Initialize Array Java Example
In this article, we are going to see how to initialize an array in Java. We will cover the following things in this article:
- What are Arrays in Java?
- Array Type
- Array Elements
- Creating an array variable
- Accessing array Elements
- Initialize array Java Example
You can watch the following video and learn how to use arrays in Java:
1. What are Arrays in Java
- In java programming language arrays are objects.
- Arrays can contain empty, one or more than one element in it. if arrays contain no element then it is said to be an empty array.
- Elements in array don’t have any name instead they are referenced by a non-negative number which is called index.
- An array of length n has indexed from 0 to n-1 to refer each element in it by a unique index.
- All element in an array is of the same type.
- The elements of an array may refer to another array(i.e. multidimensional array).
2. Array Type
The array type is used to declare which type of array is going to be created.
It is written followed by one or more number of empty pairs of square brackets []. The number of bracket pairs represents the depth or dimension of the array.
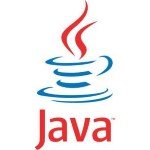
The element type of an array can be a primitive type or a reference type.
Arrays with an interface type as the element type are allowed. An element of such an array may have as its value a null reference or an instance of any type that implements the interface.
Arrays with an abstract class type as the element type are allowed. An element of such an array may have as its value a null reference or an instance of any subclass of the abstract class that is not itself abstract.
3. Array Elements
Array elements are the variable of array type holds a reference to an object. Declaring a variable of array type does not create an array object or allocate any space for array components. It creates only the variable itself, which can contain a reference to an array.
Declaring array varibles
int[] arrayOfIntegers; // array of int short[][] arrayOfArrayOfShorts; // array of array of short short arrayOfArrayOfShorts1[][]; // anotherarray of array of short Object[] arrayOfArrayOfObjects, // array of Object Collection[] arrayOfCollection; // array of Collection of unknown type
The initializer part of a declaration statement may create an array, a reference to which then becomes the initial value of the variable.
Declaring array varibles with innitilizer statement
Exception arrayofExceptions[] = new Exception[3]; // array of exceptions Object arrayOfArrayOfExceptions[][] = new Exception[2][3]; // array of array of exceptions int[] arrayofFactorialNumbers = { 1, 1, 2, 6, 24, 120, 720, 5040 }; // anotherarray of array of numbers char arrayofCharactars[] = { 'n', 'o', 't', ' ', 'a', ' ','S', 't', 'r', 'i', 'n', 'g' }; // array of charactars String[] arrayofStrings = { "array", "of", "String", }; // array of strings
The array type of a variable depends on the bracket pairs that may appear as part of the type at the beginning of a variable declaration, or as part of the declarator for the variable, or both. Specifically, in the declaration of a field (instance variable), formal parameter(method argument), or local variable.
It is not recommended to use “mixed notation” in array variable declarations, where –
- Bracket pairs appear on both the type and in declarators; nor in method declarations
- Bracket pairs appear both before and after the formal parameter list.
The local variable declaration
//The local variable declaration byte[] rowvector, colvector, matrix[]; //is equivalent to byte rowvector[], colvector[], matrix[][];
//The local variable declaration float[][] f[][], g[][][], h[]; //is equivalent to float[][][][] f; float[][][][][] g; float[][][] h;
//The local variable declaration int a, b[], c[][]; //is equivalent to int a; int[] b; int[][] c;
In all the above examples it is recommended to use the second approach instead of using the first approach which is very confusing and ambiguous.
4. Creating Array
As we saw in the above examples we can create an array by an array creation expression or an array initializer.
An array creation expression specifies –
- The element type.
- The number of levels of nested arrays.
- The length of the array for at least one of the levels of nesting.
The array’s length is available as a final
instance variable length
. An array initializer creates an array and provides initial values for all its components.
Creating Array
//array creation expression int arrayofIntegers[] = new int[10]; // array of 10 integers //array initializer int arrayofIntegers1[] = {1,2,3,4,5,6,7,8,9,10};//array of 10 integers with initialization
In the above example,
- element type is
int
- Level of the nested array is one
- length of the array is 10.
5. Accessing array Elements
Array elements are accessed by an array access expression that consists of an expression whose value is an array reference followed by an indexing expression enclosed by [
and ]
, as in A[i]
.
Arrays index are 0
-based. An array with length n can be indexed by 0
to n-1 integer numbers.
Example: Let’s create a program to calculate the sum of elements stored in an array.
Calculate sum of array elements
package com.javacodegeeks.examples.arrays; public class ArrayDemo1 { public static void main(String[] args) { int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; int sum = 0; for (int i = 0; i < numbers.length; i++) { sum = sum + numbers[i]; // accessing array element using number[i] System.out.println("Number at index " + i + " is : " + numbers[i]); // accessing array element using number[i] } System.out.println("Sum of elements are: " + sum); } }
Result
Number at index 0 is : 1 Number at index 1 is : 2 Number at index 2 is : 3 Number at index 3 is : 4 Number at index 4 is : 5 Number at index 5 is : 6 Number at index 6 is : 7 Number at index 7 is : 8 Number at index 8 is : 9 Number at index 9 is : 10 Sum of elements are: 55
6. Initialize Array Java Example
We have seen in previous sections that optionally we can initialize the array in the declaration statement. Here are a few things to remember-
- An array initializer is written as a comma-separated list of expressions, enclosed by braces
{
and}
.
//array initializer is written as a comma-separated list of values int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
- A trailing comma may appear after the last expression in an array initializer and is ignored.
//array initializer is written as a comma-separated list of values with trailing comma at the end is valid int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10,};
- Each variable initializer must be assignment-compatible with the array’s element type, otherwise, It will be a compile-time error occurs. Below example shows that “jack” is not an integer value so below statement is invalid and throws a compile-time error.
// "jack" is not an integer value so below statement is invalid int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, "jack"};
- Length of the array will be the number of values present in
{
and}
.
int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; System.out.println("Length of array is: " + numbers.length);
Result
Length of array is: 10
- The default initial value of elements of an array is 0 for numeric types and
false
forboolean
if we don’t initialize it. We can demonstrate this:
Default values of array elements
int[] age = new int[5]; System.out.println(age[0]); System.out.println(age[1]); System.out.println(age[2]); System.out.println(age[3]); System.out.println(age[4]); boolean[] boolArr = new boolean[2]; System.out.println(boolArr[0]); System.out.println(boolArr[1]);
Result
0 0 0 0 0 false false
7. Initializing multi-dimensional Array
As discussed in previous sections arrays can be of any dimension. Let’s see how initialization works in multi-dimensional arrays –
- We can create and initialize a 2-dimensional array with its default values-
Initializing the multi-dimensional array with default values
int[][] numbers = new int[2][2]; System.out.println(numbers[0][0]); System.out.println(numbers[0][1]); System.out.println(numbers[1][0]); System.out.println(numbers[1][1]);
Result
0 0 0 0 0
- We can also partially initialize multi-dimensional arrays at the time of declaration and later and initialize other dimensions later point of time. But we should remember we can’t use array before we assign all dimension of the array. Let’s see it by an example-
Partially Initializing multi-dimensional array with default values
//multidimensional array initialization with only leftmost dimension int[][] twoDimensionalArr = new int[2][]; System.out.println(twoDimensionalArr[1][2]); twoDimensionalArr[0] = new int[2]; twoDimensionalArr[1] = new int[3]; //complete initialization is required before we use the array
Result
Exception in thread "main" java.lang.NullPointerException at com.javacodegeeks.examples....
If we move the println
statement at the bottom it will execute without any error
Partially Initializing multi-dimensional array with default values
//multidimensional array initialization with only leftmost dimension int[][] twoDimensionalArr = new int[2][]; twoDimensionalArr[0] = new int[2]; twoDimensionalArr[1] = new int[3]; //complete initialization is required before we use the array System.out.println(twoDimensionalArr[1][2]);
Result
0
- We can use one shortcut method to initialize multi-dimensional array as given below-
Initializing multi-dimensional array by shortcut method
//Initializing multi-dimensional array by shortcut method int[][] twoDimensionalArr1 = {{1,2}, {1,2,3}}; System.out.println(twoDimensionalArr1[1][1]);
Result
2
In the above method of assignment, array size will be calculated dynamically and if we try to access any non-existent index it will throw ArrayIndexOfBoundException
.
IAccessing non-existent index in array
//Initializing multi-dimensional array by shortcut method int[][] twoDimensionalArr2 = {{0}}; System.out.println(twoDimensionalArr1[1][1]);
Result
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 1 at ...
- Below are some other variations of array initialization which are the incorrect way of assigning an array.
Incorrect way of assigning arrays
Result
//Since dimension is not provided here so it is an invalid syntax int[] a = new int[]; //invalid because leftmost dimension value is not provided int[][] aa = new int[][5];
compile time error
8. Bonus Points
There is a class in java.utils
named Arrays
which provides some utility methods to assign an array. Let’s take a look over these methods-
8.1 Arrays.fill()
The java.util.Arrays
class has several methods named fill() which accept different types of parameter. The specialty of this method is the It fills the whole array or some part of the array with the same value:
fill()
without one argument will fill all the elements of the array with the same value.
int array2[] = new int[5]; Arrays.fill(array2, 10); for (int i = 0; i < array2.length; i++) { System.out.println(array2[i]); }
Result
10 10 10 10 10
- there is one more variant of fill method which takes four arguments and fills the given array starting from the index given as the second argument till next n number which is given as 3rd argument and the last argument will be the value to be replaced. The element matching with the range will get filled with value give and others will be assigned their default values.
int array3[] = new int[5]; //it will fill arrays index starting from 0 onwards till three elements with value 5 Arrays.fill(array3, 0,3,5); for (int i = 0; i < array3.length; i++) { System.out.println(array3[i]); }
Result
5 5 5 0 0
8.2 Arrays.copyOf()
This method Arrays.copyOf() creates a new array by copying another array. It has many overloaded versions which accept different types of arguments.
Let’s see it with an example:
int array4[] = { 1, 2, 3, 4, 5 }; int[] copy = Arrays.copyOf(array4,5); for (int i = 0; i < array3.length; i++) { System.out.println(array4[i]); }
Result
1 2 3 4 5
A few points to remember about copyOf():
- The method accepts the source array and the length of the copy to be created.
- If the length is greater than the length of the array to be copied, then the extra elements will be initialized using their default values.
- If the source array has not been initialized, then a NullPointerException gets thrown.
- If the source array length is negative, then a NegativeArraySizeException is thrown.
8.3 Arrays.setAll()
The method Arrays.setAll()
accepts a generator function sets all elements of an array using the generated value by this generator function. It will also throw NullPointerException if the
generator function given is null. Lets see it by an example:
int[] array5 = new int[15]; Arrays.setAll(array5, p -> p > 9 ? 0 : p); for (int i = 0; i < array5.length; i++) { System.out.println(array5[i]); }
Result
0 1 2 3 4 5 6 7 8 9 0 0 0 0 0
9. Download the Source Code
That was an initialize array java example. Hope you enjoyed it.
You can download the full source code of this example here: Initialize Array Java Example