Java Comments
Comments in Java are non-executable statements that are used to provide additional information about some piece of code logic, method, or class. In this article, we shall discuss types of comments, and when and how to write them.
1. Comments in Java and their types
Comments are non-executable statements that are provided to understand code better. These are usually provided to explain the logic implemented in a class, method, or function. Comments in Java can be either implementation comments or documentation comments.
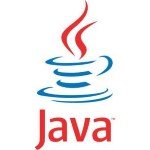
Implementation comments are meant for commenting out code or for comments about particular implementation. Doc comments are meant to describe the specification of code, from an implementation-free perspective, to be read by developers who may not necessarily have source code at hand.
Comments can be single-line or multi-line.
1.1 Single-line comments
These are short comments that can appear on a single line intended to the level of code that follows. If a comment can’t be written in a single line, it should follow block comment (multi-line) format
if (condition) { // handle the below logic for success case ... }
1.2 Multi-line comments
Block comments or multi-line comments are used to provide a description of files, methods, data structures, or algorithms. They are used at the beginning of each file and before each method.
/* * The example class provides.... */
2. When you should write comments and when you shouldn’t
Code changes or evolves over time, but unfortunately, the comments don’t always follow them. These inaccurate comments would then be misleading. Robert.C.Martin in his book Clean Code, mentions – “Truth can only be found in one place: the code.” In his book, Robert talks about why comments are usually failures, as they are not maintained as code evolves. He provides some details on good and bad comments.
2.1 Good comments
Good comments are those that are necessary or beneficial. Some that fall under this category are:
Legal comments: For example, these are comments that contain copyright information. These are necessary and reasonable information to be put into a comment at start of each source file.
Informative comments: It is sometimes useful to provide basic information with a comment.
// Returns an instance of Responder being tested protected abstract Responder responderInstance();
Even in the above case, the comment can be made redundant by renaming the function as responderBeingTested
.
Explanation of intent: Sometimes a comment goes beyond just useful information about the implementation and provides the intent behind a decision.
Warning of consequences: Sometimes it is useful to warn other programmers about certain consequences. For example, a scenario wherein by changing the number of threads can lead to memory or utilization impacts.
2.2 Bad comments
Most comments fall into this category. Usually they are cructches or excuses for poor code or justification for insufficient decisions.
Redundant comments: It is better to avoid where code makes more sense than the comments. In some cases, we notice that the comment takes longer to read than code. Such should be avoided.
Misleading comments: We need to avoid misleading comments. Make sure you don’t comment, unless you feel its accurate and unlikely to change.
Don’t use comment when you can use a function or a variable: Robert.C.Martin mentions that it is always a best practice to explain yourself in code. For example:
// Check to see if the employees is eligible for full benefits if ((employee.flags & HOURLY_FLAG ) && (employee.age>65))
The above code with comment could have been better written with a meaningful function as if (employee.isEligibleForFullBenefits)
Historical discussions in comments: Don’t put historical discussions or irrelevant descriptions of details in comments.
3. Summary
In this article, we discussed comments in Java, and when we should or shouldn’t use them. As a better practice, it is advisable to explain better through code than comments.