Java Set to List Example
This article is about a Java Set To List Example. Here, we introduce Set & List interfaces and demonstrate a few examples on how implementations of Set in Java can be converted to List in JDK 8.
1. Introduction
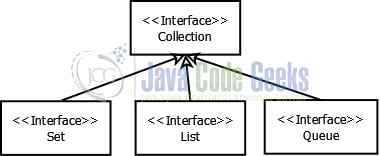
Java Collections Framework provides data structures to store and manipulate a group of Objects. The group of Objects is called a Collection. Typically operations on a Collection are
- Adding an element to Collection
- Removing an element from Collection
- Random access
- Sequential access of all the elements present in the Collection (aka iteration)
Interface Set<E> and Interface List<E> are part of the Java Collections framework, which was introduced in JDK 1.2. The Java Collection framework provides a set of Interfaces and Classes to work with a group of Objects as a single unit. Both Set and List implement the interfaces java.util.Collection<E> and java.util.Iterable<E>. Here E is the type of element that is stored in the Collection.
2. Set
A Set is a Collection that contains Unique elements. It doesn’t allow us to add duplicate entries. This duplicate check condition is enforced on a Set using equals and hashCode methods.
HashSet and TreeSet are the most frequently used implementations of Set. Other concrete implementations of Set are EnumSet, LinkedHashSet, ConcurrentSkipListSet , CopyOnWriteArraySet
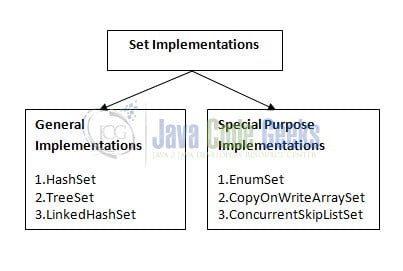
3. List
A List is a Collection that contains Ordered elements. It allows duplicate entries. It maintains the insertion order. It allows positional access of elements using index.
ArrayList and LinkedList are widely used List implementations. Vector and Stack are other Lists.
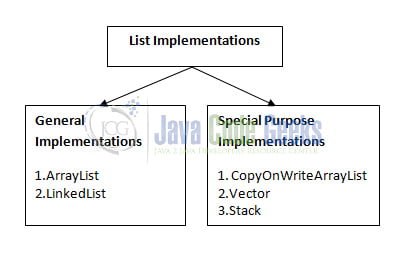
4. Streams and Collections
Java 8 has introduced Streams. A Stream is a representation of a sequence of elements. A Stream can take Collection as its source of data. It can also consume data from arrays, I/O resources, etc.
A Collection represents a group of elements. Streams let you apply SQL-like aggregate operations on the elements. Please refer here for more detailed information on Streams.
5. Converting a Set to List
Whenever finding an element from the collection is the primary operation, Set is preferred over List. Whenever iteration of elements is the primary operation, a List is preferred over Set. Here, we shall discuss some of the many ways where a Set is converted to List.
- By passing the Set as an argument to List’s constructor
- By passing Set as an input to addAll() method of the List
- Represent a Set as a stream and then collect it as a List
6. Java Set to List Example: Initialising List using a Set
In the following example, a Set is passed as an argument to
List when an object of the List is created.
SetExamples.java
public static void main(String[] args){ Set<String> countrySet = new HashSet<>(); countrySet.add("IN"); countrySet.add("HK"); countrySet.add("SG"); Iterator<String> itr = countrySet.iterator(); while(itr.hasNext()){ System.out.printf("%s ",itr.next()); } List<String> countryList = new ArrayList<>(countrySet); //Iterating the list Iterator<String> listItr = countryList.iterator(); System.out.println("\nList Iteration :"); while(listItr.hasNext()){ System.out.printf("%s ", listItr.next()); } }
In the above example, Line 2 creates a new instance of HashSet whose elements would be of type String. In Line 3 through 5, values are added to the Set object, countrySet
using add()
method. In Line 7, an Iterator object is obtained on the Set. Using the Iterator, the elements in the Set are traversed one by one. The Iterator is used to traverse objects forward only. This means, it can access the next element but not the previous one, once iterated. The hasNext()
method on the iterator returns true until the Set has no more elements to traverse. In Line 9, the next()
method returns the next element on the iteration. Thus using Iterator, we have retrieved the elements in the Set one by one. This is similar to a cursor in the Database context.
In Line 12, an Object of ArrayList is created, by passing the set countrySet
as an argument. The List countryList
is now created with an initial set of values present in the countrySet
.
In Line 15, an Iterator is created on countryList
. Then the elements in the countryList
are iterated in the same fashion as we did on the countrySet.

Iteration on List can also be done using ListIterator. Please refer the following example. Iteration is done using a ListIterator instead of Iterator. LisIterator allows bi-directional access of elements.
TestSetExample.java
public class TestSetExample { public static void main(String[] args){ Set<String> countrySet = new HashSet<>(); countrySet.add("IND"); countrySet.add("KE"); countrySet.add("SGP"); Iterator<String> itr = countrySet.iterator(); while(itr.hasNext()){ System.out.println(itr.next()); } List<String> countryLinkedList = new LinkedList<>(); countryLinkedList.addAll(countrySet); ListIterator<String> listItr = countryLinkedList.listIterator(); System.out.println("Linked List Iteration"); while(listItr.hasNext()){ System.out.println(listItr.next()); } List<String> countryArrayList = new ArrayList<>(); countryArrayList.addAll(countrySet); ListIterator<String> arrlistItr = countryArrayList.listIterator(); System.out.println("Array List Iteration"); while(arrlistItr.hasNext()){ System.out.println(arrlistItr.next()); } } }
7. Set to List Example: Adding a Set to a List
List<String> countryLinkedList = new LinkedList<>(); countryLinkedList.addAll(countrySet);
In the preceding example, a LinkedList is created in the first step. Then, it is initialized using the countrySet
via addAll()
method.
The addAll()
method accepts a Collection<? extends E>
as an argument. Here E
is the type of Object in the LinkedList declaration. Here, in List<String>
, String
Object type replaces E. The addAll()
method, adds all the elements of the countrySet
to countryList
Please refer to the code snippet below. This is an example of an error.
ErrorExample.java
public class ErrorExample { public static void main(String[] args){ Set<String> countrySet = new HashSet<>(); countrySet.add("IN"); countrySet.add("HK"); countrySet.add("SG"); Iterator<String> itr = countrySet.iterator(); while(itr.hasNext()){ System.out.printf("%s ",itr.next()); } List<StringBuffer> countryList = new ArrayList<>(); countryList.addAll(countrySet); //Compilation Error Iterator<StringBuffer> listItr = countryList.iterator(); System.out.println("\nList Iteration :"); while(listItr.hasNext()){ System.out.printf("%s ", listItr.next()); } System.out.println(); } }
The above code will show a compilation error. This is because, on Line 4, HashSet is created with String
as elements. On Line 14, ArrayList is created with StringBuffer
as elements. On Line 15, we are trying to add a Set of String
elements to a List of StringBuffer
elements. Hence the error. Why is it? Recollect the method signature. boolean addAll(Collection<? extends E> c)
. String
does not extend StringBuffer
. Hence this leads to incompatibility between the collections countrySet
and countryList
.
8. Set to List Example: Set to Stream and Stream to List
List<String> countryList = countrySet.stream().collect(Collectors.toList());
List<String> countryList = countrySet.stream().collect(Collectors.toCollection(ArrayList::new));
In the preceding two examples, the Set countrySet
is converted to a Stream using stream()
method of Collection.
In short, a Stream is a sequence of elements in a pipeline, that is designed to support aggregate operations, either sequentially or in parallel. The aggregating operations on the stream of elements may result in a Collection.
In the above example, please note the stream()
method (which is used to convert the Set to a Stream), is a method of Collection interface. This means that any Collection can be converted to a Stream.
The collect()
method on the Stream is a type of reduction operation on the stream of objects, which in turn returns a collection instead of a single value.
In the above examples, the Set is processed as a Stream. An aggregating operation may be applied to all of the objects on the underlying Set. The resulting objects are collected in a List.
9. Download the Source Code
You can download the full source code of this example here: Java Set To List Example