Java Implements Keyword Example
1. Introduction
This article introduces the implements keyword, the need, and its usages in Java. It starts with a short introduction and explains it with the help of an example.
2. What is the Java Implements keyword
Java is an Object-Oriented Programming language. Like any other OOP language, Java supports inheritance, the essential feature for code re-use. Java does not support extending multiple base classes. However, we need any object to assume more than one form (polymorphism) when interacting with many objects to suit the context. This is when the “implements” keyword comes into rescue.
For information on interfaces refer to Java documentation.
In this post, we will take a real-world problem and try to solve using implements.
3. Pizza Restaurant – the story
Let us consider an example of a pizza restaurant that uses a vending machine to deliver pizzas. A vending machine is required to prepare different varieties of pizzas including thin-crust pizza, thick-crust, and cheese-blast pizza.
To make it more interesting, let us consider we need the vending machine to prepare different country-specific versions of pizzas including Greek Pizza, and California Pizza to start with.
4. Example of implements keyword
4.1 Interfaces
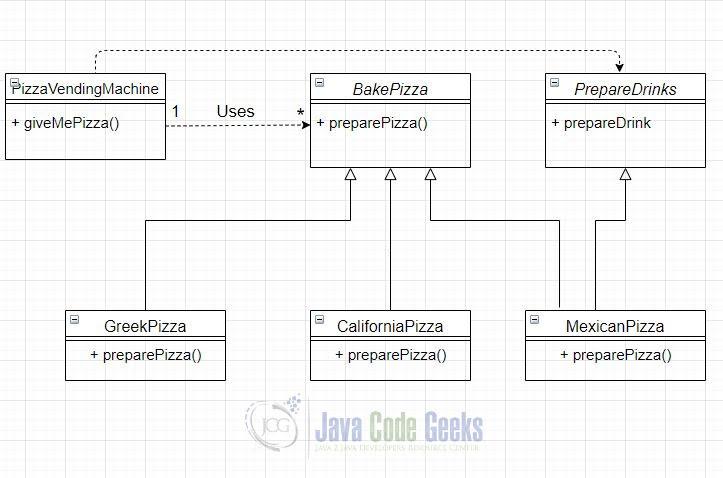
In the context of pizza above, a pizza vending machine should assume a pizza maker for pizza lovers and a drink maker for the drinks.
Hence, we can think about an interface BakePizza
which lists all the methods to bake a pizza. Here is the code for BakePizza interface:
BakePizza.java
package com.javacodegeeks.examples; /** * This is the interface for baking pizzas. * * @author Shivakumar Ramannavar */ public interface BakePizza { void preparePizza(String pizzaType, String ... ingredients); }
We can also think of another interface to serve a drink. Any machine capable of preparing a drink must call the implementations of the PrepareDrink
interface.
PrepareDrinks.java
package com.javacodegeeks.examples; /** * This is the interface for preparing drinks. * * @author Shivakumar Ramannavar */ public interface PrepareDrinks { void prepareDrink(String drinkTtype); }
4.2 Implementations of BakePizza
As per the story, the vending machine is required to make different kinds of Pizzas. Hence, here are the implementations of BakePizza
:
GreekPizza.java
package com.javacodegeeks.examples; /** * This is the Greek-style implementation of Bake Pizza interface. * * @author Shivakumar Ramannavar * */ public class GreekPizza implements BakePizza { /* (non-Javadoc) * @see com.javacodegeeks.examples.BakePizza#preparePizza(java.lang.String, java.lang.String[]) */ @Override public void preparePizza(String pizzaType, String... toppings) { System.out.println("\tPreparing the " + pizzaType + " pizza in a Greek style ..."); System.out.println("\tPutting it into the oven ..."); System.out.println("\tThe pizza is baked!"); System.out.println("\tTake your pizza!\n"); } }
CaliforniaPizza.java
package com.javacodegeeks.examples; /** * This is the California-style implementation of Bake Pizza interface. * * @author Shivakumar Ramannavar */ public class CaliforniaPizza implements BakePizza { /* (non-Javadoc) * @see com.javacodegeeks.examples.BakePizza#preparePizza(java.lang.String, java.lang.String[]) */ @Override public void preparePizza(String pizzaType, String... toppings) { System.out.println("\tPreparing the " + pizzaType + " pizza in a California style ..."); System.out.println("\tPutting it into the oven ..."); System.out.println("\tThe pizza is baked!"); System.out.println("\tTake your pizza!\n"); } }
4.3 Multiple Interfaces
Just as an addition to the story, the restaurant has decided to offer pink lemonade drinks along with the pizzas only for Mexican pizzas. Any machine capable of preparing a drink must call the implementations of the PrepareDrinks
interface.
As the MexicanPizza is capable of preparing both the pizzas and the drinks, the class MexicanPizza
must implement both the interfaces – BakePizza
and PrepareDrinks
. Here is an example of one class implementing multiple interfaces.
Here the MexicanPizza
class implements two interfaces –BakePizza
and PrepareDrink
. Here is the class implementation:
MexicanPizza.java
package com.javacodegeeks.examples; /** * This is the Mexican-style implementation of Bake Pizza interface. * * @author Shivakumar Ramannavar * */ public class MexicanPizza implements BakePizza, PrepareDrinks { /* (non-Javadoc) * @see com.javacodegeeks.examples. * BakePizza#preparePizza(java.lang.String, java.lang.String[]) */ @Override public void preparePizza(String pizzaType, String... toppings) { System.out.println("\tPreparing the " + pizzaType + " pizza in a Mexican style ..."); System.out.println("\tPutting it into the oven ..."); System.out.println("\tThe pizza is baked!"); System.out.println("\tTake your pizza!\n"); } /* * (non-Javadoc) * @see com.javacodegeeks.examples.PrepareDrinks#prepareDrink(java.lang.String) */ @Override public void prepareDrink(String drinkType) { System.out.println("\tPreparing the " + drinkType + " drink in a Mexican style ..."); System.out.println("\tPutting it into the cup ..."); System.out.println("\tTake your drink!\n"); } }
5. Put them into the action
Here is the PizzaVendingMachine
class which simulates customers coming to a Pizza restaurant. To make it simple we have fixed the toppings and the type of pizza crust.
This class comes with giveMePizza()
method which does a series of calls to each of the implementations of the BakePizza
interface.
For the Mexican style of pizza, it simulates the customer ordering pink lemonade and it calls the implementation of the PrepareDrinks
interface. The code for vending machine is as follows:
PizzaVendingMachine.java
package com.javacodegeeks.examples; /** * This class is just a demo to invoke different * implementations of the interfaces. * * @author Shivakumar Ramannavar * */ public class PizzaVendingMachine { public void giveMePizza() { // Make it simple, assume only 3 toppings String[] toppings = new String[]{"Onion", "Tomato", "Capscicum"}; String pizzaType = "Thick Crust"; // First customer orders Greek Thick Crust pizza System.out.println("Customer ordered " + "Greek Thick Crust Pizza"); BakePizza bakePizza = new GreekPizza(); bakePizza.preparePizza(pizzaType, toppings); // The next customer orders California Thick Crust pizza System.out.println("Customer ordered " + "California Thick Crust Pizza"); BakePizza californiaPizza = new CaliforniaPizza(); californiaPizza.preparePizza(pizzaType, toppings); // Initialise to serve Coke by default String choiceOfDrink = "Pink Lemonade"; // The third customer orders Mexican Thick Crust pizza // and a pink lemonade System.out.println("Customer ordered Mexican " + "Thick Crust Pizza and a Pink Lemonade"); BakePizza mexicanPizza = new MexicanPizza(); mexicanPizza.preparePizza(pizzaType, toppings); PrepareDrinks prepareDrinks = new MexicanPizza(); prepareDrinks.prepareDrink(choiceOfDrink); } public static void main(String[] args) { PizzaVendingMachine vendingMachine = new PizzaVendingMachine(); vendingMachine.giveMePizza(); } }
5. Execution
In this section we will execute the programs and see how it is working.
Prerequisites:
- Java 1.7 installed in the system. Environment variables JAVA_HOME set to the Java location and PATH set to the directory containing javac and java binaries ( %JAVA_HOME%/bin on windows or $JAVA_HOME/bin on Linux machines)
- Source code zip and downloaded to a location (say, C:\JavaCodeGeeks. This would be different for Linux)
- Eclipse IDE (Photon Release (4.8.0) is used for this example)
5.1 Execution using eclipse
Step 1: Open the Eclipse IDE.
Step 2: Click On File >> Import.
Step 3: From the “Import” menu select “Existing Projects into Workspace”.
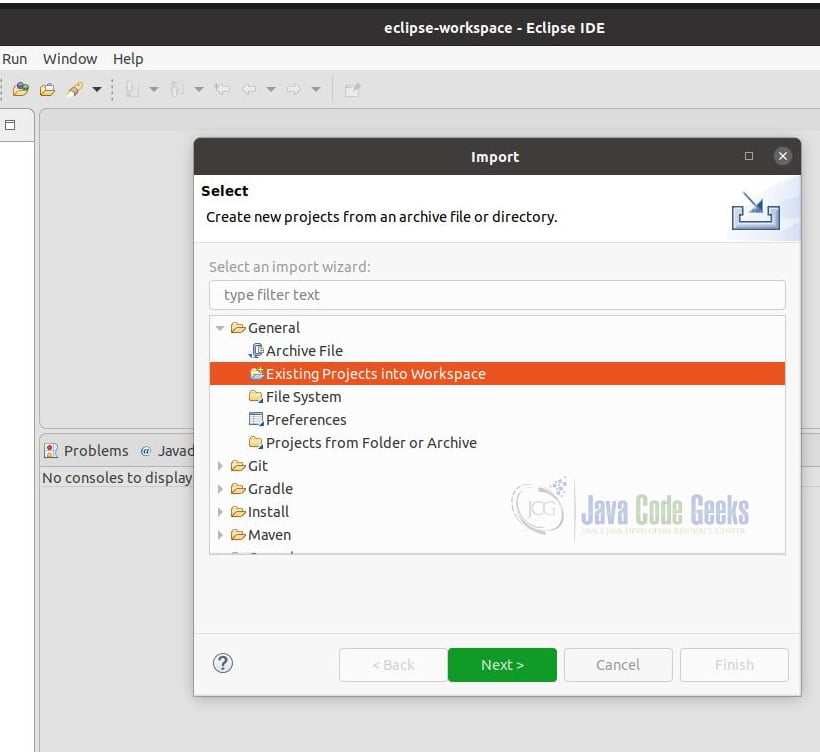
Step 4: Click Next.
Step 5: In the next page, click browse and select the root of the example folder (say, C:\JavaCodeGeeks). Click on “Finish” button.
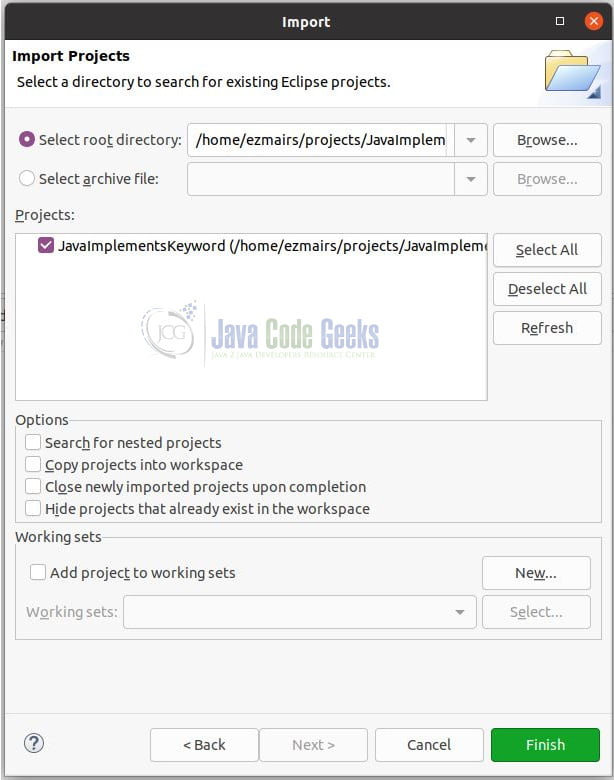
Step 6: Ensure Package Explorer is loaded and lists all the files as shown in the figure below.
Step 7: Click on BakePizza
from the Package Explorer and examine the BakePizza
Interface.
Step 8: Click on GreekPizza
to examine the implements keyword.
Step 9: Click on MexicanPizza
to examine the multiple interfaces
implementation.
Step 10: Right-click on PizzaVendingMachine
, from the menu, choose
“Run As” >> “Java Application”
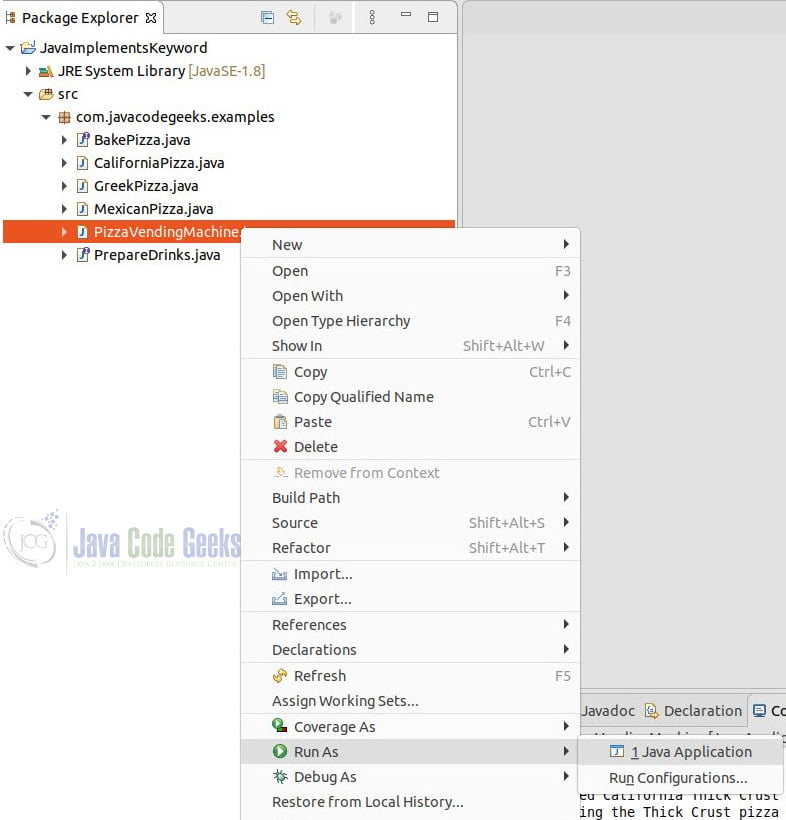
See the sample output as below:
Customer ordered Greek Thick Crust Pizza Preparing the Thick Crust pizza in a Greek style ... Putting it into the oven ... The Pizza is baked! Take your pizza! Customer ordered California Thick Crust Pizza Preparing the Thick Crust pizza in a California style ... Putting it into the oven ... The Pizza is baked! Take your pizza! Customer ordered Mexican Thick Crust Pizza and a Pink Lemonade Preparing the Thick Crust pizza in a Mexican style ... Putting it into the oven ... The Pizza is baked! Take your pizza! Preparing the Pink Lemonade drink in a Mexican style ... Putting it into the cup ... Take your drink!
6. Download the Eclipse Project
That was a tutorial about the Java implements
keyword.
You can download the full source code of this example here: Java Implements Keyword Example
Good try to explain.
Thanks, Nehal! I hope you enjoyed it. Any ideas to make the information interesting are welcome!
Nice Example