int idx) | add(E ele) | Iterator.remove() | Iterator.add(E ele) |
ArrayList | O(n) | O(1) | O(1) | O(n) | O(n) |
LinkedList | O(n) | O(n) |
O(1) when index=0 Else O(n) |
O(1) | O(1) |
5. Related articles
- Java Collections Tutorial
- Java List Example
- Java Array – java.util.Arrays Example
- LinkedList Java Example
6. Download the Source Code
This was an ArrayList example in Java.
Download the source code here: ArrayList Java Example – How to use ArrayList
Last updated on Jan. 11th, 2022
Don’t forget to check out our Academy premium site for advanced Java training!
Thanks for good information !