Multidimensional Array Java Example
In this article, we will see what a multidimensional array is in Java, various syntaxes, and how we can use such arrays in real-world examples.
1. Introduction
An array is a basic data type that holds multiple values of the same datatype. Each individual value can be accessed using a number based index relative to the first element in the array, starting with 0. For example, below are the arrays of numbers and characters. Arrays can also be of non-basic data types such as an array of Employees.
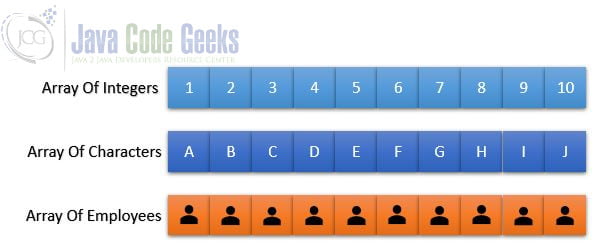
For more details on arrays in java check Java documentation at Javadocs.
2. Types of arrays
Arrays are categorized by the number of dimensions where each dimension consists of an array of predefined elements. For example, the arrays illustrated in the section-1 are 1D array or simply called arrays.
In an array, if each element consists of another array of elements then its called 2-D array. A typical example of 2-D arrays is seating rows in a cinema hall. Here, the cinema hall contains an N-number of rows and an M-number of seats. You must have seen cinema tickets bearing seat numbers as D-3 where D represents the name of the row and number represents the position of the seat starting from the first seat 1.
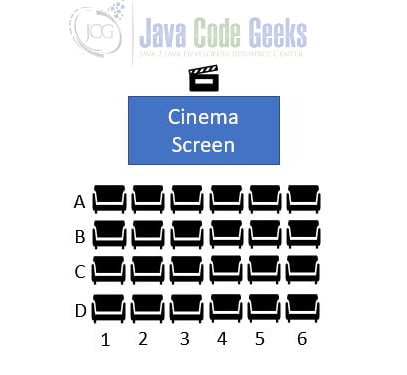
Similarly, a 3-D array is an array containing array which in turn contains another array (3 levels). A typical example of 3-D arrays in real life is that of scanning a book. A book contains P-number of pages, with each page containing L-lines and in turn, each line containing W-words.
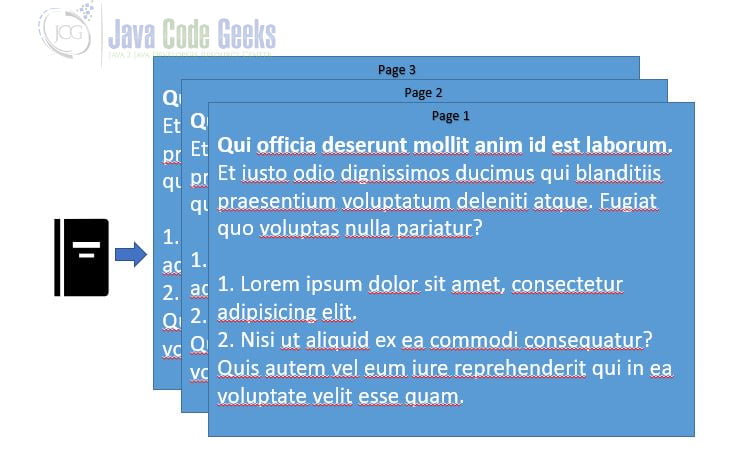
3. Examples
In this section, we give examples of how to initialize, access, and modify one of the elements in a 1-D, 2-D, and a 3-D array.
Generic syntax to declare an array:
<datatype>[][][]...n <variableName>;
Example for a 1-D array:
tryArrays() method:
// Initialise an array of numbers int[] arrayOfIntegers = new int[] { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; // Access the 5th element at index 4 System.out.println("The element at 5th position is : " + arrayOfIntegers[4]); // Modify the 5th element at index 4 arrayOfIntegers[4] = 10; System.out.println("The updated element at the 5th position is :" + arrayOfIntegers[4]);
Examples for a 2-D array:
try2DArrays() method:
// Initialise an array of numbers // This contains 4 rows of 6 seats // If seat is empty, initialise with seat numbers String[][] movieSeats = new String[][] { { "A1", "A2", "A3", "A4", "A5", "A6" }, { "B1", "B2", "B3", "B4", "B5", "B6" }, { "C1", "C2", "C3", "C4", "C5", "C6" }, { "D1", "D2", "D3", "D4", "D5", "D6" } }; // Access the seat at D3 System.out.println("The seat at D3 is unallocated : " + movieSeats[3][2]); // Allocate the D3 to Bob movieSeats[3][2] = "Bob"; System.out.println("The seat at D3 is occupied by : " + movieSeats[3][2]);
Examples for a 3-D array:
try3DArrays() method:
// Initialise an array of pages, lines and words String[][][] pagesLinesWords = new String[][][] { { // Page 1 { "This ", "is page 1 : ", "first line", "wrngly", "spelled", "word" }, // Line 1 { "This ", "is page 1 : ", "second line", "correctly", "spelled", "word" }, // Line 2 { "This ", "is page 1 : ", "thrid line", "correctly", "spelled", "word" }, // Line 3 { "This ", "is page 1 : ", "fourth line", "wrngly", "spelled", "word" }, // Line 4 }, { // Page 2 { "This ", "is page 2 : ", "first line", "wrngly", "phrased", "word" }, // Line 1 { "This ", "is page 2 : ", "second line", "correctly", "phrased", "word" }, // Line 2 { "This ", "is page 2 : ", "thrid line", "correctly", "phrased", "word" }, // Line 3 { "This ", "is page 2 : ", "fourth line", "wrngly", "phrased", "word" }, // Line 4 }, { // Page 3 { "This ", "is page 3 : ", "first line", "wrngly", "spelled", "word" }, // Line 1 { "This ", "is page 3 : ", "second line", "correctly", "spelled", "word" }, // Line 2 { "This ", "is page 3 : ", "thrid line", "correctly", "spelled", "word" }, // Line 3 { "This ", "is page 3 : ", "fourth line", "wrngly", "spelled", "word" }, // Line 4 }, { // Page 4 { "This ", "is page 4 : ", "first line", "wrngly", "spelled", "word" }, // Line 1 { "This ", "is page 4 : ", "second line", "correctly", "spelled", "word" }, // Line 2 { "This ", "is page 4 : ", "thrid line", "correctly", "spelled", "word" }, // Line 3 { "This ", "is page 4 : ", "fourth line", "wrngly", "spelled", "word" }, // Line 4 } }; // Access the 4th word on page 1, line 1 System.out.println("The word at page 1, line 1 is 4th word is " + "wrongly spelled ==> " + pagesLinesWords[0][0][3]); // Modify the word pagesLinesWords[0][0][3] = "correctly"; System.out.println("The word on page 1, line 1 is 4th word after correction ==> " + pagesLinesWords[0][0][3] + ".");
4. When do we use a multi-dimensional arrays
We find multi-dimensional arrays are very powerful when analyzing a lot of complex structured problems. Here are some of the examples for such complex structured problems:
- Solving the famous cubes matching in a Rubik cube,
- Solving graphical problems involving X and Y axis on a 2-D plane,
- Solving graphical problems involving X, Y and Z axis on a 3-D plane,
- Solving problems involving the array of network elements connected in a grid pattern in X, Y and Z axis
- Solving complex graph-related problems such as Dijkstra’s algorithm
5. Put them all together
Here is MultidimensionalArrayExample
class which demos the usage of 1-D, 2-D and 3-D arrays. In this class, it exposes 3 methods, tryArrays()
, try2DArray()
, try3DArray()
for 1-D, 2-D and 3-D arrays respectively. This also contains a main()
method to call all the methods.
/** * This class is a demo to explain multi-dimensional arrays. * * @author Shivakumar Ramannavar */ public class MultidimensionalArrayExample { /** * Try 1-D array */ public static void try1DArray() { System.out.println("Trying the 1-D array"); System.out.println("===================="); int numberOfNumbers = 10; // Initialise an array of numbers int[] arrayOfIntegers = new int[] { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; System.out.printf("There are %d numbers in the array.\n", numberOfNumbers); // Changing the fifth element to 10, index is 4 System.out.println("The element at the 5th position is:" + arrayOfIntegers[4]); System.out.println("Modifying the item at arrayOfIntegers[4]. " + "Assigning the value to 10."); arrayOfIntegers[4] = 10; System.out.println("The updated element at the 5th position is :" + arrayOfIntegers[4]); } /** * Try 2-D array */ public static void try2DArray() { System.out.println("\n\nTrying the 2-D array"); System.out.println("===================="); int numOfRows = 4; int numOfSeatsInEachRow = 6; System.out.printf("There are %d rows and %d seats in each row " + "in the cinema hall.", numOfRows, numOfSeatsInEachRow); // Initialise an array of numbers // This contains 4 rows of 6 seats // If seat is empty, initialise with seat numbers String[][] movieSeats = new String[][] { { "A1", "A2", "A3", "A4", "A5", "A6" }, { "B1", "B2", "B3", "B4", "B5", "B6" }, { "C1", "C2", "C3", "C4", "C5", "C6" }, { "D1", "D2", "D3", "D4", "D5", "D6" }, }; // Access the seat at D3 System.out.println("The seat at D3 is unallocated : " + movieSeats[3][2]); System.out.println("Assiging the seat to Bob by modifying the item at movieSeats[3][2]."); // Allocate the D3 to Bob movieSeats[3][2] = "Bob"; System.out.println("The seat at D3 is occupied by : " + movieSeats[3][2]); } /** * Try 3-D array */ public static void try3DArray() { System.out.println("\n\nTrying the 3-D array"); System.out.println("===================="); int numfOfPages = 4; int numOfLines = 40; int numOfWords = 10; System.out.printf("There are %d pages in the book.\n", numfOfPages); System.out.printf("There are %d lines on a page.\n", numOfLines); System.out.printf("There are %d words in a line.\n", numOfWords); // Initialise an array of pages, lines and words String[][][] pagesLinesWords = new String[][][] { { // Page 1 { "This ", "is page 1 : ", "first line", "wrngly", "spelled", "word" }, // Line 1 { "This ", "is page 1 : ", "second line", "correctly", "spelled", "word" }, // Line 2 { "This ", "is page 1 : ", "thrid line", "correctly", "spelled", "word" }, // Line 3 { "This ", "is page 1 : ", "fourth line", "wrngly", "spelled", "word" }, // Line 4 }, { // Page 2 { "This ", "is page 2 : ", "first line", "wrngly", "phrased", "word" }, // Line 1 { "This ", "is page 2 : ", "second line", "correctly", "phrased", "word" }, // Line 2 { "This ", "is page 2 : ", "thrid line", "correctly", "phrased", "word" }, // Line 3 { "This ", "is page 2 : ", "fourth line", "wrngly", "phrased", "word" }, // Line 4 }, { // Page 3 { "This ", "is page 3 : ", "first line", "wrngly", "spelled", "word" }, // Line 1 { "This ", "is page 3 : ", "second line", "correctly", "spelled", "word" }, // Line 2 { "This ", "is page 3 : ", "thrid line", "correctly", "spelled", "word" }, // Line 3 { "This ", "is page 3 : ", "fourth line", "wrngly", "spelled", "word" }, // Line 4 }, { // Page 4 { "This ", "is page 4 : ", "first line", "wrngly", "spelled", "word" }, // Line 1 { "This ", "is page 4 : ", "second line", "correctly", "spelled", "word" }, // Line 2 { "This ", "is page 4 : ", "thrid line", "correctly", "spelled", "word" }, // Line 3 { "This ", "is page 4 : ", "fourth line", "wrngly", "spelled", "word" }, // Line 4 } }; // Access the 4th word on page 1, line 1 System.out.println("\nThe line on page 1, line 1 is ==> [" + String.join(" ", pagesLinesWords[0][0]) + "]"); System.out.println( "The word on page 1, line 1 is 4th word is " + "wrongly spelled ==> " + pagesLinesWords[0][0][3]); System.out.println("\nCorrecting the word by modifying array element pagesLinesWords[0][0][3]."); // Modify the word pagesLinesWords[0][0][3] = "correctly"; System.out.println("The word on page 1, line 1 is 4th word after correction ==> " + pagesLinesWords[0][0][3] + "."); } /** * @param args */ public static void main(String[] args) { // Initialise 1-D array and, access and modify one of the elements try1DArray(); // Initialise 2-D array and, access and modify one of the elements try2DArray(); // Initialise 3-D array and, access and modify one of the elements try3DArray(); } }
6. Execution
In this section, we will execute the programs for the multidimensional array and see how it is working.
Prerequisites:
- Java 1.8 installed in the system. Environment variables
JAVA_HOME
set to the Java location andPATH
set to the directory containing javac and java binaries (%JAVA_HOME%/bin
on windows or$JAVA_HOME/bin
on Linux machines) - Source code zip and downloaded to a location (say,
C:\JavaCodeGeeks
. This would be different for Linux) - Eclipse IDE (Photon Release (4.8.0) is used for this example)
3.1 Execution using eclipse
Step 1: Open the Eclipse IDE.
Step 2: Click On File >> Import.
Step 3: From the “Import” menu select “Existing Projects into Workspace”.
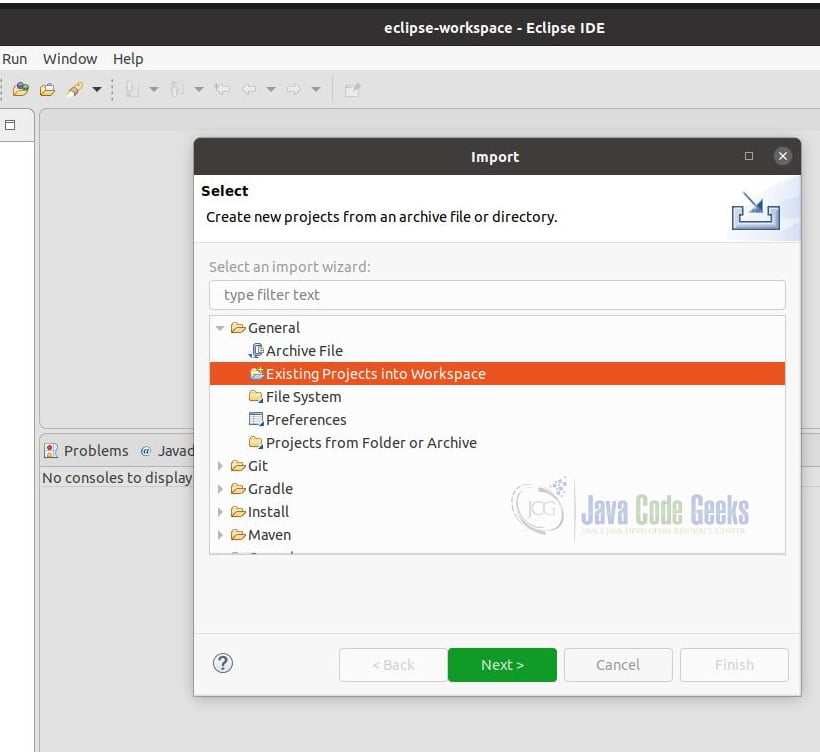
Step 4: Click Next.
Step 5: On the next page, click browse and select the root of the example folder (say,C:\JavaCodeGeeks
). Click on the “Finish” button.
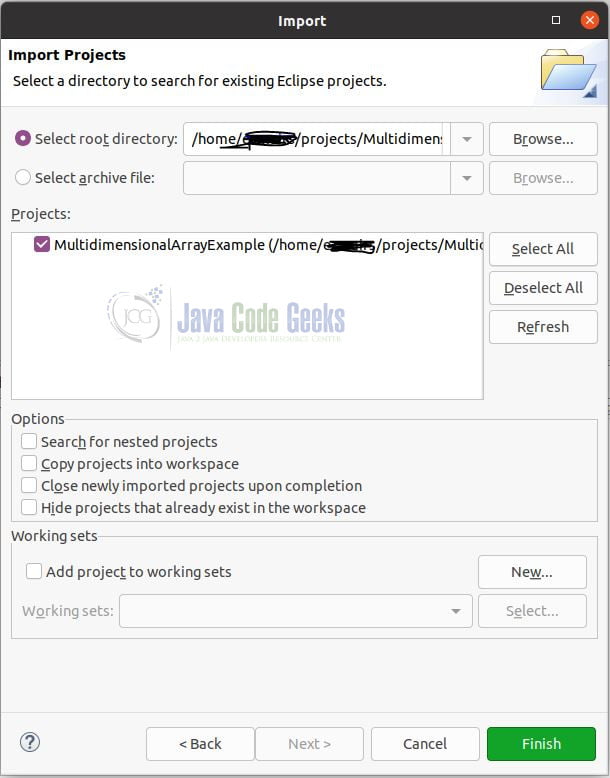
Step 6: Ensure Package Explorer is loaded and lists all the files as shown in the figure below.
Step 7: Click on src >> com.javacodegeeks.examples >> MultidimensionalArrayExample
Step 8: Right-click on MultidimensionalArrayExample.java
, from the menu, choose
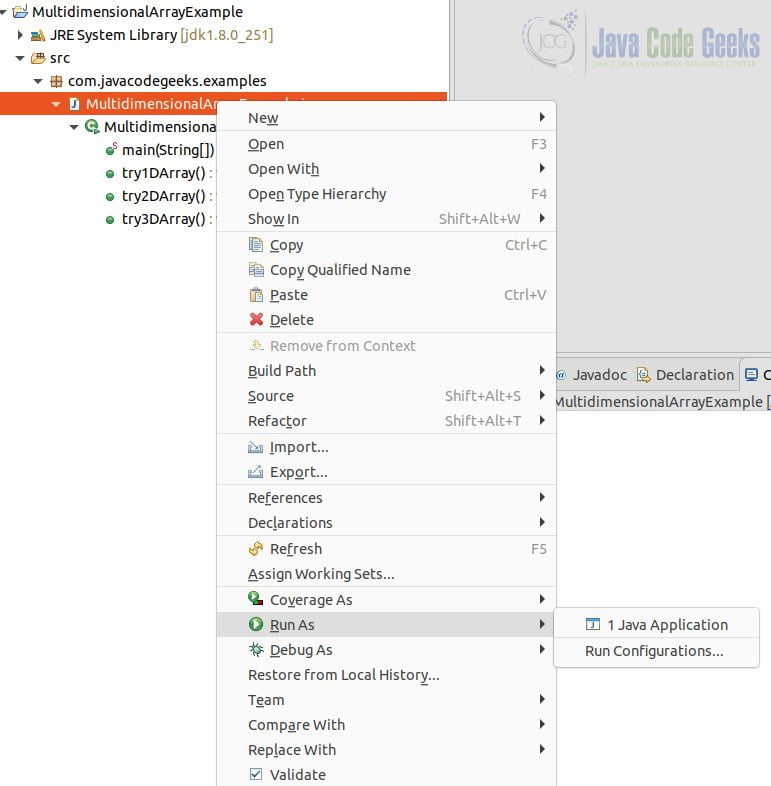
See the sample output as below:
Trying the 1-D array ==================== There are 10 numbers in the array. The element at the 5th position is:5 Modifying the item at arrayOfIntegers[4]. Assigning the value to 10. The updated element at the 5th position is :10 Trying the 2-D array ==================== There are 4 rows and 6 seats in each row in the cinema hall. The seat at D3 is unallocated: D3 Assiging the seat to Bob by modifying the item at movieSeats[3][2]. The seat at D3 is occupied by Bob Trying the 3-D array ==================== There are 4 pages in the book. There are 40 lines on a page. There are 10 words in a line. The line on page 1, line 1 is ==> [This is page 1 : first line wrngly spelled word] The word on page 1, line 1 is 4th word is wrongly spelled ==> wrngly Correcting the word by modifying array element pagesLinesWords[0][0][3]. The word on page 1, line 1 is 4th word after correction ==> correctly.
7. Download the Eclipse Project
You can download the full source code of this example here: Multidimensional Array Java Example