Java Collections Tutorial
1. Introduction
A collection is a group of objects and treated as a single unit. It is used to store, retrieve, and manipulate aggregate data. Collections are part of the core java and all java developers must have familiarity with them. Java has provided a collection framework which contains interfaces, classes, and algorithms since version 1.2 and enhanced it over time. The most important interfaces are Java Set, List, and Map. Here are some enhancements:
- Major enhancement at version 5, including generic type.
- Added more interfaces and classes at version 6, including
Deque
,BlockingDeque
,NavigableSet
,NavigableMap
,ConcurrentNavigableMap
, etc. - Added a new
TransferQueue
interface at version 7. Collection
added theforeach(Consumer<? super T>> action)
method at version 8.- Added factory methods for creating immutable lists at version 9, such as
static
of()
method. List
addedstatic
copyOf()
method at version 10.Collection
added a new default methodtoArray(IntFunction<T[]> generator)
at version 11.
You can also check this tutorial in the following video:
In this example, I will create several test classes to demonstrate how to use the most commonly used interfaces: List
, Set
, Queue
and classes: ArrayList
, LinkedList
, Vector
, Stack
, HashSet
, TreeSet
.
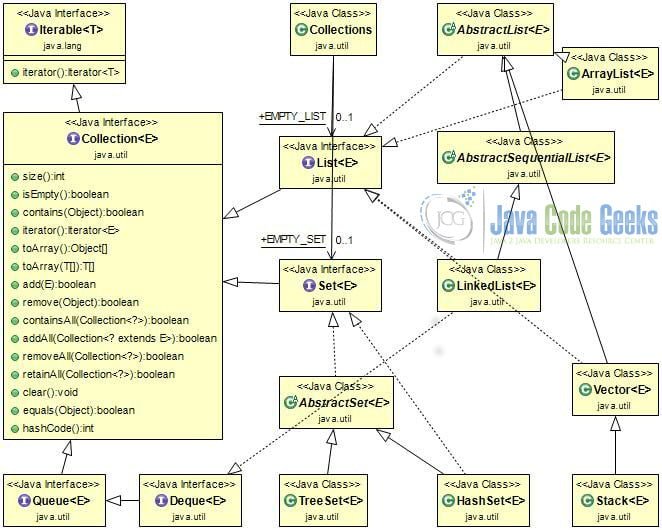
2. Technologies Used
The example code in this article was built and run using:
- Java 11
- Maven 3.3.9
- Eclipse Oxygen
- Logback 1.2.3
3. Maven Project
3.1 Pom.xml
Pom.xml
manages the project dependencies.
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>jcg.zheng.demo</groupId> <artifactId>java-collections-demo</artifactId> <version>0.0.1-SNAPSHOT</version> <build> <sourceDirectory>src</sourceDirectory> <plugins> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.0</version> <configuration> <release>11</release> </configuration> </plugin> </plugins> </build> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> </dependency> <dependency> <groupId>ch.qos.logback</groupId> <artifactId>logback-access</artifactId> <version>1.2.3</version> </dependency> <dependency> <groupId>ch.qos.logback</groupId> <artifactId>logback-classic</artifactId> <version>1.2.3</version> </dependency> <dependency> <groupId>ch.qos.logback</groupId> <artifactId>logback-core</artifactId> <version>1.2.3</version> </dependency> </dependencies> </project>
3.2 SomeData
Java collection classes work with Object, not the primitive data type. In this step, I will create a SomeData
class which implements the Comparable
interface.
SomeData.java
package jcg.zheng.demo.data; import java.io.Serializable; public class SomeData implements Comparable , Serializable { private static final long serialVersionUID = -5243901184267064976L; private int id; private String name; @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null) return false; if (getClass() != obj.getClass()) return false; SomeData other = (SomeData) obj; if (id != other.id) return false; if (name == null) { if (other.name != null) return false; } else if (!name.equals(other.name)) return false; return true; } public int getId() { return id; } public String getName() { return name; } @Override public int hashCode() { final int prime = 31; int result = 1; result = prime * result + id; result = prime * result + ((name == null) ? 0 : name.hashCode()); return result; } public void setId(int id) { this.id = id; } public void setName(String name) { this.name = name; } @Override public String toString() { return "SomeData [name=" + name + ", id=" + id + "]"; } @Override public int compareTo(SomeData other) { int byName = this.name.compareTo(other.getName()); return byName; } }
4. Abstract Test Classes
In this step, I will create three abstract test classes:
CollectionBase_POJOTest
– It demonstrates how toadd
,remove
,clear
,contains
,getSize
,foreach
for aCollection
ofSomeData
objects.CollectionBase_StringTest
– It does the same as above class but forString
objects.ListBase_StringTest
– It extends fromCollectionBase_StringTest
with additional test methods for theList
interface withString
objects.
4.1 CollectionBase_StringTest
The Collection
interface includes methods to add, remove, and retrieve elements from a collection. It checks the element’s existence with the contains
method. In this example, I will create a test class which demonstrates how to use these methods for String
objects.
CollectionBase_StringTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertFalse; import static org.junit.Assert.assertTrue; import java.util.Arrays; import java.util.Collection; import java.util.Iterator; import org.junit.After; import org.junit.FixMethodOrder; import org.junit.Test; import org.junit.runners.MethodSorters; import org.slf4j.Logger; @FixMethodOrder(MethodSorters.NAME_ASCENDING) public abstract class CollectionBase_StringTest { protected Collection<String> colItems; protected Logger logger; @Test public void Collection_add() { colItems.add("new Item"); assertEquals(5, colItems.size()); logger.info("The arraycolItems contains the following elements: " + colItems); } @After public void Collection_clear() { colItems.clear(); assertTrue(colItems.isEmpty()); } @Test public void Collection_contains() { assertTrue(colItems.contains("Item1")); assertFalse(colItems.contains("Item5")); } @Test public void Collection_getSize() { assertEquals(4, colItems.size()); } @Test public void Collection_isEmpty() { assertFalse(colItems.isEmpty()); } @Test public void Collection_loop_via_for() { logger.info("Retrieving items using for loop"); for (String str : colItems) { logger.info("Item is: " + str); } } @Test public void Collection_loop_via_foreach_java8() { logger.info("Retrieving items using Java 8 Stream"); colItems.forEach((item) -> { logger.info(item); }); } @Test public void Collection_loop_via_iterator_next() { logger.info("Retrieving items using iterator"); for (Iterator<String> it = colItems.iterator(); it.hasNext();) { logger.info("Item is: " + it.next()); } } @Test public void Collection_loop_via_iterator_while() { Iterator<String> it = colItems.iterator(); logger.info("Retrieving items using iterator"); while (it.hasNext()) { logger.info("Item is: " + it.next()); } } @Test public void Collection_remove() { boolean removed = colItems.remove("Item1"); assertTrue(removed); assertEquals(3, colItems.size()); colItems.remove("Item3"); assertEquals(2, colItems.size()); } @Test public void Collection_remove_via_iterator_remove() { logger.info("Removing items using iterator"); for (Iterator<String> it = colItems.iterator(); it.hasNext();) { String item = it.next(); logger.info("Item is: " + item); it.remove(); assertFalse(colItems.contains(item)); } assertTrue(colItems.isEmpty()); } @Test public void Collection_toArray_default_java11() { String[] simpleArray = colItems.toArray(new String[colItems.size()]); logger.info("The array created after the conversion of our arraycolItems is: " + Arrays.toString(simpleArray)); String[] testArr = colItems.toArray(size -> new String[size]); logger.info("default toArray(intfunction):" + Arrays.toString(testArr)); } }
Note: the colItems
variable is a type of Collection<String>
4.2 CollectionBase_POJOTest
In this step, I will create a CollectionBase_POJOTest
class which has the same methods as the CollectionBase_StringTest
class but with the SomeData
type.
CollectionBase_POJOTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertFalse; import static org.junit.Assert.assertTrue; import java.util.Arrays; import java.util.Collection; import java.util.Iterator; import org.junit.After; import org.junit.FixMethodOrder; import org.junit.Test; import org.junit.runners.MethodSorters; import org.slf4j.Logger; import jcg.zheng.demo.data.SomeData; @FixMethodOrder(MethodSorters.NAME_ASCENDING) public abstract class CollectionBase_POJOTest { Collection<SomeData> list; protected Logger logger; protected SomeData buildSomeData(int id, String name) { SomeData sd = new SomeData(); sd.setId(id); sd.setName(name); return sd; } @Test public void Collection_add() { list.add(buildSomeData(1, "test")); assertEquals(5, list.size()); logger.info("The arraylist contains the following elements: " + list); } @After public void Collection_clear() { list.clear(); assertTrue(list.isEmpty()); } @Test public void Collection_contains() { assertTrue(list.contains(buildSomeData(1, "Item1"))); assertFalse(list.contains(buildSomeData(5, "Item5"))); } @Test public void Collection_getSize() { assertEquals(4, list.size()); } @Test public void Collection_isEmpty() { assertFalse(list.isEmpty()); } @Test public void Collection_loop_via_for() { logger.info("Retrieving items using for loop"); for (SomeData str : list) { logger.info("Item is: " + str); } } @Test public void Collection_loop_via_foreach_lambda() { logger.info("Retrieving items using Java 8 Stream"); list.forEach((item) -> { logger.info(item.toString()); }); } @Test public void Collection_loop_via_iterator_for() { logger.info("Retrieving items using iterator"); for (Iterator<SomeData> it = list.iterator(); it.hasNext();) { logger.info("Item is: " + it.next().toString()); } } @Test public void Collection_loop_via_iterator_while() { Iterator<SomeData> it = list.iterator(); logger.info("Retrieving items using iterator"); while (it.hasNext()) { logger.info("Item is: " + it.next().toString()); } } @Test public void Collection_remove() { list.remove(buildSomeData(3, "Item3")); assertEquals(3, list.size()); } @Test public void Collection_toArray() { SomeData[] simpleArray = list.toArray(new SomeData[list.size()]); logger.info("The array created after the conversion of our arraylist is: " + Arrays.toString(simpleArray)); } }
Note: the list
variable is a type of Collection<SomeData>
4.3 ListBase_StringTest
The List interface extends from the Collection interface with additional methods. In this step, I will demonstrate how to add, remove, and retrieve at a given index position for a List
of String
. I will also demonstrate two Collections algorithms with the sort
and binarySearch
methods.
ListBase_StringTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.Collections; import java.util.List; import org.junit.Test; public abstract class ListBase_StringTest extends CollectionBase_StringTest { protected List<String> listItems; @Test public void Collections_sort_binarySearch() { listItems.add("Tom"); listItems.add("Mary"); listItems.add("Shan"); listItems.add("Zheng"); Collections.sort(listItems); assertEquals("Mary", listItems.get(0)); assertEquals("Shan", listItems.get(1)); assertEquals("Tom", listItems.get(2)); assertEquals("Zheng", listItems.get(3)); logger.info("Sorted List"); for (int i = 0; i < listItems.size(); i++) { logger.info("Index: " + i + " - Item: " + listItems.get(i)); } int foundTom = Collections.binarySearch(listItems, "Tom"); assertEquals(2, foundTom); } @Test public void List_can_add_get_remove_via_index() { listItems.add(0, "Mary"); assertEquals(1, listItems.size()); int pos = listItems.indexOf("Mary"); assertEquals(0, pos); String item0 = listItems.get(0); assertEquals("Mary", item0); listItems.set(0, "Zheng"); assertEquals("Zheng", listItems.get(0)); listItems.remove(0); assertTrue(listItems.isEmpty()); } @Test public void List_copyOf_java10() { listItems.add("JCG"); listItems.add("Demo"); List<String> copiedValues = List.copyOf(listItems); assertEquals(2, copiedValues.size()); } @Test public void List_loop_via_for_get_index() { listItems.add("Tom"); listItems.add("Mary"); listItems.add("Shan"); listItems.add("Zheng"); logger.info("Retrieving items with loop using index and size list"); for (int i = 0; i < listItems.size(); i++) { logger.info("Index: " + i + " - Item: " + listItems.get(i)); } } @Test public void List_of_java9() { List<String> emptyList = List.of(); assertTrue(emptyList.isEmpty()); List<String> itemList = List.of("Mary", "Zheng", "Developer"); assertEquals(3, itemList.size()); } }
Note: the listItems
variable is a type of List<String>
5. Test Classes
In this step, I will create test classes for the commonly used Collection
classes. Each test class is extends from one of the three abstract test classes with its unique operations. The test method is named as {$InterfaceName}_{$MethodName}
.
5.1 ArrayList_StringTest
ArrayList implements List, Serializable, Cloneable, Iterable, Collection, and RandomAccess interfaces. It is used to store dynamically sized collection of elements.
In this step, I will create an ArrayList_StringTest
class which extends from ListBase_StringTest
.
In the setup_list_with_4_items
method, it will create an ArrayList
instance and add four items. This method is also annotated with @Before
annotation so it will be called for each test method.
In the ArrayList_clone
method, it will clone an array list.
ArrayList_StringTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.ArrayList; import org.junit.Before; import org.junit.Test; import org.slf4j.LoggerFactory; public class ArrayList_StringTest extends ListBase_StringTest { @SuppressWarnings("unchecked") @Test public void Object_clone() { ArrayList<String> arrList = (ArrayList<String>) listItems; arrList.add("Mary"); arrList.add("JCG"); ArrayList<String> cloneArr = (ArrayList<String>) arrList.clone(); assertEquals(2, cloneArr.size()); } @Before public void setup_list_with_4_items() { logger = LoggerFactory.getLogger(this.getClass()); colItems = new ArrayList<String>(); assertTrue(colItems.isEmpty()); colItems.add("Item1"); assertEquals(1, colItems.size()); colItems.add("Item2"); assertEquals(2, colItems.size()); colItems.add("Item3"); assertEquals(3, colItems.size()); colItems.add("Item4"); assertEquals(4, colItems.size()); listItems = new ArrayList<String>(); listItems.addAll(colItems); assertEquals(4, listItems.size()); listItems.clear(); } }
Execute mvn test -Dtest=ArrayList_StringTest
Output
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.ArrayList_StringTest 05:19:33.334 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Retrieving items with loop using index and size list 05:19:33.377 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Index: 0 - Item: Tom 05:19:33.377 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Index: 1 - Item: Mary 05:19:33.377 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Index: 2 - Item: Shan 05:19:33.377 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Index: 3 - Item: Zheng 05:19:33.381 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Sorted List 05:19:33.383 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Index: 0 - Item: Mary 05:19:33.384 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Index: 1 - Item: Shan 05:19:33.384 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Index: 2 - Item: Tom 05:19:33.390 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Index: 3 - Item: Zheng 05:19:33.417 [main] INFO jcg.zheng.demo.ArrayList_StringTest - The arraycolItems contains the following elements: [Item1, Item2, Item3, Item4, new Item] 05:19:33.425 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Retrieving items using for loop 05:19:33.426 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item1 05:19:33.427 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item2 05:19:33.427 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item3 05:19:33.427 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item4 05:19:33.428 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Retrieving items using Java 8 Stream 05:19:33.431 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item1 05:19:33.431 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item2 05:19:33.431 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item3 05:19:33.431 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item4 05:19:33.432 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Retrieving items using iterator 05:19:33.432 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item1 05:19:33.433 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item2 05:19:33.433 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item3 05:19:33.433 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item4 05:19:33.434 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Retrieving items using iterator 05:19:33.435 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item1 05:19:33.436 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item2 05:19:33.437 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item3 05:19:33.437 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item4 05:19:33.445 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Removing items using iterator 05:19:33.446 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item1 05:19:33.446 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item2 05:19:33.447 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item3 05:19:33.448 [main] INFO jcg.zheng.demo.ArrayList_StringTest - Item is: Item4 05:19:33.455 [main] INFO jcg.zheng.demo.ArrayList_StringTest - The array created after the conversion of our arraycolItems is: [Item1, Item2, Item3, Item4] 05:19:33.458 [main] INFO jcg.zheng.demo.ArrayList_StringTest - default toArray(intfunction):[Item1, Item2, Item3, Item4] Tests run: 17, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.526 sec Results : Tests run: 17, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 11.850 s [INFO] Finished at: 2019-08-04T05:19:33-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\java-collections-demo>
5.2 LinkedList_StringTest
LinkedList implements Serializable, Cloneable, Iterable, Collection, List and Queue interfaces. It models the linked-list data structure.
In this step, I will create a LinkedList_StringTest
class which extends from ListBase_StringTest
.
In the setup_colItem_with_4_items
method, it will create a LinkedList
instance and add four items. This method is also annotated with @Before
annotation so it will be called for each test method.
In the DeQue_peekFirst_peekLast
method, it will find the first element and last element from the DeQue
interface’s methods.
In the DeQue_peek
method, it will find the head element from Deque
‘s method.
LinkedList_StringTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.LinkedList; import org.junit.Before; import org.junit.Test; import org.slf4j.LoggerFactory; public class LinkedList_StringTest extends ListBase_StringTest { @Test public void DeQue_peekFirst_peekLast() { LinkedList<String> linkedLst = (LinkedList<String>) listItems; linkedLst.add("Mary"); linkedLst.add("Zheng"); assertEquals("Mary", linkedLst.peekFirst()); assertEquals("Zheng", linkedLst.peekLast()); assertEquals("Mary", linkedLst.peek()); } @Test public void DeQue_peek() { LinkedList<String> linkedLst = (LinkedList<String>) listItems; linkedLst.add("Mary"); linkedLst.add("Zheng"); assertEquals("Mary", linkedLst.peek()); } @Before public void setup_colItems_with_4_items() { logger = LoggerFactory.getLogger(this.getClass()); colItems = new LinkedList<String>(); assertTrue(colItems.isEmpty()); colItems.add("Item1"); assertEquals(1, colItems.size()); colItems.add("Item2"); assertEquals(2, colItems.size()); colItems.add("Item3"); assertEquals(3, colItems.size()); colItems.add("Item4"); assertEquals(4, colItems.size()); listItems = new LinkedList<String>(); listItems.addAll(colItems); assertEquals(4, listItems.size()); listItems.clear(); } }
Execute mvn test -Dtest=LinkedList_StringTest
Output
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.LinkedList_StringTest 05:22:22.802 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Retrieving items with loop using index and size list 05:22:22.865 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Index: 0 - Item: Tom 05:22:22.866 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Index: 1 - Item: Mary 05:22:22.867 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Index: 2 - Item: Shan 05:22:22.869 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Index: 3 - Item: Zheng 05:22:22.873 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Sorted List 05:22:22.875 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Index: 0 - Item: Mary 05:22:22.876 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Index: 1 - Item: Shan 05:22:22.877 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Index: 2 - Item: Tom 05:22:22.878 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Index: 3 - Item: Zheng 05:22:22.908 [main] INFO jcg.zheng.demo.LinkedList_StringTest - The arraycolItems contains the following elements: [Item1, Item2, Item3, Item4, new Item] 05:22:22.914 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Retrieving items using for loop 05:22:22.915 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item1 05:22:22.917 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item2 05:22:22.918 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item3 05:22:22.918 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item4 05:22:22.920 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Retrieving items using Java 8 Stream 05:22:22.921 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item1 05:22:22.922 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item2 05:22:22.922 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item3 05:22:22.922 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item4 05:22:22.922 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Retrieving items using iterator 05:22:22.923 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item1 05:22:22.923 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item2 05:22:22.924 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item3 05:22:22.925 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item4 05:22:22.925 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Retrieving items using iterator 05:22:22.926 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item1 05:22:22.926 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item2 05:22:22.926 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item3 05:22:22.926 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item4 05:22:22.930 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Removing items using iterator 05:22:22.930 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item1 05:22:22.931 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item2 05:22:22.931 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item3 05:22:22.932 [main] INFO jcg.zheng.demo.LinkedList_StringTest - Item is: Item4 05:22:22.934 [main] INFO jcg.zheng.demo.LinkedList_StringTest - The array created after the conversion of our arraycolItems is: [Item1, Item2, Item3, Item4] 05:22:22.936 [main] INFO jcg.zheng.demo.LinkedList_StringTest - default toArray(intfunction):[Item1, Item2, Item3, Item4] Tests run: 18, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.726 sec Results : Tests run: 18, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 13.643 s
5.3 Vector_StringTestBase
Vector implements Serializable, Cloneable, Iterable, Collection, List, and RandomAccess interfaces. It implements a grow-able array of objects.
In this step, I will create a Vector_StringTest
class which extends from ListBase_StringTest
.
In the setup_colItems_with_4_items
method, it will create a Vector
instance and add four items. This method is also annotated with @Before
annotation so it will be called for each test method.
In the Vector_firstElement_lastElement
method, it will find the first and last elements.
Vector_StringTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.Vector; import org.junit.Before; import org.junit.Test; import org.slf4j.LoggerFactory; public class Vector_StringTest extends ListBase_StringTest { @Before public void setup_colItems_with_4_items() { logger = LoggerFactory.getLogger(this.getClass()); colItems = new Vector<String>(); assertTrue(colItems.isEmpty()); colItems.add("Item1"); assertEquals(1, colItems.size()); colItems.add("Item2"); assertEquals(2, colItems.size()); colItems.add("Item3"); assertEquals(3, colItems.size()); colItems.add("Item4"); assertEquals(4, colItems.size()); listItems = new Vector<String>(); listItems.addAll(colItems); assertEquals(4, listItems.size()); listItems.clear(); } @Test public void Vector_firstElement_lastElement() { Vector<String> vectorObj = (Vector<String>) listItems; vectorObj.add("Mary"); vectorObj.add("Zheng"); assertEquals("Mary", vectorObj.firstElement()); assertEquals("Zheng", vectorObj.lastElement()); } }
Execute mvn test -Dtest=Vector_StringTest
Output
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.Vector_StringTest 05:25:12.397 [main] INFO jcg.zheng.demo.Vector_StringTest - Retrieving items with loop using index and size list 05:25:12.443 [main] INFO jcg.zheng.demo.Vector_StringTest - Index: 0 - Item: Tom 05:25:12.443 [main] INFO jcg.zheng.demo.Vector_StringTest - Index: 1 - Item: Mary 05:25:12.443 [main] INFO jcg.zheng.demo.Vector_StringTest - Index: 2 - Item: Shan 05:25:12.443 [main] INFO jcg.zheng.demo.Vector_StringTest - Index: 3 - Item: Zheng 05:25:12.447 [main] INFO jcg.zheng.demo.Vector_StringTest - Sorted List 05:25:12.448 [main] INFO jcg.zheng.demo.Vector_StringTest - Index: 0 - Item: Mary 05:25:12.448 [main] INFO jcg.zheng.demo.Vector_StringTest - Index: 1 - Item: Shan 05:25:12.449 [main] INFO jcg.zheng.demo.Vector_StringTest - Index: 2 - Item: Tom 05:25:12.449 [main] INFO jcg.zheng.demo.Vector_StringTest - Index: 3 - Item: Zheng 05:25:12.488 [main] INFO jcg.zheng.demo.Vector_StringTest - The arraycolItems contains the following elements: [Item1, Item2, Item3, Item4, new Item] 05:25:12.493 [main] INFO jcg.zheng.demo.Vector_StringTest - Retrieving items using for loop 05:25:12.498 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item1 05:25:12.498 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item2 05:25:12.498 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item3 05:25:12.498 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item4 05:25:12.500 [main] INFO jcg.zheng.demo.Vector_StringTest - Retrieving items using Java 8 Stream 05:25:12.501 [main] INFO jcg.zheng.demo.Vector_StringTest - Item1 05:25:12.502 [main] INFO jcg.zheng.demo.Vector_StringTest - Item2 05:25:12.502 [main] INFO jcg.zheng.demo.Vector_StringTest - Item3 05:25:12.502 [main] INFO jcg.zheng.demo.Vector_StringTest - Item4 05:25:12.503 [main] INFO jcg.zheng.demo.Vector_StringTest - Retrieving items using iterator 05:25:12.504 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item1 05:25:12.504 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item2 05:25:12.504 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item3 05:25:12.504 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item4 05:25:12.505 [main] INFO jcg.zheng.demo.Vector_StringTest - Retrieving items using iterator 05:25:12.507 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item1 05:25:12.508 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item2 05:25:12.508 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item3 05:25:12.508 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item4 05:25:12.516 [main] INFO jcg.zheng.demo.Vector_StringTest - Removing items using iterator 05:25:12.517 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item1 05:25:12.517 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item2 05:25:12.518 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item3 05:25:12.518 [main] INFO jcg.zheng.demo.Vector_StringTest - Item is: Item4 05:25:12.522 [main] INFO jcg.zheng.demo.Vector_StringTest - The array created after the conversion of our arraycolItems is: [Item1, Item2, Item3, Item4] 05:25:12.524 [main] INFO jcg.zheng.demo.Vector_StringTest - default toArray(intfunction):[Item1, Item2, Item3, Item4] Tests run: 17, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.518 sec Results : Tests run: 17, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 01:09 min [INFO] Finished at: 2019-08-04T05:25:12-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\java-collections-demo>
5.4 Stack_StringTest
Stack extends from Vector and implements List interface. It models the Stack data structure.
In this step, I will create a Stack_StringTest
class which extends from ListBase_StringTest
.
In the setup_colItems_with_4_items
method, it will create a Stack
instance and add four items. This method is also annotated with @Before
annotation so it will be called for each test method.
In the Stack_push_pop method
, it will push an element and pop an element based on first-in-last-out order.
Stack_StringTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.Stack; import org.junit.Before; import org.junit.Test; import org.slf4j.LoggerFactory; public class Stack_StringTest extends ListBase_StringTest { @Before public void setup_colItems_with_4_items() { logger = LoggerFactory.getLogger(this.getClass()); colItems = new Stack<String>(); assertTrue(colItems.isEmpty()); colItems.add("Item1"); assertEquals(1, colItems.size()); colItems.add("Item2"); assertEquals(2, colItems.size()); colItems.add("Item3"); assertEquals(3, colItems.size()); colItems.add("Item4"); assertEquals(4, colItems.size()); listItems = new Stack<String>(); listItems.addAll(colItems); assertEquals(4, listItems.size()); listItems.clear(); } @Test public void Stack_push_pop() { ((Stack<String>) listItems).push("Mary"); String item = ((Stack<String>) listItems).pop(); assertEquals("Mary", item); } }
Execute mvn test -Dtest=Stack_StringTest
Output
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.Stack_StringTest 05:26:53.868 [main] INFO jcg.zheng.demo.Stack_StringTest - Retrieving items with loop using index and size list 05:26:53.933 [main] INFO jcg.zheng.demo.Stack_StringTest - Index: 0 - Item: Tom 05:26:53.934 [main] INFO jcg.zheng.demo.Stack_StringTest - Index: 1 - Item: Mary 05:26:53.935 [main] INFO jcg.zheng.demo.Stack_StringTest - Index: 2 - Item: Shan 05:26:53.936 [main] INFO jcg.zheng.demo.Stack_StringTest - Index: 3 - Item: Zheng 05:26:53.939 [main] INFO jcg.zheng.demo.Stack_StringTest - Sorted List 05:26:53.940 [main] INFO jcg.zheng.demo.Stack_StringTest - Index: 0 - Item: Mary 05:26:53.942 [main] INFO jcg.zheng.demo.Stack_StringTest - Index: 1 - Item: Shan 05:26:53.943 [main] INFO jcg.zheng.demo.Stack_StringTest - Index: 2 - Item: Tom 05:26:53.943 [main] INFO jcg.zheng.demo.Stack_StringTest - Index: 3 - Item: Zheng 05:26:53.973 [main] INFO jcg.zheng.demo.Stack_StringTest - The arraycolItems contains the following elements: [Item1, Item2, Item3, Item4, new Item] 05:26:53.975 [main] INFO jcg.zheng.demo.Stack_StringTest - Retrieving items using for loop 05:26:53.977 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item1 05:26:53.978 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item2 05:26:53.978 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item3 05:26:53.978 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item4 05:26:53.981 [main] INFO jcg.zheng.demo.Stack_StringTest - Retrieving items using Java 8 Stream 05:26:53.984 [main] INFO jcg.zheng.demo.Stack_StringTest - Item1 05:26:53.984 [main] INFO jcg.zheng.demo.Stack_StringTest - Item2 05:26:53.984 [main] INFO jcg.zheng.demo.Stack_StringTest - Item3 05:26:53.984 [main] INFO jcg.zheng.demo.Stack_StringTest - Item4 05:26:53.985 [main] INFO jcg.zheng.demo.Stack_StringTest - Retrieving items using iterator 05:26:53.986 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item1 05:26:53.986 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item2 05:26:53.986 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item3 05:26:53.986 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item4 05:26:53.987 [main] INFO jcg.zheng.demo.Stack_StringTest - Retrieving items using iterator 05:26:53.988 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item1 05:26:53.988 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item2 05:26:53.988 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item3 05:26:53.988 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item4 05:26:53.994 [main] INFO jcg.zheng.demo.Stack_StringTest - Removing items using iterator 05:26:53.995 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item1 05:26:53.996 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item2 05:26:53.996 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item3 05:26:53.996 [main] INFO jcg.zheng.demo.Stack_StringTest - Item is: Item4 05:26:53.997 [main] INFO jcg.zheng.demo.Stack_StringTest - The array created after the conversion of our arraycolItems is: [Item1, Item2, Item3, Item4] 05:26:53.999 [main] INFO jcg.zheng.demo.Stack_StringTest - default toArray(intfunction):[Item1, Item2, Item3, Item4] Tests run: 18, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.616 sec Results : Tests run: 18, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 10.761 s [INFO] Finished at: 2019-08-04T05:26:54-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\java-collections-demo>
5.5 ArrayList_POJOTest
In this step, I will create an ArrayList_POJOTest
class which extends from CollectionBase_POJOTest
.
In the setup_list_with_4_items
method, it will create an ArrayList
instance and add four items. This method is also annotated with @Before
annotation so it will be called for each test method.
ArrayList_POJOTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.ArrayList; import org.junit.Before; import org.slf4j.LoggerFactory; import jcg.zheng.demo.data.SomeData; public class ArrayList_POJOTest extends CollectionBase_POJOTest { @Before public void setup_list_with_4_items() { logger = LoggerFactory.getLogger(this.getClass()); list = new ArrayList<SomeData>(); assertTrue(list.isEmpty()); list.add(buildSomeData(1, "Item1")); assertEquals(1, list.size()); list.add(buildSomeData(2, "Item2")); assertEquals(2, list.size()); list.add(buildSomeData(3, "Item3")); assertEquals(3, list.size()); list.add(buildSomeData(4, "Item4")); assertEquals(4, list.size()); } }
Execute mvn test -Dtest=ArrayList_POJOTest
Output
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.ArrayList_POJOTest 05:28:57.110 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - The arraylist contains the following elements: [SomeData [name=Item1, id=1], SomeData [name=Item2, id=2], SomeData [name=Item3, id=3], SomeData [name=Item4, id=4], SomeData [name=test, id=1]] 05:28:57.127 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Retrieving items using for loop 05:28:57.129 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Item is: SomeData [name=Item1, id=1] 05:28:57.130 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Item is: SomeData [name=Item2, id=2] 05:28:57.130 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Item is: SomeData [name=Item3, id=3] 05:28:57.131 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Item is: SomeData [name=Item4, id=4] 05:28:57.133 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Retrieving items using Java 8 Stream 05:28:57.138 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - SomeData [name=Item1, id=1] 05:28:57.139 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - SomeData [name=Item2, id=2] 05:28:57.140 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - SomeData [name=Item3, id=3] 05:28:57.140 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - SomeData [name=Item4, id=4] 05:28:57.143 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Retrieving items using iterator 05:28:57.145 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Item is: SomeData [name=Item1, id=1] 05:28:57.145 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Item is: SomeData [name=Item2, id=2] 05:28:57.145 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Item is: SomeData [name=Item3, id=3] 05:28:57.145 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Item is: SomeData [name=Item4, id=4] 05:28:57.147 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Retrieving items using iterator 05:28:57.148 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Item is: SomeData [name=Item1, id=1] 05:28:57.148 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Item is: SomeData [name=Item2, id=2] 05:28:57.149 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Item is: SomeData [name=Item3, id=3] 05:28:57.149 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - Item is: SomeData [name=Item4, id=4] 05:28:57.157 [main] INFO jcg.zheng.demo.ArrayList_POJOTest - The array created after the conversion of our arraylist is: [SomeData [name=Item1, id=1], SomeData [name=Item2, id=2], SomeData [name=Item3, id=3], SomeData [name=Item4, id=4]] Tests run: 10, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.473 sec Results : Tests run: 10, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 10.397 s [INFO] Finished at: 2019-08-04T05:28:57-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\java-collections-demo>
5.6 HashSet_POJOTest
HashSet implements the Java Set interface. Java Set interface is implemented by HashSet, Treeset and other classes. It is used to store a collection of unique elements.
In this step, I will create a HashSet_POJOTest
class which extends from CollectionBase_POJOTest
.
In the setup_with_4_items
method, it will create a HashSet
instance and add four items. This method is also annotated with @Before
annotation so it will be called for each test method.
In the allow_null
method, it will add a null
object.
In the Set_no_duplicate
method, it will show that Set
does not allow any duplicate elements.
HashSet_POJOTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.HashSet; import java.util.Set; import org.junit.Before; import org.junit.Test; import org.slf4j.LoggerFactory; import jcg.zheng.demo.data.SomeData; public class HashSet_POJOTest extends CollectionBase_POJOTest { @Test public void allow_null() { Set<SomeData> setData = new HashSet<>(); setData.add(null); assertEquals(1, setData.size()); } @Test public void Set_no_duplicate() { Set<SomeData> setData = new HashSet<>(); setData.add(buildSomeData(1, "Tom")); setData.add(buildSomeData(3, "Mary")); setData.add(buildSomeData(2, "Shan")); setData.add(buildSomeData(2, "Shan")); setData.add(buildSomeData(4, "Zheng")); assertEquals(4, setData.size()); } @Before public void setup_with_4_items() { logger = LoggerFactory.getLogger(this.getClass()); list = new HashSet<SomeData>(); assertTrue(list.isEmpty()); list.add(buildSomeData(1, "Item1")); assertEquals(1, list.size()); list.add(buildSomeData(2, "Item2")); assertEquals(2, list.size()); list.add(buildSomeData(3, "Item3")); assertEquals(3, list.size()); list.add(buildSomeData(4, "Item4")); assertEquals(4, list.size()); } }
Execute mvn test -Dtest=HashSet_POJOTest
Output
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.HashSet_POJOTest 05:30:28.475 [main] INFO jcg.zheng.demo.HashSet_POJOTest - The arraylist contains the following elements: [SomeData [name=Item1, id=1], SomeData [name=Item2, id=2], SomeData [name=Item3, id=3], SomeData [name=Item4, id=4], SomeData [name=test, id=1]] 05:30:28.488 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Retrieving items using for loop 05:30:28.489 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Item is: SomeData [name=Item1, id=1] 05:30:28.489 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Item is: SomeData [name=Item2, id=2] 05:30:28.489 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Item is: SomeData [name=Item3, id=3] 05:30:28.490 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Item is: SomeData [name=Item4, id=4] 05:30:28.491 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Retrieving items using Java 8 Stream 05:30:28.494 [main] INFO jcg.zheng.demo.HashSet_POJOTest - SomeData [name=Item1, id=1] 05:30:28.496 [main] INFO jcg.zheng.demo.HashSet_POJOTest - SomeData [name=Item2, id=2] 05:30:28.497 [main] INFO jcg.zheng.demo.HashSet_POJOTest - SomeData [name=Item3, id=3] 05:30:28.497 [main] INFO jcg.zheng.demo.HashSet_POJOTest - SomeData [name=Item4, id=4] 05:30:28.498 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Retrieving items using iterator 05:30:28.500 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Item is: SomeData [name=Item1, id=1] 05:30:28.500 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Item is: SomeData [name=Item2, id=2] 05:30:28.500 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Item is: SomeData [name=Item3, id=3] 05:30:28.500 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Item is: SomeData [name=Item4, id=4] 05:30:28.502 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Retrieving items using iterator 05:30:28.503 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Item is: SomeData [name=Item1, id=1] 05:30:28.504 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Item is: SomeData [name=Item2, id=2] 05:30:28.504 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Item is: SomeData [name=Item3, id=3] 05:30:28.505 [main] INFO jcg.zheng.demo.HashSet_POJOTest - Item is: SomeData [name=Item4, id=4] 05:30:28.508 [main] INFO jcg.zheng.demo.HashSet_POJOTest - The array created after the conversion of our arraylist is: [SomeData [name=Item1, id=1], SomeData [name=Item2, id=2], SomeData [name=Item3, id=3], SomeData [name=Item4, id=4]] Tests run: 12, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.483 sec Results : Tests run: 12, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 10.608 s [INFO] Finished at: 2019-08-04T05:30:28-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\java-collections-demo>
5.7 TreeSet_POJOTest
TreeSet extends from AbstractSet which implements the Java Set interface. Objects in a TreeSet
are stored in a sorted and ascending order.
In this step, I will create a TreeSet_POJOTest
class which extends from CollectionBase_POJOTest
.
In the setup_with_4_items
method, it will create a TreeSet
instance and add four items. This method is also annotated with @Before
annotation so it will be called for each test method.
In the Set_no_duplicate_ordered
method, it will show that TreeSet
orders its unique elements.
TreeSet_POJOTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.Set; import java.util.TreeSet; import org.junit.Before; import org.junit.Test; import org.slf4j.LoggerFactory; import jcg.zheng.demo.data.SomeData; public class TreeSet_POJOTest extends CollectionBase_POJOTest { @Test public void Set_no_duplicate_ordered() { Set<SomeData> testSet = new TreeSet<>(); testSet.add(buildSomeData(1, "Tom")); testSet.add(buildSomeData(3, "Mary")); testSet.add(buildSomeData(2, "Shan")); testSet.add(buildSomeData(2, "Shan")); testSet.add(buildSomeData(4, "Zheng")); assertEquals(4, testSet.size()); logger.info("TreeSet is ordered"); testSet.forEach(item -> { logger.info(item.toString()); }); } @Before public void setup_with_4_items() { logger = LoggerFactory.getLogger(this.getClass()); list = new TreeSet<SomeData>(); assertTrue(list.isEmpty()); list.add(buildSomeData(1, "Item1")); assertEquals(1, list.size()); list.add(buildSomeData(2, "Item2")); assertEquals(2, list.size()); list.add(buildSomeData(3, "Item3")); assertEquals(3, list.size()); list.add(buildSomeData(4, "Item4")); assertEquals(4, list.size()); } }
Execute mvn test -Dtest=TreeSet_POJOTest
Output
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.TreeSet_POJOTest 05:31:47.416 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - TreeSet is ordered 05:31:47.472 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - SomeData [name=Mary, id=3] 05:31:47.472 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - SomeData [name=Shan, id=2] 05:31:47.472 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - SomeData [name=Tom, id=1] 05:31:47.473 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - SomeData [name=Zheng, id=4] 05:31:47.499 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - The arraylist contains the following elements: [SomeData [name=Item1, id=1], SomeData [name=Item2, id=2], SomeData [name=Item3, id=3], SomeData [name=Item4, id=4], SomeData [name=test, id=1]] 05:31:47.504 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Retrieving items using for loop 05:31:47.507 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Item is: SomeData [name=Item1, id=1] 05:31:47.508 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Item is: SomeData [name=Item2, id=2] 05:31:47.508 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Item is: SomeData [name=Item3, id=3] 05:31:47.508 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Item is: SomeData [name=Item4, id=4] 05:31:47.511 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Retrieving items using Java 8 Stream 05:31:47.514 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - SomeData [name=Item1, id=1] 05:31:47.515 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - SomeData [name=Item2, id=2] 05:31:47.515 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - SomeData [name=Item3, id=3] 05:31:47.516 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - SomeData [name=Item4, id=4] 05:31:47.517 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Retrieving items using iterator 05:31:47.518 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Item is: SomeData [name=Item1, id=1] 05:31:47.518 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Item is: SomeData [name=Item2, id=2] 05:31:47.518 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Item is: SomeData [name=Item3, id=3] 05:31:47.519 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Item is: SomeData [name=Item4, id=4] 05:31:47.520 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Retrieving items using iterator 05:31:47.522 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Item is: SomeData [name=Item1, id=1] 05:31:47.522 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Item is: SomeData [name=Item2, id=2] 05:31:47.523 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Item is: SomeData [name=Item3, id=3] 05:31:47.523 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - Item is: SomeData [name=Item4, id=4] 05:31:47.528 [main] INFO jcg.zheng.demo.TreeSet_POJOTest - The array created after the conversion of our arraylist is: [SomeData [name=Item1, id=1], SomeData [name=Item2, id=2], SomeData [name=Item3, id=3], SomeData [name=Item4, id=4]] Tests run: 11, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.489 sec Results : Tests run: 11, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 11.171 s [INFO] Finished at: 2019-08-04T05:31:47-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\java-collections-demo>
6. Summary
In this article, I covered the fundamental of Java Collections framework, which is part of the core java skillset. I created several test classes to demonstrate how to add, remove, retrieve, clear, sort, and search items from a collection of objects.
Please check here for more details about the Big-O differences among these interfaces and classes in the Java collection framework.
7. Related articles
8. Download the Source Code
This example consists of Maven project to demonstrate the Collections framework, which is part of the core java skillset.
You can download the full source code of this example here: Java Collections Tutorial