Upsert in MongoDB
1. Introduction
This is an in-depth article on how to create MongoDB Upsert Example. Mongo Database is a no SQL database. It has capabilities such as query language to retrieve from the database. It also provides operational and administrative procedures. A document in Mongo Database is a data structure that has field and value pairs. These documents are like JSON objects. The values of fields can be other documents, arrays, and arrays of documents. Upsert operation is for updating a document. It is like update and insert together.
2. MongoDB Upsert
2.1 Prerequisites
MongoDB needs to be installed for the MongoDB Upsert example.
2.2 Download
You can download the Mongo DB from the Mongo Database website for Linux, windows, or macOS versions.
2.3 Setup
On macOS, you need to tap the formula repository of MongoDB. This repo needs to be added to the formula list. The command below adds the formula repository of MongoDB to the formula list:
Brew Tap Command
brew tap mongodb/brew
After setting the formula list, you can install the Mongo DB with the following command :
Brew Install command
brew install mongodb-community@4.0
2.4 MongoDB CommandLine
After installation, you can run MongoDB on the command line. To run MongoDB on the command line, the following command can be used:
Mongo Execution command
mongod --config /usr/local/etc/mongod.conf
The output of the executed command is shown below.
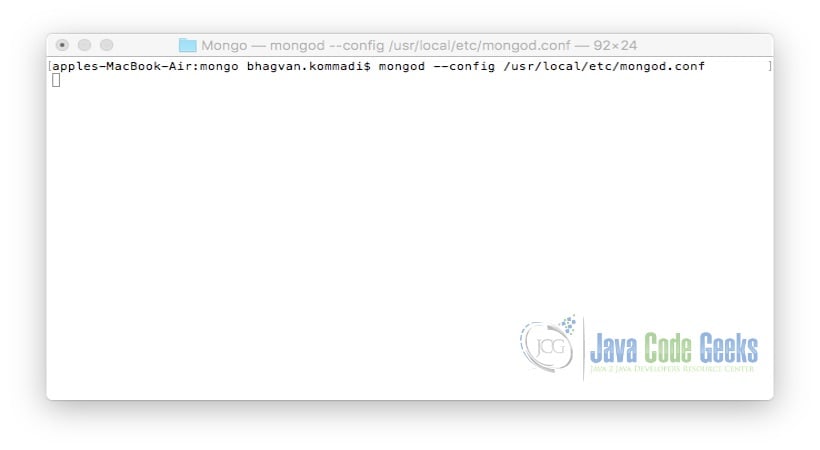
2.5 Mongo DB Operations
After starting the Mongod process, Mongo Shell can be invoked on the command line. Mongo shell can be run using the command below:
Mongo Shell command
mongo
The output of the executed command is shown below.
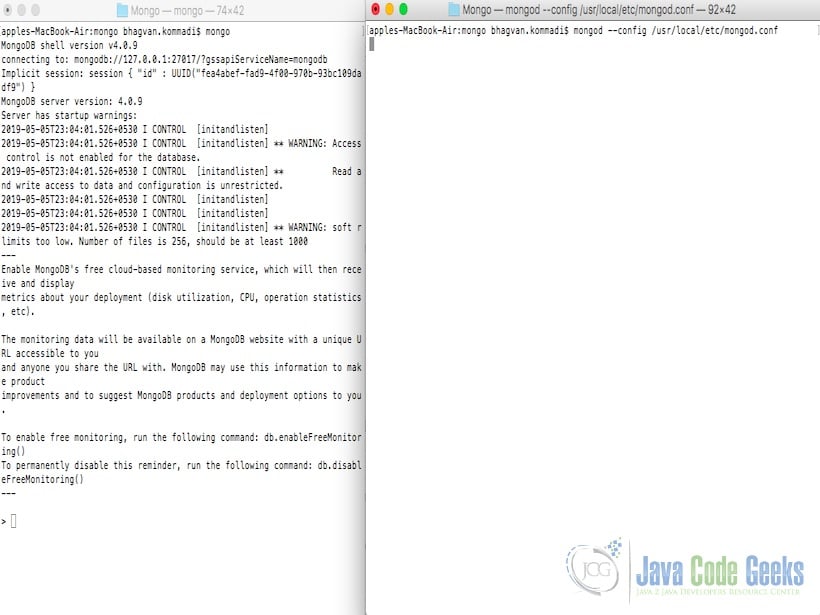
2.5.1 Database Initialization
You can use database_name to create a database. This command will create a new database. If the database exists, it will start using the existing database. The command below is used to create “octopus” database:
Database creation command
use octopus
The output of the executed command is shown below.
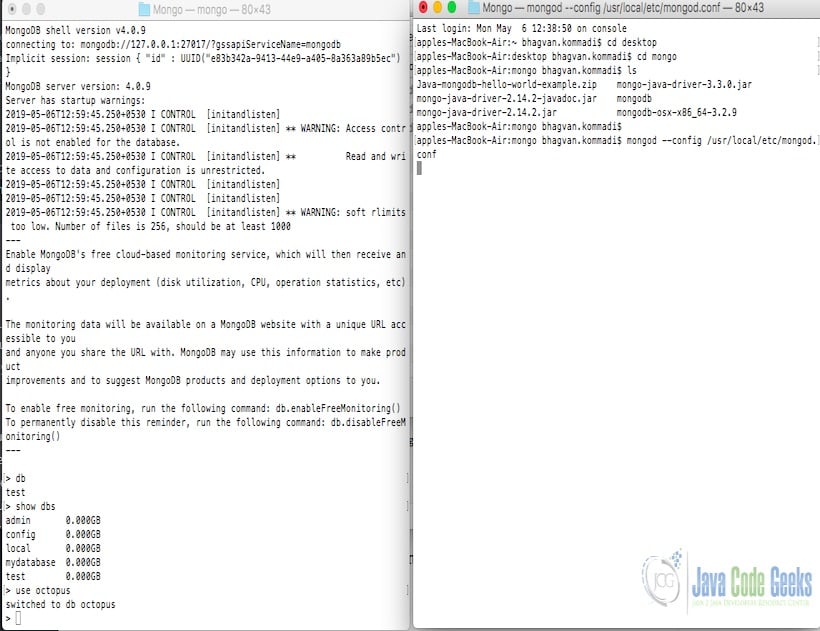
2.5.2 Drop Database
You can use the dropDatabase()
command to drop the existing database. The command below is used to drop a database. This command will delete the database. If use this command without db, then the default ‘test’ database is deleted.
Delete Database command
db.dropDatabase()
The output of the executed command is shown below.
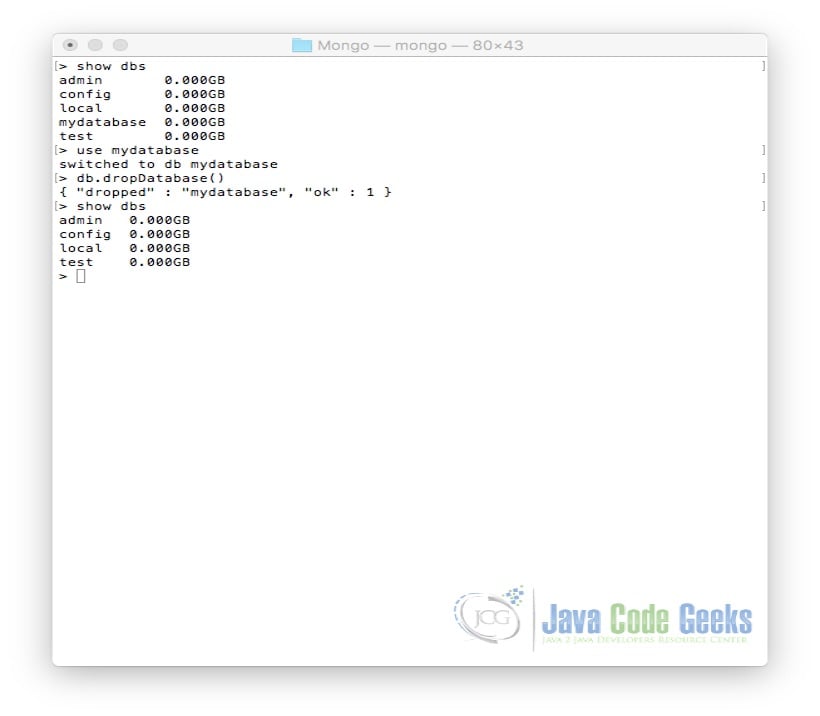
2.5.3 Create Document
You can use createCollection
command to create a set of documents. The created collection is used to create a document. The command below is used to create “persons” collection:
Create Document command
db.createCollection("persons")
The output of the executed command is shown below.
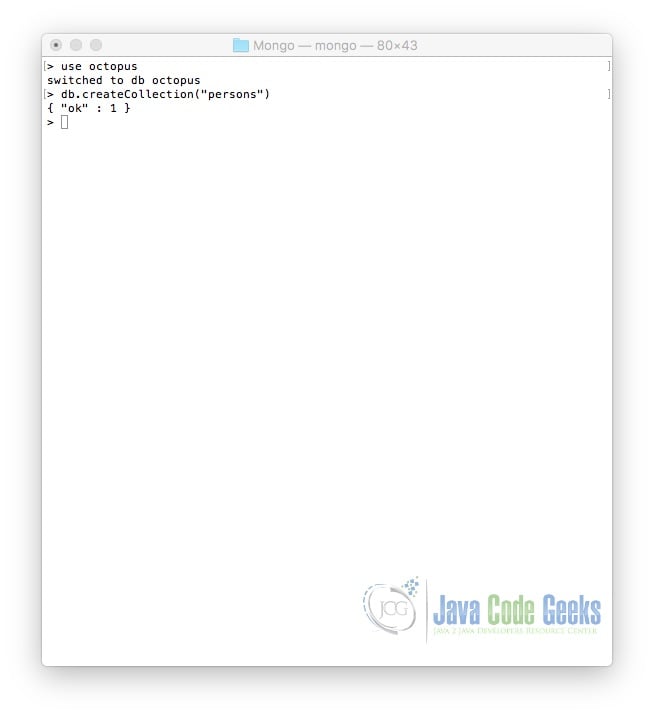
2.5.4 Upsert Document
You can use findAndModify
() method to update the document into a collection. This method update the values in the specified document
The command below is used to update the person’s document in the person’s collection if it exists. Otherwise, create a new person to be stored in the octopus database:
Upsert command
db.persons.findAndModify({ query:{person:"john clay"}, update:{ $set: { "id": 002, "ssn": 323455678 } }, upsert:true } )
The output of the executed command is shown below.
> use octopus switched to db octopus > db.persons.findAndModify({ ... query:{person:"john clay"}, ... update:{ ... $set: { "id": 002, "ssn": 323455678 } ... }, ... upsert:true ... } ... ) { "_id" : ObjectId("629fadec92cc30a1b678322f"), "person" : "john clay", "id" : 2, "lastModified" : ISODate("2022-06-07T19:58:36.972Z"), "ssn" : 323455678 } > db.persons.find().pretty() { "_id" : ObjectId("629fad5e92cc30a1b6783225"), "name" : "john smith", "id" : 2, "lastModified" : ISODate("2022-06-07T19:56:14.065Z"), "ssn" : 323455678 } { "_id" : ObjectId("629fad9892cc30a1b6783228"), "person" : "john smith", "id" : 2, "lastModified" : ISODate("2022-06-07T19:57:12.641Z"), "ssn" : 323455678 } { "_id" : ObjectId("629fadec92cc30a1b678322f"), "person" : "john clay", "id" : 2, "lastModified" : ISODate("2022-06-07T19:58:36.972Z"), "ssn" : 323455678 }
You can also use the update method to modify only if the person exists. Below is an example of update method
Update Document command
db.persons.update( {person:"john clay"}, {$set: { "ssn": 555555555 }}, {upsert:true} )
The output is as shown below:
> db.persons.update( ... {person:"john clay"}, ... {$set: { "ssn": 555555555 }}, ... {upsert:true} ... ... ) WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 }) > db.persons.find().pretty() { "_id" : ObjectId("629fad5e92cc30a1b6783225"), "name" : "john smith", "id" : 2, "lastModified" : ISODate("2022-06-07T19:56:14.065Z"), "ssn" : 323455678 } { "_id" : ObjectId("629fad9892cc30a1b6783228"), "person" : "john smith", "id" : 2, "lastModified" : ISODate("2022-06-07T19:57:12.641Z"), "ssn" : 323455678 } { "_id" : ObjectId("629fadec92cc30a1b678322f"), "person" : "john clay", "id" : 2, "lastModified" : ISODate("2022-06-07T19:58:36.972Z"), "ssn" : 555555555 }
You can use replaceOne()
method to replace the existing document with the modified one. Below is the example
Replace Document command
db.persons.replaceOne( { "person" : "john clay" }, { "person" : "John May"} )
The output for the above command is shown below:
> db.persons.replaceOne( ... { "person" : "john clay" }, ... { "person" : "John May"} ... ) { "acknowledged" : true, "matchedCount" : 1, "modifiedCount" : 1 } > db.persons.find().pretty() { "_id" : ObjectId("629fad5e92cc30a1b6783225"), "name" : "john smith", "id" : 2, "lastModified" : ISODate("2022-06-07T19:56:14.065Z"), "ssn" : 323455678 } { "_id" : ObjectId("629fad9892cc30a1b6783228"), "person" : "john smith", "id" : 2, "lastModified" : ISODate("2022-06-07T19:57:12.641Z"), "ssn" : 323455678 } { "_id" : ObjectId("629fadec92cc30a1b678322f"), "person" : "John May" }
3. Download the Source Code
You can download the full source code of this example here: Upsert in MongoDB