Java BufferedReader Class Example
In this article, we are going to learn about the BufferedReader class in Java, its uses and a simple program to showcase how to read data using BufferedReader and readline() method.
1. What is BufferedReader
BufferedReader
provides a way to seamlessly read characters from an Input Stream. InputStream
can be an instance of a File or System IO or a Socket. BufferedReader
improves the IO reading performance by maintaining an internal buffer. Internal buffer stores chunks of data and the Reader reads from it instead of reading directly from the underlying physical IO system.
2. BufferedReader constructors
BufferedReader
offers below overloaded constructors,
BufferedReader(Reader in)
– Creates a buffered input stream with default buffer sizeBufferedReader(Reader in, int size)
– Users buffer of specified size
Use the appropriate constructor based on your use case.
3. BufferedReader methods
In this section, I am going to list some of the useful methods that are part of BufferedReader
class.
Type | Method Name | Description |
---|---|---|
int | read() | Reads a single character and returns the character as an integer |
int | read(char[] buffer, int offset, int length) | Reads characters into an array buffer – destination array offset – start point at which characters to be stored length – maximum number of character to read |
String | readLine() | BufferedReader readline() method reads a line of text. End of line is identified by a newline or a carriage return character |
long | skip(int n) | Skips number of characters specified and returns the actual number of characters skipped |
boolean | ready() | Tells whether the steam is ready to be read |
void | mark(int readAheadLimit) | Marks the present position. the parameter passed limits the number of characters read |
boolean | markSupported() | Tells whether the stream supports mark operation |
void | reset() | Resets buffer to recent mark |
void | close() | Closes the stream and any resources associated with it |
Stream<String> | lines() | Returns a stream, elements of which are read from this BufferedReader |
4. Java BufferedReader Class Example
4.1 Reading from the console
In this example, I am going to show how you can read from the console using the BufferedReader
class.
BufferedReaderConsoleRead.java
package com.jcg; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; public class BufferedReaderConsoleRead { public static void main(String[] args) throws IOException { //Enter data to BufferedReader from console System.out.println("Enter your name : "); BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); // Reading data using readLine String name = reader.readLine(); // Printing the read line System.out.println("Hello " + name + "!!!"); } }
BufferedReader
takes a Reader
as input. Here we are passing System.in
to indicate receive input from the console. Method readline()
reads the data from the buffer when the user presses the enter key. Below is the output from the program,
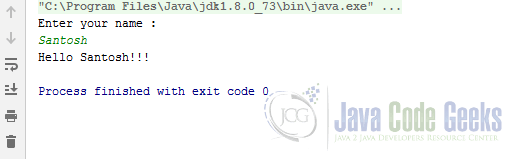
4.2 Reading from a file
In this example, I am going to show how you can read from a file line by line.
BufferedReaderFileRead.java
package com.jcg; import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class BufferedReaderFileRead { public static void main(String[] args) throws IOException { //Enter data to BufferedReader from a file String line = null; System.out.println("Reading fom file"); BufferedReader reader = new BufferedReader(new FileReader(System.getProperty("user.dir") + "\\src\\com\\jcg\\test-file.txt")); // Reading data using readLine while ((line = reader.readLine()) != null) { // Printing the read line System.out.println(line); } System.out.println("Finished reading file"); } }
Here we are passing an instance of a FileReader
. The while loop iterates till we reach end of the file. The output of the program is as below,
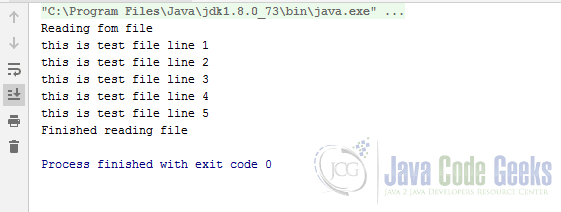
Both examples use default buffer size. If you would like to specify your own buffer size then use the other variety of constructor as below,
BufferedReader reader = new BufferedReader(System.in, 1024);
1024 is the size of buffer you are intending to use.
5. When to use BufferedReader
BufferedReader
is a preferred choice when we have to read from a long stream of Strings. BufferedReader
can read in chunks of data, it is efficient as compared to Scanner
class.
BufferedReader
is thread-safe. Hence it is suitable in the multi-threaded environment as compared to the Scanner
.
As compared to Scanner
, BufferedReader
is fast and less CPU intensive.
6. Download the source code
For the demo program, I am using JDK11 and IntelliJ Idea IDE.
You can download the full source code of this example here: Java BufferedReader Class Example
You neither close the BufferedReader nor use trx-wirh-resource.
Just to not bully your readers with error handling code?
Thank Bernd for pointing this out.
To everyone’s note,
you need to close all the opened BufferedReader to keep yourself running out of resources.