Int Array Java Example
In this article, we will explain what arrays are in java and then we will create a Int Array Java Example.
An array is a container object that holds a fixed number of values of a single type. As the name indicates, an int array holds only int values. In this article, let us look at examples of int array and the means to update.
You can watch the following video and learn how to use arrays in Java:
1. Int array Java Example
An int array is an array of int values. The length of an array is established when the array is created. Let us look at an example to create, initialize and access an array:
IntArrayExample
public class IntArrayExample { public static void main(String args[]){ // declare an int array int[] intArr; // initialize an int array intArr = new int[5]; // assign some values intArr[0] = 100; intArr[1] = 200; intArr[2] = 300; for(int i=0;i<5;i++) System.out.println("at index "+i+" :"+intArr[i]); } }
at index 0 :100 at index 1 :200 at index 2 :300 at index 3 :0 at index 4 :0
An alternate way to create and initialize an int array is as shown below. In this case, the length of the array is determined by the number of values provided between braces and delimited by commas.
int[] anArray = { 100, 200, 300 };
We could also create a multi-dimensional array (an array of arrays) by using two or more sets of brackets, such as int[][] intArray.
2. Difference between int and Integer array
We know that int is a primitive with default value as 0. Integer is an object, a wrapper over int. An object of type Integer contains a single field whose type is int and can store null. The Integer class also provides methods and constants that can be used to deal with an int.
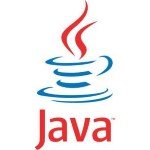
int[]
is an array of primitive int values, while Integer[] is an object array holding references to Integer objects. An Integer[] array can hold null as shown in the below example.
IntegerArrayExample
public class IntegerArrayExample{ public static void main(String args[]){ // declare an int array Integer[] intArr; // initialize an Integer array intArr = new Integer[5]; // assign some values intArr[0] = 100; intArr[1] = 200; intArr[2] = 300; for(int i=0;i<5;i++) System.out.println("at index "+i+" :"+intArr[i]); } }
at index 0 :100 at index 1 :200 at index 2 :300 at index 3 :null at index 4 :null
3. Access and change values in an Int array
Each item in the array is called an element, and each element is accessed by its numerical index. Let us look at an example to create, access and change values in an int array.
IntExample
public class IntExample{ public static void main(String args[]){ // declare an int array int[] intArr; // initialize an int array intArr = new int[3]; // assign some values intArr[0] = 100; intArr[1] = 200; intArr[2] = 300; // change values for(int i=0;i<3;i++) intArr[i] = intArr[i]+i; // updated array for(int i=0;i<3;i++) System.out.println("at index "+i+" :"+intArr[i]); } }
at index 0 :100 at index 1 :201 at index 2 :302
4. Download the Source Code
You can download the full source code of this example here: Int Array Java Example