Spring Security Roles and Privileges Example
Welcome readers, in this tutorial, we will implement the role-based access in the security module of the spring framework.
1. Introduction
- Spring Boot is a module that provides rapid application development feature to the spring framework including auto-configuration, standalone-code, and production-ready code
- It creates applications that are packaged as jar and are directly started using embedded servlet container (such as Tomcat, Jetty or Undertow). Thus, no need to deploy the war files
- It simplifies the maven configuration by providing the starter template and helps to resolve the dependency conflicts. It automatically identifies the required dependencies and imports them in the application
- It helps in removing the boilerplate code, extra annotations, and xml configurations
- It provides a powerful batch processing and manages the rest endpoints
- It provides an efficient jpa-starter library to effectively connect the application with the relational databases
1.1 Security module of the spring framework
Spring security is a powerful and high customizable authentication and access-control framework. It focuses on,
- Providing authentication and authorization to the applications
- Takes care of the incoming http requests via servlet filters and implements the user-defined security checking
- Easy integration with servlet api and web mvc. This feature provides default login and logout functionalities
Now, open the eclipse ide and let’s see how to implement this tutorial in the spring boot module.
2. Spring Security Roles and Privileges Example
Here is a systematic guide for implementing this tutorial.
2.1 Tools Used
We are using Eclipse Kepler SR2, JDK 8, and Maven.
2.2 Project Structure
In case you are confused about where you should create the corresponding files or folder, let us review the project structure of the spring boot application.
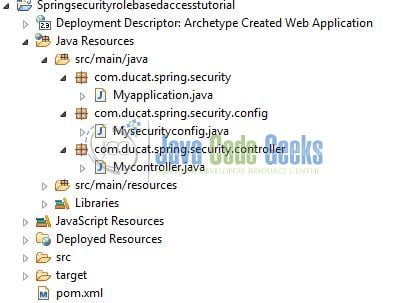
2.3 Project Creation
This section will demonstrate how to create a Java-based Maven project with Eclipse. In Eclipse IDE, go to File -> New -> Maven Project
.
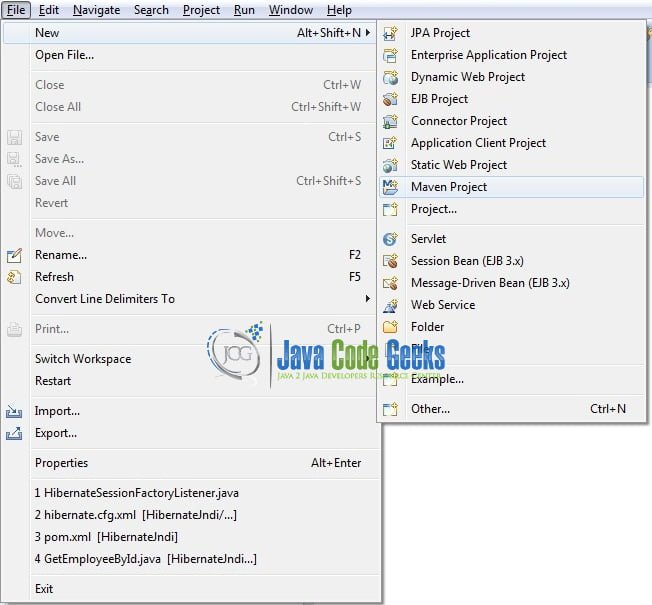
In the New Maven Project window, it will ask you to select a project location. By default, ‘Use default workspace location’ will be selected. Just click on the next button to proceed.
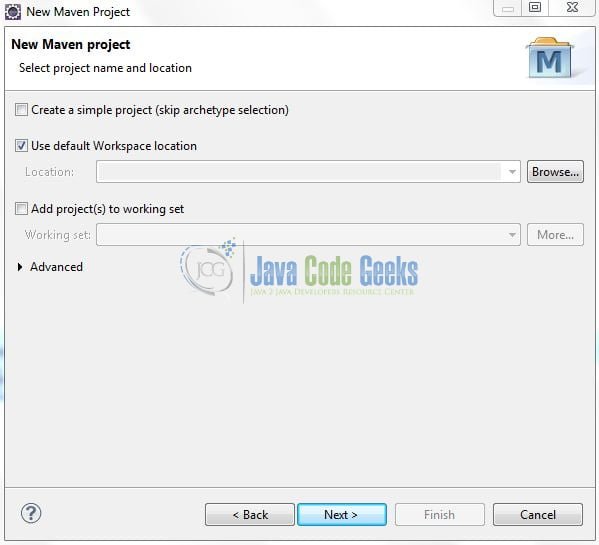
Select the Maven Web App archetype from the list of options and click next.
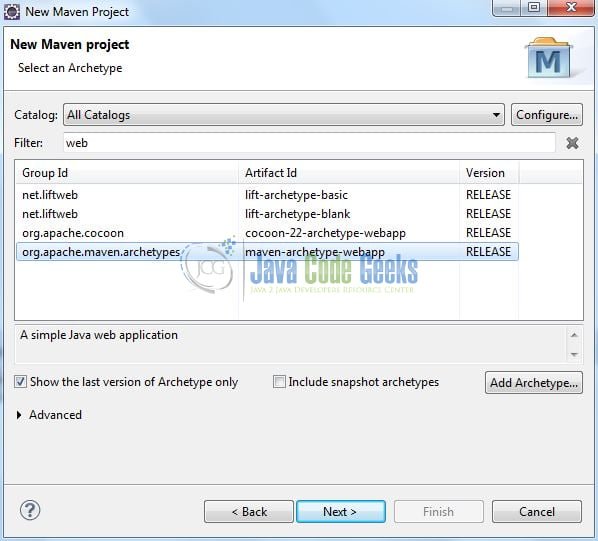
It will ask you to ‘Enter the group and the artifact id for the project’. We will input the details as shown in the below image. The version number will be by default: 0.0.1-SNAPSHOT
.
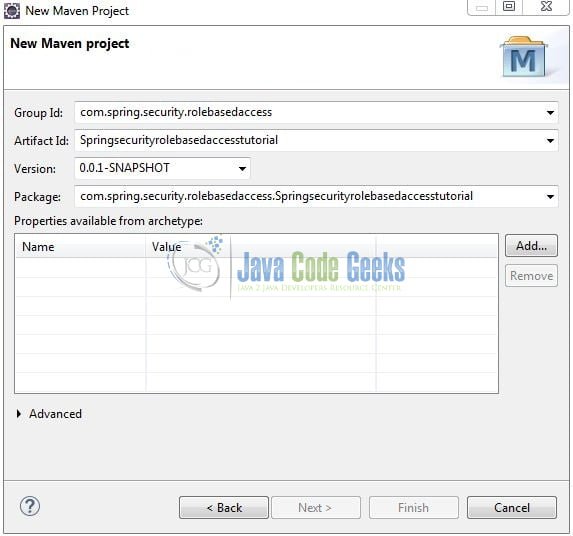
Click on Finish and the creation of a maven project is completed. If you observe, it has downloaded the maven dependencies and a pom.xml
file will be created. It will have the following code:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.spring.security</groupId> <artifactId>Springsecurityrolebasedaccesstutorial</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> </project>
Let’s start building the application!
3. Creating a Spring Boot application
Below are the steps involved in developing the application.
3.1 Maven Dependencies
Here, we specify the dependencies for the Spring Boot and Security. Maven will automatically resolve the other dependencies. The updated file will have the following code.
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.spring.security</groupId> <artifactId>Springsecurityrolebasedaccesstutorial</artifactId> <packaging>war</packaging> <version>0.0.1-SNAPSHOT</version> <name>Springsecurityrolebasedaccesstutorial Maven Webapp</name> <url>http://maven.apache.org</url> <!-- spring boot parent dependency jar. --> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.1.RELEASE</version> </parent> <dependencies> <!-- to implement security in a spring boot application. --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency> <!-- spring boot web mvc jar. --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> </dependencies> <build> <finalName>Springsecurityrolebasedaccesstutorial</finalName> </build> </project>
3.2 Java Classes
Let’s write all the java classes involved in this application.
3.2.1 Implementation/Main class
Add the following code in the main class to bootstrap the application from the main method. Here,
- The entry point of the spring boot application is the class containing the
@SpringBootApplication
annotation and the static main method @EnableWebSecurity
annotation enables web security in any web application
Myapplication.java
package com.ducat.spring.security; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; @SpringBootApplication // Enable spring security java configuration. @EnableWebSecurity public class Myapplication { public static void main(String[] args) { SpringApplication.run(Myapplication.class, args); } }
3.2.2 Security configuration class
Add the following code to the configuration class to handle the role-based access and authentication.
Mysecurityconfig.java
package com.ducat.spring.security.config; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; import org.springframework.security.crypto.password.NoOpPasswordEncoder; @Configuration @SuppressWarnings("deprecation") public class Mysecurityconfig extends WebSecurityConfigurerAdapter { @Override protected void configure(AuthenticationManagerBuilder auth) throws Exception { auth.inMemoryAuthentication().withUser("myadmin").password("password1").roles("ADMIN"); auth.inMemoryAuthentication().withUser("myuser").password("password2").roles("USER"); } // Security based on role. // Here the user role will be shown the Http 403 - Access Denied Error. But the admin role will be shown the successful page. @Override protected void configure(HttpSecurity http) throws Exception { http.csrf().disable(); http.authorizeRequests().antMatchers("/rest/**").hasAnyRole("ADMIN").anyRequest().fullyAuthenticated().and().httpBasic(); } @Bean public static NoOpPasswordEncoder passwordEncoder() { return (NoOpPasswordEncoder) NoOpPasswordEncoder.getInstance(); } }
3.2.3 Controller class
Add the following code to the controller class designed to handle the incoming requests which are configured by the @RequestMapping
annotation.
Mycontroller.java
package com.ducat.spring.security.controller; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @RestController @RequestMapping(value="/rest/auth") public class Mycontroller { // Method will only be accessed by the user who has 'admin' role attached to it. @GetMapping(value="/getmsg") public String getmsg() { System.out.println(getClass() + "- showing admin welcome page to the user."); return "Spring security - Role based access example!"; } }
4. Run the Application
As we are ready with all the changes, let us compile the spring boot project and run the application as a java project. Right click on the Myapplication.java
class, Run As -> Java Application
.
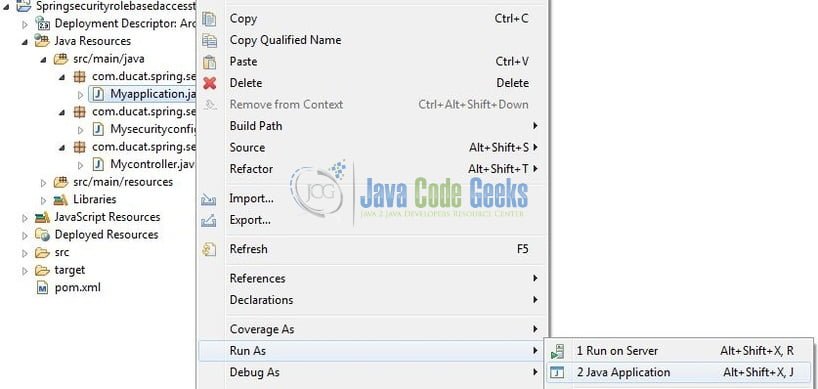
Developers can debug the example and see what happens after every step. Enjoy!
5. Project Demo
Open your favorite browser and hit the following URL to display the authentication prompt.
http://localhost:8082/rest/auth/getmsg
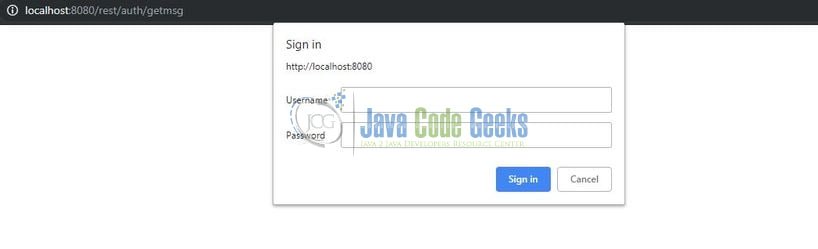
Enter the admin credentials (myadmin/password1) and the secure page will be displayed as shown in Fig. 8.

Access the url again and enter the following credentials (myuser/password1). As the attached role is a user, HTTP 403 error page will be thrown as shown in Fig. 9.
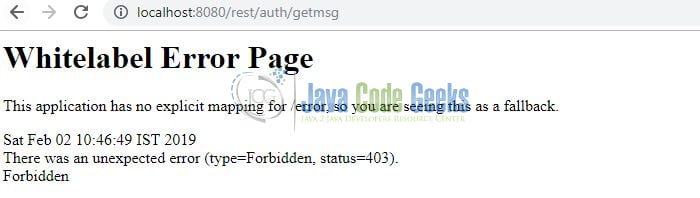
That’s all for this tutorial and I hope the article served you whatever you were looking for. Happy Learning and don’t forget to share!
6. Conclusion
In this section, developers learned how to implement role-based authentication in spring security. Developers can download the sample application as an Eclipse project in the Downloads section.
7. Download the Eclipse Project
This was an example of implementing the role-based authentication in spring security.
You can download the full source code of this example here: Spring Security Roles and Privileges Example
Failed to introspect annotated methods on class org.springframework.security.config.annotation.web.configuration.WebSecurityConfiguration
I just searched and some say ServletRegistrationBean has been changed after Spring 1.5, I tried to downgrade but I am not able to create a project from start.spring.io with a lower spring-boot version ..
Can you please suggest a modification to make this work..
And thank you very much for the article..