Integer Java Class Example
In this article, we will learn about the Integer Java class. We will discuss some of the important methods, like the integer.parseint method (Java parseint method), the toString(), the intValue(), the toUnsignedString(), the toHexString(), the toOctalString(), the toBinaryString(), the valueOf(), and the decode().
1. Introduction
The Integer Java class wraps a value of the primitive type int
in an object. An object of type Integer contains a single field whose type is int
. In addition, this class provides several methods for converting an int
to a String
and a String
to an int
, as well as other constants and methods useful when dealing with an int.
2. Class Signature
java.lang.Integer
is a final class which extends java.lang.Number
and implements java.lang.Comparable
public final class Integer extends Number implements Comparable<Integer>
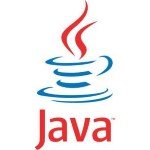
2.1 Constructor
In this section, we will discuss the constructors of Integer
class. Integer
class has two constructors.
The first one constructs a newly allocated Integer
object that represents the specified int
value.
Integer myIntegerObject1 = new Integer(10);
There is another constructor which takes String
as a parameter. It constructs a newly allocated Integer
object that represents the int
value indicated by the String
parameter. The string is converted to an int value in exactly the manner used by the parseInt method for radix 10.
Integer myIntegerObject2 = new Integer("10");
2.2 Fields
In this section, we will discuss some of the fields available in the Integer
class. The first two are the min and max values. MIN_VALUE represents a constant holding the minimum value an int can have. MAX_VALUE represents a constant holing the maximum value an int can have.
System.out.println("Integer minimun value: " + Integer.MIN_VALUE); System.out.println("Integer maximum value: " + Integer.MAX_VALUE);
If we run the above code we will see the output as below:
Integer minimun value: -2147483648 Integer maximum value: 2147483647
2.3 Methods
In this section, we will discuss some of the important methods in Integer
class.
2.3.1 toString()
In this section, we will look into the toString()
methods available in the Integer
class.
public static String toString(int i, int radix)
This method returns a string representation of the first argument in the radix specified by the second argument. If the radix is smaller than Character.MIN_RADIX
or larger than Character.MAX_RADIX
, then the radix 10 is used instead.
If the first argument is negative, the first element of the result is the ASCII minus character ‘-‘ (‘\u002D
‘). If the first argument is not negative, no sign character appears in the result.
The remaining characters of the result represent the magnitude of the first argument. If the magnitude is zero, it is represented by a single zero character ‘0’ (‘\u0030
‘); otherwise, the first character of the representation of the magnitude will not be the zero character. The following ASCII characters are used as digits: 0123456789abcdefghijklmnopqrstuvwxyz
These are ‘\u0030
‘ through ‘\u0039
‘ and ‘\u0061
‘ through ‘\u007A
‘.
If radix is N, then the first N of these characters are used as radix-N digits in the order shown. Thus, the digits for hexadecimal (radix 16) are 0123456789abcdef
. If uppercase letters are desired, the String.toUpperCase()
method may be called on the result.
Below is the code snippet of the method
if (radix Character.MAX_RADIX) radix = 10; /* Use the faster version */ if (radix == 10) { return toString(i); } char buf[] = new char[33]; boolean negative = (i < 0); int charPos = 32; if (!negative) { i = -i; } while (i <= -radix) { buf[charPos--] = digits[-(i % radix)]; i = i / radix; } buf[charPos] = digits[-i]; if (negative) { buf[--charPos] = '-'; } return new String(buf, charPos, (33 - charPos));
There is also an overloaded method which just takes one parameter. It returns a String
object representing the specified integer. The argument is converted to signed decimal representation and returned as a string, exactly as if the argument and radix 10 were given as arguments to the toString(int, int)
method.
2.3.2 toUnsignedString()
public static String toUnsignedString(int i, int radix)
This method returns a string representation of the first argument as an unsigned integer value in the radix specified by the second argument. If the radix is smaller than Character.MIN_RADIX
or larger than Character.MAX_RADIX
, then the radix 10 is used instead. Note that since the first argument is treated as an unsigned value, no leading sign character is printed. If the magnitude is zero, it is represented by a single zero character ‘0’ (‘\u0030
‘); otherwise, the first character of the representation of the magnitude will not be the zero character. The behavior of radixes and the characters used as digits are the same as toString.
There is another overloaded method which does not take the radix. It returns a string representation of the argument as an unsigned decimal value. The argument is converted to unsigned decimal representation and returned as a string exactly as if the argument and radix 10 were given as arguments to the toUnsignedString(int, int)
method.
2.3.3 toHexString()
This method returns a string representation of the integer argument as an unsigned integer in base 16. The unsigned integer value is the argument plus 232 if the argument is negative; otherwise, it is equal to the argument. This value is converted to a string of ASCII digits in hexadecimal (base 16) with no extra leading 0s. The value of the argument can be recovered from the returned string ‘s’ by calling Integer.parseUnsignedInt(s, 16)
.
If the unsigned magnitude is zero, it is represented by a single zero character ‘0’ (‘\u0030
‘); otherwise, the first character of the representation of the unsigned magnitude will not be the zero character. The following characters are used as hexadecimal digits: 0123456789abcdef
These are the characters ‘\u0030
‘ through ‘\u0039
‘ and ‘\u0061
‘ through ‘\u0066
‘. If uppercase letters are desired, the String.toUpperCase()
method may be called on the result: Integer.toHexString(n).toUpperCase()
System.out.println("Integer.toHexString(39) => " + Integer.toHexString(39));
The above code will give the below output:
Integer.toHexString(39) => 27
2.3.4 toOctalString()
This method returns a string representation of the integer argument as an unsigned integer in base 8. The unsigned integer value is the argument plus 232 if the argument is negative; otherwise, it is equal to the argument. This value is converted to a string of ASCII digits in octal (base 8) with no extra leading 0s.
The value of the argument can be recovered from the returned string s by calling Integer.parseUnsignedInt(s, 8)
.
System.out.println("Integer.toOctalString(39) => " + Integer.toOctalString(39));
The above code will give you the below output:
Integer.toOctalString(39) => 47
2.3.5 toBinaryString()
This method returns a string representation of the integer argument as an unsigned integer in base 2. The unsigned integer value is the argument plus 232 if the argument is negative; otherwise, it is equal to the argument. This value is converted to a string of ASCII digits in binary (base 2) with no extra leading 0s.
System.out.println("Integer.toBinaryString(39) => " + Integer.toBinaryString(39));
The above code will give the output as below:
Integer.toBinaryString(39) => 100111
2.3.6 parseInt()
In this section we will see an example of the parseint method in Java. This method parses the string argument as a signed decimal integer. The characters in the string must all be decimal digits, except that the first character may be an ASCII minus sign ‘-‘ (‘\u002D
‘) to indicate a negative value or an ASCII plus sign ‘+’ (‘\u002B
‘) to indicate a positive value. The resulting integer value is returned, exactly as if the argument and the radix 10 were given as arguments to the parseInt(String, int)
method.
System.out.println(" Integer.parseInt(45) => " + Integer.parseInt("45") );
The above code will give the output as below:
Integer.parseInt(45) => 45
We have another similar method; public static int parseUnsignedInt(String s, int radix)
. This method parses the string argument as an unsigned integer in the radix specified by the second argument. An unsigned integer maps the values usually associated with negative numbers to positive numbers larger than MAX_VALUE
. The characters in the string must all be digits of the specified radix (as determined by whether Character.digit(char, int)
returns a nonnegative value), except that the first character may be an ASCII plus sign ‘+’ (‘\u002B
‘). The resulting integer value is returned.
2.3.7 valueOf()
This method returns an Integer
object holding the value of the specified String
. The argument is interpreted as representing a signed decimal integer, exactly as if the argument were given to the parseInt(String)
method. The result is an Integer
object that represents the integer value specified by the string. In other words, this method returns an Integer object equal to the value of: new Integer( Integer.parseInt(s) )
System.out.println("Integer.valueOf(String 100) => " + Integer.valueOf("100")); System.out.println("Integer.valueOf(int 100) => " + Integer.valueOf(100)); System.out.println("Integer.valueOf(100, 8) => " + Integer.valueOf("100", 8));
The above code will give the out as below:
Integer.valueOf(String 100) => 100 Integer.valueOf(int 100) => 100 Integer.valueOf(100, 8) => 64
2.3.8 intValue()
There are some instance methods that return the byte, short, int, long, float and double value of the integer object.
Integer int1 = Integer.MAX_VALUE; System.out.println("Byte value" + int1.byteValue()); System.out.println("Double value" + int1.doubleValue()); System.out.println("Float value" + int1.floatValue()); System.out.println("Int value" + int1.intValue()); System.out.println("Long value" + int1.longValue()); System.out.println("Short value" + int1.shortValue());
Running the above code will give output as:
Byte value of 203-1 Double value of 2032.147483647E9 Float value of 2032.14748365E9 Int value of 2032147483647 Long value of 2032147483647 Short value of 203-1
2.3.9 decode()
This method decodes a String
into an Integer
. It accepts decimal, hexadecimal, and octal numbers. DecimalNumeral
, HexDigits
, and OctalDigits
are as defined in section 3.10.1 of The Java™ Language Specification, except that underscores are not accepted between digits. The sequence of characters following an optional sign and/or radix specifier (“0x”, “0X”, “#”, or leading zero) is parsed as by the Integer.parseInt method with the indicated radix (10, 16, or 8). This sequence of characters must represent a positive value or a NumberFormatException
will be thrown. The result is negated if the first character of the specified String is the minus sign. No whitespace characters are permitted in the String
.
System.out.println("Integer.decode(20) => " + Integer.decode("0x20"));
Running the above code will give the output as below:
Integer.decode(20) => 32
3. Summary
In this article, we learned about the java.lang.Integer
Integer Java Class. We looked at the types of ways of constructing the Integer
object. We also looked at some of the important static and instance methods and their usages.
4. More articles
- Java String Class Example (with video)
- String to Int Java Example (with video)
- Convert int to string Java Example (with video)
5. Download the source code
That was an example of the Java Integer Class.
You can download the full source code of this example here: Integer Java Class Example
Last updated on May 31st, 2021