Java Max Int Example
In this article, we will implement a Java example to see how we can get the int (integer) max value in a program.
1. Introduction
Int is one of the most popular primitive data types of java and we use it almost everywhere. This article explains technical specs about max int.
2. Java Max Int
According to java, int data type is 32-bit signed two’s complement integer, which has a range from [ -2^31,2^31-1].
Create a new class with name MaxIntegerExample1
and copy-paste the following code :
MaxIntegerExample1.java
package com.javacodegeeks; /** * @author Petros Koulianos * */ public class MaxIntegerExample1 { public static void main(String[] args) { // we use Integer wrapper class int max_int = Integer.MAX_VALUE; int min_int = Integer.MIN_VALUE; System.out.println( "Int MAX_VALUE: "+max_int); System.out.println( "Int MIN_VALUE: "+min_int); } }
Output
Int MAX_VALUE: 2147483647 Int MIN_VALUE: -2147483648
The above code snippet prints in console the max and min value of int with the help of the Integer class constants.
3. Int background
This section explains why the integer variable has max value 2^31-1 and min value -2^31.
Java stores integer sign numbers in bits with two’s complement representation. Since int has 32 bits and two’s complement representation the first bit indicates the sign of the number ( 0 = positive, 1 = negative ) and uses the rest 31 bits to store the number.
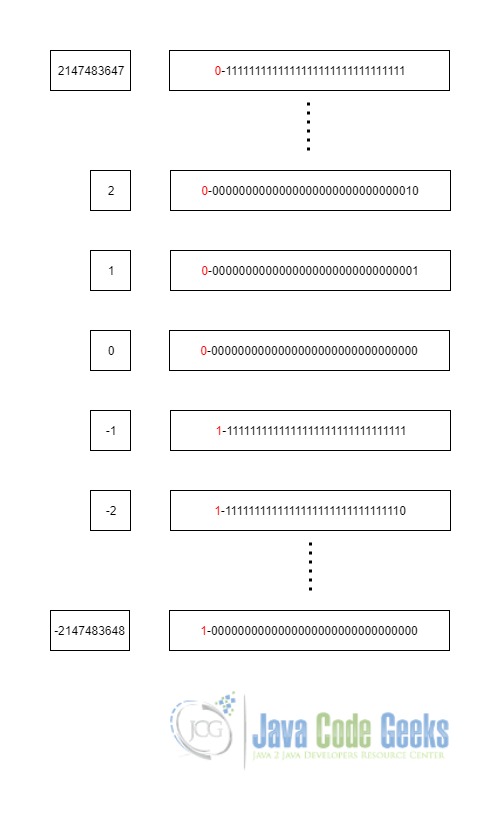
The max positive int number in binary is 0-111111111111111111111111111111 and in decimal is 2147483647 = 2^31-1. Consequently, the max integer positive number equals +2^31-1.
The min negative int number in binary with two’s complement representation is 1-0000000000000000000000000000000, and converting to normal form we get 1-1111111111111111111111111111111 = 2147483648 = 2^31. Consequently, min integer negative number equals -2^31.
Create a new class with name MaxIntegerExample2
and copy-paste the following code :
MaxIntegerExample2.java
package com.javacodegeeks; /** * @author Petros Koulianos * */ public class MaxIntegerExample2 { public static void main(String[] args) { System.out.println("(decimal) "+Integer.MAX_VALUE+" | (binary) "+Integer.toBinaryString(Integer.MAX_VALUE)); System.out.println("------------------------"); System.out.println("(decimal) "+2+" | (binary) "+Integer.toBinaryString(2)); System.out.println("(decimal) "+1+" | (binary) "+Integer.toBinaryString(1)); System.out.println("(decimal) "+0+" | (binary) "+Integer.toBinaryString(0)); System.out.println("(decimal) "+-1+" | (binary) "+Integer.toBinaryString(-1)); System.out.println("(decimal) "+-2+" | (binary) "+Integer.toBinaryString(-2)); System.out.println("------------------------"); System.out.println("(decimal) "+Integer.MIN_VALUE+" | (binary) "+Integer.toBinaryString(Integer.MIN_VALUE)); } }
Output
(decimal) 2147483647 | (binary) 1111111111111111111111111111111 ------------------------ (decimal) 2 | (binary) 10 (decimal) 1 | (binary) 1 (decimal) 0 | (binary) 0 (decimal) -1 | (binary) 11111111111111111111111111111111 (decimal) -2 | (binary) 11111111111111111111111111111110 ------------------------ (decimal) -2147483648 | (binary) 10000000000000000000000000000000
Τhe above code example print in console positive and negative integers , in decimal and binary form.
4. Download the Source Code
This was an example about integer max value in Java.
You can download the full source code of this example here: Java Max Int Example
The only thing I would add is not to depend on the fact that Integer.MAX_VALUE and Integer.MIN_VALUE have the exact values 2147483647 and -2147483648 respectively.
While it might be unlikely, it’s possible that these values could change in the future if the underlying integer representation changes – for instance, going to 64 bit integers or (gasp!) going down to 16 bit integers. Or even, maybe sometime in the far future, going to some system not dependent on bits at all.
Just use Integer.MAX_VALUE and Integer.MIN_VALUE to abstract away the largest and smallest possible Integer values.