Understanding WebLogic Web Service
1. Overview
In this article, we will take a look at Weblogic Web Service examples.
2. WebLogic Web Service
Using weblogic webservices framework, SOAP web services are built. SOAP is an acronym for Simple Object Access Protocol. SOAP is used for developing web services that are based on XML based industry-standard protocol. SOAP security is based on WS Security. SOAP web services are platform and language independent.
2.1 Prerequisites
Java 8 is required on the Linux, windows or mac operating system. Ant 1.10.5 is required for ant projects. Weblogic 12.1.3 is used as a servlet container to deploy the examples.
2.2 Download
You can download Java 8 from the Oracle web site. Apache Ant 1.10.5 can be downloaded from this link. Weblogic 12.1.3 can be downloaded from the weblogic website.
2.3 Setup
Below are the setup commands required for the Java Environment.
Setup
JAVA_HOME="/desktop/jdk1.8.0_73" export JAVA_HOME PATH=$JAVA_HOME/bin:$PATH export PATH
2.3.1. Ant – Setup
The environment variables for ant are set as below:
Ant Environment
JAVA_HOME="/desktop/jdk1.8.0_73" ANT_HOME= "Users/bhagvan.kommadi/desktop/opensource/apache-ant-1.10.5" export ANT_HOME=$ANT_HOME\bin\ant export PATH=$ANT_HOME:$PATH
2.4 Weblogic Web Service in Java
SOAP is based on Web Services Description Language (WSDL). First, we create a service. The code below shows the service Greetings
service implementation.
GreetingsImpl
package webservices; import javax.jws.WebService; @WebService(name="GreetingsPortType", serviceName="GreetingsService") public class GreetingsImpl { public String message(String message) { try { System.out.println("message is" + message); } catch (Exception exception) { exception.printStackTrace(); } return "The message sent is'" + message + "'"; } }
EAR is created by using the ant script. The ant build.xml is shown below.
Ant Build.xml
<project name="webservices-greetings" default="all"> <!-- set global properties for this build --> <property name="wls.username" value="manager" /> <property name="wls.password" value="manager1$" /> <property name="wls.hostname" value="localhost" /> <property name="wls.port" value="7001" /> <property name="wls.server.name" value="myserver" /> <property name="ear.deployed.name" value="greetingsEar" /> <property name="example-output" value="output" /> <property name="ear-dir" value="${example-output}/greetingsEar" /> <property name="clientclass-dir" value="${example-output}/clientclasses" /> <path id="client.class.path"> <pathelement path="${clientclass-dir}"/> <pathelement path="${java.class.path}"/> <pathelement path="${classpath}"/> </path> <path id="weblogic.class.path"> <fileset dir="${weblogic.dir}"> <include name="*.jar"/> </fileset> </path> <taskdef name="jwsc" classname="weblogic.wsee.tools.anttasks.JwscTask" /> <taskdef name="clientgen" classname="weblogic.wsee.tools.anttasks.ClientGenTask" /> <taskdef name="wldeploy" classname="weblogic.ant.taskdefs.management.WLDeploy"/> <target name="all" depends="clean,build-service,deploy,client" /> <target name="clean" depends="undeploy"> <delete dir="${example-output}"/> </target> <target name="build-service"> <jwsc srcdir="src" destdir="${ear-dir}"> <jws file="webservices/GreetingsImpl.java" type="JAXWS"/> </jwsc> </target> <target name="deploy"> <wldeploy action="deploy" name="${ear.deployed.name}" source="${ear-dir}" user="${wls.username}" password="${wls.password}" verbose="true" adminurl="t3://${wls.hostname}:${wls.port}" targets="${wls.server.name}" /> </target> <target name="undeploy"> <wldeploy action="undeploy" name="${ear.deployed.name}" failonerror="false" user="${wls.username}" password="${wls.password}" verbose="true" adminurl="t3://${wls.hostname}:${wls.port}" targets="${wls.server.name}" /> </target> <target name="client"> <clientgen wsdl="http://${wls.hostname}:${wls.port}/GreetingsImpl/GreetingsService?WSDL" destDir="${clientclass-dir}" packageName="webservices.client" type="JAXWS"/> <javac srcdir="${clientclass-dir}" destdir="${clientclass-dir}" includes="**/*.java"/> <javac srcdir="src" destdir="${clientclass-dir}" includes="webservices/client/**/*.java"/> </target> <target name="run"> <javac srcdir="src" destdir="${clientclass-dir}" includes="webservices/client/*.java"/> <java classname="webservices.client.Main" fork="true" failonerror="true" > <classpath refid="client.class.path"/> <arg line="http://${wls.hostname}:${wls.port}/GreetingsImpl/GreetingsService" /> </java> </target> </project>
Ant command build-service is used to build the output ear. The following command is used to execute the building of web services.
Ant Build Service
ant build-service
The output of the ant build service command is shown below.
WSDL is created for the Greetings
Service. WSDL created is shown below in the code.
GreetingsImpl
<definitions xmlns:wsu="http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd" xmlns:wsp="http://www.w3.org/ns/ws-policy" xmlns:wsp1_2="http://schemas.xmlsoap.org/ws/2004/09/policy" xmlns:wsam="http://www.w3.org/2007/05/addressing/metadata" xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/" xmlns:tns="http://webservices/" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns="http://schemas.xmlsoap.org/wsdl/" targetNamespace="http://webservices/" name="GreetingsService"> <script id="tinyhippos-injected"/> <types> <xsd:schema> <xsd:import namespace="http://webservices/" schemaLocation="http://localhost:7001/GreetingsImpl/GreetingsService?xsd=1"/> </xsd:schema> </types> <message name="message"> <part name="parameters" element="tns:message"/> </message> <message name="messageResponse"> <part name="parameters" element="tns:messageResponse"/> </message> <portType name="GreetingsPortType"> <operation name="message"> <input wsam:Action="http://webservices/GreetingsPortType/messageRequest" message="tns:message"/> <output wsam:Action="http://webservices/GreetingsPortType/messageResponse" message="tns:messageResponse"/> </operation> </portType> <binding name="GreetingsPortTypePortBinding" type="tns:GreetingsPortType"> <soap:binding transport="http://schemas.xmlsoap.org/soap/http" style="document"/> <operation name="message"> <soap:operation soapAction=""/> <input> <soap:body use="literal"/> </input> <output> <soap:body use="literal"/> </output> </operation> </binding> <service name="GreetingsService"> <port name="GreetingsPortTypePort" binding="tns:GreetingsPortTypePortBinding"> <soap:address location="http://localhost:7001/GreetingsImpl/GreetingsService"/> </port> </service> </definitions>
Weblogic server is configured using the command below:
Weblogic Configure
./configure.sh
The output of the command is shown below:
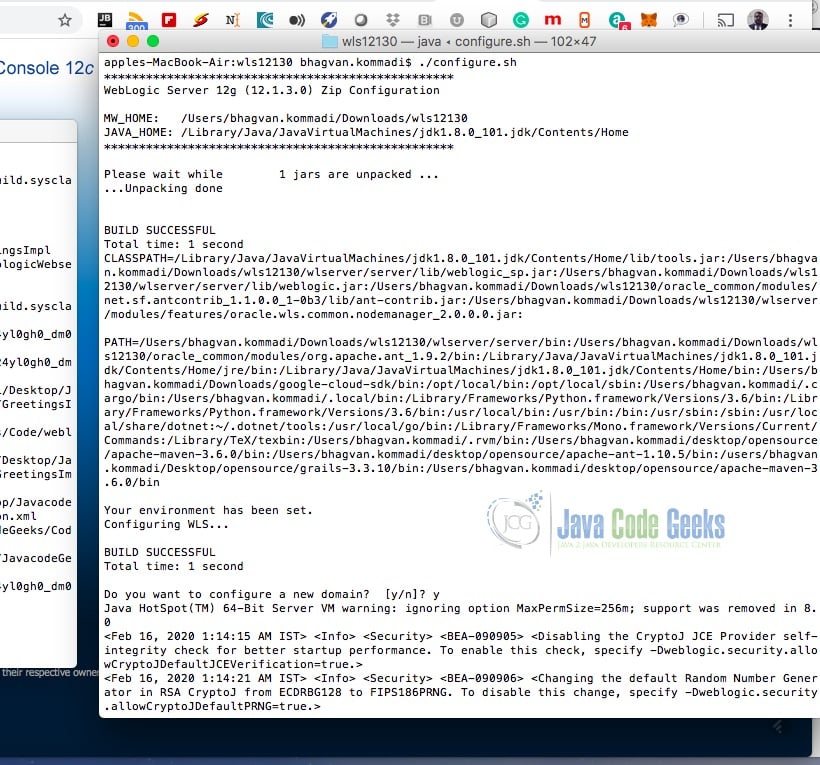
The console can be accessed using the URL: http://localhost:7001/console
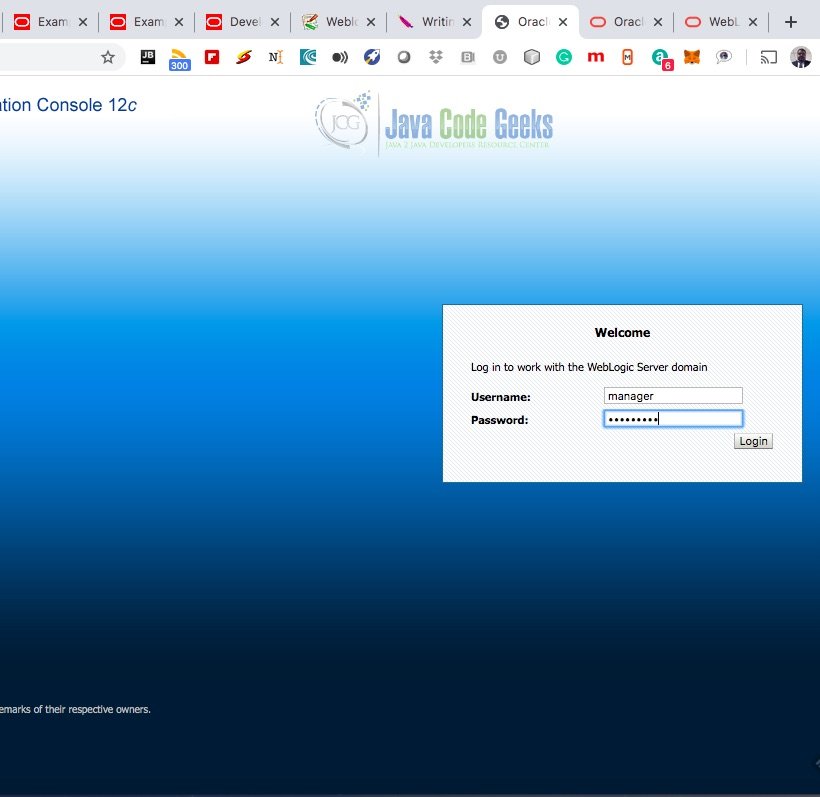
Greetings Ear can be accessed from the applications on the console
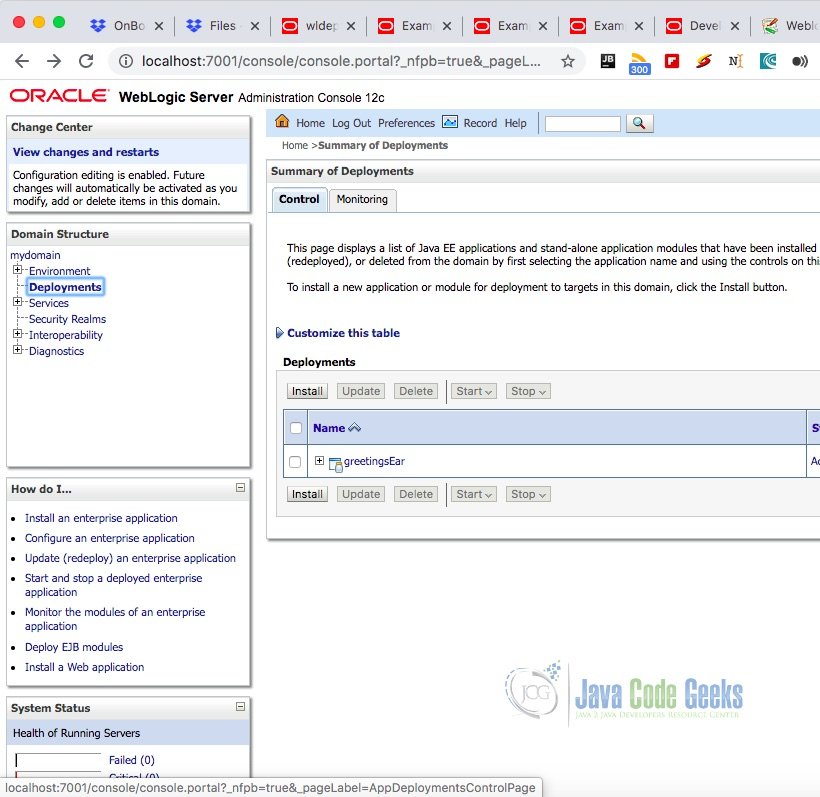
The WSDL can be accessed here
The output is shown in the screenshot below:
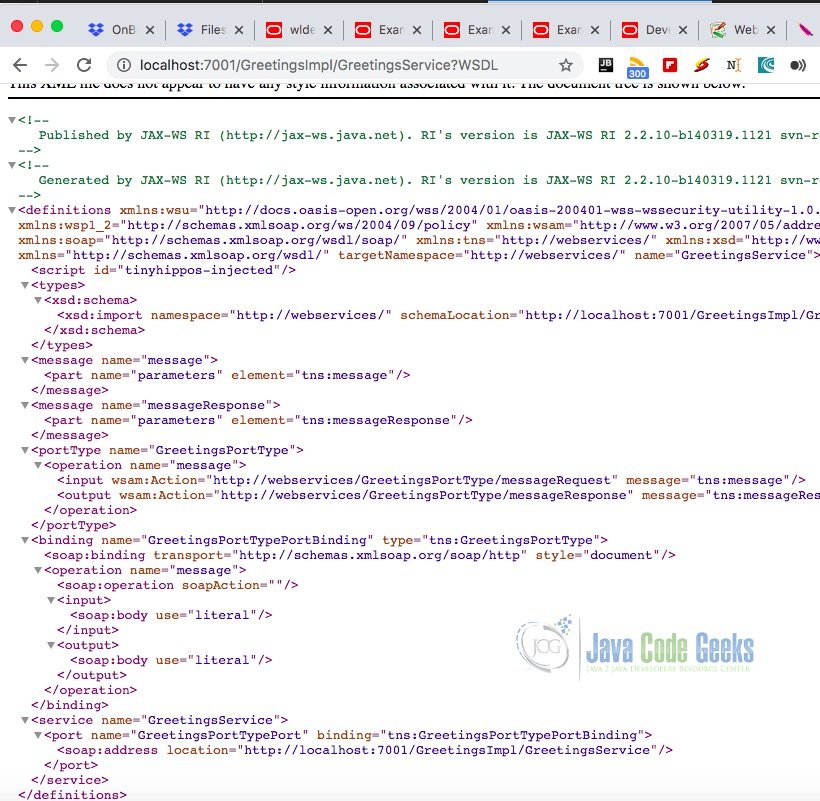
Webservices client is generated for Greetings Webservice. The command used is shown below:
Ant Client
ant client
The output of the command is shown below:
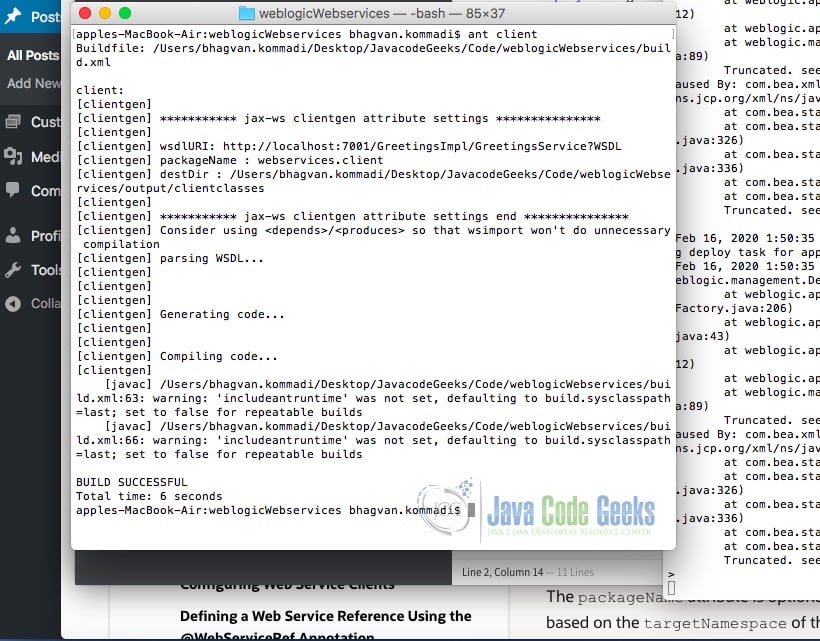
Client classes are used to invoke the service. The code is shown below.
Client Main
package webservices.client; import webservices.client.*; public class Main { public static void main(String[] args) { GreetingsService test = new GreetingsService(); GreetingsPortType port = test.getGreetingsPortTypePort(); String result = port.message("Greetings to you"); System.out.println("Greeting Service called. Result: " + result); } }
Greeting Webservice is invoked by executing the client. The command used is shown below:
Ant Run
ant run
The output of the command is shown below:
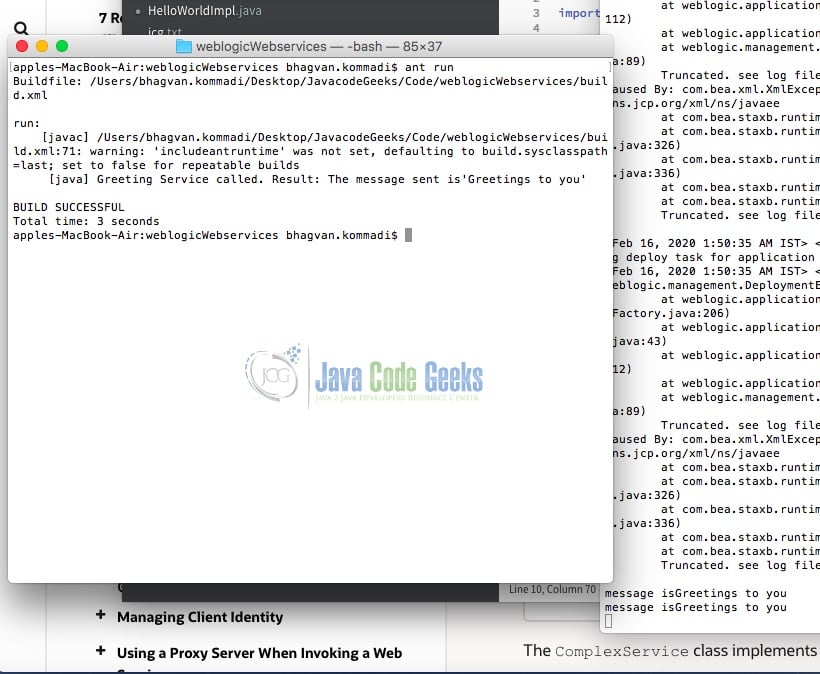
The output is “Greetings to You” which is the message Sent.
3. Download the Source Code
You can download the full source code of this example here: Understanding WebLogic Web Service
Nice but should have used some new build tools like Maven or Gradle for a vast majority of tech people out there.
ok