Java throw Exception Example
In this post, we feature a comprehensive Java throw Exception Example.
1. What is an Exception in Java?
Exception is mechanism which Java uses to handle any unforeseen use-case/scenario. Basically an exception is thrown when either something unexpected happened during code execution which is not covered in any code block(converting some null value to lowercase()
) or some IO operation communicating with some third party resource(reading a non existent file).
2. Types Of Exceptions in Java
Exceptions are broadly divided into 2 categories.
2.1 Checked Exception
Checked Exceptions are the exceptions which are notified at the compilation step of the Java program. They are also known as the compile-time exceptions. For these kinds of exceptions, the onus of handling lies on the programmer.
Following code snippet shows the example of the checked exception.
CheckedException.java
public class CheckedException {
public static void main(String[] args) {
try {
int a = 2 / 0;
} catch (Exception e) {
System.out.println("Cannot divide a number with zero.");
}
}
}
the output of the above code is shown in Fig. 1 below.

2.2 Unchecked Exception
An Unchecked Exception occurs at the runtime of the java code.These are called Runtime Exceptions. For these kinds of exceptions, the onus of handling lies on the programmer.
Following code snippet shows an example of Unchecked Exceptions
UnCheckedException.java
public class UnCheckedException {
public static void main(String args[]) {
int num[] = {1, 2, 3, 4};
System.out.println(num[5]);
}
}
the output of above code is shown in the Fig. 2 below.

Above mentioned error can be handled using the try-catch as shown in code snippet below.
UnCheckedExceptionWithTryCatch.java
public class UnCheckedExceptionWithTryCatch {
public static void main(String[] args) {
try{
int num[] = {1, 2, 3, 4};
System.out.println(num[5]);
}catch (Exception e){
System.out.println("Index out of bound");
}
}
}
The output of the above code can be shown in fig. 3 below.

3. Exception Hierarchy
Exceptions follow a certain hierarchy while dictates how exceptions are resolved. Following diagram in Fig. 4. show the hierarchy.
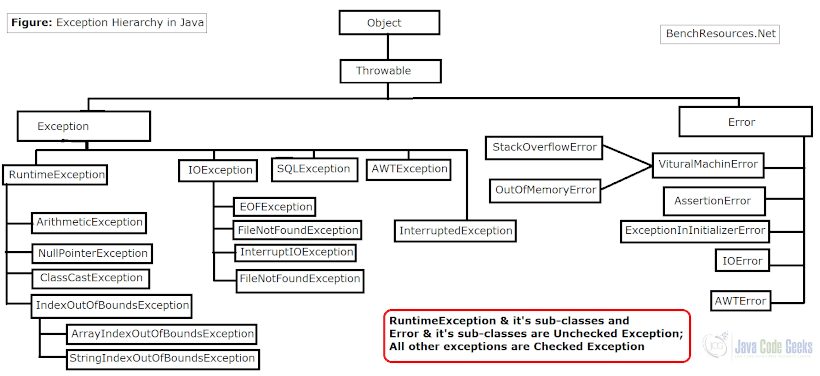
4. Methods in Exception Class
Following are the most commonly used methods of the Exception class.
- public String getMessage()
Returned the detailed message about what caused the exception that has occurred. - public Throwable getCause()
Returned the cause of the Exception in the form of Throwable Object. - public String toString()
Return the name of the class concatenated with the result of thegetMessage()
. - public void printStackTrace()
Print the output of the Java Error stream,System.err
, along with the output of thetoString()
function. - public StackTraceElement [] getStackTrace()
Return an array containing containing all the method calls which resulted in error, with element on index 0 represent the last method invoked before error and element on last index represent the method at the bottom of the call stack. - public Throwable fillInStackTrace()
Fills the stack trace of this Throwable object with the current stack trace, adding to any previous information in the stack trace.
5. Exception Keywords
There are couple of Java Keywords which are used extensively in Exception Handling.
- try:
try
keyword is used to specify a block where we should place exception code. The try block must be followed by either catch or finally. It means, we can’t use try block alone.. - catch
catch
code block contains the code sequence which should execute once exception has occurred. - finally
finally
code block contains the code which will execute whether the exception has occurred or not. - throw
throw
keyword is used to explicitly throw an exception from the code flow. - throws
throws
keyword is used to declare exceptions. It doesn’t throw an exception. It specifies that there may occur an exception in the method. It is always used with method signature.
6. Exception Handling with Multiple Catch Blocks
Now we will discuss a code example with the multiple catch
blocks. The idea is to handle more than one exceptions for a single try
block.
CatchMultipleException.java
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class CatchMultipleException {
public static void main(String[] args) {
try {
FileInputStream file = new FileInputStream("");
byte x = (byte) file.read();
} catch (FileNotFoundException f) {
f.printStackTrace();
} catch (IOException i) {
i.printStackTrace();
}
}
}
The output of the above code can be shown in Fig. 5 below.
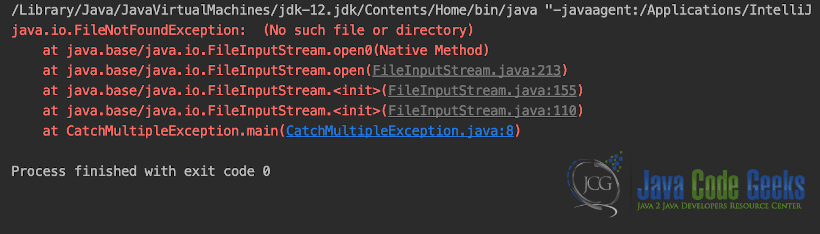
In the above code example there were 2 possibilities of the Java exceptions which could be thrown. One is IOException
and other is FileNotFoundException
. FileNotFoundException
is the Specific one and IOException
is the generic one, which means that the IOException
is parent class of FileNotFoundException
. If we put the catch block of IOException
above the FileNotFoundException
then there will be a compilation failure. Compilation failure will be there because we are catching the Exception of parent class (IOException
) before the exception of the child Class (FileNotFoundException
).
7. Exception Handling with Custom Exception Class
In this example, we feature a comprehensive Java throw Exception Example. We are going to demonstrate how to catch multiple exceptions, using a try-catch
block. To catch multiple exceptions we have followed the steps below:
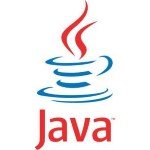
- We have created a method,
checkPass(String pass)
that reads a String password and throws aNoPassException
if the password is null and aShortPassException
if the password’s length is shorter that a specific min length. NoPassException
class extends the Exception and uses the Exception’s constructors in its constructors. It is the exception thrown for no password.ShortPassException
class extends the Exception and also uses the Exception’s constructors in its constructors. It is the exception thrown for short password.- We invoke the
checkPass(String pass)
method in atry-catch
block, using the two exceptions in the catch keywords. - There is also a
finally
keyword, whose block will always be executed.
Let’s take a look at the code snippet that follows:
CatchMultipleExceptionWithCustomException.java
public class CatchMultipleExceptionsWithCustomException { public static void main(String[] args) { // We demonstrate with a short password try { CatchMultipleExceptions.checkPass("pass"); } catch (NoPassException e) { e.printStackTrace(); } catch (ShortPassException e) { e.printStackTrace(); } finally { System.out.println("Finally block is always executed"); } // We demonstrate with no password try { CatchMultipleExceptions.checkPass(null); } catch (NoPassException e) { e.printStackTrace(); } catch (ShortPassException e) { e.printStackTrace(); } finally { System.out.println("Finally block is always executed"); } // We demonstrate with valid password try { CatchMultipleExceptions.checkPass("123456"); System.out.println("Password check : OK"); } catch (NoPassException e) { e.printStackTrace(); } catch (ShortPassException e) { e.printStackTrace(); } finally { System.out.println("Finally block is always executed"); } } // Our business method that check password validity and throws NoPassException and ShortPassException public static void checkPass(String pass) throws NoPassException, ShortPassException { int minPassLength = 5; if (pass == null) throw new NoPassException("No pass provided"); if (pass.length() < minPassLength) throw new ShortPassException("The password provided is too short"); } } //A custom business exception for no password class NoPassException extends Exception { NoPassException() { } NoPassException(String message) { super(message); } NoPassException(String message, Throwable cause) { super(message, cause); } } // A custom business exception for short password class ShortPassException extends Exception { ShortPassException() { } ShortPassException(String message) { super(message); } ShortPassException(String message, Throwable cause) { super(message, cause); } }
This was an example of Java throw Exception. Now you know how to catch multiple exceptions, using a try-catch
block in Java.
The output of the above code can be shown in Fig. 6 below.
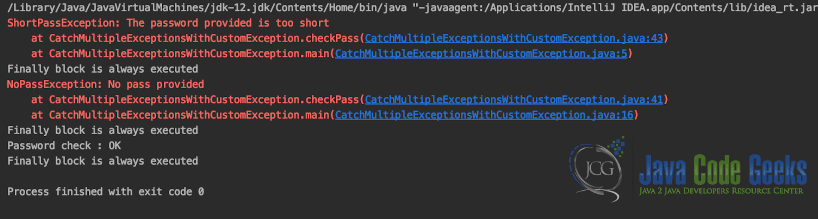
8. Best Practices for Exception Handling
Exception handling is quite complex and troubles newbies and seasoned developers alike. But over the years with countless trial and errors there are few practices which have been developed and used by java developers across the world. Some of those are
- Always clean up the resources in the finally block.
In your code if have any IO connections open, use thefinally
block to close them. - Always prefer specific Exceptions over generic ones
This is good practice for development. If a generic exception is thrown it would be difficult to debug as the generic exception more than one error scenario. - Document the Custom Exception
Documenting theCustom Exception
with details and specify the use-case associated with each of them. It will help in the debugging the code. - Use Descriptive message while throwing Exception.
Always set precise messages with each thrown exception.The exception’s message gets read by everyone who has to understand what had happened when the exception was reported in the log file or your monitoring tool. - Catch the Most Specific Exceptions first
Always catch the most specific exception class first and add the less specific catch blocks to the end of your list. you can see the example of the same inCatchMultipleException.java
.
9. Download the Source Code
You can download the full source code of this example here: Java throw Exception Example
Last updated on Aug 22, 2019