String.format Java Example
In this post, we feature a comprehensive String.format Java Example. In Java, printf is exactly like string.format, but it does not return anything, it only prints the result.
We are going to see how to format Strings in Java. Developers familiar with C, will find the methods used here resembling printf
function. And the formatting specifications are very similar. In fact, PrintStream
class in Java, has a member function called printf designed to work similarly with C’s printf
.
You can also check the Printf Java Example in the following video:
Let’s see a simple String.format Java example and how you can use it.
1. Using printf
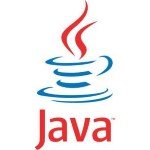
As we mentioned in the beginning, in Java, printf is exactly like string.format, but it does not return anything, it only prints the result. The complete signature of printf
is printf(String format, Object... args)
. The first argument is a String
that describes the desired formatting of the output. From there on, printf
can have multiple number of arguments of any type. At runtime, these arguments will be converted to String
and will be printed according to the formatting instructions.
The most basic rule that string formatting follows is this:
"%[Parameter field][Flags field][Width field][.Precision field][Length field][Type field]"
So let’s see what all of the above mean :
%
is a special character denoting that a formatting instruction follows.[Parameter field]
or[argument_index$]
explicitly denoted the index of the arguments to be formatted. If it not present, arguments will be formatted in the same order as they appear in the arguments list. ($
indicates there is an argument index)[Flags field]
is a special formatting instruction. For example, the+
flag specifies that a numeric value should always be formatted with a sign, and the0
flag specifies that0
is the padding character. Other flags include–
that is pad on the right,+
pad on the left (if the formatted object is a string) and#
is for alternative format. (Note that some flags cannot be combined with certain other flags or with certain formatted objects)[Width field]
denotes the minimum number of output characters for that Object.[.Precision field]
denotes the precision of floating point numbers in the output. That is basically the number of decimal digits you wish to print on the output. But it can be used for other types to truncate the output width.[Length field]
:hh
– Convert a variable of type char to integer and printh
– Convert a variable of type short to integer and printl
– For integers, a variable of type long is expected.ll
– For integers, a variable of type long long is expected.L
– For floating point, a variable of type long double is expected.z
– or integers, an argument is expected of type size_t.[Type field]
type along with%
, are the only mandatory formatting arguments. type simply denotes the type of the object that will be formatted in the output. For integers that isd
, for strings that iss
, for floating point numbers that isf
, for integers with hex format that isx
.
Let’s see a basic example.
StringFormatExample.java
01 02 03 04 05 06 07 08 09 10 11 12 13 | package com.javacodegeeks.core.string; public class StringFormatExample { public static void main(String[] args) { System.out.printf( "Integer : %d\n" , 15 ); System.out.printf( "Floating point number with 3 decimal digits: %.3f\n" , 1.21312939123 ); System.out.printf( "Floating point number with 8 decimal digits: %.8f\n" , 1.21312939123 ); System.out.printf( "String: %s, integer: %d, float: %.6f" , "Hello World" , 89 , 9.231435 ); } } |
Output
Integer : 15
Floating point number with 3 decimal digits: 1.213
Floating point number with 8 decimal digits: 1.21312939
String: Hello World, integer: 89, float: 9.231435
The following example depicts the usage of ‘%b’ which will print true if the argument is non null, otherwise prints false.
StringFormatExample2.java:
public class StringFormatExample2{ public static void main(String[] args){ System.out.printf("%b\n","Java Code Geeks"); System.out.printf("%b\n",25); System.out.printf("%b\n",null); } }
Output
true true false
The following example depicts the usage of ‘%c’ which will print the char value of ASCII code provided in the argument.
StringFormatExample3.java:
public class StringFormatExample3 { public static void main(String[] args){ System.out.printf("%c\n", 74); System.out.printf("%c\n", 65); System.out.printf("%c\n", 86); System.out.printf("%c\n", 65); } }
Output
J A V A
The following example depicts the usage of ‘%h’ which prints the hashcode value of the argument.
StringFormatExample4.java:
public class StringFormatExample4 { public static void main(String[] args){ System.out.printf("%h", "Java Code Geeks"); } }
Output
ec23cb9a
The following example depicts the usage of ‘%o’ which prints the Octal value of the argument.
StringFormatExample5.java:
public class StringFormatExample5 { public static void main(String[] args){ System.out.printf("%o\n",25); System.out.printf("%o\n", 100); } }
Output
31 144
The following example depicts the usage of ‘%x’ which prints the Hex value of the argument.
StringFormatExample6.java:
public class StringFormatExample6 { public static void main(String[] args){ System.out.printf("%x\n", 25); System.out.printf("%x\n", 100); } }
Output
19 64
So let’s see some basic rules around string formatting.
Specifier | Applies to | Output |
%a | floating point (except BigDecimal) | Hex output of floating point number |
%b | Any type | “true” if non-null, “false” if null |
%c | character | Unicode character |
%d | integer (incl. byte, short, int, long, bigint) | Decimal Integer |
%e | floating point | decimal number in scientific notation |
%f | floating point | decimal number |
%g | floating point | decimal number, possibly in scientific notation depending on the precision and value. |
%h | any type | Hex String of value from hashCode() method. |
%n | none | Platform-specific line separator. |
%o | integer (incl. byte, short, int, long, bigint) | Octal number |
%s | any type | String value |
%t | Date/Time (incl. long, Calendar, Date and TemporalAccessor) | %t is the prefix for Date/Time conversions. |
%x | integer (incl. byte, short, int, long, bigint) | Hex string. |
2. String.format Java – Formatting a String
Here is a basic list of the most important rules when you want to format a String.
2.1 Integer formatting
%d
: will print the integer as it is.%6d
: will pint the integer as it is. If the number of digits is less than 6, the output will be padded on the left.%-6d
: will pint the integer as it is. If the number of digits is less than 6, the output will be padded on the right.%06d
: will pint the integer as it is. If the number of digits is less than 6, the output will be padded on the left with zeroes.%.2d
: will print maximum 2 digits of the integer.
Here is an example:
StringFormatExample.java:
01 02 03 04 05 06 07 08 09 10 | package com.javacodegeeks.core.string; public class StringFromatExample { public static void main(String[] args) { System.out.printf( "%-12s%-12s%s\n" , "Column 1" , "Column 2" , "Column3" ); System.out.printf( "%-12d%-12d%07d\n" , 15 , 12 , 5 ); } } |
The above program will print out :
Column 1 Column 2 Column3
15 12 0000005
The following example depicts the usage of ‘%d’ which formats the integer argument.
StringFormatExample7.java:
public class StringFormatExample7 { public static void main(String[] args){ System.out.printf("%d\n", 100); System.out.printf("%10d\n", 100); System.out.printf("%010d\n", 100); } }
Output
100 100 0000000100
The following example depicts the usage of ‘%d’ which formats the integer argument.
StringFormatExample8.java:
public class StringFormatExample8 { public static void main(String[] args){ System.out.printf("%,d\n", 1000000000); System.out.printf("%,d\n", 1234); } }
Output
1,000,000,000 1,234
The following example depicts the usage of ‘%d’ which formats the integer argument.
StringFormatExample9.java:
public class StringFormatExample9 { public static void main(String[] args){ System.out.printf("%(d", -25); } }
Output
(25)
2.2 String formatting
%s
: will print the string as it is.%15s
: will pint the string as it is. If the string has less than 15 characters, the output will be padded on the left.%-6s
: will pint the string as it is. If the string has less than 6 characters, the output will be padded on the right.%.8s
: will print maximum 8 characters of the string.
StringFormatExample.java:
01 02 03 04 05 06 07 08 09 10 11 12 | package com.javacodegeeks.core.string; public class StringFormatExample { public static void main(String[] args) { System.out.printf( "%-12s%-12s%s\n" , "Column 1" , "Column 2" , "Column3" ); System.out.printf( "%-12.5s%s" , "Hello World" , "World" ); } } |
The above program will print out :
Column 1 Column 2 Column3
Hello World
The following example depicts the usage of ‘%s’ which formats the String argument.
StringFormatExample10.java:
public class StringFormatExample10 { public static void main(String[] args){ System.out.printf("%.4s\n", "Java Code Geeks"); System.out.printf("%12.9s\n", "Java Code Geeks"); System.out.printf("|%s|\n", "Java Code Geeks"); } }
Output
Java Java Code |Java Code Geeks|
2.3 Floating point formatting
%f
: will print the number as it is.%15f
: will pint the number as it is. If the number has less than 15 digits, the output will be padded on the left.%.8f
: will print maximum 8 decimal digits of the number.%9.4f
: will print maximum 4 decimal digits of the number. The output will occupy 9 characters at least. If the number of digits is not enough, it will be padded
StringFormatExample.java:
01 02 03 04 05 06 07 08 09 10 11 12 | package com.javacodegeeks.core.string; public class StringFormatExample { public static void main(String[] args) { System.out.printf( "%-12s%-12s\n" , "Column 1" , "Column 2" ); System.out.printf( "%-12.5f%.20f" , 12.23429837482 , 9.10212023134 ); } } |
The above program will print out :
Column 1 Column 2
12.23430 9.10212023134000000000
As you can see if you truncate the number of decimal digits, some of the precision is lost. On the other hand if you specify more decimal numbers in the formatting options, the number will be padded is necessary.
2.4 Date and time formatting
Using the formatting characters with %T
instead of %t
in the table below makes the output uppercase.
Flag | Notes |
%tA | Full name of the day of the week – (e.g. “Monday“) |
%ta | Abbreviated name of the week day – (e.g. “Mon“) |
%tB | Full name of the month – (e.g. “January“) |
%tb | Abbreviated month name – (e.g. “Jan“) |
%tC | Century part of year formatted with two digits – (e.g. “00” through “99”) |
%tc | Date and time formatted with “%ta %tb %td %tT %tZ %tY” – (e.g. “Mon Jan 11 03:35:51 PST 2019“) |
%tD | Date formatted as “%tm/%td/%ty“ |
%td | Day of the month formatted with two digits – (e.g. “01” to “31“) |
%te | Day of the month formatted without a leading 0 – (e.g. “1” to “31”) |
%tF | ISO 8601 formatted date with “%tY-%tm-%td“. |
%tH | Hour of the day for the 24-hour clock – (e.g. “00” to “23“) |
%th | Same as %tb. |
%tI | Hour of the day for the 12-hour clock – (e.g. “01” – “12“) |
%tj | Day of the year formatted with leading 0s – (e.g. “001” to “366“) |
%tk | Hour of the day for the 24-hour clock without a leading 0 – (e.g. “0” to “23“) |
%tl | Hour of the day for the 12-hour click without a leading 0 – (e.g. “1” to “12“) |
%tM | Minute within the hour formatted a leading 0 – (e.g. “00” to “59“) |
%tm | Month formatted with a leading 0 – (e.g. “01” to “12“) |
%tN | Nanosecond formatted with 9 digits and leading 0s – (e.g. “000000000” to “999999999”) |
%tp | Locale specific “am” or “pm” marker. |
%tQ | Milliseconds since epoch Jan 1, 1970 00:00:00 UTC. |
%tR | Time formatted as 24-hours – (e.g. “%tH:%tM“) |
%tr | Time formatted as 12-hours – (e.g. “%tI:%tM:%tS %Tp“) |
%tS | Seconds within the minute formatted with 2 digits – (e.g. “00” to “60”. “60” is required to support leap seconds) |
%ts | Seconds since the epoch Jan 1, 1970 00:00:00 UTC. |
%tT | Time formatted as 24-hours – (e.g. “%tH:%tM:%tS“) |
%tY | Year formatted with 4 digits – (e.g. “0000” to “9999“) |
%ty | Year formatted with 2 digits – (e.g. “00” to “99“) |
%tZ | Time zone abbreviation. – (e.g. “UTC“) |
%tz | Time Zone Offset from GMT – (e.g. “-0200“) |
The following example depicts the usage of various forms of ‘%t’ which formats the timestamp.
StringFormatExample11.java:
public class StringFormatExample11 { public static void main(String[] args){ System.out.printf("Current Time - %tT\n",new Date()); System.out.printf("TimeStamp - %tc\n",new Date()); System.out.printf("ISO 8601 formatted date - %tF\n",new Date()); } }
Output
Current Time - 13:07:41 TimeStamp - Fri Aug 30 13:07:41 IST 2019 ISO 8601 formatted date - 2019-08-30
The following example depicts the usage of various forms of ‘%t’ which formats the timestamp.
StringFormatExample12.java:
public class StringFormatExample12 { public static void main(String[] args){ String longDate = String.format("Today is %tA %<tB %<td, %<tY", new Date()); System.out.println(longDate); } }
Output
Today is Friday August 30, 2019
The following example depicts the usage of various forms of ‘%t’ which formats the timestamp.
StringFormatExample13.java:
public class StringFormatExample13 { public static void main(String[] args){ System.out.printf("%td %tb %tY %tl:%tM %tp",new Date(),new Date(),new Date(),new Date(),new Date(),new Date()); } }
Output
30 Aug 2019 1:22 pm
3. Using String.format Java
If you don’t want to print out the String
and just want to format it for later use, you can use the static format
method of the String
class (sort of like sprintf
in C). It works in exactly the same way as printf
as far as formatting is concerned, but it doesn’t print the String, it returns a new formatted String
.
Let’s see an example:
StringFormatExample.java:
01 02 03 04 05 06 07 08 09 10 11 | package com.javacodegeeks.core.string; public class StringFormatExample { public static void main(String[] args) { String s = String.format( "%-12.5f%.20f" , 12.23429837482 , 9.10212023134 ); System.out.println(s); } } |
The above program will print out :
12.23430 9.10212023134000000000
The following example depicts the usage of String.format( ) method which stores the formatted String in a variable.
StringFormatExample14.java:
public class StringFormatExample14 { public static void main(String[] args){ long k = 25000; String italian = String.format(Locale.ITALY,"%,d %n", k); String french = String.format(Locale.FRANCE,"%,d %n", k); String english = String.format(Locale.ENGLISH,"%,d %n", k); System.out.print(italian); System.out.print(french); System.out.print(english); } }
Output
25.000 25 000 25,000
4. Argument Index
StringArgumentIndexExample.java:
1 2 3 4 5 6 7 8 9 | package com.javacodegeeks.core.string; public class StringArgumentIndexExample { public static void main(String[] args) { System.out.println( "%4$5s %3$5s %2$5s %1$5s" , "a" , "b" , "c" , "d" ); } } |
The above program will print out:
d c b a
There is spacing of 5 characters between every character
The following example depicts the usage of argument index in String.format( ).
StringFormatExample15.java:
public class StringFormatExample15 { public static void main(String[] args){ String str = String.format("%3$10s %2$10s %1$10s", "Geeks","Code","Java"); System.out.println(str); } }
Output
Java Code Geeks
5. Exception
- IllegalFormatException (IllegalArgumentException – Unchecked exception thrown when a format string contains an illegal syntax or a format specifier that is incompatible with the given arguments. Only explicit subtypes of this exception which correspond to specific errors should be instantiated.
- NullPointerException − If the format is null.
6. Download the Source Code
This was a String.format Java Example.
You can download the full source code of this example here: String.format Java Example
String.format Java was last updated on Sept. 05, 2019
Don’t forget to check out our Academy premium site for advanced Java training!
You don’t explain what the “$” is used for regarding argument index in ::
“% [argument index] [flag] [width] [.precision] type”