Polymorphism Java Example (with video)
In this post, we feature a comprehensive Polymorphism Java Example.
1. Introduction
In word Polymorphism, the word Poly stands for ‘many’ and the word morph stands for ‘forms’. Polymorphism is the ability of an object to take on many forms. Polymorphism is the capability of an action or method to do different things based on the object that it is an action upon. Polymorphism is one of the Object-Oriented Programming principles used along with Encapsulation and Inheritance in Programming languages like C++, Java, and Python. It provides flexibility to the programmers to interact with different objects by using the same message.
You can also check this tutorial in the following video:
2. Types of Polymorphism
In Java, Polymorphism can be achieved in two ways using Method Overloading and Method Overriding. Method Overloading is also called as Compile time Polymorphism or early binding, whereas Method Overriding is also called as Runtime Polymorphism or late binding. Let us now understand in detail both of them.
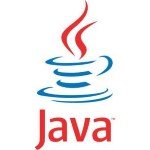
2.1 Method Overloading
Two methods are said to overloaded if they have the same method name and different parameters i.e either two methods should have a different number of parameters or the data types of the parameters of two methods must be different.
Let us understand this with a coding snippet below:
// program to show method overloading in java package com.javacodegeeks.snippets.core; class Example1 { public static String add(String s1, String s2) //method to perform String concatenation { return s1+s2; } public static int add(int a,int b) //method to perform int addition { return a+b; } public static void main(String[] args) { System.out.println(add(10,30)); //calls add function with int params System.out.println(add("Rajesh","Kumar")); //calls add function with String params } }
Output
40 RajeshKumar
In this example, when we pass String parameters to the method add, add() method at Line 6 is getting executed. This method returns the String after concatenation of both Strings s1 and s2. But when we passed integer values to the add method, add() method at Line 11 is getting executed and it returns the addition of two values. Here we used the same method name add, we are able to interact with two functionalities based on the parameters which are passed.
The compiler is able to recognize these method calls and therefore it is also called Compile time Polymorphism.
2.2 Method Overriding
In Method Overriding, during Inheritance, a child class provides its own implementation to the Parent’s method, thereby overriding the implementation of the parent. Two methods are said to be overridden only if they have the same Signature, that is, the same name and same parameters.
In this context, the Parent’s method is called an Overridden method and the Child’s method is called an Overriding method.
Let us understand what does below coding snippet contains :
Shape
class has two methods,drawShape()
anderaseShape()
.- Classes
Circle
,Square
andTriangle
extendShape
and override its two methods, each one in a different way. RandomShapeGenerator
class has a method,Shape next()
that randomly creates one of the above classes,Circle
,Square
andTriangle
.- We create a new
RandomShapeGenerator
instance and a newShape
array. - We fill the
Shape
array, by creating random shapes, using thenext()
method ofRandomShapeGenerator
. - Then we call
drawShape()
method, so each time the method of the object created randomly is called.
Let us take a look at the code snippet that follows:
001
002
003
004
005
006
007
008
009
010
011
012
013
014
015
016
017
018
019
020
021
022
023
024
025
026
027
028
029
030
031
032
033
034
035
036
037
038
039
040
041
042
043
044
045
046
047
048
049
050
051
052
053
054
055
056
057
058
059
060
061
062
063
064
065
066
067
068
069
070
071
072
073
074
075
076
077
078
079
080
081
082
083
084
085
086
087
088
089
090
091
092
093
094
095
096
097
098
099
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
|
package com.javacodegeeks.snippets.core; import java.util.Random; class Shape { void drawShape() { } void eraseShape() { } } class Circle extends Shape { @Override void drawShape() { System.out.println( "Circle.draw()" ); } @Override void eraseShape() { System.out.println( "Circle.erase()" ); } } class Square extends Shape { @Override void drawShape() { System.out.println( "Square.draw()" ); } @Override void eraseShape() { System.out.println( "Square.erase()" ); } } class Triangle extends Shape { @Override void drawShape() { System.out.println( "Triangle.draw()" ); } @Override void eraseShape() { System.out.println( "Triangle.erase()" ); } } // A "factory" that randomly creates shapes: class RandomShapeGenerator { private Random rand = new Random(); //Choose randomly a circle, a squere or a triangle public Shape next() { switch (rand.nextInt( 3 )) { default : case 0 : return new Circle(); case 1 : return new Square(); case 2 : return new Triangle(); } } } public class Polymorphism { private static RandomShapeGenerator gen = new RandomShapeGenerator(); public static void main(String[] args) { //This is an array of references of the superclass Shape Shape[] s = new Shape[ 9 ]; // Fill up the array with random shapes shapes: for ( int i = 0 ; i < s.length; i++) { s[i] = gen.next(); } //s[x].drawShape will call the specific drawShape function of whichever //shape is s[x] and not the drawShape function of class Shape //That's polymorphism. for ( int i = 0 ; i < s.length; i++) { s[i].drawShape(); } } } |
Output
Square.draw()
Square.draw()
Circle.draw()
Triangle.draw()
Triangle.draw()
Square.draw()
Circle.draw()
Circle.draw()
Square.draw()
3. Benefits of Polymorphism
1. Method overloading allows methods that perform similar or closely related functions to be accessed through a common name. For example, a program performs operations on an array of numbers which can be int, float, or double type. Method overloading allows you to define three methods with the same name and different types of parameters to handle the array operations.
2. Method overloading can be implemented on constructors allowing different ways to initialize objects of a class. This enables you to define multiple constructors for handling different types of initializations.
3. Method overriding allows a subclass to use all the general definitions that a superclass provides and adds specialized definitions through overridden methods.
4. Method overriding works together with inheritance to enable code-reuse of existing classes without the need for re-compilation.
4. Polymorphism Java Example
In this post, we have started with Polymorphism definition, how it can be achieved in Java using Method Overloading and Method Overriding. Then we clearly understood how we can implement Method Overloading and Method Overriding programmatically. Finally, we ended our topic by understanding the benefits of using Polymorphism.
5. More articles
- Java Tutorial for Beginners
- Best Way to Learn Java Programming Online
- Java Constructor Example
- Java Collections Tutorial
- Hashmap Java Example
6. Download the Source Code
This is an example of Polymorphism in java.
You can download the full source code of this example here: Polymorphism Java Example
Last updated on May 22nd, 2021