Java Naming Conventions
In this example, we will be discussing naming code conventions for the Java Programming Language. We will talk about different identifier types and the best practices to follow while naming them in your program.
1. Introduction
As a team member of a codebase of any size (small, medium, or large), it is the responsibility of the programmer or the developer to write code that is readable, maintainable, and easy to understand.
Martin Fowler says:
Any fool can write code that a computer can understand. Good programmers write code that humans can understand.
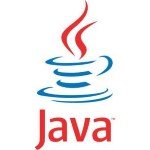
I am sure that after following the naming conventions explained in this article, you can write clean, readable, and easy to maintain code. These conventions are guidelines or recommendations which should be followed when programming in java. Following is a deep dive.
2. Java Naming Conventions
Let’s discuss various identifier types in Java and their naming conventions in detail.
2.1 Packages
Package name should be a dot (.) separated set of words, unique, consisting of lower cases ASCII letters. Underscores can also be used.
- If you are working for an enterprise, use the company’s internet domain name in a reversed manner. For example, for the domain it.cornell.edu, the package name should be edu.cornell.it.[mypackagename] and for cloud.oracle.com it should be com.oracle.cloud.[mypackagename].
- The project name may be added to the package name following Step 1. Usage of such words helps in resolving name collisions in a big enterprise across different projects/teams. For example, com.oracle.cloud.compute.[mypackagename] where the word “compute” represents the project name.
- Further, as per the company’s internal naming conventions, the division, the business unit, the department, or any internal name may also be used to write the subsequent components of the package name.
Package name examples
package com.google.docs.core package com.oracle.cloud.analytics package org.jcg.examples.java.namingconventions
2.2 Classes
- Use nouns in Title Case to represent Class names.
- The first letter of each word must be capitalized.
- Try to avoid acronyms and abbreviations.
- The class name represents an object so keep it simple and descriptive.
Class name examples
public class Car {...} public class CarService {...} public class CarFactory {...} public class Driver {...}
2.3 Interfaces
- For interfaces, follow the same capitalization naming convention as in class names.
- Whenever an Interface offers a capability, end the name with “able“. For example, the Cloneable, the Serializable, and the Comparable interfaces in Java follow such code conventions.
Interface name examples
public interface Vehicle {...} public interface VehicleRepository {...} public interface VehicleService {...}
2.4 Methods
- Method names describe an action taken by an object on its state. Hence, it’s recommended to use verbs for naming methods.
- The first letter of the method name should be a lowercase letter and each subsequent internal word’s first letter should be capitalized.
Method name examples
public void wash(Vehicle vehicle) {...} public Balancing calculateWheelBalancing(Tyre[] tyres) {...}
2.5 Variables
Variables can be classified into static, instance, local variables, and method parameters. Let’s discuss the naming conventions for each of them.
2.5.1. Instance and Static Variables
- Use nouns for instance and static variable names.
- Follow the same capitalization naming convention as in method names.
- Do not start these names with an _(underscore) or a $ (dollar sign), even though both are allowed.
2.5.2 Parameter and Local Variables
- The use of descriptive lowercase single word is advisable for parameter and local variable names.
- If you wish to use multiple words, follow the same capitalization naming convention as in method names.
- Temporary variable names for integers should be single characters e.g. i, j, and k.
Variable name examples
// instance & static variables private String engineName; public static int gears; // parameter & local variables public void calculateMaximumSpeed(float engineDisplacement, int numberOfGears) { int maximumSpeed = 0; for (int i = 0; i < numberOfGears; i++) { } }
2.6 Constants
- Use all uppercase letters for naming constants.
- If there are multiple words, separate them using underscores.
Constants name examples
public static final int MAX_FILE_SIZE = 8192;
2.7 Enumeration and Annotations
- Follow the same naming conventions as in Class names for Enumerations. The set of choices of an enumeration should be all uppercase letters.
- Annotation names should also follow the Title Case notation as in Class names. Based on the requirements, use an adjective, verb, or a noun.
Enumeration and Annotation name examples
public enum Status {SUCCESS, ERROR, WARNING, PENDING , COMPLETED} @Target(ElementType.METHOD) @Retention(RetentionPolicy.SOURCE) public @interface Override { }
2.8 Generic Types
- The letter ‘T’ is typically recommended for Generic type parameter names.
- The classes in Java Development Kit use the letter ‘E’ for collection elements, ‘S’ for service loaders, and ‘K’ and ‘V‘ in case of Maps.
Generic Types name examples
public interface Map { V put(K key, V value); V get(Object key); ... } public interface Comparable { public int compareTo(T o); }
3. Summary
In this article, we explained in detail the naming code conventions someone should follow when programming in Java. These are one of the best coding practices that result in a clean, readable, and easy to understand code.
4. Download the source code
You can download the full source code of this example here: Java Naming Conventions
Nice and useful information !