Map to Array in JavaScript
This article aims to explore various techniques to transform map data into an array format, allowing easy iteration and manipulation of key-value pairs in your JavaScript projects.
1. Introduction
Converting a map to an array in JavaScript involves transforming key-value
pairs from a Map object into an array of arrays or arrays of objects. Let’s begin with explaining what Maps and Arrays are in JavaScript and some common reasons and scenarios where a person might want to convert a Map to an Array in JavaScript.
1.1 Introduction to Arrays in Javascript
In JavaScript, An Array is a way of storing more than one value in a single place. An array provides a method of tracking a list of items. With an array, A developer can create a list of items and add, remove, or change items in the array at any time. To create an array, you put a list of items between opening and closing brackets []
. Below is an example code of creating an array containing some famous authors in JavaScript.
var authors = ['William Shakespear', 'Chinua Achebe', 'Thomas Paine'];
1.2 Introduction to Maps in JavaScript
A Map is a collection and data structure that stores key / value
pairs. A Map allows you to find an value if you provide the key. Below is an example code of how to set up a Map for storing data in JavaScript.
const staff = new Map(); staff.set(1, 'William'); staff.set(2, 'Achebe'); staff.set(3, 'Thomas');
1.3 Reasons for Converting Maps to Arrays
Converting Maps to Arrays can be beneficial for different reasons. Here are some common reasons and scenarios where someone might want to convert a Map to an Array.
- Serialization and Data Exchange: When sending data to a server or storing it locally in local storage or cookies, using arrays can be more convenient, especially if the data needs to be serialized into JSON format.
- Simplified Iteration: Arrays have a more straightforward and familiar iteration mechanism using methods like
forEach
,map
,filter
, etc. Converting a Map to an array allows a developer to leverage these array iteration methods, making the code cleaner and easier to read. - Sorting and Ordering: Arrays are naturally ordered, whereas Maps do not guarantee a specific order. Converting a Map to an array allows you to easily apply sorting algorithms to the data if needed.
- Exporting Data: When exporting data from an application, many formats such as
CSV
andJSON
are typically based on arrays. Converting a Map to an array simplifies the process of exporting data in such formats. - Compatibility with Libraries and APIs: Many JavaScript libraries and APIs expect data in the form of arrays rather than Maps. Converting Maps to arrays enables seamless integration with third-party libraries and APIs.
- Reducing Memory Usage: In some cases, arrays can be more memory-efficient than Maps, particularly if the data is not associated with complex keys or requires a large number of unique keys.
2. Converting Maps to Arrays
You can convert a Map to an Array of Arrays or to an Array of Objects in JavaScript. Listed below are some different approaches.
- Use
Array.from()
- Use the
Spread Operator(...)
withArray.prototype.map()
- Use
Map.prototype.forEach()
loop
2.1 Converting Maps to Array of Arrays
2.1.1 Convert Map to Array of Arrays Using the Array.from() Method
Array.from()
method works on Iterables
such as Map. It returns an array from an iterable object. The returned result would be an array of arrays containing keys and/or values from the Map. Illustrated below is an example code that converts a Map to an Array of Arrays.
const authorsMap = new Map(); // Create a new Map Object authorsMap.set(1, 'William Shakespear'); authorsMap.set(2, 'Chinua Achebe'); authorsMap.set(3, 'Jonathan Swift'); const arr = Array.from(authorsMap); console.log(arr)
The output on a web browser console is
2.1.2 Converting Map Keys to Arrays using Array.from() method
To convert Map keys to an Array, we can use the Array.from()
method to create a new Array[]
and then use the Map.prototype.keys()
method to store the Map keys using the newly created Array. Below is an example code that converts Map keys to an Array using the Array.from()
method with the Map.prototype.keys
const authorsMap = new Map(); // Create a new Map Object authorsMap.set(1, 'William Shakespear'); authorsMap.set(2, 'Chinua Achebe'); authorsMap.set(3, 'Jonathan Swift'); //Return the Array let authorArray = Array.from(authorsMap.keys()); console.log(authorArray);
The output of the newly created Array on a web browser console is
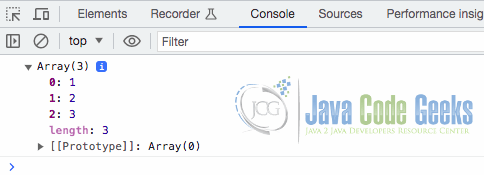
2.1.3 Converting Map Values to Arrays using Array.from() method
To convert Map values to Array, We can use the same method above, but this time we would use the Map.prototype.values()
method to store the values in the newly created Array. Below is the change to the example code above
let authorArray = Array.from(authorsMap.values());
The output on a web browser console is shown below
2.2 Using the Spread Operator(…) with Array.prototype.map()
We can use the JavaScript Spread operator(...)
to expand an array into individual array elements. Using the Array.prototype.map()
method with the Spread Operator(...)
, we can take each entry in a map and convert them into a set of keys
, values
or key/pair
values in an Array.
2.2.1 Convert Map to Array using Spread Operator(…)
Below is an example code to illustrate how to convert a Map to an Array using the Spread Operator
const authorsMap = new Map(); // Create a new Map Object authorsMap.set(1, 'William Shakespear'); authorsMap.set(2, 'Chinua Achebe'); authorsMap.set(3, 'Jonathan Swift'); const arr = [...authorsMap]; console.log(arr);
2.2.2 Convert Map keys to Arrays using Spread Operator (…) and Map.keys() method
The Map.keys()
returns all the keys of a Map in an Iterable form. To Convert Map keys into an array, First, get all the Map keys using Map.keys()
and then use the Spread Operator(...)
to extract all the map keys in an array. The example code shows this
const authorsMap = new Map(); // Create a new Map Object authorsMap.set(1, 'William Shakespear'); authorsMap.set(2, 'Chinua Achebe'); authorsMap.set(3, 'Jonathan Swift'); let keys; //extract all keys from map using Spread Operator(...) keys = [...authorsMap.keys()]; console.log(keys);
2.2.3 Converting Map values to Arrays using Spread Operator (…) and Map.values() method
const authorsMap = new Map(); // Create a new Map Object authorsMap.set(1, 'William Shakespear'); authorsMap.set(2, 'Chinua Achebe'); authorsMap.set(3, 'Jonathan Swift'); let values; //extract all values from map using Spread Operator(...) values = [...authorsMap.values()]; console.log(values);
2.3 Convert Map to Arrays Using the Map.prototype.forEach() method
We can convert Maps to Arrays using the Map.prototype.forEach()
loop. First, we would iterate over the Map object using the forEach iteration method and then use the Array.push() method to add each item’s keys, values, or key/value pairs as an object into the resulting array.
2.3.1 Convert Map Keys and Values to Arrays using Map.forEach() method
The example code below shows how to use the Map.forEach()
method to convert Map keys
and values
to an Array.
const authorsMap = new Map(); // Create a new Map Object authorsMap.set(1, 'William Shakespear'); authorsMap.set(2, 'Chinua Achebe'); authorsMap.set(3, 'Jonathan Swift'); let keys = []; let values = []; authorsMap.forEach((value, key) => { keys.push(key); values.push(value); }); console.log(keys); console.log(values);
The output on a web browser console is
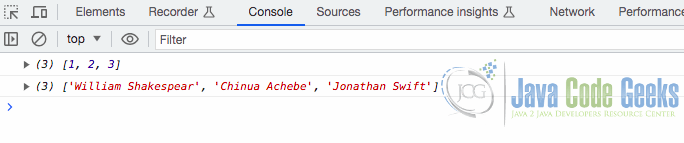
3. Convert Map to Arrays of Objects
We can convert a Map to an array of objects in JavaScript by transforming each key-value
pair in the Map into an object with a key
and value
property.
3.1 Convert Map to Arrays of Object using Array.from() method
To convert a Map
object’s key-value
pairs to an Array []
of objects, we can use the Array.from()
method to create a new Array and then use the arrow function
in JavaScript to store each key-value
pair in that Array like this
const authorsMap = new Map(); // Create a new Map Object authorsMap.set(1, 'William Shakespear'); authorsMap.set(2, 'Chinua Achebe'); authorsMap.set(3, 'Jonathan Swift'); //Return the Array authorsArray = Array.from(authorsMap, ([key, author]) => ({key, author})); console.log(authorsArray);
The corresponding output on a web browser console is
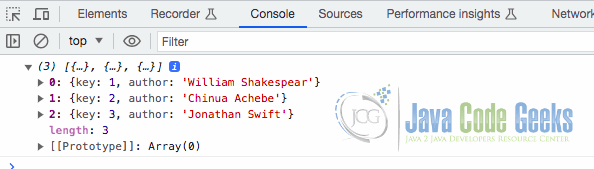
3.2 Convert Map to Arrays of Object using Spread Operator (…) with Array.prototype.map() method
To transform an array of arrays to an array of objects using the Spread Operator(...)
, we can use the Array.prototype.map()
method on the resulting array as shown below
const authorsMap = new Map(); // Create a new Map Object authorsMap.set(1, 'William Shakespear'); authorsMap.set(2, 'Chinua Achebe'); authorsMap.set(3, 'Jonathan Swift'); const authorsArray = [...authorsMap].map(([key, value]) => ({ key, value })); console.log(authorsArray);
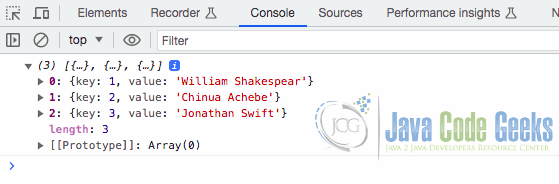
3.3. Convert Map to Arrays of Object using Map.forEach() method
To convert a Map to an array of objects using the Map.forEach()
method, we can iterate over the Map’s key-value
pairs and construct objects with key
and value
properties as illustrated with the code below
const authorsMap = new Map(); // Create a new Map Object authorsMap.set(1, 'William Shakespear'); authorsMap.set(2, 'Chinua Achebe'); authorsMap.set(3, 'Jonathan Swift'); authorsArray = []; // Convert Map to Array of Objects using Map.forEach() authorsMap.forEach( (author, key) => authorsArray.push( {key, author})); // Output console.log(authorsArray);
4. Conclusion
In this blog post, we discussed some reasons and scenarios why developers would consider converting a Map to an Array in JavaScript. We explored different methods to convert Maps to Arrays in JavaScript. We discussed using the Array.from()
method, the Spread Operator(...)
method, and the Map.forEach()
method. We used the Map.values()
and the Map.keys()
method to get the Map’s values and keys. In conclusion, converting a Map to an array empowers developers to handle data more effectively and take advantage of existing JavaScript array methods.