Java Switch Case Example
In this post, we feature a comprehensive Java Switch Case Example. Java provides decision making statements so as to control the flow of your program. The switch statement in java is the one that we will explain in this article. These statements are:
if...then
if...then...else
switch..case
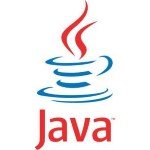
A switch statement in java checks if a variable is equal to a list of values. The variable in the switch statement can be a byte, short, int
, or char
. However, Java 7 supports also switch
statements with Strings. We will see such an example in the next sections.
1. Syntax of Java switch case
The syntax of a switch
case statement is the following:
switch (variable) {
case c1:
statements // they are executed if variable == c1
break;
case c2:
statements // they are executed if variable == c2
break;
case c3:
case c4:
statements // they are executed if variable == any of the above c's
break;
. . .
default:
statements // they are executed if none of the above case is satisfied
break;
}
switch
: theswitch
keyword is followed by a parenthesized expression, which is tested for equality with the following cases. There is no bound to the number of cases in aswitch
statement.case
: thecase
keyword is followed by the value to be compared to and a colon. Its value is of the same data type as the variable in theswitch
. The case which is equal to the value of the expression is executed.default
: If no case value matches theswitch
expression value, execution continues at thedefault
clause. This is the equivalent of the"else"
for theswitch
statement. It is conventionally written after the last case, and typically isn’t followed bybreak
because execution just continues out of theswitch
. However, it would be better to use abreak
keyword todefault
case, too. If no case matched and there is nodefault
clause, execution continues after the end of theswitch
statement.break
: Thebreak
statement causes execution to exit theswitch
statement. If there is nobreak
, execution flows through into the next case, but generally, this way is not preferred.
2. Example of switch case
Let’s see an example of the switch case
. Create a java class named SwitchCaseExample.java
with the following code:
SwitchCaseExample.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 | package com.javacodegeeks.javabasics.switchcase; public class SwitchCaseExample { public static void main(String[] args) { grading( 'A' ); grading( 'C' ); grading( 'E' ); grading( 'G' ); } public static void grading( char grade) { int success; switch (grade) { case 'A' : System.out.println( "Excellent grade" ); success = 1 ; break ; case 'B' : System.out.println( "Very good grade" ); success = 1 ; break ; case 'C' : System.out.println( "Good grade" ); success = 1 ; break ; case 'D' : case 'E' : case 'F' : System.out.println( "Low grade" ); success = 0 ; break ; default : System.out.println( "Invalid grade" ); success = - 1 ; break ; } passTheCourse(success); } public static void passTheCourse( int success) { switch (success) { case - 1 : System.out.println( "No result" ); break ; case 0 : System.out.println( "Final result: Fail" ); break ; case 1 : System.out.println( "Final result: Success" ); break ; default : System.out.println( "Unknown result" ); break ; } } } |
In the above code we can see two switch
case statements, one using char
as data type of the expression of the switch
keyword and one using int
.
Output
Excellent grade
Final result: Success
Good grade
Final result: Success
Low grade
Final result: Fail
Invalid grade
No result
Below is the equivalent of the switch
case statement in the method passTheCourse()
using if..then..else
:
1 2 3 4 5 6 7 8 9 | if (success == - 1 ) { System.out.println( "No result" ); } else if (success == 0 ) { System.out.println( "Final result: Fail" ); } else if (success == 1 ) { System.out.println( "Final result: Success" ); } else { System.out.println( "Unknown result" ); } |
3. Example of switch case using String
As we mentioned in the introduction of this example, Java SE 7 supports String in switch
case statements. Let’s see such an example. Create a java class named StringSwitchCase.java
with the following code:
StringSwitchCase.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | package com.javacodegeeks.javabasics.switchcase; public class StringSwitchCase { public static void main(String args[]) { visitIsland( "Santorini" ); visitIsland( "Crete" ); visitIsland( "Paros" ); } public static void visitIsland(String island) { switch (island) { case "Corfu" : System.out.println( "User wants to visit Corfu" ); break ; case "Crete" : System.out.println( "User wants to visit Crete" ); break ; case "Santorini" : System.out.println( "User wants to visit Santorini" ); break ; case "Mykonos" : System.out.println( "User wants to visit Mykonos" ); break ; default : System.out.println( "Unknown Island" ); break ; } } } |
If we run the above code, we will have the following result:
Output
User wants to visit Santorini
User wants to visit Crete
Unknown Island
4. More articles
5. Download the source code
This was a Java Switch-Case Example.
You can download the source code from here: Java Switch Case Example
Last updated on Apr. 29th, 2021
How differ performance for individual options? Have you try microbenchmarking it?
Switch statement in java programing switch statement in java programing The switch statement is another conditional structure. it is good of alternate of nested if-else .it can be used easily when there are many choices available and only one should be executed. Nested if becomes very difficult in such a situation. If the statement makes a selection based on single true or false condition. there are four cases of computing taxes which depends on the value of status .to full account for all case nested if statement were used. Overuse of nested if statement makes a program difficult to read… Read more »